
Example for updating the MTi-1's firmware. Uses a platform independent, retargetable pure C implementation of the firmware updater protocol.
fwupdate.h
00001 /*! 00002 * \file 00003 * \copyright Copyright (C) Xsens Technologies B.V., 2015. 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 */ 00017 #ifndef FWUPDATE_H 00018 #define FWUPDATE_H 00019 00020 #include "xbusmessage.h " 00021 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif 00025 00026 #define FWU_REQUIRED_TXBUFFER_SIZE 300 00027 00028 /*! \brief Result value 00029 */ 00030 typedef enum 00031 { 00032 FWU_Success, 00033 FWU_Failed 00034 } FWU_Result; 00035 00036 00037 /*! \brief Definition of a xff section header 00038 */ 00039 typedef struct 00040 { 00041 uint32_t m_globalId; 00042 uint32_t m_sectionSize; 00043 uint8_t m_firmwareRevision[3]; 00044 uint8_t m_xffVersion[2]; 00045 uint8_t m_chipId; 00046 uint8_t m_numberOfSections; 00047 uint8_t m_addressLength; 00048 uint16_t m_pageSize; 00049 uint16_t m_sliceSize; 00050 } XffHeader; 00051 00052 00053 /*! \brief Internal state 00054 */ 00055 typedef enum 00056 { 00057 STATE_Idle, 00058 STATE_Start, 00059 STATE_WaitReady, 00060 STATE_WaitHeaderResult, 00061 STATE_WaitSliceReady, 00062 STATE_WaitPageOk, 00063 STATE_WaitPageReady 00064 } FWU_State; 00065 00066 00067 00068 /*! \brief FwUpdate object definition 00069 */ 00070 typedef struct 00071 { 00072 /* External dependencies. Host should fill in these members */ 00073 00074 /*! \brief Callback function by which FwUpdate requests for xff data 00075 \param buffer Target buffer in which the xff data should be written by the host 00076 \param offset Offset in the xff file where reading should start 00077 \param length Number of bytes which is requested 00078 \returns Number of bytes which is actually written to the buffer 00079 */ 00080 int (*m_readXffData)(uint8_t *buffer, int offset, int length); 00081 00082 /*! \brief Callback function via which FwUpdate can send xbus messages to the module 00083 \param xbusMessage Xbus message that should be send to the module 00084 */ 00085 void (*m_sendXbusMessage)(struct XbusMessage const* xbusMessage); 00086 00087 /*! \brief Callback function by which FwUpdate notifies the host that a firmware update has finished 00088 \param result FWU_Success or FWU_Failed 00089 */ 00090 void (*m_readyHandler)(FWU_Result result); 00091 00092 /*! \brief Memory needed by the FwUpdate. Host must allocate a block of memory 00093 of size FWU_REQUIRED_TXBUFFER_SIZE 00094 */ 00095 uint8_t *m_txBuffer; 00096 00097 /* State variables for internal use (the user must not touch these) */ 00098 FWU_State m_state; 00099 XffHeader m_xffHeader; 00100 int m_nofPages; 00101 int m_nofSlicesPerPage; 00102 int m_pageCounter; 00103 int m_sliceCounter; 00104 int m_readIndex; 00105 uint8_t m_endOfFile; 00106 } FwUpdate; 00107 00108 00109 void FwUpdate_init(FwUpdate *thisPtr); 00110 00111 void FwUpdate_start(FwUpdate *thisPtr); 00112 00113 void FwUpdate_handleXbus(FwUpdate *thisPtr, struct XbusMessage *xbusMessage); 00114 00115 00116 #ifdef __cplusplus 00117 } 00118 #endif /* extern "C" */ 00119 00120 00121 #endif /* FWUPDATE_H */
Generated on Wed Jul 13 2022 07:56:15 by
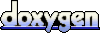