Lcd companion boards support (VKLCD50RTA & VKLCD70RT)
Embed:
(wiki syntax)
Show/hide line numbers
vdc5.c
Go to the documentation of this file.
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file vdc5.c 00025 * @version 00026 * $Rev: 00027 * $Date:: 00028 * @brief VDC5 driver API wrapper function in C interface 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include <stdio.h> 00035 #include <string.h> 00036 00037 #include "r_typedefs.h" 00038 #include "r_vdc5.h" 00039 #include "video_decoder.h" 00040 #include "lvds_pll_calc.h" 00041 #include "vdc5.h" 00042 00043 #include "mbed_assert.h" 00044 #include "pinmap.h" 00045 00046 #include "lcd_panel.h" 00047 00048 /****************************************************************************** 00049 Macro definitions 00050 ******************************************************************************/ 00051 #define STP91_BIT (0x02u) 00052 #define STP90_BIT (0x01u) 00053 #define STBRQ25_BIT (0x20u) 00054 #define STBAK25_BIT (0x20u) 00055 #define STBRQ24_BIT (0x10u) 00056 #define STBAK24_BIT (0x10u) 00057 00058 /****************************************************************************** 00059 Typedef definitions 00060 ******************************************************************************/ 00061 typedef enum { 00062 VDC5_CH0, VDC5_CH1, 00063 } VDC5Name; 00064 00065 /****************************************************************************** 00066 Imported global variables and functions (from other files) 00067 ******************************************************************************/ 00068 00069 /****************************************************************************** 00070 Exported global variables (to be accessed by other files) 00071 ******************************************************************************/ 00072 static const PinMap PinMap_DV_INPUT_PIN[] = { { P8_11, VDC5_CH0, 8 }, /* DV0_CLK */ 00073 { P1_12, VDC5_CH0, 2 }, /* DV0_VSYNC */ 00074 { P1_13, VDC5_CH0, 2 }, /* DV0_HSYNC */ 00075 { P1_1, VDC5_CH0, 6 }, /* DV0_HSYNC */ 00076 { P1_0, VDC5_CH0, 6 }, /* DV0_VSYNC */ 00077 { P1_9, VDC5_CH0, 6 }, /* DV0_DATA15 */ 00078 { P2_15, VDC5_CH0, 3 }, /* DV0_DATA15 */ 00079 { P4_7, VDC5_CH0, 7 }, /* DV0_DATA15 */ 00080 { P5_7, VDC5_CH0, 4 }, /* DV0_DATA15 */ 00081 { P1_8, VDC5_CH0, 6 }, /* DV0_DATA14 */ 00082 { P2_14, VDC5_CH0, 3 }, /* DV0_DATA14 */ 00083 { P4_6, VDC5_CH0, 7 }, /* DV0_DATA14 */ 00084 { P5_6, VDC5_CH0, 4 }, /* DV0_DATA14 */ 00085 { P1_7, VDC5_CH0, 6 }, /* DV0_DATA13 */ 00086 { P2_13, VDC5_CH0, 3 }, /* DV0_DATA13 */ 00087 { P4_5, VDC5_CH0, 7 }, /* DV0_DATA13 */ 00088 { P5_5, VDC5_CH0, 4 }, /* DV0_DATA13 */ 00089 { P1_6, VDC5_CH0, 6 }, /* DV0_DATA12 */ 00090 { P4_4, VDC5_CH0, 7 }, /* DV0_DATA12 */ 00091 { P5_4, VDC5_CH0, 4 }, /* DV0_DATA12 */ 00092 { P2_10, VDC5_CH0, 3 }, /* DV0_DATA10 */ 00093 { P2_9, VDC5_CH0, 3 }, /* DV0_DATA9 */ 00094 { P2_7, VDC5_CH0, 3 }, /* DV0_DATA7 */ 00095 { P2_6, VDC5_CH0, 3 }, /* DV0_DATA6 */ 00096 { P2_5, VDC5_CH0, 3 }, /* DV0_DATA5 */ 00097 { P2_4, VDC5_CH0, 3 }, /* DV0_DATA4 */ 00098 { P2_3, VDC5_CH0, 3 }, /* DV0_DATA3 */ 00099 { P2_2, VDC5_CH0, 3 }, /* DV0_DATA2 */ 00100 { P2_1, VDC5_CH0, 3 }, /* DV0_DATA1 */ 00101 { P2_0, VDC5_CH0, 3 }, /* DV0_DATA0 */ 00102 { NC, NC, 0 } }; 00103 00104 #ifdef MAX_PERI 00105 static const PinMap PinMap_LCD_DISP_PIN[] = { 00106 { P2_7 , VDC5_CH0, 8}, {P4_15 , VDC5_CH0, 1}, {P5_7 , VDC5_CH0, 3}, /* LCD0_DATA23 */ 00107 { P2_6 , VDC5_CH0, 8}, {P4_14 , VDC5_CH0, 1}, {P5_6 , VDC5_CH0, 3}, /* LCD0_DATA22 */ 00108 { P2_5 , VDC5_CH0, 8}, {P4_13 , VDC5_CH0, 1}, {P5_5 , VDC5_CH0, 3}, /* LCD0_DATA21 */ 00109 { P2_4 , VDC5_CH0, 8}, {P4_12 , VDC5_CH0, 1}, {P5_4 , VDC5_CH0, 3}, /* LCD0_DATA20 */ 00110 { P2_3 , VDC5_CH0, 8}, {P4_11 , VDC5_CH0, 1}, {P5_3 , VDC5_CH0, 3}, /* LCD0_DATA19 */ 00111 { P2_2 , VDC5_CH0, 8}, {P4_10 , VDC5_CH0, 1}, {P5_2 , VDC5_CH0, 3}, /* LCD0_DATA18 */ 00112 { P2_1 , VDC5_CH0, 8}, {P4_9 , VDC5_CH0, 1}, {P5_1 , VDC5_CH0, 3}, /* LCD0_DATA17 */ 00113 { P2_0 , VDC5_CH0, 8}, {P4_8 , VDC5_CH0, 1}, {P5_0 , VDC5_CH0, 3}, /* LCD0_DATA16 */ 00114 { P4_7 , VDC5_CH0, 1}, /* LCD0_DATA15 */ 00115 { P4_6 , VDC5_CH0, 1}, /* LCD0_DATA14 */ 00116 { P4_5 , VDC5_CH0, 1}, /* LCD0_DATA13 */ 00117 { P4_4 , VDC5_CH0, 1}, /* LCD0_DATA12 */ 00118 { P4_3 , VDC5_CH0, 1}, /* LCD0_DATA11 */ 00119 { P4_2 , VDC5_CH0, 1}, /* LCD0_DATA10 */ 00120 { P4_1 , VDC5_CH0, 1}, /* LCD0_DATA9 */ 00121 { P4_0 , VDC5_CH0, 1}, /* LCD0_DATA8 */ 00122 { P3_15 , VDC5_CH0, 1}, {P6_15 , VDC5_CH0, 6}, /* LCD0_DATA7 */ 00123 { P3_14 , VDC5_CH0, 1}, {P6_14 , VDC5_CH0, 6}, /* LCD0_DATA6 */ 00124 { P3_13 , VDC5_CH0, 1}, {P6_13 , VDC5_CH0, 6}, /* LCD0_DATA5 */ 00125 { P3_12 , VDC5_CH0, 1}, {P6_12 , VDC5_CH0, 6}, /* LCD0_DATA4 */ 00126 { P3_11 , VDC5_CH0, 1}, {P6_11 , VDC5_CH0, 6}, /* LCD0_DATA3 */ 00127 { P3_10 , VDC5_CH0, 1}, {P6_10 , VDC5_CH0, 6}, /* LCD0_DATA2 */ 00128 { P3_9 , VDC5_CH0, 1}, {P6_9 , VDC5_CH0, 6}, /* LCD0_DATA1 */ 00129 { P3_8 , VDC5_CH0, 1}, {P6_8 , VDC5_CH0, 6}, /* LCD0_DATA0 */ 00130 { P3_7 , VDC5_CH0, 1}, {P6_7 , VDC5_CH0, 4}, {P2_8 , VDC5_CH0, 5}, /* LCD0_TCON6 {P6_11 , VDC5_CH0, 4} */ 00131 { P3_6 , VDC5_CH0, 1}, {P6_6 , VDC5_CH0, 4}, /* LCD0_TCON5 {P6_10 , VDC5_CH0, 4} */ 00132 { P3_5 , VDC5_CH0, 1}, /* LCD0_TCON4 */ 00133 { P3_4 , VDC5_CH0, 1}, /* LCD0_TCON3 */ 00134 { P3_3 , VDC5_CH0, 1}, /* LCD0_TCON2 */ 00135 { P3_2 , VDC5_CH0, 1}, /* LCD0_TCON1 */ 00136 { P3_1 , VDC5_CH0, 1}, /* LCD0_TCON0 */ 00137 { P3_0 , VDC5_CH0, 1}, /* LCD0_CLK */ 00138 { P5_8 , VDC5_CH0, 1}, /* LCD0_EXTCLK */ 00139 { NC , NC , 0} 00140 }; 00141 #else 00142 static const PinMap PinMap_LCD_DISP_PIN[] = { 00143 //R 00144 { P4_7, VDC5_CH0, 1 }, /* LCD0_DATA15 */ 00145 { P4_6, VDC5_CH0, 1 }, /* LCD0_DATA14 */ 00146 { P4_5, VDC5_CH0, 1 }, /* LCD0_DATA13 */ 00147 { P4_4, VDC5_CH0, 1 }, /* LCD0_DATA12 */ 00148 { P4_3, VDC5_CH0, 1 }, /* LCD0_DATA11 */ 00149 //G 00150 { P4_2, VDC5_CH0, 1 }, /* LCD0_DATA10 */ 00151 { P4_1, VDC5_CH0, 1 }, /* LCD0_DATA9 */ 00152 { P4_0, VDC5_CH0, 1 }, /* LCD0_DATA8 */ 00153 { P3_15, VDC5_CH0, 1 }, /* LCD0_DATA7 */ 00154 { P3_14, VDC5_CH0, 1 }, /* LCD0_DATA6 */ 00155 { P3_13, VDC5_CH0, 1 }, /* LCD0_DATA5 */ 00156 //B 00157 { P3_12, VDC5_CH0, 1 }, /* LCD0_DATA4 */ 00158 { P3_11, VDC5_CH0, 1 }, /* LCD0_DATA3 */ 00159 { P3_10, VDC5_CH0, 1 }, /* LCD0_DATA2 */ 00160 { P3_9, VDC5_CH0, 1 }, /* LCD0_DATA1 */ 00161 { P3_8, VDC5_CH0, 1 }, /* LCD0_DATA0 */ 00162 //DE 00163 { P3_1, VDC5_CH0, 1 }, /* LCD0_TCON0 */ 00164 { P3_0, VDC5_CH0, 1 }, /* LCD0_CLK */ 00165 { NC, NC, 0 } }; 00166 #endif 00167 00168 #if 0 00169 static const PinMap PinMap_LCD_DISP_PIN[] = { 00170 { P2_7 , VDC5_CH0, 8}, /* LCD0_DATA23 */ 00171 { P5_7 , VDC5_CH0, 3}, /* LCD0_DATA23 */ 00172 { P2_6 , VDC5_CH0, 8}, /* LCD0_DATA22 */ 00173 { P5_6 , VDC5_CH0, 3}, /* LCD0_DATA22 */ 00174 { P2_5 , VDC5_CH0, 8}, /* LCD0_DATA21 */ 00175 { P5_5 , VDC5_CH0, 3}, /* LCD0_DATA21 */ 00176 { P2_4 , VDC5_CH0, 8}, /* LCD0_DATA20 */ 00177 { P5_4 , VDC5_CH0, 3}, /* LCD0_DATA20 */ 00178 { P2_3 , VDC5_CH0, 8}, /* LCD0_DATA19 */ 00179 { P5_3 , VDC5_CH0, 3}, /* LCD0_DATA19 */ 00180 { P2_2 , VDC5_CH0, 8}, /* LCD0_DATA18 */ 00181 { P5_2 , VDC5_CH0, 3}, /* LCD0_DATA18 */ 00182 { P2_1 , VDC5_CH0, 8}, /* LCD0_DATA17 */ 00183 { P5_1 , VDC5_CH0, 3}, /* LCD0_DATA17 */ 00184 { P2_0 , VDC5_CH0, 8}, /* LCD0_DATA16 */ 00185 { P5_0 , VDC5_CH0, 3}, /* LCD0_DATA16 */ 00186 { P4_7 , VDC5_CH0, 1}, /* LCD0_DATA15 */ 00187 { P4_6 , VDC5_CH0, 1}, /* LCD0_DATA14 */ 00188 { P4_5 , VDC5_CH0, 1}, /* LCD0_DATA13 */ 00189 { P4_4 , VDC5_CH0, 1}, /* LCD0_DATA12 */ 00190 { P4_0 , VDC5_CH0, 1}, /* LCD0_DATA8 */ 00191 { P3_15 , VDC5_CH0, 1}, /* LCD0_DATA7 */ 00192 { P3_14 , VDC5_CH0, 1}, /* LCD0_DATA6 */ 00193 { P3_13 , VDC5_CH0, 1}, /* LCD0_DATA5 */ 00194 { P3_12 , VDC5_CH0, 1}, /* LCD0_DATA4 */ 00195 { P3_11 , VDC5_CH0, 1}, /* LCD0_DATA3 */ 00196 { P3_10 , VDC5_CH0, 1}, /* LCD0_DATA2 */ 00197 { P3_9 , VDC5_CH0, 1}, /* LCD0_DATA1 */ 00198 { P3_8 , VDC5_CH0, 1}, /* LCD0_DATA0 */ 00199 { P3_2 , VDC5_CH0, 1}, /* LCD0_TCON1 */ 00200 { NC , NC , 0} 00201 }; 00202 #endif 00203 00204 static const PinMap PinMap_LVDS_DISP_PIN[] = { { P5_7, VDC5_CH0, 1 }, /* TXOUT0M */ 00205 { P5_6, VDC5_CH0, 1 }, /* TXOUT0P */ 00206 { P5_5, VDC5_CH0, 1 }, /* TXOUT1M */ 00207 { P5_4, VDC5_CH0, 1 }, /* TXOUT1P */ 00208 { P5_3, VDC5_CH0, 1 }, /* TXOUT2M */ 00209 { P5_2, VDC5_CH0, 1 }, /* TXOUT2P */ 00210 { P5_1, VDC5_CH0, 1 }, /* TXCLKOUTM */ 00211 { P5_0, VDC5_CH0, 1 }, /* TXCLKOUTP */ 00212 { NC, NC, 0 } }; 00213 00214 static const IRQn_Type vdc5_irq_set_tbl[] = { S0_VI_VSYNC0_IRQn, 00215 S0_LO_VSYNC0_IRQn, S0_VSYNCERR0_IRQn, GR3_VLINE0_IRQn, S0_VFIELD0_IRQn, 00216 IV1_VBUFERR0_IRQn, IV3_VBUFERR0_IRQn, IV5_VBUFERR0_IRQn, 00217 IV6_VBUFERR0_IRQn, S0_WLINE0_IRQn, S1_VI_VSYNC0_IRQn, S1_LO_VSYNC0_IRQn, 00218 S1_VSYNCERR0_IRQn, S1_VFIELD0_IRQn, IV2_VBUFERR0_IRQn, 00219 IV4_VBUFERR0_IRQn, S1_WLINE0_IRQn, OIR_VI_VSYNC0_IRQn, 00220 OIR_LO_VSYNC0_IRQn, OIR_VSYNCERR0_IRQn, OIR_VFIELD0_IRQn, 00221 IV7_VBUFERR0_IRQn, IV8_VBUFERR0_IRQn }; 00222 00223 /****************************************************************************** 00224 Private global variables and functions 00225 ******************************************************************************/ 00226 static void init_func(const uint32_t user_num); 00227 static void DRV_Graphics_Irq_Set(vdc5_int_type_t irq, uint32_t enable); 00228 00229 /**************************************************************************//** 00230 * @brief User-defined function within R_VDC5_Initialize 00231 * @param[in] user_num : VDC5 channel 00232 * @retval None 00233 ******************************************************************************/ 00234 static void init_func(const uint32_t user_num) { 00235 uint32_t reg_data; 00236 volatile uint8_t dummy_read; 00237 00238 if ((vdc5_channel_t ) user_num == VDC5_CHANNEL_0 ) { 00239 00240 /* Standby control register 9 (STBCR9) 00241 b1 ------0-; MSTP91 : 0 : Video display controller channel 0 & LVDS enable */ 00242 reg_data = (uint32_t) CPG.STBCR9 & (uint32_t) ~STP91_BIT; 00243 CPG.STBCR9 = (uint8_t) reg_data; 00244 /* In order to reflect the change, a dummy read should be done. */ 00245 dummy_read = CPG.STBCR9; 00246 00247 /* Standby Request Register 2 (STBREQ2) 00248 b5 --0-----; STBRQ25 : The standby request to VDC5 channel 0 is invalid. */ 00249 reg_data = (uint32_t) CPG.STBREQ2 & (uint32_t) ~STBRQ25_BIT; 00250 CPG.STBREQ2 = (uint8_t) reg_data; 00251 /* Standby Acknowledge Register 2 (STBACK2) 00252 b5 --*-----; STBAK25 : Standby acknowledgement from VDC5 channel 0. */ 00253 while (((uint32_t) CPG.STBACK2 & (uint32_t) STBAK25_BIT) != 0u) { 00254 /* Wait for the STBAK25 to be cleared to 0. */ 00255 } 00256 00257 /* Standby control register 9 (STBCR9) 00258 b0 -------0; MSTP90 : 0 : Video display controller channel 1 enable */ 00259 reg_data = (uint32_t) CPG.STBCR9 & (uint32_t) ~(STP91_BIT | STP90_BIT); 00260 CPG.STBCR9 = (uint8_t) reg_data; 00261 /* In order to reflect the change, a dummy read should be done. */ 00262 dummy_read = CPG.STBCR9; 00263 00264 /* Standby Request Register 2 (STBREQ2) 00265 b4 ---0----; STBRQ24 : The standby request to VDC5 channel 1 is invalid. */ 00266 reg_data = (uint32_t) CPG.STBREQ2 & (uint32_t) ~STBRQ24_BIT; 00267 CPG.STBREQ2 = (uint8_t) reg_data; 00268 /* Standby Acknowledge Register 2 (STBACK2) 00269 b4 ---*----; STBAK24 : Standby acknowledgement from VDC5 channel 1. */ 00270 while (((uint32_t) CPG.STBACK2 & (uint32_t) STBAK24_BIT) != 0u) { 00271 /* Wait for the STBAK24 to be cleared to 0. */ 00272 } 00273 } 00274 } /* End of function init_func() */ 00275 00276 /**************************************************************************//** 00277 * @brief Interrupt service routine acquisition processing 00278 * 00279 * Description:<br> 00280 * This function returns the function pointer to the specified interrupt service routine. 00281 * @param[in] irq : VDC5 interrupt type 00282 * @param[in] enable : VDC5 interrupt enable 00283 * @retval None 00284 ******************************************************************************/ 00285 static void DRV_Graphics_Irq_Set(vdc5_int_type_t irq, uint32_t enable) { 00286 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00287 IRQn_Type IRQn; 00288 IRQHandler handler; 00289 00290 IRQn = vdc5_irq_set_tbl[irq]; 00291 handler = R_VDC5_GetISR(ch, irq); 00292 00293 if (enable) { 00294 InterruptHandlerRegister(IRQn, (void (*)(uint32_t)) handler); 00295 GIC_SetPriority(IRQn, 5); 00296 GIC_EnableIRQ(IRQn); 00297 } else { 00298 GIC_DisableIRQ(IRQn); 00299 } 00300 } /* End of function DRV_Graphics_Irq_Set() */ 00301 00302 /**************************************************************************//** 00303 * @brief Interrupt callback setup 00304 * This function performs the following processing: 00305 * - Enables the interrupt when the pointer to the corresponding interrupt callback function is specified. 00306 * - Registers the specified interrupt callback function. 00307 * - Disables the interrupt when the pointer to the corresponding interrupt callback function is not 00308 * specified. 00309 * @param[in] irq : VDC5 interrupt type 00310 * @param[in] num : Interrupt line number 00311 * @param[in] * callback : Interrupt callback function pointer 00312 * @retval Error code 00313 ******************************************************************************/ 00314 drv_graphics_error_t DRV_Graphics_Irq_Handler_Set(vdc5_int_type_t irq, 00315 uint16_t num, void (*callback)(vdc5_int_type_t )) { 00316 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00317 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00318 vdc5_error_t error; 00319 vdc5_int_t interrupt; 00320 00321 if (callback == NULL) { 00322 DRV_Graphics_Irq_Set(irq, 0); 00323 } else { 00324 DRV_Graphics_Irq_Set(irq, 1); 00325 } 00326 00327 /* Interrupt parameter */ 00328 interrupt.type = irq; /* Interrupt type */ 00329 interrupt.line_num = num; /* Line number */ 00330 00331 /* Interrupt parameter */ 00332 interrupt.callback = callback; /* Callback function pointer */ 00333 /* Set interrupt service routine */ 00334 error = R_VDC5_CallbackISR(ch, &interrupt); 00335 if (error != VDC5_OK ) { 00336 drv_error = DRV_GRAPHICS_VDC5_ERR; 00337 } 00338 return drv_error; 00339 } /* End of function DRV_Graphics_Irq_Handler_Set() */ 00340 00341 /**************************************************************************//** 00342 * @brief LCD output port initialization processing 00343 * @param[in] pin : Pin assign for LCD output 00344 * @param[in] pin_count : Total number of pin assign 00345 * @retval Error code 00346 ******************************************************************************/ 00347 drv_graphics_error_t DRV_Graphics_Lcd_Port_Init(PinName *pin, 00348 uint32_t pin_count) { 00349 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00350 uint32_t count; 00351 00352 for (count = 0; count < pin_count; count++) { 00353 pinmap_peripheral(pin[count], PinMap_LCD_DISP_PIN); 00354 pinmap_pinout(pin[count], PinMap_LCD_DISP_PIN); 00355 } 00356 return drv_error; 00357 } /* End of function DRV_Graphics_Lcd_Port_Init() */ 00358 00359 /**************************************************************************//** 00360 * @brief LVDS output port initialization processing 00361 * @param[in] pin : Pin assign for LVDS output 00362 * @param[in] pin_count : Total number of pin assign 00363 * @retval Error code 00364 ******************************************************************************/ 00365 drv_graphics_error_t DRV_Graphics_Lvds_Port_Init(PinName *pin, 00366 uint32_t pin_count) { 00367 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00368 uint32_t count; 00369 00370 for (count = 0; count < pin_count; count++) { 00371 pinmap_peripheral(pin[count], PinMap_LVDS_DISP_PIN); 00372 pinmap_pinout(pin[count], PinMap_LVDS_DISP_PIN); 00373 } 00374 return drv_error; 00375 } /* End of function DRV_Graphics_Lvds_Port_Init() */ 00376 00377 /**************************************************************************//** 00378 * @brief Digital video inpout port initialization processing 00379 * @param[in] pin : Pin assign for digital video input port 00380 * @param[in] pin_count : Total number of pin assign 00381 * @retval Error code 00382 ******************************************************************************/ 00383 drv_graphics_error_t DRV_Graphics_Dvinput_Port_Init(PinName *pin, 00384 uint32_t pin_count) { 00385 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00386 uint32_t count; 00387 00388 for (count = 0; count < pin_count; count++) { 00389 pinmap_peripheral(pin[count], PinMap_DV_INPUT_PIN); 00390 pinmap_pinout(pin[count], PinMap_DV_INPUT_PIN); 00391 } 00392 return drv_error; 00393 } /* End of function DRV_Graphics_Dvinput_Port_Init() */ 00394 00395 /**************************************************************************//** 00396 * @brief Graphics initialization processing 00397 * @param[in] drv_lcd_config : LCD configuration 00398 * @retval Error code 00399 ******************************************************************************/ 00400 drv_graphics_error_t DRV_Graphics_Init(drv_lcd_config_t * drv_lcd_config) { 00401 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00402 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00403 vdc5_error_t error; 00404 vdc5_init_t init; 00405 vdc5_lvds_t vdc5_lvds; 00406 pll_parameter_t pll_parameter; 00407 double InputClock = DEFAULT_INPUT_CLOCK; 00408 double OutputClock = DEFAULT_OUTPUT_CLOCK; 00409 uint32_t LvdsUsed = LVDS_IF_USE; 00410 00411 if (drv_lcd_config != NULL) { 00412 InputClock = drv_lcd_config->intputClock; 00413 OutputClock = drv_lcd_config->outputClock; 00414 LvdsUsed = 00415 (drv_lcd_config->lcd_type == DRV_LCD_TYPE_LVDS) ? 00416 LVDS_IF_USE : LVDS_IF_NOT_USE; 00417 } 00418 00419 /* Initialization parameter */ 00420 if (LvdsUsed) { 00421 /* LVDS PLL Setting Calculation */ 00422 init.panel_icksel = VDC5_PANEL_ICKSEL_LVDS_DIV7 ; /* Panel clock select */ 00423 init.panel_dcdr = VDC5_PANEL_CLKDIV_1_2 ; /* Panel clock frequency division ratio */ 00424 lvds_pll_calc(InputClock, OutputClock, LvdsUsed, &pll_parameter); 00425 00426 vdc5_lvds.lvds_in_clk_sel = VDC5_LVDS_INCLK_SEL_PERI ; /* P1 */ 00427 vdc5_lvds.lvds_idiv_set = (vdc5_lvds_ndiv_t ) pll_parameter.nidiv; 00428 vdc5_lvds.lvdspll_tst = 16u; 00429 vdc5_lvds.lvds_odiv_set = (vdc5_lvds_ndiv_t ) pll_parameter.nodiv; 00430 vdc5_lvds.lvdspll_tst = 16u; 00431 vdc5_lvds.lvds_vdc_sel = ch; 00432 vdc5_lvds.lvdspll_fd = pll_parameter.nfd; 00433 vdc5_lvds.lvdspll_rd = pll_parameter.nrd; 00434 vdc5_lvds.lvdspll_od = (vdc5_lvds_pll_nod_t ) pll_parameter.nod; 00435 init.lvds = &vdc5_lvds; /* LVDS parameter */ 00436 } else { 00437 init.panel_icksel = VDC5_PANEL_ICKSEL_PERI ; /* Panel clock select */ 00438 init.panel_dcdr = VDC5_PANEL_CLKDIV_1_7 ; /* Panel clock frequency division ratio */ 00439 init.lvds = NULL; 00440 } 00441 00442 /* Initialize (Set module clock to VDC5) */ 00443 error = R_VDC5_Initialize(ch, &init, &init_func, (uint32_t) ch); 00444 if (error != VDC5_OK ) { 00445 drv_error = DRV_GRAPHICS_VDC5_ERR; 00446 } 00447 00448 if (drv_error == DRV_GRAPHICS_OK) { 00449 vdc5_sync_ctrl_t sync_ctrl; 00450 00451 /* Sync signal control */ 00452 sync_ctrl.res_vs_sel = VDC5_ON ; /* Vsync signal output select (free-running Vsync on/off control) */ 00453 /* Sync signal output and full-screen enable signal select */ 00454 sync_ctrl.res_vs_in_sel = VDC5_RES_VS_IN_SEL_SC0 ; 00455 sync_ctrl.res_fv = drv_lcd_config->v_toatal_period - 1; /* Free-running Vsync period setting */ 00456 sync_ctrl.res_fh = drv_lcd_config->h_toatal_period - 1; /* Hsync period setting */ 00457 sync_ctrl.res_vsdly = (uint16_t) 0u; /* Vsync signal delay control */ 00458 /* Full-screen enable control */ 00459 sync_ctrl.res_f .vs = (drv_lcd_config->v_back_porch); 00460 sync_ctrl.res_f .vw = (drv_lcd_config->v_disp_widht); 00461 sync_ctrl.res_f .hs = (drv_lcd_config->h_back_porch); 00462 sync_ctrl.res_f .hw = (drv_lcd_config->h_disp_widht); 00463 sync_ctrl.vsync_cpmpe = NULL; /* Vsync signal compensation */ 00464 /* Sync control */ 00465 error = R_VDC5_SyncControl(ch, &sync_ctrl); 00466 if (error != VDC5_OK ) { 00467 drv_error = DRV_GRAPHICS_VDC5_ERR; 00468 } 00469 } 00470 00471 if (drv_error == DRV_GRAPHICS_OK) { 00472 vdc5_output_t output; 00473 vdc5_lcd_tcon_timing_t lcd_tcon_timing_VS; 00474 vdc5_lcd_tcon_timing_t lcd_tcon_timing_VE; 00475 vdc5_lcd_tcon_timing_t lcd_tcon_timing_HS; 00476 vdc5_lcd_tcon_timing_t lcd_tcon_timing_HE; 00477 vdc5_lcd_tcon_timing_t lcd_tcon_timing_DE; 00478 00479 /* Output parameter */ 00480 output.tcon_half = (drv_lcd_config->h_toatal_period - 1) / 2; /* TCON reference timing, 1/2fH timing */ 00481 output.tcon_offset = 0; /* TCON reference timing, offset Hsync signal timing */ 00482 00483 /* LCD TCON timing setting */ 00484 if (drv_lcd_config->v_sync_port != DRV_LCD_TCON_PIN_NON) { 00485 lcd_tcon_timing_VS.tcon_hsvs = 0u; 00486 lcd_tcon_timing_VS.tcon_hwvw = (drv_lcd_config->v_sync_width * 2u); 00487 lcd_tcon_timing_VS.tcon_md = VDC5_LCD_TCON_POLMD_NORMAL ; 00488 lcd_tcon_timing_VS.tcon_hs_sel = VDC5_LCD_TCON_REFSEL_HSYNC ; 00489 lcd_tcon_timing_VS.tcon_inv = 00490 (vdc5_sig_pol_t ) drv_lcd_config->v_sync_port_polarity; 00491 lcd_tcon_timing_VS.tcon_pin = 00492 (vdc5_lcd_tcon_pin_t ) drv_lcd_config->v_sync_port; 00493 lcd_tcon_timing_VS.outcnt_edge = VDC5_EDGE_FALLING ; 00494 output.outctrl [VDC5_LCD_TCONSIG_STVA_VS ] = &lcd_tcon_timing_VS; /* STVA/VS: Vsync */ 00495 } else { 00496 output.outctrl [VDC5_LCD_TCONSIG_STVA_VS ] = NULL; /* STVA/VS: Vsync */ 00497 } 00498 00499 if (drv_lcd_config->h_sync_port != DRV_LCD_TCON_PIN_NON) { 00500 lcd_tcon_timing_HS.tcon_hsvs = 0u; 00501 lcd_tcon_timing_HS.tcon_hwvw = drv_lcd_config->h_sync_width; 00502 lcd_tcon_timing_HS.tcon_md = VDC5_LCD_TCON_POLMD_NORMAL ; 00503 lcd_tcon_timing_HS.tcon_hs_sel = VDC5_LCD_TCON_REFSEL_HSYNC ; 00504 lcd_tcon_timing_HS.tcon_inv = 00505 (vdc5_sig_pol_t ) drv_lcd_config->h_sync_port_polarity; 00506 lcd_tcon_timing_HS.tcon_pin = 00507 (vdc5_lcd_tcon_pin_t ) drv_lcd_config->h_sync_port; 00508 lcd_tcon_timing_HS.outcnt_edge = VDC5_EDGE_FALLING ; 00509 output.outctrl [VDC5_LCD_TCONSIG_STH_SP_HS ] = &lcd_tcon_timing_HS; /* STH/SP/HS: Hsync */ 00510 } else { 00511 output.outctrl [VDC5_LCD_TCONSIG_STH_SP_HS ] = NULL; /* STH/SP/HS: Hsync */ 00512 } 00513 00514 if (drv_lcd_config->de_port != DRV_LCD_TCON_PIN_NON) { 00515 lcd_tcon_timing_VE.tcon_hsvs = (drv_lcd_config->v_back_porch * 2u); 00516 lcd_tcon_timing_VE.tcon_hwvw = (drv_lcd_config->v_disp_widht * 2u); 00517 lcd_tcon_timing_VE.tcon_md = VDC5_LCD_TCON_POLMD_NORMAL ; 00518 lcd_tcon_timing_VE.tcon_hs_sel = VDC5_LCD_TCON_REFSEL_HSYNC ; 00519 lcd_tcon_timing_VE.tcon_inv = 00520 (vdc5_sig_pol_t ) drv_lcd_config->de_port_polarity; 00521 lcd_tcon_timing_VE.tcon_pin = VDC5_LCD_TCON_PIN_NON ; 00522 lcd_tcon_timing_VE.outcnt_edge = VDC5_EDGE_FALLING ; 00523 output.outctrl [VDC5_LCD_TCONSIG_STVB_VE ] = &lcd_tcon_timing_VE; /* STVB/VE: Not used */ 00524 00525 lcd_tcon_timing_HE.tcon_hsvs = drv_lcd_config->h_back_porch; 00526 lcd_tcon_timing_HE.tcon_hwvw = drv_lcd_config->h_disp_widht; 00527 lcd_tcon_timing_HE.tcon_md = VDC5_LCD_TCON_POLMD_NORMAL ; 00528 lcd_tcon_timing_HE.tcon_hs_sel = VDC5_LCD_TCON_REFSEL_HSYNC ; 00529 lcd_tcon_timing_HE.tcon_inv = 00530 (vdc5_sig_pol_t ) drv_lcd_config->de_port_polarity; 00531 lcd_tcon_timing_HE.tcon_pin = VDC5_LCD_TCON_PIN_NON ; 00532 lcd_tcon_timing_HE.outcnt_edge = VDC5_EDGE_FALLING ; 00533 output.outctrl [VDC5_LCD_TCONSIG_STB_LP_HE ] = &lcd_tcon_timing_HE; /* STB/LP/HE: Not used */ 00534 00535 lcd_tcon_timing_DE.tcon_hsvs = 0u; 00536 lcd_tcon_timing_DE.tcon_hwvw = 0u; 00537 lcd_tcon_timing_DE.tcon_md = VDC5_LCD_TCON_POLMD_NORMAL ; 00538 lcd_tcon_timing_DE.tcon_hs_sel = VDC5_LCD_TCON_REFSEL_HSYNC ; 00539 lcd_tcon_timing_DE.tcon_inv = 00540 (vdc5_sig_pol_t ) drv_lcd_config->de_port_polarity; 00541 lcd_tcon_timing_DE.tcon_pin = 00542 (vdc5_lcd_tcon_pin_t ) drv_lcd_config->de_port; 00543 lcd_tcon_timing_DE.outcnt_edge = VDC5_EDGE_FALLING ; 00544 output.outctrl [VDC5_LCD_TCONSIG_DE ] = &lcd_tcon_timing_DE; /* DE */ 00545 } else { 00546 output.outctrl [VDC5_LCD_TCONSIG_STVB_VE ] = NULL; /* STVB/VE: Not used */ 00547 output.outctrl [VDC5_LCD_TCONSIG_STB_LP_HE ] = NULL; /* STB/LP/HE: Not used */ 00548 output.outctrl [VDC5_LCD_TCONSIG_DE ] = NULL; /* DE */ 00549 } 00550 00551 output.outctrl [VDC5_LCD_TCONSIG_CPV_GCK ] = NULL; 00552 output.outctrl [VDC5_LCD_TCONSIG_POLA ] = NULL; 00553 output.outctrl [VDC5_LCD_TCONSIG_POLB ] = NULL; 00554 00555 output.outcnt_lcd_edge = (vdc5_edge_t ) drv_lcd_config->lcd_edge; /* Output phase control of LCD_DATA23 to LCD_DATA0 pin */ 00556 output.out_endian_on = VDC5_OFF ; /* Bit endian change on/off control */ 00557 output.out_swap_on = VDC5_OFF ; /* B/R signal swap on/off control */ 00558 output.out_format = 00559 (vdc5_lcd_outformat_t ) drv_lcd_config->lcd_outformat; /* Output format select */ 00560 output.out_frq_sel = VDC5_LCD_PARALLEL_CLKFRQ_1 ; /* Clock frequency control */ 00561 output.out_dir_sel = VDC5_LCD_SERIAL_SCAN_FORWARD ; /* Scan direction select */ 00562 output.out_phase = VDC5_LCD_SERIAL_CLKPHASE_0 ; /* Clock phase adjustment */ 00563 output.bg_color = (uint32_t) 0x00000000u; /* Background color in 24-bit RGB color format */ 00564 /* Display output */ 00565 error = R_VDC5_DisplayOutput(ch, &output); 00566 if (error != VDC5_OK ) { 00567 drv_error = DRV_GRAPHICS_VDC5_ERR; 00568 } 00569 } 00570 return drv_error; 00571 } /* End of function DRV_Graphics_Init() */ 00572 00573 /**************************************************************************//** 00574 * @brief Video initialization processing 00575 * @param[in] drv_video_ext_in_config : Video configuration 00576 * @retval Error code 00577 ******************************************************************************/ 00578 drv_graphics_error_t DRV_Graphics_Video_init( 00579 drv_video_input_sel_t drv_video_input_sel, 00580 drv_video_ext_in_config_t * drv_video_ext_in_config) { 00581 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00582 vdc5_error_t error; 00583 vdc5_input_t input; 00584 vdc5_ext_in_sig_t ext_in_sig; 00585 vdc5_sync_delay_t sync_delay; 00586 00587 input.inp_sel = (vdc5_input_sel_t ) drv_video_input_sel; /* Input select */ 00588 input.inp_fh50 = (uint16_t) VSYNC_1_2_FH_TIMING; /* Vsync signal 1/2fH phase timing */ 00589 input.inp_fh25 = (uint16_t) VSYNC_1_4_FH_TIMING; /* Vsync signal 1/4fH phase timing */ 00590 00591 if (drv_video_input_sel == DRV_INPUT_SEL_VDEC ) { 00592 input.dly = NULL; /* Sync signal delay adjustment */ 00593 input.ext_sig = NULL; /* External input signal */ 00594 } else { 00595 ext_in_sig.inp_format = 00596 (vdc5_extin_format_t ) drv_video_ext_in_config->inp_format; 00597 ext_in_sig.inp_pxd_edge = 00598 (vdc5_edge_t ) drv_video_ext_in_config->inp_pxd_edge; 00599 ext_in_sig.inp_vs_edge = 00600 (vdc5_edge_t ) drv_video_ext_in_config->inp_vs_edge; 00601 ext_in_sig.inp_hs_edge = 00602 (vdc5_edge_t ) drv_video_ext_in_config->inp_hs_edge; 00603 ext_in_sig.inp_endian_on = 00604 (vdc5_onoff_t ) drv_video_ext_in_config->inp_endian_on; 00605 ext_in_sig.inp_swap_on = 00606 (vdc5_onoff_t ) drv_video_ext_in_config->inp_swap_on; 00607 ext_in_sig.inp_vs_inv = 00608 (vdc5_sig_pol_t ) drv_video_ext_in_config->inp_vs_inv; 00609 ext_in_sig.inp_hs_inv = 00610 (vdc5_sig_pol_t ) drv_video_ext_in_config->inp_hs_inv; 00611 ext_in_sig.inp_h_edge_sel = 00612 (vdc5_extin_ref_hsync_t ) drv_video_ext_in_config->inp_hs_edge; 00613 ext_in_sig.inp_f525_625 = 00614 (vdc5_extin_input_line_t ) drv_video_ext_in_config->inp_f525_625; 00615 ext_in_sig.inp_h_pos = 00616 (vdc5_extin_h_pos_t ) drv_video_ext_in_config->inp_h_pos; 00617 00618 sync_delay.inp_vs_dly_l = 0u; 00619 sync_delay.inp_vs_dly = 16u; 00620 sync_delay.inp_hs_dly = 16u; 00621 sync_delay.inp_fld_dly = 16u; 00622 00623 input.dly = &sync_delay; /* Sync signal delay adjustment */ 00624 input.ext_sig = &ext_in_sig; /* External input signal */ 00625 } 00626 /* Video input 0ch */ 00627 error = R_VDC5_VideoInput(VDC5_CHANNEL_0 , &input); 00628 if (error != VDC5_OK ) { 00629 drv_error = DRV_GRAPHICS_VDC5_ERR; 00630 } 00631 00632 if (drv_video_input_sel == DRV_INPUT_SEL_VDEC ) { 00633 if (drv_error == DRV_GRAPHICS_OK) { 00634 /* Video input 1ch */ 00635 error = R_VDC5_VideoInput(VDC5_CHANNEL_1 , &input); 00636 if (error != VDC5_OK ) { 00637 drv_error = DRV_GRAPHICS_VDC5_ERR; 00638 } 00639 } 00640 } 00641 return drv_error; 00642 } /* End of function DRV_Video_Init() */ 00643 00644 /**************************************************************************//** 00645 * @brief Start the graphics surface read process 00646 * @param[in] layer_id : Graphics layer ID 00647 * @retval drv_graphics_error_t 00648 ******************************************************************************/ 00649 drv_graphics_error_t DRV_Graphics_Start(drv_graphics_layer_t layer_id) { 00650 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00651 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00652 vdc5_error_t error; 00653 vdc5_start_t start; 00654 vdc5_gr_disp_sel_t gr_disp_sel; 00655 vdc5_layer_id_t vdc5_layer_id; 00656 00657 switch (layer_id) { 00658 case DRV_GRAPHICS_LAYER_0: 00659 vdc5_layer_id = VDC5_LAYER_ID_0_RD ; 00660 gr_disp_sel = VDC5_DISPSEL_CURRENT ; 00661 break; 00662 case DRV_GRAPHICS_LAYER_1: 00663 vdc5_layer_id = VDC5_LAYER_ID_1_RD ; 00664 gr_disp_sel = VDC5_DISPSEL_BLEND ; 00665 break; 00666 case DRV_GRAPHICS_LAYER_2: 00667 vdc5_layer_id = VDC5_LAYER_ID_2_RD ; 00668 gr_disp_sel = VDC5_DISPSEL_BLEND ; 00669 break; 00670 case DRV_GRAPHICS_LAYER_3: 00671 vdc5_layer_id = VDC5_LAYER_ID_3_RD ; 00672 gr_disp_sel = VDC5_DISPSEL_BLEND ; 00673 break; 00674 default: 00675 drv_error = DRV_GRAPHICS_LAYER_ERR; 00676 break; 00677 } 00678 00679 if (drv_error == DRV_GRAPHICS_OK) { 00680 /* Start process */ 00681 start.gr_disp_sel = &gr_disp_sel; 00682 error = R_VDC5_StartProcess(ch, vdc5_layer_id, &start); 00683 if (error != VDC5_OK ) { 00684 drv_error = DRV_GRAPHICS_VDC5_ERR; 00685 } 00686 } 00687 return drv_error; 00688 } /* End of function DRV_Graphics_Start() */ 00689 00690 /**************************************************************************//** 00691 * @brief Stop the graphics surface read process 00692 * @param[in] layer_id : Graphics layer ID 00693 * @retval Error code 00694 ******************************************************************************/ 00695 drv_graphics_error_t DRV_Graphics_Stop(drv_graphics_layer_t layer_id) { 00696 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00697 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00698 vdc5_error_t error; 00699 vdc5_layer_id_t vdc5_layer_id; 00700 00701 switch (layer_id) { 00702 case DRV_GRAPHICS_LAYER_0: 00703 vdc5_layer_id = VDC5_LAYER_ID_0_RD ; 00704 break; 00705 case DRV_GRAPHICS_LAYER_1: 00706 vdc5_layer_id = VDC5_LAYER_ID_1_RD ; 00707 break; 00708 case DRV_GRAPHICS_LAYER_2: 00709 vdc5_layer_id = VDC5_LAYER_ID_2_RD ; 00710 break; 00711 case DRV_GRAPHICS_LAYER_3: 00712 vdc5_layer_id = VDC5_LAYER_ID_3_RD ; 00713 break; 00714 default: 00715 drv_error = DRV_GRAPHICS_LAYER_ERR; 00716 break; 00717 } 00718 00719 if (drv_error == DRV_GRAPHICS_OK) { 00720 /* Stop process */ 00721 error = R_VDC5_StopProcess(ch, vdc5_layer_id); 00722 if (error != VDC5_OK ) { 00723 drv_error = DRV_GRAPHICS_VDC5_ERR; 00724 } 00725 } 00726 return drv_error; 00727 } /* End of function DRV_Graphics_Stop() */ 00728 00729 /**************************************************************************//** 00730 * @brief Start the video surface write process 00731 * @param[in] video_input_ch : Video input channel 00732 * @retval Error code 00733 ******************************************************************************/ 00734 drv_graphics_error_t DRV_Video_Start(drv_video_input_channel_t video_input_ch) { 00735 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00736 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00737 vdc5_error_t error; 00738 vdc5_start_t start; 00739 vdc5_gr_disp_sel_t gr_disp_sel; 00740 vdc5_layer_id_t vdc5_layer_id; 00741 00742 if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_0) { 00743 vdc5_layer_id = VDC5_LAYER_ID_0_WR ; 00744 } else if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_1) { 00745 vdc5_layer_id = VDC5_LAYER_ID_1_WR ; 00746 } else { 00747 drv_error = DRV_GRAPHICS_LAYER_ERR; 00748 } 00749 00750 if (drv_error == DRV_GRAPHICS_OK) { 00751 /* Start process */ 00752 gr_disp_sel = VDC5_DISPSEL_CURRENT ; /* CURRENT fixed for weave input mode */ 00753 start.gr_disp_sel = &gr_disp_sel; 00754 error = R_VDC5_StartProcess(ch, vdc5_layer_id, &start); 00755 if (error != VDC5_OK ) { 00756 drv_error = DRV_GRAPHICS_VDC5_ERR; 00757 } 00758 } 00759 return drv_error; 00760 } /* End of function DRV_Video_Start() */ 00761 00762 /**************************************************************************//** 00763 * @brief Stop the video surface write process 00764 * @param[in] video_input_ch : Video input channel 00765 * @retval Error code 00766 ******************************************************************************/ 00767 drv_graphics_error_t DRV_Video_Stop(drv_video_input_channel_t video_input_ch) { 00768 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00769 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00770 vdc5_error_t error; 00771 vdc5_layer_id_t vdc5_layer_id; 00772 00773 switch (video_input_ch) { 00774 case DRV_VIDEO_INPUT_CHANNEL_0: 00775 vdc5_layer_id = VDC5_LAYER_ID_0_WR ; 00776 break; 00777 case DRV_VIDEO_INPUT_CHANNEL_1: 00778 vdc5_layer_id = VDC5_LAYER_ID_1_WR ; 00779 break; 00780 default: 00781 drv_error = DRV_GRAPHICS_LAYER_ERR; 00782 break; 00783 } 00784 00785 if (drv_error == DRV_GRAPHICS_OK) { 00786 /* Stop process */ 00787 error = R_VDC5_StopProcess(ch, vdc5_layer_id); 00788 if (error != VDC5_OK ) { 00789 drv_error = DRV_GRAPHICS_VDC5_ERR; 00790 } 00791 } 00792 return drv_error; 00793 } /* End of function DRV_Video_Stop() */ 00794 00795 /**************************************************************************//** 00796 * @brief Graphics surface read process setting 00797 * 00798 * Description:<br> 00799 * This function supports the following 4 image format. 00800 * YCbCr422, RGB565, RGB888, ARGB8888 00801 * @param[in] layer_id : Graphics layer ID 00802 * @param[in] framebuff : Base address of the frame buffer 00803 * @param[in] fb_stride : Line offset address of the frame buffer 00804 * @param[in] gr_format : Format of the frame buffer read signal 00805 * @param[in] gr_rect : Graphics display area 00806 * @retval Error code 00807 ******************************************************************************/ 00808 drv_graphics_error_t DRV_Graphics_Read_Setting(drv_graphics_layer_t layer_id, 00809 void * framebuff, uint32_t fb_stride, drv_graphics_format_t gr_format, 00810 drv_wr_rd_swa_t wr_rd_swa, drv_rect_t * gr_rect) { 00811 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00812 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00813 vdc5_error_t error; 00814 vdc5_layer_id_t vdc5_layer_id; 00815 vdc5_gr_format_t vdc5_gr_format; 00816 vdc5_read_t read; 00817 00818 switch (layer_id) { 00819 case DRV_GRAPHICS_LAYER_0: 00820 vdc5_layer_id = VDC5_LAYER_ID_0_RD ; 00821 break; 00822 case DRV_GRAPHICS_LAYER_1: 00823 vdc5_layer_id = VDC5_LAYER_ID_1_RD ; 00824 break; 00825 case DRV_GRAPHICS_LAYER_2: 00826 vdc5_layer_id = VDC5_LAYER_ID_2_RD ; 00827 break; 00828 case DRV_GRAPHICS_LAYER_3: 00829 vdc5_layer_id = VDC5_LAYER_ID_3_RD ; 00830 break; 00831 default: 00832 drv_error = DRV_GRAPHICS_LAYER_ERR; 00833 break; 00834 } 00835 00836 if (drv_error == DRV_GRAPHICS_OK) { 00837 switch (gr_format) { 00838 case DRV_GRAPHICS_FORMAT_YCBCR422: 00839 vdc5_gr_format = VDC5_GR_FORMAT_YCBCR422 ; 00840 break; 00841 case DRV_GRAPHICS_FORMAT_RGB565: 00842 vdc5_gr_format = VDC5_GR_FORMAT_RGB565 ; 00843 break; 00844 case DRV_GRAPHICS_FORMAT_RGB888: 00845 vdc5_gr_format = VDC5_GR_FORMAT_RGB888 ; 00846 break; 00847 case DRV_GRAPHICS_FORMAT_ARGB8888: 00848 vdc5_gr_format = VDC5_GR_FORMAT_ARGB8888 ; 00849 break; 00850 case DRV_GRAPHICS_FORMAT_ARGB4444: 00851 vdc5_gr_format = VDC5_GR_FORMAT_ARGB4444 ; 00852 break; 00853 default: 00854 drv_error = DRV_GRAPHICS_FORMAT_ERR; 00855 break; 00856 } 00857 } 00858 00859 if (drv_error == DRV_GRAPHICS_OK) { 00860 /* Read data parameter */ 00861 read.gr_ln_off_dir = VDC5_GR_LN_OFF_DIR_INC ; /* Line offset address direction of the frame buffer */ 00862 read.gr_flm_sel = VDC5_GR_FLM_SEL_FLM_NUM ; /* Selects a frame buffer address setting signal */ 00863 read.gr_imr_flm_inv = VDC5_OFF ; /* Frame buffer number for distortion correction */ 00864 read.gr_bst_md = VDC5_BST_MD_32BYTE ; /* Frame buffer burst transfer mode */ 00865 read.gr_base = framebuff; /* Frame buffer base address */ 00866 read.gr_ln_off = fb_stride; /* Frame buffer line offset address */ 00867 00868 read.width_read_fb = NULL; /* Width of the image read from frame buffer */ 00869 00870 read.adj_sel = VDC5_OFF ; /* Measures to decrease the influence 00871 by folding pixels/lines (on/off) */ 00872 read.gr_format = vdc5_gr_format; /* Format of the frame buffer read signal */ 00873 read.gr_ycc_swap = VDC5_GR_YCCSWAP_CBY0CRY1; /* Controls swapping of data read from buffer 00874 in the YCbCr422 format */ 00875 read.gr_rdswa = (vdc5_wr_rd_swa_t ) wr_rd_swa; /* Frame buffer swap setting */ 00876 /* Display area */ 00877 read.gr_grc .vs = gr_rect->vs; 00878 read.gr_grc .vw = gr_rect->vw; 00879 read.gr_grc .hs = gr_rect->hs; 00880 read.gr_grc .hw = gr_rect->hw; 00881 00882 /* Read data control */ 00883 error = R_VDC5_ReadDataControl(ch, vdc5_layer_id, &read); 00884 if (error != VDC5_OK ) { 00885 drv_error = DRV_GRAPHICS_VDC5_ERR; 00886 } 00887 } 00888 return drv_error; 00889 } /* End of function DRV_Graphics_Read_Setting() */ 00890 00891 /**************************************************************************//** 00892 * @brief Graphics surface read buffer change process 00893 * @param[in] layer_id : Graphics layer ID 00894 * @param[in] framebuff : Base address of the frame buffer 00895 * @retval Error code 00896 ******************************************************************************/ 00897 drv_graphics_error_t DRV_Graphics_Read_Change(drv_graphics_layer_t layer_id, 00898 void * framebuff) { 00899 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 00900 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 00901 vdc5_error_t error; 00902 vdc5_layer_id_t vdc5_layer_id; 00903 vdc5_read_chg_t read_chg; 00904 00905 switch (layer_id) { 00906 case DRV_GRAPHICS_LAYER_0: 00907 vdc5_layer_id = VDC5_LAYER_ID_0_RD ; 00908 break; 00909 case DRV_GRAPHICS_LAYER_1: 00910 vdc5_layer_id = VDC5_LAYER_ID_1_RD ; 00911 break; 00912 case DRV_GRAPHICS_LAYER_2: 00913 vdc5_layer_id = VDC5_LAYER_ID_2_RD ; 00914 break; 00915 case DRV_GRAPHICS_LAYER_3: 00916 vdc5_layer_id = VDC5_LAYER_ID_3_RD ; 00917 break; 00918 default: 00919 drv_error = DRV_GRAPHICS_LAYER_ERR; 00920 break; 00921 } 00922 00923 if (drv_error == DRV_GRAPHICS_OK) { 00924 /* Read data parameter */ 00925 read_chg.width_read_fb = NULL; /* Width of the image read from frame buffer */ 00926 read_chg.gr_grc = NULL; /* Display area */ 00927 read_chg.gr_disp_sel = NULL; /* Graphics display mode */ 00928 read_chg.gr_base = framebuff; /* Frame buffer base address */ 00929 00930 /* Change read process */ 00931 error = R_VDC5_ChangeReadProcess(ch, vdc5_layer_id, &read_chg); 00932 if (error != VDC5_OK ) { 00933 drv_error = DRV_GRAPHICS_VDC5_ERR; 00934 } 00935 } 00936 return drv_error; 00937 } /* End of function DRV_Graphics_Read_Change() */ 00938 00939 //touch_pos_t dot_border 00940 void Slide(uint32_t fb_stride, vdc5_chg_read_chg_t * param, slide_dir_t* slide_dir) { 00941 00942 #define SLIDE_STEP 12 00943 00944 static int32_t v_line = 0; /* Vertical direction offset variable */ 00945 static int32_t h_pixel = 0; /* Horizontal direction offset variable */ 00946 int32_t stride_pix = fb_stride / LCD_CH0_DISP_HW; /* Size(px) of image buffer stride */ 00947 uint16_t *pos_buff = (uint16_t *) param->gr_base ; /* Image buffer position */ 00948 00949 /* Wait Vsync */ 00950 00951 switch (*slide_dir) { 00952 case SLIDE_OUT_RIGHT: 00953 h_pixel += SLIDE_STEP; /* Increment offset value : 0u -> 800u by 16u step */ 00954 if (h_pixel >= LCD_CH0_DISP_HW) /* 800u */ 00955 { 00956 h_pixel = LCD_CH0_DISP_HW; 00957 *slide_dir = SLIDE_NONE; 00958 } 00959 param->gr_grc ->hs = (uint16_t) ((int32_t) LCD_CH0_DISP_HS + h_pixel); 00960 param->gr_grc ->hw = (uint16_t) ((int32_t) LCD_CH0_DISP_HW - h_pixel); 00961 break; 00962 case SLIDE_IN_RIGHT: 00963 h_pixel -= SLIDE_STEP; /* Decrement offset value : 800u -> 0u by 16u step */ 00964 if (h_pixel <= 0) /* 0u */ 00965 { 00966 h_pixel = 0; 00967 *slide_dir = SLIDE_NONE;; 00968 } 00969 param->gr_grc ->hs = (uint16_t) ((int32_t) LCD_CH0_DISP_HS + h_pixel); 00970 param->gr_grc ->hw = (uint16_t) ((int32_t) LCD_CH0_DISP_HW - h_pixel); 00971 break; 00972 00973 case SLIDE_OUT_BOTTOM: 00974 v_line += SLIDE_STEP; /* Increment offset value : 0u -> 600u by 16u step */ 00975 if (v_line >= LCD_CH0_DISP_VW) /* 600u */ 00976 { 00977 v_line = LCD_CH0_DISP_VW; 00978 *slide_dir = SLIDE_NONE;; 00979 } 00980 param->gr_grc ->vs = (uint16_t) ((int32_t) LCD_CH0_DISP_VS + v_line); 00981 param->gr_grc ->vw = (uint16_t) ((int32_t) LCD_CH0_DISP_VW - v_line); 00982 break; 00983 case SLIDE_IN_BOTTOM: 00984 v_line -= SLIDE_STEP; /* Decrement offset value : 600u -> 0u by 16u step */ 00985 if (v_line <= 0) /* 0u */ 00986 { 00987 v_line = 0; 00988 *slide_dir = SLIDE_NONE;; 00989 } 00990 param->gr_grc ->vs = (uint16_t) ((int32_t) LCD_CH0_DISP_VS + v_line); 00991 param->gr_grc ->vw = (uint16_t) ((int32_t) LCD_CH0_DISP_VW - v_line); 00992 break; 00993 00994 case SLIDE_OUT_LEFT: 00995 h_pixel += SLIDE_STEP; /* Increment offset value : 0u -> 800u by 16u step */ 00996 if (h_pixel >= LCD_CH0_DISP_HW) /* 800u */ 00997 { 00998 h_pixel = LCD_CH0_DISP_HW; 00999 *slide_dir = SLIDE_NONE;; 01000 } 01001 param->gr_base = &pos_buff[h_pixel]; 01002 param->gr_grc ->hw = (uint16_t) ((int32_t) LCD_CH0_DISP_HW - h_pixel); 01003 break; 01004 case SLIDE_IN_LEFT: 01005 h_pixel -= SLIDE_STEP; /* Decrement offset value : 800u -> 0u by 16u step */ 01006 if (h_pixel <= 0) /* 0u */ 01007 { 01008 h_pixel = 0; 01009 *slide_dir = SLIDE_NONE;; 01010 } 01011 param->gr_base = &pos_buff[h_pixel]; 01012 param->gr_grc ->hw = (uint16_t) ((int32_t) LCD_CH0_DISP_HW - h_pixel); 01013 break; 01014 01015 case SLIDE_OUT_TOP: 01016 v_line += SLIDE_STEP; /* Increment offset value : 0u -> 800u by 16u step */ 01017 if (v_line >= LCD_CH0_DISP_VW) /* 600u */ 01018 { 01019 v_line = LCD_CH0_DISP_VW; 01020 *slide_dir = SLIDE_NONE;; 01021 } 01022 param->gr_base = &pos_buff[v_line * stride_pix]; 01023 param->gr_grc ->vw = (uint16_t) ((int32_t) LCD_CH0_DISP_VW - v_line); 01024 break; 01025 case SLIDE_IN_TOP: 01026 v_line -= SLIDE_STEP; /* Decrement offset value : 800u -> 0u by 16u step */ 01027 if (v_line <= 0) /* 0u */ 01028 { 01029 v_line = 0; 01030 *slide_dir = SLIDE_NONE;; 01031 } 01032 param->gr_base = &pos_buff[v_line * stride_pix]; 01033 param->gr_grc ->vw = (uint16_t) ((int32_t) LCD_CH0_DISP_VW - v_line); 01034 break; 01035 01036 default: 01037 *slide_dir = SLIDE_NONE; 01038 h_pixel = 0; 01039 v_line = 0; 01040 break; 01041 } 01042 } 01043 01044 /**************************************************************************//** 01045 * @brief Graphics surface read buffer change process 01046 * @param[in] layer_id : Graphics layer ID 01047 * @param[in] framebuff : Base address of the frame buffer 01048 * @retval Error code 01049 ******************************************************************************/ 01050 drv_graphics_error_t DRV_Graphics_Read_Change_More( 01051 drv_graphics_layer_t layer_id, void * framebuff, uint32_t fb_stride, void * slide_dir) { 01052 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 01053 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 01054 vdc5_error_t error; 01055 vdc5_layer_id_t vdc5_layer_id; 01056 vdc5_chg_read_chg_t read_chg; 01057 01058 vdc5_period_rect_t grN_grc = { LCD_CH0_DISP_VS, LCD_CH0_DISP_VW, 01059 LCD_CH0_DISP_HS, LCD_CH0_DISP_HW }; 01060 01061 switch (layer_id) { 01062 case DRV_GRAPHICS_LAYER_0: 01063 vdc5_layer_id = VDC5_LAYER_ID_0_RD ; 01064 break; 01065 case DRV_GRAPHICS_LAYER_1: 01066 vdc5_layer_id = VDC5_LAYER_ID_1_RD ; 01067 break; 01068 case DRV_GRAPHICS_LAYER_2: 01069 vdc5_layer_id = VDC5_LAYER_ID_2_RD ; 01070 break; 01071 case DRV_GRAPHICS_LAYER_3: 01072 vdc5_layer_id = VDC5_LAYER_ID_3_RD ; 01073 break; 01074 default: 01075 drv_error = DRV_GRAPHICS_LAYER_ERR; 01076 break; 01077 } 01078 01079 if (drv_error == DRV_GRAPHICS_OK) { 01080 /* Read data parameter */ 01081 01082 read_chg.width_read_fb = NULL; /* Width of the image read from frame buffer */ 01083 read_chg.gr_grc = &grN_grc; /* Display area */ 01084 read_chg.gr_disp_sel = NULL; /* Graphics display mode */ 01085 read_chg.gr_base = framebuff; 01086 01087 Slide( fb_stride, &read_chg, (slide_dir_t*)slide_dir); 01088 01089 //read_chg.gr_base = framebuff; /* Frame buffer base address */ 01090 01091 /* Change read process */ 01092 error = R_VDC5_ChangeReadProcess(ch, vdc5_layer_id, 01093 (vdc5_read_chg_t *) &read_chg); 01094 if (error != VDC5_OK ) { 01095 drv_error = DRV_GRAPHICS_VDC5_ERR; 01096 } 01097 } 01098 return drv_error; 01099 } /* End of function DRV_Graphics_Read_Change_More() */ 01100 01101 /**************************************************************************//** 01102 * @brief Video surface write process setting 01103 * 01104 * Description:<br> 01105 * This function set the video write process. Input form is weave 01106 * (progressive) mode fixed. 01107 * This function supports the following 3 image format. 01108 * YCbCr422, RGB565, RGB888 01109 * @param[in] video_input_ch : Video input channel 01110 * @param[in] col_sys : Analog video signal color system 01111 * @param[in] adc_vinsel : Video input pin 01112 * @param[in] framebuff : Base address of the frame buffer 01113 * @param[in] fb_stride [byte] : Line offset address of the frame buffer 01114 * @param[in] video_format : Frame buffer video-signal writing format 01115 * @param[in] wr_rd_swa : Frame buffer swap setting 01116 * @param[in] video_write_size_vw [px]: output height 01117 * @param[in] video_write_size_hw [px]: output width 01118 * @param[in] video_adc_vinsel : Input pin control 01119 * @retval Error code 01120 ******************************************************************************/ 01121 drv_graphics_error_t DRV_Video_Write_Setting( 01122 drv_video_input_channel_t video_input_ch, 01123 drv_graphics_video_col_sys_t col_sys, void * framebuff, 01124 uint32_t fb_stride, drv_video_format_t video_format, 01125 drv_wr_rd_swa_t wr_rd_swa, uint16_t video_write_buff_vw, 01126 uint16_t video_write_buff_hw, drv_video_adc_vinsel_t video_adc_vinsel) { 01127 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 01128 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 01129 vdc5_error_t error; 01130 vdc5_layer_id_t vdc5_layer_id; 01131 vdc5_write_t write; 01132 vdc5_scalingdown_rot_t * scldw_rot; 01133 vdc5_res_md_t res_md; 01134 drv_rect_t video_in_rect; 01135 uint8_t * framebuffer_t; 01136 uint8_t * framebuffer_b; 01137 01138 if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_0) { 01139 GRAPHICS_VideoDecoderInit((vdec_adc_vinsel_t ) video_adc_vinsel, 01140 VDEC_CHANNEL_0 , (graphics_col_sys_t ) col_sys); 01141 } else if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_1) { 01142 GRAPHICS_VideoDecoderInit((vdec_adc_vinsel_t ) video_adc_vinsel, 01143 VDEC_CHANNEL_1 , (graphics_col_sys_t ) col_sys); 01144 } else { 01145 drv_error = DRV_GRAPHICS_CHANNEL_ERR; 01146 } 01147 01148 if (drv_error == DRV_GRAPHICS_OK) { 01149 if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_0) { 01150 vdc5_layer_id = VDC5_LAYER_ID_0_WR ; 01151 } else if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_1) { 01152 vdc5_layer_id = VDC5_LAYER_ID_1_WR ; 01153 } else { 01154 drv_error = DRV_GRAPHICS_CHANNEL_ERR; 01155 } 01156 } 01157 01158 if (drv_error == DRV_GRAPHICS_OK) { 01159 if (video_format == DRV_VIDEO_FORMAT_YCBCR422) { 01160 res_md = VDC5_RES_MD_YCBCR422 ; 01161 } else if (video_format == DRV_VIDEO_FORMAT_RGB888) { 01162 res_md = VDC5_RES_MD_RGB888 ; 01163 } else if (video_format == DRV_VIDEO_FORMAT_RGB565) { 01164 res_md = VDC5_RES_MD_RGB565 ; 01165 } else { 01166 drv_error = DRV_GRAPHICS_FORMAT_ERR; 01167 } 01168 } 01169 01170 if (col_sys == DRV_COL_SYS_NTSC_358 || col_sys == DVV_COL_SYS_NTSC_443 01171 || col_sys == DRV_COL_SYS_NTSC_443_60) { 01172 video_in_rect.hs = IMGCAP_SIZE_NTSC_HS * 2; 01173 video_in_rect.hw = IMGCAP_SIZE_NTSC_HW * 2; 01174 video_in_rect.vs = IMGCAP_SIZE_NTSC_VS; 01175 video_in_rect.vw = IMGCAP_SIZE_NTSC_VW; 01176 } else { 01177 video_in_rect.hs = IMGCAP_SIZE_PAL_HS * 2; 01178 video_in_rect.hw = IMGCAP_SIZE_PAL_HW * 2; 01179 video_in_rect.vs = IMGCAP_SIZE_PAL_VS; 01180 video_in_rect.vw = IMGCAP_SIZE_PAL_VW; 01181 } 01182 01183 #ifdef NO_SCALE_UP 01184 if( drv_error == DRV_GRAPHICS_OK ) { 01185 if( col_sys == DRV_COL_SYS_NTSC_358 || col_sys == DVV_COL_SYS_NTSC_443 || col_sys == DRV_COL_SYS_NTSC_443_60 ) { 01186 if( (video_write_buff_vw / 2u) > video_in_rect.vw ) { 01187 drv_error = DRV_GRAPHICS_VIDEO_NTSC_SIZE_ERR; 01188 } 01189 } else { 01190 if( (video_write_buff_vw / 2u) > video_in_rect.vw ) { 01191 drv_error = DRV_GRAPHICS_VIDEO_PAL_SIZE_ERR; 01192 } 01193 } 01194 } 01195 01196 if( drv_error == DRV_GRAPHICS_OK ) { 01197 if( video_write_buff_hw > 800 ) { 01198 drv_error = DRV_GRAPHICS_PARAM_RANGE_ERR; 01199 } 01200 } 01201 #endif 01202 01203 if (drv_error == DRV_GRAPHICS_OK) { 01204 /* Scaling-down and rotation parameter */ 01205 scldw_rot = &write.scalingdown_rot ; 01206 /* Image area to be captured */ 01207 scldw_rot->res .vs = (uint16_t) ((uint32_t) video_in_rect.vs - 1u); 01208 scldw_rot->res .vw = video_in_rect.vw; 01209 scldw_rot->res .hs = video_in_rect.hs; 01210 scldw_rot->res .hw = video_in_rect.hw; 01211 01212 /* Width of the image output from scaling-down control block 01213 Width of the image read from frame buffer */ 01214 if (video_write_buff_vw > LCD_CH0_DISP_VW) { 01215 video_write_buff_vw = LCD_CH0_DISP_VW; 01216 } 01217 01218 if (video_write_buff_hw > LCD_CH0_DISP_HW) { 01219 video_write_buff_hw = LCD_CH0_DISP_HW; 01220 } 01221 01222 //scldw_rot->res 01223 /* Write data parameter */ 01224 framebuffer_t = framebuff; 01225 framebuffer_b = &framebuffer_t[fb_stride]; 01226 scldw_rot->res_pfil_sel = VDC5_ON ; /* Prefilter mode select for brightness signals (on/off) */ 01227 scldw_rot->res_out_vw = video_write_buff_vw / 2u; /* Number of valid lines in vertical direction 01228 output by scaling-down control block */ 01229 scldw_rot->res_out_hw = video_write_buff_hw; /* Number of valid horizontal pixels 01230 output by scaling-down control block */ 01231 scldw_rot->adj_sel = VDC5_ON ; /* Measures to decrease the influence 01232 by lack of last-input line (on/off) */ 01233 scldw_rot->res_ds_wr_md = VDC5_WR_MD_NORMAL ; /* Frame buffer writing mode */ 01234 write.res_wrswa = (vdc5_wr_rd_swa_t ) wr_rd_swa; /* Frame buffer swap setting */ 01235 write.res_md = res_md; /* Frame buffer video-signal writing format */ 01236 write.res_bst_md = VDC5_BST_MD_32BYTE ; /* Transfer burst length for frame buffer */ 01237 write.res_inter = VDC5_RES_INTER_PROGRESSIVE ; /* Field operating mode select */ 01238 write.res_fs_rate = VDC5_RES_FS_RATE_PER1; /* Writing rate */ 01239 write.res_fld_sel = VDC5_RES_FLD_SEL_TOP ; /* Write field select */ 01240 write.res_dth_on = VDC5_ON ; /* Dither correction on/off */ 01241 write.base = framebuff; /* Frame buffer base address */ 01242 write.ln_off = fb_stride * 2u; /* Frame buffer line offset address [byte] */ 01243 write.flm_num = (uint32_t) (1u - 1u); /* Number of frames of buffer (res_flm_num + 1) */ 01244 /* Frame buffer frame offset address */ 01245 write.flm_off = fb_stride * 2u * (uint32_t) scldw_rot->res_out_vw ; 01246 write.btm_base = framebuffer_b; /* Frame buffer base address for bottom */ 01247 01248 /* Write data control */ 01249 error = R_VDC5_WriteDataControl(ch, vdc5_layer_id, &write); 01250 if (error != VDC5_OK ) { 01251 drv_error = DRV_GRAPHICS_VDC5_ERR; 01252 } 01253 } 01254 return drv_error; 01255 } /* End of function DRV_Video_Write_Setting() */ 01256 01257 /**************************************************************************//** 01258 * @brief Video surface write process setting for digital input 01259 * 01260 * Description:<br> 01261 * This function set the video write process for digital input. 01262 * This function supports the following 3 image format. 01263 * YCbCr422, RGB565, RGB888 01264 * @param[in] framebuff : Base address of the frame buffer 01265 * @param[in] fb_stride [byte] : Line offset address of the frame buffer 01266 * @param[in] video_format : Frame buffer video-signal writing format 01267 * @param[in] wr_rd_swa : Frame buffer swap setting 01268 * @param[in] video_write_size_vw [px]: output height 01269 * @param[in] video_write_size_hw [px]: output width 01270 * @param[in] cap_area : Capture area 01271 * @retval Error code 01272 ******************************************************************************/ 01273 drv_graphics_error_t DRV_Video_Write_Setting_Digital(void * framebuff, 01274 uint32_t fb_stride, drv_video_format_t video_format, 01275 drv_wr_rd_swa_t wr_rd_swa, uint16_t video_write_buff_vw, 01276 uint16_t video_write_buff_hw, drv_rect_t * cap_area) { 01277 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 01278 vdc5_channel_t ch = VDC5_CHANNEL_0 ; 01279 vdc5_error_t error; 01280 vdc5_layer_id_t vdc5_layer_id; 01281 vdc5_write_t write; 01282 vdc5_scalingdown_rot_t * scldw_rot; 01283 vdc5_res_md_t res_md; 01284 01285 vdc5_layer_id = VDC5_LAYER_ID_0_WR ; 01286 01287 if (drv_error == DRV_GRAPHICS_OK) { 01288 if (video_format == DRV_VIDEO_FORMAT_YCBCR422) { 01289 res_md = VDC5_RES_MD_YCBCR422 ; 01290 } else if (video_format == DRV_VIDEO_FORMAT_RGB888) { 01291 res_md = VDC5_RES_MD_RGB888 ; 01292 } else if (video_format == DRV_VIDEO_FORMAT_RGB565) { 01293 res_md = VDC5_RES_MD_RGB565 ; 01294 } else { 01295 drv_error = DRV_GRAPHICS_FORMAT_ERR; 01296 } 01297 } 01298 01299 if (drv_error == DRV_GRAPHICS_OK) { 01300 /* Scaling-down and rotation parameter */ 01301 scldw_rot = &write.scalingdown_rot ; 01302 /* Image area to be captured */ 01303 scldw_rot->res .vs = (uint16_t) ((uint32_t) cap_area->vs - 1u); 01304 scldw_rot->res .vw = cap_area->vw; 01305 scldw_rot->res .hs = cap_area->hs; 01306 scldw_rot->res .hw = cap_area->hw; 01307 01308 /* Write data parameter */ 01309 scldw_rot->res_pfil_sel = VDC5_ON ; /* Prefilter mode select for brightness signals (on/off) */ 01310 scldw_rot->res_out_vw = video_write_buff_vw; /* Number of valid lines in vertical direction 01311 output by scaling-down control block */ 01312 scldw_rot->res_out_hw = video_write_buff_hw; /* Number of valid horizontal pixels 01313 output by scaling-down control block */ 01314 scldw_rot->adj_sel = VDC5_ON ; /* Measures to decrease the influence 01315 by lack of last-input line (on/off) */ 01316 scldw_rot->res_ds_wr_md = VDC5_WR_MD_NORMAL ; /* Frame buffer writing mode */ 01317 write.res_wrswa = (vdc5_wr_rd_swa_t ) wr_rd_swa; /* Frame buffer swap setting */ 01318 write.res_md = res_md; /* Frame buffer video-signal writing format */ 01319 write.res_bst_md = VDC5_BST_MD_32BYTE ; /* Transfer burst length for frame buffer */ 01320 write.res_inter = VDC5_RES_INTER_PROGRESSIVE ; /* Field operating mode select */ 01321 write.res_fs_rate = VDC5_RES_FS_RATE_PER1; /* Writing rate */ 01322 write.res_fld_sel = VDC5_RES_FLD_SEL_TOP ; /* Write field select */ 01323 write.res_dth_on = VDC5_ON ; /* Dither correction on/off */ 01324 write.base = framebuff; /* Frame buffer base address */ 01325 write.ln_off = fb_stride; 01326 /* Frame buffer line offset address [byte] */ 01327 write.flm_num = (uint32_t) (1u - 1u); /* Number of frames of buffer (res_flm_num + 1) */ 01328 /* Frame buffer frame offset address */ 01329 write.flm_off = fb_stride * (uint32_t) scldw_rot->res_out_vw ; 01330 write.btm_base = NULL; /* Frame buffer base address for bottom */ 01331 01332 /* Write data control */ 01333 error = R_VDC5_WriteDataControl(ch, vdc5_layer_id, &write); 01334 if (error != VDC5_OK ) { 01335 drv_error = DRV_GRAPHICS_VDC5_ERR; 01336 } 01337 } 01338 return drv_error; 01339 } /* End of function DRV_Video_Write_Setting_Digital() */ 01340 01341 /**************************************************************************//** 01342 * @brief Video surface write buffer change process 01343 * @param[in] video_input_ch : Video input channle 01344 * @param[in] framebuff : Base address of the frame buffer 01345 * @param[in] fb_stride : Line offset address of the frame buffer 01346 * @retval Error code 01347 ******************************************************************************/ 01348 drv_graphics_error_t DRV_Video_Write_Change( 01349 drv_video_input_channel_t video_input_ch, void * framebuff, 01350 uint32_t fb_stride) { 01351 drv_graphics_error_t drv_error = DRV_GRAPHICS_OK; 01352 uint8_t * framebuffer_t; 01353 uint8_t * framebuffer_b; 01354 01355 framebuffer_t = (uint8_t *) ((uint32_t) framebuff & ~0x1F); 01356 framebuffer_b = &framebuffer_t[fb_stride]; 01357 01358 if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_0) { 01359 VDC50.SC0_SCL1_WR2 = (uint32_t) framebuffer_t; 01360 VDC50.SC0_SCL1_WR8 = (uint32_t) framebuffer_b; 01361 VDC50.SC0_SCL1_UPDATE = 0x10; 01362 } else if (video_input_ch == DRV_VIDEO_INPUT_CHANNEL_1) { 01363 VDC50.SC1_SCL1_WR2 = (uint32_t) framebuffer_t; 01364 VDC50.SC1_SCL1_WR8 = (uint32_t) framebuffer_b; 01365 VDC50.SC1_SCL1_UPDATE = 0x10; 01366 } else { 01367 drv_error = DRV_GRAPHICS_CHANNEL_ERR; 01368 } 01369 return drv_error; 01370 } /* End of function DRV_Video_Write_Change() */ 01371 01372 /* End of file */ 01373
Generated on Tue Jul 12 2022 14:32:48 by
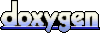