Driver for the Silicon Labs Si1133 Visible Light/UV sensor
Dependents: TBSense2_Sensor_Demo mbed-BLE-coragem-teste Pulga_BLE_GPS pulga-mbed-lorawan-gps ... more
Si1133 Class Reference
#include <Si1133.h>
Public Member Functions | |
Si1133 (PinName sda, PinName scl, int hz=400000) | |
Create an Si1133 object connected to the specified I2C pins with the specified I2C slave address. | |
~Si1133 (void) | |
Si1133 destructor. | |
bool | open () |
Probe for the Si1133 and try to initialize the sensor. | |
float | get_light_level () |
Measure the current light level (in lux) on the Si1133. | |
float | get_uv_index () |
Measure the current UV Index on the Si1133. | |
bool | get_light_and_uv (float *light_level, float *uv_index) |
Do a combined measurement and return both the light level and UV index. | |
operator float () | |
A shorthand for get_light_level() |
Detailed Description
Si1133 class.
Used for taking Light level and UV index measurements.
Example:
#include "mbed.h" #include "Si1133.h " //Create an Si1133 object Si1133 sensor(PC4, PC5); int main() { //Try to open the Si1133 if (sensor.open()) { printf("Device detected!\n"); while (1) { //Print the current light level printf("Lux = %.3f\n", (float)sensor.get_light_level()); //Print the current UV index printf("UV index = %.3f\n", (float)sensor.get_uv_index()); //Sleep for 0.5 seconds wait(0.5); } } else { error("Device not detected!\n"); } }
Definition at line 61 of file Si1133.h.
Constructor & Destructor Documentation
Si1133 | ( | PinName | sda, |
PinName | scl, | ||
int | hz = 400000 |
||
) |
Create an Si1133 object connected to the specified I2C pins with the specified I2C slave address.
- Parameters:
-
sda The I2C data pin. scl The I2C clock pin. hz The I2C bus frequency (defaults to 400kHz).
Definition at line 91 of file Si1133.cpp.
~Si1133 | ( | void | ) |
Si1133 destructor.
Definition at line 97 of file Si1133.cpp.
Member Function Documentation
bool get_light_and_uv | ( | float * | light_level, |
float * | uv_index | ||
) |
Do a combined measurement and return both the light level and UV index.
- Parameters:
-
[out] light_level Measured light level in Lux [out] uv_index Measured UV index
- Returns:
- true if measurement was successful
Definition at line 139 of file Si1133.cpp.
float get_light_level | ( | ) |
Measure the current light level (in lux) on the Si1133.
- Returns:
- The current temperature measurement in Lux.
Definition at line 121 of file Si1133.cpp.
float get_uv_index | ( | ) |
Measure the current UV Index on the Si1133.
- Returns:
- The current UV index measurement.
- The current UV index.
Definition at line 132 of file Si1133.cpp.
bool open | ( | ) |
Probe for the Si1133 and try to initialize the sensor.
- Returns:
- 'true' if the device exists on the bus, 'false' if the device doesn't exist on the bus.
Definition at line 102 of file Si1133.cpp.
operator float | ( | ) |
A shorthand for get_light_level()
- Returns:
- The current temperature measurement in Lux.
Generated on Sat Jul 16 2022 18:44:29 by
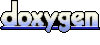