BMI160 Initial
Dependents: MAX32630HSP3_IMU_HelloWorld MAX32630HSP3_IMU_HelloWorld MAX32630HSP3_Pitch_Charles Maxim_Squeeks
bmi160.h
00001 /********************************************************************** 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 00034 #ifndef BMI160_H 00035 #define BMI160_H 00036 00037 #include "mbed.h" 00038 00039 #include "bmi160_defs.h" 00040 00041 #include <math.h> 00042 //#include <bmi160_math.h> 00043 /* 00044 #ifdef __KERNEL__ 00045 00046 #else 00047 00048 #include <string.h> 00049 */ 00050 #include <stdlib.h> 00051 00052 /** 00053 @brief The BMI160 is a small, low power, low noise 16-bit inertial measurement 00054 unit designed for use in mobile applications like augmented reality or indoor 00055 navigation which require highly accurate, real-time sensor data. 00056 00057 In full operation mode, with both the accelerometer and gyroscope enabled, the 00058 current consumption is typically 950 μA, enabling always-on applications in 00059 battery driven devices. It is available in a compact 14-pin 2.5 x 3.0 x 0.8 mm³ 00060 LGA package." 00061 00062 This class is an abstract base class and can not be instaniated, use BMI160_I2C 00063 or BMI160_SPI. 00064 */ 00065 class BMI160 00066 { 00067 public: 00068 00069 ///Return value on success. 00070 static const uint8_t RTN_NO_ERROR = 0; 00071 00072 ///Sensor types 00073 enum Sensors 00074 { 00075 MAG = 0, ///<Optional external sensor 00076 GYRO, ///<Angular rate sensor 00077 ACC ///<g sensor 00078 }; 00079 00080 ///Sensor Axis 00081 enum SensorAxis 00082 { 00083 X_AXIS = 0, 00084 Y_AXIS, 00085 Z_AXIS 00086 }; 00087 00088 ///Structure for axis data 00089 struct AxisData 00090 { 00091 int16_t raw; ///<Axis raw data 00092 float scaled; ///<Axis scaled data 00093 }; 00094 00095 ///Structure for sensor time data 00096 struct SensorTime 00097 { 00098 uint32_t raw; ///<raw SensorTime 00099 float seconds; ///<SensorTime as seconds 00100 }; 00101 00102 ///Structure for holding sensor data 00103 struct SensorData 00104 { 00105 AxisData xAxis; ///<Sensor X axis data 00106 AxisData yAxis; ///<Sensor Y axis data 00107 AxisData zAxis; ///<Sensor Z axis data 00108 }; 00109 00110 00111 ///BMI160 registers 00112 enum Registers 00113 { 00114 CHIP_ID = 0x00, ///<Chip Identification. 00115 ERR_REG = 0x02, ///<Reports sensor error flags. Flags reset when read. 00116 PMU_STATUS, ///<Reports current power mode for sensors. 00117 DATA_0, ///<MAG_X axis bits7:0 00118 DATA_1, ///<MAG_X axis bits15:8 00119 DATA_2, ///<MAG_Y axis bits7:0 00120 DATA_3, ///<MAG_Y axis bits15:8 00121 DATA_4, ///<MAG_Z axis bits7:0 00122 DATA_5, ///<MAG_Z axis bits15:8 00123 DATA_6, ///<RHALL bits7:0 00124 DATA_7, ///<RHALL bits15:8 00125 DATA_8, ///<GYR_X axis bits7:0 00126 DATA_9, ///<GYR_X axis bits15:8 00127 DATA_10, ///<GYR_Y axis bits7:0 00128 DATA_11, ///<GYR_Y axis bits15:8 00129 DATA_12, ///<GYR_Z axis bits7:0 00130 DATA_13, ///<GYR_Z axis bits15:8 00131 DATA_14, ///<ACC_X axis bits7:0 00132 DATA_15, ///<ACC_X axis bits15:8 00133 DATA_16, ///<ACC_Y axis bits7:0 00134 DATA_17, ///<ACC_Y axis bits15:8 00135 DATA_18, ///<ACC_Z axis bits7:0 00136 DATA_19, ///<ACC_Z axis bits15:8 00137 SENSORTIME_0, ///<24bit counter synchronized with data, bits7:0 00138 SENSORTIME_1, ///<24bit counter synchronized with data, bits15:8 00139 SENSORTIME_2, ///<24bit counter synchronized with data, bits23:16 00140 STATUS, ///<Reports sensors status flags 00141 INT_STATUS_0, ///<Contains interrupt status flags 00142 INT_STATUS_1, ///<Contains interrupt status flags 00143 INT_STATUS_2, ///<Contains interrupt status flags 00144 INT_STATUS_3, ///<Contains interrupt status flags 00145 TEMPERATURE_0, ///<Contains temperature of sensor, bits7:0 00146 TEMPERATURE_1, ///<Contains temperature of sensor, bits15:8 00147 FIFO_LENGTH_0, ///<Current fill level of FIFO, bits7:0 00148 FIFO_LENGTH_1, ///<Current fill level of FIFO, bits10:8 00149 FIFO_DATA, ///<FIFO data read out register, burst read 00150 ACC_CONF = 0x40, ///<Set ODR, bandwidth, and read mode of accelerometer 00151 ACC_RANGE, ///<Sets accelerometer g-range 00152 GYR_CONF = 0x42, ///<Set ODR, bandwidth, and read mode of gyroscope 00153 GYR_RANGE, ///<Sets gyroscope angular rate measurement range 00154 MAG_CONF, ///<Sets ODR of magnetometer interface 00155 FIFO_DOWNS, ///<Sets down sampling ratios of accel and gyro data 00156 ///<for FIFO 00157 FIFO_CONFIG_0, ///<Sets FIFO Watermark 00158 FIFO_CONFIG_1, ///<Sets which sensor data is available in FIFO, 00159 ///<Header/Headerless mode, Ext Int tagging, Sensortime 00160 MAG_IF_0 = 0x4B, ///<Magnetometer 7-bit I2C address, bits7:1 00161 MAG_IF_1, ///<Magnetometer interface configuration 00162 MAG_IF_2, ///<Magnetometer address to read 00163 MAG_IF_3, ///<Magnetometer address to write 00164 MAG_IF_4, ///<Magnetometer data to write 00165 INT_EN_0, ///<Interrupt enable bits 00166 INT_EN_1, ///<Interrupt enable bits 00167 INT_EN_2, ///<Interrupt enable bits 00168 INT_OUT_CTRL, ///<Contains the behavioral configuration of INT pins 00169 INT_LATCH, ///<Contains the interrupt rest bit and the interrupt 00170 ///<mode selection 00171 INT_MAP_0, ///<Controls which interrupt signals are mapped to the 00172 ///<INT1 and INT2 pins 00173 INT_MAP_1, ///<Controls which interrupt signals are mapped to the 00174 ///<INT1 and INT2 pins 00175 INT_MAP_2, ///<Controls which interrupt signals are mapped to the 00176 ///<INT1 and INT2 pins 00177 INT_DATA_0, ///<Contains the data source definition for the two 00178 ///<interrupt groups 00179 INT_DATA_1, ///<Contains the data source definition for the two 00180 ///<interrupt groups 00181 INT_LOWHIGH_0, ///<Contains the configuration for the low g interrupt 00182 INT_LOWHIGH_1, ///<Contains the configuration for the low g interrupt 00183 INT_LOWHIGH_2, ///<Contains the configuration for the low g interrupt 00184 INT_LOWHIGH_3, ///<Contains the configuration for the low g interrupt 00185 INT_LOWHIGH_4, ///<Contains the configuration for the low g interrupt 00186 INT_MOTION_0, ///<Contains the configuration for the any motion and 00187 ///<no motion interrupts 00188 INT_MOTION_1, ///<Contains the configuration for the any motion and 00189 ///<no motion interrupts 00190 INT_MOTION_2, ///<Contains the configuration for the any motion and 00191 ///<no motion interrupts 00192 INT_MOTION_3, ///<Contains the configuration for the any motion and 00193 ///<no motion interrupts 00194 INT_TAP_0, ///<Contains the configuration for the tap interrupts 00195 INT_TAP_1, ///<Contains the configuration for the tap interrupts 00196 INT_ORIENT_0, ///<Contains the configuration for the oeientation 00197 ///<interrupt 00198 INT_ORIENT_1, ///<Contains the configuration for the oeientation 00199 ///<interrupt 00200 INT_FLAT_0, ///<Contains the configuration for the flat interrupt 00201 INT_FLAT_1, ///<Contains the configuration for the flat interrupt 00202 FOC_CONF = 0x69, ///<Contains configuration for the fast offset 00203 ///<compensation for the accelerometer and gyroscope 00204 CONF, ///<Configuration of sensor, nvm_prog_en bit 00205 IF_CONF, ///<Contains settings for the digital interface 00206 PMU_TRIGGER, ///<Sets trigger conditions to change gyro power modes 00207 SELF_TEST, ///<Self test configuration 00208 NV_CONF = 0x70, ///<Contains settings for the digital interface 00209 OFFSET_0 = 0x71, ///<Contains offset comp values for acc_off_x7:0 00210 OFFSET_1 = 0x71, ///<Contains offset comp values for acc_off_y7:0 00211 OFFSET_2 = 0x71, ///<Contains offset comp values for acc_off_z7:0 00212 OFFSET_3 = 0x74, ///<Contains offset comp values for gyr_off_x7:0 00213 OFFSET_4 = 0x75, ///<Contains offset comp values for gyr_off_y7:0 00214 OFFSET_5 = 0x76, ///<Contains offset comp values for gyr_off_z7:0 00215 OFFSET_6 = 0x77, ///<gyr/acc offset enable bit and gyr_off_(zyx) bits9:8 00216 STEP_CNT_0, ///<Step counter bits 15:8 00217 STEP_CNT_1, ///<Step counter bits 7:0 00218 STEP_CONF_0, ///<Contains configuration of the step detector 00219 STEP_CONF_1, ///<Contains configuration of the step detector 00220 CMD = 0x7E ///<Command register triggers operations like 00221 ///<softreset, NVM programming, etc. 00222 }; 00223 00224 00225 ///@name ERR_REG(0x02) 00226 ///Error register data 00227 ///@{ 00228 00229 static const uint8_t FATAL_ERR_MASK = 0x01; 00230 static const uint8_t FATAL_ERR_POS = 0x00; 00231 static const uint8_t ERR_CODE_MASK = 0x1E; 00232 static const uint8_t ERR_CODE_POS = 0x01; 00233 static const uint8_t I2C_FAIL_ERR_MASK = 0x20; 00234 static const uint8_t I2C_FAIL_ERR_POS = 0x05; 00235 static const uint8_t DROP_CMD_ERR_MASK = 0x40; 00236 static const uint8_t DROP_CMD_ERR_POS = 0x06; 00237 static const uint8_t MAG_DRDY_ERR_MASK = 0x80; 00238 static const uint8_t MAG_DRDY_ERR_POS = 0x08; 00239 00240 static const uint8_t FOC_VALUE = 0x7D; 00241 static const uint8_t FOC_START = 0x03; 00242 static const uint8_t FOC_ENABLE_GYR_ACC = 0xC0; 00243 00244 ///Enumerated error codes 00245 enum ErrorCodes 00246 { 00247 NO_ERROR = 0, ///<No Error 00248 ERROR_1, ///<Listed as error 00249 ERROR_2, ///<Listed as error 00250 LPM_INT_PFD, ///<Low-power mode and interrupt uses pre-filtered 00251 ///<data 00252 ODR_MISMATCH = 0x06, ///<ODRs of enabled sensors in headerless mode do 00253 ///<not match 00254 PFD_USED_LPM ///<Pre-filtered data are used in low power mode 00255 }; 00256 ///@} 00257 00258 00259 ///@name ACC_CONF(0x40) and ACC_RANGE(0x41) 00260 ///Data for configuring accelerometer 00261 ///@{ 00262 00263 static const uint8_t ACC_ODR_MASK = 0x0F; 00264 static const uint8_t ACC_ODR_POS = 0x00; 00265 static const uint8_t ACC_BWP_MASK = 0x70; 00266 static const uint8_t ACC_BWP_POS = 0x04; 00267 static const uint8_t ACC_US_MASK = 0x80; 00268 static const uint8_t ACC_US_POS = 0x07; 00269 static const uint8_t ACC_RANGE_MASK = 0x0F; 00270 static const uint8_t ACC_RANGE_POS = 0x00; 00271 static const uint8_t GYRO_RANGE_500 = 0x02; 00272 00273 ///Accelerometer output data rates 00274 enum AccOutputDataRate 00275 { 00276 ACC_ODR_1 = 1, ///< 25/32Hz 00277 ACC_ODR_2, ///< 25/16Hz 00278 ACC_ODR_3, ///< 25/8Hz 00279 ACC_ODR_4, ///< 25/4Hz 00280 ACC_ODR_5, ///< 25/2Hz 00281 ACC_ODR_6, ///< 25Hz 00282 ACC_ODR_7, ///< 50Hz 00283 ACC_ODR_8, ///< 100Hz 00284 ACC_ODR_9, ///< 200Hz 00285 ACC_ODR_10, ///< 400Hz 00286 ACC_ODR_11, ///< 800Hz 00287 ACC_ODR_12 ///< 1600Hz 00288 }; 00289 00290 ///Accelerometer bandwidth parameters 00291 enum AccBandWidthParam 00292 { 00293 ACC_BWP_0 = 0, ///< Average 1 cycle; when acc_us = 0 OSR4 00294 ACC_BWP_1, ///< Average 2 cycles; when acc_us = 0 OSR2 00295 ACC_BWP_2, ///< Average 4 cycles; when acc_us = 0 normal mode 00296 ACC_BWP_3, ///< Average 8 cycles 00297 ACC_BWP_4, ///< Average 16 cycles 00298 ACC_BWP_5, ///< Average 32 cycles 00299 ACC_BWP_6, ///< Average 64 cycles 00300 ACC_BWP_7 ///< Average 128 cycles 00301 }; 00302 00303 ///Accelerometer undersampling 00304 enum AccUnderSampling 00305 { 00306 ACC_US_OFF = 0, 00307 ACC_US_ON 00308 }; 00309 00310 ///Accelerometer ranges 00311 enum AccRange 00312 { 00313 SENS_2G = 0x03, ///<Accelerometer range +-2G 00314 SENS_4G = 0x05, ///<Accelerometer range +-4G 00315 SENS_8G = 0x08, ///<Accelerometer range +-8G 00316 SENS_16G = 0x0C ///<Accelerometer range +-16G 00317 }; 00318 00319 00320 00321 00322 00323 ///Accelerometer configuration data structure 00324 struct AccConfig 00325 { 00326 AccRange range; ///<Accelerometer range 00327 AccUnderSampling us; ///<Accelerometr undersampling mode 00328 AccBandWidthParam bwp; ///<Accelerometer bandwidth param 00329 AccOutputDataRate odr; ///<Accelerometr output data rate 00330 }; 00331 00332 ///Accelerometer default configuration 00333 static const AccConfig DEFAULT_ACC_CONFIG; 00334 ///@} 00335 00336 00337 ///@name GYR_CONF(0x42) and GYR_RANGE(0x43) 00338 ///Data for configuring gyroscope 00339 ///@{ 00340 00341 static const uint8_t GYRO_ODR_MASK = 0x0F; 00342 static const uint8_t GYRO_ODR_POS = 0x00; 00343 static const uint8_t GYRO_BWP_MASK = 0x30; 00344 static const uint8_t GYRO_BWP_POS = 0x04; 00345 static const uint8_t GYRO_RANGE_MASK = 0x07; 00346 static const uint8_t GYRO_RANGE_POS = 0x00; 00347 00348 ///Gyroscope output data rates 00349 enum GyroOutputDataRate 00350 { 00351 GYRO_ODR_6 = 0x06, ///<25Hz 00352 GYRO_ODR_7 = 0x07, ///<50Hz 00353 GYRO_ODR_8 = 0x08, ///<100Hz 00354 GYRO_ODR_9 = 0x09, ///<200Hz 00355 GYRO_ODR_10 = 0x0A, ///<400Hz 00356 GYRO_ODR_11 = 0x0B, ///<800Hz 00357 GYRO_ODR_12 = 0x0C, ///<1600Hz 00358 GYRO_ODR_13 = 0x0D ///<3200Hz 00359 }; 00360 00361 ///Gyroscope bandwidth paramaters 00362 enum GyroBandWidthParam 00363 { 00364 GYRO_BWP_0 = 0, ///<OSR4 Over Sampling Rate of 4 00365 GYRO_BWP_1, ///<OSR2 Over Sampling Rate of 2 00366 GYRO_BWP_2 ///<Normal Mode, Equidistant Sampling 00367 }; 00368 00369 ///Gyroscope ranges 00370 enum GyroRange 00371 { 00372 DPS_2000 = 0, ///<+-2000dps, 16.4LSB/dps 00373 DPS_1000, ///<+-1000dps, 32.8LSB/dps 00374 DPS_500, ///<+-500dps, 65.6LSB/dps 00375 DPS_250, ///<+-250dps, 131.2LSB/dps 00376 DPS_125 ///<+-125dps, 262.4LSB/dps, 00377 }; 00378 00379 ///Gyroscope configuration data structure 00380 struct GyroConfig 00381 { 00382 GyroRange range; ///<Gyroscope range 00383 GyroBandWidthParam bwp; ///<Gyroscope bandwidth param 00384 GyroOutputDataRate odr; ///<Gyroscope output data rate 00385 }; 00386 00387 ///Gyroscope default configuration 00388 static const GyroConfig DEFAULT_GYRO_CONFIG; 00389 ///@} 00390 00391 00392 ///Enumerated power modes 00393 enum PowerModes 00394 { 00395 SUSPEND = 0, ///<Acc and Gyro, No sampling, No FIFO data readout 00396 NORMAL, ///<Acc and Gyro, Full chip operation 00397 LOW_POWER, ///<Acc duty-cycling between suspend and normal 00398 FAST_START_UP ///<Gyro start up delay time to normal mode <= 10 ms 00399 }; 00400 00401 00402 ///Enumerated commands used with CMD register 00403 enum Commands 00404 { 00405 START_FOC = 0x03, ///<Starts Fast Offset Calibrartion 00406 ACC_SET_PMU_MODE = 0x10, ///<Sets acc power mode 00407 GYR_SET_PMU_MODE = 0x14, ///<Sets gyro power mode 00408 MAG_SET_PMU_MODE = 0x18, ///<Sets mag power mode 00409 PROG_NVM = 0xA0, ///<Writes NVM backed registers into NVM 00410 FIFO_FLUSH = 0xB0, ///<Clears FIFO 00411 INT_RESET, ///<Clears interrupt engine, INT_STATUS, and 00412 ///<the interrupt pin 00413 STEP_CNT_CLR, ///<Triggers reset of the step counter 00414 SOFT_RESET = 0xB6 ///<Triggers a reset including a reboot. 00415 }; 00416 00417 00418 ///@brief BMI160 Destructor.\n 00419 /// 00420 ///On Entry: 00421 ///@param[in] none 00422 /// 00423 ///On Exit: 00424 ///@param[out] none 00425 /// 00426 ///@returns none 00427 virtual ~BMI160(){ } 00428 00429 00430 ///@brief Reads a single register.\n 00431 /// 00432 ///On Entry: 00433 ///@param[in] data - pointer to memory for storing read data 00434 /// 00435 ///On Exit: 00436 ///@param[out] data - holds contents of read register on success 00437 /// 00438 ///@returns 0 on success, non 0 on failure 00439 virtual int32_t readRegister(Registers reg, uint8_t *data) = 0; 00440 00441 00442 ///@brief Writes a single register.\n 00443 /// 00444 ///On Entry: 00445 ///@param[in] data - data to write to register 00446 /// 00447 ///On Exit: 00448 ///@param[out] none 00449 /// 00450 ///@returns 0 on success, non 0 on failure 00451 virtual int32_t writeRegister(Registers reg, const uint8_t data) = 0; 00452 00453 00454 ///@brief Reads a block of registers.\n 00455 ///@detail User must ensure that all registers between 'startReg' and 00456 ///'stopReg' exist and are readable. Function reads up to, including, 00457 ///'stopReg'.\n 00458 /// 00459 ///On Entry: 00460 ///@param[in] startReg - register to start reading from 00461 ///@param[in] stopReg - register to stop reading from 00462 ///@param[in] data - pointer to memory for storing read data 00463 /// 00464 ///On Exit: 00465 ///@param[out] data - holds contents of read registers on success 00466 /// 00467 ///@returns 0 on success, non 0 on failure 00468 virtual int32_t readBlock(Registers startReg, Registers stopReg, 00469 uint8_t *data) = 0; 00470 00471 00472 ///@brief Writes a block of registers.\n 00473 ///@detail User must ensure that all registers between 'startReg' and 00474 ///'stopReg' exist and are writeable. Function writes up to, including, 00475 ///'stopReg'.\n 00476 /// 00477 ///On Entry: 00478 ///@param[in] startReg - register to start writing at 00479 ///@param[in] stopReg - register to stop writing at 00480 ///@param[in] data - pointer to data to write to registers 00481 /// 00482 ///On Exit: 00483 ///@param[out] none 00484 /// 00485 ///@returns 0 on success, non 0 on failure 00486 virtual int32_t writeBlock(Registers startReg, Registers stopReg, 00487 const uint8_t *data) = 0; 00488 00489 00490 ///@brief Sets sensors power mode through CMD register.\n 00491 ///@details Observe command execution times given in datasheet.\n 00492 /// 00493 ///On Entry: 00494 ///@param[in] sensor - Sensor which power mode we are setting 00495 ///@param[in] pwrMode - Desired powermode of the sensor 00496 /// 00497 ///On Exit: 00498 ///@param[out] 00499 /// 00500 ///@returns 0 on success, non 0 on failure 00501 int32_t setSensorPowerMode(Sensors sensor, PowerModes pwrMode); 00502 00503 00504 ///@brief Configure sensor.\n 00505 /// 00506 ///On Entry: 00507 ///@param[in] config - sSensor configuration data structure 00508 /// 00509 ///On Exit: 00510 ///@param[out] none 00511 /// 00512 ///@returns 0 on success, non 0 on failure 00513 int32_t setSensorConfig(const AccConfig &config); 00514 int32_t setSensorConfig(const GyroConfig &config); 00515 00516 00517 ///@brief Get sensor configuration.\n 00518 /// 00519 ///On Entry: 00520 ///@param[in] config - Sensor configuration data structure 00521 /// 00522 ///On Exit: 00523 ///@param[out] config - on success, holds sensor's current 00524 ///configuration 00525 /// 00526 ///@returns 0 on success, non 0 on failure 00527 int32_t getSensorConfig(AccConfig &config); 00528 int32_t getSensorConfig(GyroConfig &config); 00529 00530 00531 ///@brief Get sensor axis.\n 00532 /// 00533 ///On Entry: 00534 ///@param[in] axis - Sensor axis 00535 ///@param[in] data - AxisData structure 00536 ///@param[in] range - Sensor range 00537 /// 00538 ///On Exit: 00539 ///@param[out] data - Structure holds raw and scaled axis data 00540 /// 00541 ///@returns 0 on success, non 0 on failure 00542 int32_t getSensorAxis(SensorAxis axis, AxisData &data, AccRange range); 00543 int32_t getSensorAxis(SensorAxis axis, AxisData &data, GyroRange range); 00544 00545 00546 ///@brief Get sensor xyz axis.\n 00547 /// 00548 ///On Entry: 00549 ///@param[in] data - SensorData structure 00550 ///@param[in] range - Sensor range 00551 /// 00552 ///On Exit: 00553 ///@param[out] data - Structure holds raw and scaled data for all three axis 00554 /// 00555 ///@returns 0 on success, non 0 on failure 00556 int32_t getSensorXYZ(SensorData &data, AccRange range); 00557 int32_t getSensorXYZ(SensorData &data, GyroRange range); 00558 00559 00560 ///@brief Get sensor xyz axis and sensor time.\n 00561 /// 00562 ///On Entry: 00563 ///@param[in] data - SensorData structure 00564 ///@param[in] sensorTime - SensorTime structure for data 00565 ///@param[in] range - Sensor range 00566 /// 00567 ///On Exit: 00568 ///@param[out] data - Structure holds raw and scaled data for all three axis 00569 ///@param[out] sensorTime - Holds sensor time on success 00570 /// 00571 ///@returns 0 on success, non 0 on failure 00572 int32_t getSensorXYZandSensorTime(SensorData &data, SensorTime &sensorTime, 00573 AccRange range); 00574 int32_t getSensorXYZandSensorTime(SensorData &data, SensorTime &sensorTime, 00575 GyroRange range); 00576 00577 00578 ///@brief Get Gyroscope/Accelerometer data and sensor time.\n 00579 /// 00580 ///On Entry: 00581 ///@param[in] accData - Sensor data structure for accelerometer 00582 ///@param[in] gyroData - Sensor data structure for gyroscope 00583 ///@param[in] sensorTime - SensorTime data structure 00584 ///@param[in] accRange - Accelerometer range 00585 ///@param[in] gyroRange - Gyroscope range 00586 /// 00587 ///On Exit: 00588 ///@param[out] accData - Synchronized accelerometer data 00589 ///@param[out] gyroData - Synchronized gyroscope data 00590 ///@param[out] sensorTime - Synchronized sensor time 00591 /// 00592 ///@returns 0 on success, non 0 on failure 00593 int32_t getGyroAccXYZandSensorTime(SensorData &accData, 00594 SensorData &gyroData, 00595 SensorTime &sensorTime, 00596 AccRange accRange, GyroRange gyroRange); 00597 00598 00599 ///@brief Get sensor time.\n 00600 /// 00601 ///On Entry: 00602 ///@param[in] sensorTime - SensorTime structure for data 00603 /// 00604 ///On Exit: 00605 ///@param[out] sensorTime - Holds sensor time on success 00606 /// 00607 ///@returns returns 0 on success, non 0 on failure 00608 int32_t getSensorTime(SensorTime &sensorTime); 00609 00610 00611 00612 00613 ///@brief Get die temperature.\n 00614 /// 00615 ///On Entry: 00616 ///@param[in] temp - pointer to float for temperature 00617 /// 00618 ///On Exit: 00619 ///@param[out] temp - on success, holds the die temperature 00620 /// 00621 ///@returns 0 on success, non 0 on failure 00622 int32_t getTemperature(float *temp); 00623 }; 00624 00625 00626 /** 00627 @brief BMI160_I2C - supports BMI160 object with I2C interface 00628 */ 00629 class BMI160_I2C: public BMI160 00630 { 00631 public: 00632 00633 ///BMI160 default I2C address. 00634 static const uint8_t I2C_ADRS_SDO_LO = 0x68; 00635 ///BMI160 optional I2C address. 00636 static const uint8_t I2C_ADRS_SDO_HI = 0x69; 00637 00638 00639 ///@brief BMI160_I2C Constructor.\n 00640 /// 00641 ///On Entry: 00642 ///@param[in] i2cBus - reference to I2C bus for this device 00643 ///@param[in] i2cAdrs - 7-bit I2C address 00644 /// 00645 ///On Exit: 00646 ///@param[out] none 00647 /// 00648 ///@returns none 00649 BMI160_I2C(I2C &i2cBus, uint8_t i2cAdrs); 00650 00651 00652 ///@brief Reads a single register.\n 00653 /// 00654 ///On Entry: 00655 ///@param[in] data - pointer to memory for storing read data 00656 /// 00657 ///On Exit: 00658 ///@param[out] data - holds contents of read register on success 00659 /// 00660 ///@returns 0 on success, non 0 on failure 00661 virtual int32_t readRegister(Registers reg, uint8_t *data); 00662 00663 00664 ///@brief Writes a single register.\n 00665 /// 00666 ///On Entry: 00667 ///@param[in] data - data to write to register 00668 /// 00669 ///On Exit: 00670 ///@param[out] none 00671 /// 00672 ///@returns 0 on success, non 0 on failure 00673 virtual int32_t writeRegister(Registers reg, const uint8_t data); 00674 00675 00676 ///@brief Reads a block of registers.\n 00677 ///@detail User must ensure that all registers between 'startReg' and 00678 ///'stopReg' exist and are readable. Function reads up to, including, 00679 ///'stopReg'.\n 00680 /// 00681 ///On Entry: 00682 ///@param[in] startReg - register to start reading from 00683 ///@param[in] stopReg - register to stop reading from 00684 ///@param[in] data - pointer to memory for storing read data 00685 /// 00686 ///On Exit: 00687 ///@param[out] data - holds contents of read registers on success 00688 /// 00689 ///@returns 0 on success, non 0 on failure 00690 virtual int32_t readBlock(Registers startReg, Registers stopReg, 00691 uint8_t *data); 00692 00693 00694 ///@brief Writes a block of registers.\n 00695 ///@detail User must ensure that all registers between 'startReg' and 00696 ///'stopReg' exist and are writeable. Function writes up to, including, 00697 ///'stopReg'.\n 00698 /// 00699 ///On Entry: 00700 ///@param[in] startReg - register to start writing at 00701 ///@param[in] stopReg - register to stop writing at 00702 ///@param[in] data - pointer to data to write to registers 00703 /// 00704 ///On Exit: 00705 ///@param[out] none 00706 /// 00707 ///@returns 0 on success, non 0 on failure 00708 virtual int32_t writeBlock(Registers startReg, Registers stopReg, 00709 const uint8_t *data); 00710 00711 private: 00712 00713 I2C &m_i2cBus; 00714 uint8_t m_Wadrs, m_Radrs; 00715 }; 00716 00717 00718 /** 00719 @brief BMI160_SPI - supports BMI160 object with SPI interface 00720 */ 00721 class BMI160_SPI: public BMI160 00722 { 00723 public: 00724 00725 ///@brief BMI160_SPI Constructor.\n 00726 /// 00727 ///On Entry: 00728 ///@param[in] spiBus - reference to SPI bus for this device 00729 ///@param[in] cs - reference to DigitalOut used for chip select 00730 /// 00731 ///On Exit: 00732 ///@param[out] none 00733 /// 00734 ///@returns none 00735 BMI160_SPI(SPI &spiBus, DigitalOut &cs); 00736 00737 00738 ///@brief Reads a single register.\n 00739 /// 00740 ///On Entry: 00741 ///@param[in] data - pointer to memory for storing read data 00742 /// 00743 ///On Exit: 00744 ///@param[out] data - holds contents of read register on success 00745 /// 00746 ///@returns 0 on success, non 0 on failure 00747 virtual int32_t readRegister(Registers reg, uint8_t *data); 00748 00749 00750 ///@brief Writes a single register.\n 00751 /// 00752 ///On Entry: 00753 ///@param[in] data - data to write to register 00754 /// 00755 ///On Exit: 00756 ///@param[out] none 00757 /// 00758 ///@returns 0 on success, non 0 on failure 00759 virtual int32_t writeRegister(Registers reg, const uint8_t data); 00760 00761 00762 ///@brief Reads a block of registers.\n 00763 ///@detail User must ensure that all registers between 'startReg' and 00764 ///'stopReg' exist and are readable. Function reads up to, including, 00765 ///'stopReg'.\n 00766 /// 00767 ///On Entry: 00768 ///@param[in] startReg - register to start reading from 00769 ///@param[in] stopReg - register to stop reading from 00770 ///@param[in] data - pointer to memory for storing read data 00771 /// 00772 ///On Exit: 00773 ///@param[out] data - holds contents of read registers on success 00774 /// 00775 ///@returns 0 on success, non 0 on failure 00776 virtual int32_t readBlock(Registers startReg, Registers stopReg, 00777 uint8_t *data); 00778 00779 00780 ///@brief Writes a block of registers.\n 00781 ///@detail User must ensure that all registers between 'startReg' and 00782 ///'stopReg' exist and are writeable. Function writes up to, including, 00783 ///'stopReg'.\n 00784 /// 00785 ///On Entry: 00786 ///@param[in] startReg - register to start writing at 00787 ///@param[in] stopReg - register to stop writing at 00788 ///@param[in] data - pointer to data to write to registers 00789 /// 00790 ///On Exit: 00791 ///@param[out] none 00792 /// 00793 ///@returns 0 on success, non 0 on failure 00794 virtual int32_t writeBlock(Registers startReg, Registers stopReg, 00795 const uint8_t *data); 00796 00797 private: 00798 00799 SPI &m_spiBus; 00800 DigitalOut m_cs; 00801 }; 00802 00803 #endif /* BMI160_H */ 00804 00805 00806 ///@brief fx documentation template.\n 00807 /// 00808 ///On Entry: 00809 ///@param[in] none 00810 /// 00811 ///On Exit: 00812 ///@param[out] none 00813 /// 00814 ///@returns none 00815 int8_t start_foc(struct bmi160_dev *dev); 00816 00817 int8_t bmi160_start_foc(const struct bmi160_foc_conf *foc_conf, struct bmi160_offsets *offset, 00818 struct bmi160_dev const *dev);
Generated on Wed Jul 13 2022 00:38:35 by
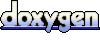