A class for driving a DC motor using a full-bridge (H-bridge) driver.
Dependencies: RateLimiter
Dependents: L298N-Breakout-Test Zavrsni_rad_NXP_cup
HBridgeDCMotor Class Reference
A class for driving a DC motor using a full-bridge driver (H-bridge). More...
#include <HBridgeDCMotor.h>
Public Member Functions | |
HBridgeDCMotor (PinName GH_A, PinName GL_A, PinName GH_B, PinName GL_B) | |
Constructor for independently driven transistors. | |
HBridgeDCMotor (PinName GH_A, PinName GH_B) | |
Constructor for complementary driven transistors. | |
void | configure (float sampleTime, float switchingFrequency, float rampUpTime, float rampDownTime) |
Configure the parameters. | |
void | setDutyCycle (float dutyCycle) |
Set the motor speed by changing a duty cycle value. | |
void | coast () |
Set the drive in a coast mode. | |
float | getDutyCycle () |
Get the current duty cycle. |
Detailed Description
A class for driving a DC motor using a full-bridge driver (H-bridge).
The class has an option to drive all 4 transistors gates independently by using 4 PwmOut objects, or to drive the H-bridge with complementary driven transistors using only 2 PwmOut objects.
Example of use:
#include "mbed.h" #include "HBridgeDCMotor.h" HBridgeDCMotor motor(p21, p22); int main() { float sampleTime = 50e-3, switchingFrequency = 25e3, rampTime = 3; motor.configure(sampleTime, switchingFrequency, rampTime, rampTime); while(true) { motor.setDutyCycle(1); wait(10); motor.setDutyCycle(-1); wait(10); } }
Definition at line 29 of file HBridgeDCMotor.h.
Constructor & Destructor Documentation
HBridgeDCMotor | ( | PinName | GH_A, |
PinName | GL_A, | ||
PinName | GH_B, | ||
PinName | GL_B | ||
) |
Constructor for independently driven transistors.
H stands for high side and L for low side transistor. A and B designate the individual half-bridges.
- Parameters:
-
GH_A PWM signal for driving the high side transistor of the half-bridge A GL_A PWM signal for driving the low side transistor of the half-bridge A GH_B PWM signal for driving the high side transistor of the half-bridge B GL_B PWM signal for driving the low side transistor of the half-bridge B
Definition at line 3 of file HBridgeDCMotor.cpp.
HBridgeDCMotor | ( | PinName | GH_A, |
PinName | GH_B | ||
) |
Constructor for complementary driven transistors.
The high side transistors are driven directly, while the low side transistors are complemented by the driver itself using logic circuits. A and B designate the individual half-bridges.
- Parameters:
-
GH_A PWM signal for driving the high side transistor of the half-bridge A GH_B PWM signal for driving the high side transistor of the half-bridge B
Definition at line 13 of file HBridgeDCMotor.cpp.
Member Function Documentation
void coast | ( | ) |
Set the drive in a coast mode.
Definition at line 72 of file HBridgeDCMotor.cpp.
void configure | ( | float | sampleTime, |
float | switchingFrequency, | ||
float | rampUpTime, | ||
float | rampDownTime | ||
) |
Configure the parameters.
- Parameters:
-
sampleTime sample time in seconds switchingFrequency switching frequency in Hz rampUpTime set the ramp up time (in seconds) from 0 to maximum speed rampDownTime set the ramp down time (in seconds) from maximum speed to 0
Definition at line 27 of file HBridgeDCMotor.cpp.
float getDutyCycle | ( | ) |
Get the current duty cycle.
- Returns:
- the current value of the duty cycle.
Definition at line 84 of file HBridgeDCMotor.cpp.
void setDutyCycle | ( | float | dutyCycle ) |
Set the motor speed by changing a duty cycle value.
- Parameters:
-
dutyCycle Duty cycle value in a range of (-1, 1). Negative value means opposite direction.
Definition at line 63 of file HBridgeDCMotor.cpp.
Generated on Fri Jul 15 2022 14:36:58 by
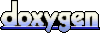