Driver for the Silicon Labs Si1133 Visible Light/UV sensor
Dependents: TBSense2_Sensor_Demo mbed-BLE-coragem-teste Pulga_BLE_GPS pulga-mbed-lorawan-gps ... more
Si1133.h
00001 /***************************************************************************//** 00002 * @file Si1133.h 00003 ******************************************************************************* 00004 * @section License 00005 * <b>(C) Copyright 2017 Silicon Labs, http://www.silabs.com</b> 00006 ******************************************************************************* 00007 * 00008 * SPDX-License-Identifier: Apache-2.0 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00011 * not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00018 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef SI1133_H 00025 #define SI1133_H 00026 00027 #include "mbed.h" 00028 00029 /** Si1133 class. 00030 * Used for taking Light level and UV index measurements. 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 * #include "Si1133.h" 00036 * 00037 * //Create an Si1133 object 00038 * Si1133 sensor(PC4, PC5); 00039 * 00040 * int main() 00041 * { 00042 * //Try to open the Si1133 00043 * if (sensor.open()) { 00044 * printf("Device detected!\n"); 00045 * 00046 * while (1) { 00047 * //Print the current light level 00048 * printf("Lux = %.3f\n", (float)sensor.get_light_level()); 00049 * //Print the current UV index 00050 * printf("UV index = %.3f\n", (float)sensor.get_uv_index()); 00051 * 00052 * //Sleep for 0.5 seconds 00053 * wait(0.5); 00054 * } 00055 * } else { 00056 * error("Device not detected!\n"); 00057 * } 00058 * } 00059 * @endcode 00060 */ 00061 class Si1133 00062 { 00063 public: 00064 00065 /** Create an Si1133 object connected to the specified I2C pins with the specified I2C slave address 00066 * 00067 * @param sda The I2C data pin. 00068 * @param scl The I2C clock pin. 00069 * @param hz The I2C bus frequency (defaults to 400kHz). 00070 */ 00071 Si1133(PinName sda, PinName scl, int hz = 400000); 00072 00073 /** 00074 * Si1133 destructor 00075 */ 00076 ~Si1133(void); 00077 00078 /** Probe for the Si1133 and try to initialize the sensor 00079 * 00080 * @returns 00081 * 'true' if the device exists on the bus, 00082 * 'false' if the device doesn't exist on the bus. 00083 */ 00084 bool open(); 00085 00086 /** Measure the current light level (in lux) on the Si1133 00087 * 00088 * @returns The current temperature measurement in Lux. 00089 */ 00090 float get_light_level(); 00091 00092 /** Measure the current UV Index on the Si1133 00093 * 00094 * @returns The current UV index measurement. 00095 */ 00096 float get_uv_index(); 00097 00098 /** Do a combined measurement and return both the light level and UV index 00099 * 00100 * @param[out] light_level Measured light level in Lux 00101 * @param[out] uv_index Measured UV index 00102 * 00103 * @returns true if measurement was successful 00104 */ 00105 bool get_light_and_uv(float *light_level, float *uv_index); 00106 00107 #ifdef MBED_OPERATORS 00108 /** A shorthand for get_light_level() 00109 * 00110 * @returns The current temperature measurement in Lux. 00111 */ 00112 operator float(); 00113 #endif 00114 00115 private: 00116 /** 00117 * @name I2C Registers 00118 * @{ 00119 */ 00120 enum Register { 00121 REG_PART_ID = 0x00, /**< Part ID */ 00122 REG_HW_ID = 0x01, /**< Hardware ID */ 00123 REG_REV_ID = 0x02, /**< Hardware revision */ 00124 REG_HOSTIN0 = 0x0A, /**< Data for parameter table on PARAM_SET write to COMMAND register */ 00125 REG_COMMAND = 0x0B, /**< Initiated action in Sensor when specific codes written here */ 00126 REG_IRQ_ENABLE = 0x0F, /**< Interrupt enable */ 00127 REG_RESPONSE1 = 0x10, /**< Contains the readback value from a query or a set command */ 00128 REG_RESPONSE0 = 0x11, /**< Chip state and error status */ 00129 REG_IRQ_STATUS = 0x12, /**< Interrupt status */ 00130 REG_HOSTOUT0 = 0x13, /**< Captured Sensor Data */ 00131 REG_HOSTOUT1 = 0x14, /**< Captured Sensor Data */ 00132 REG_HOSTOUT2 = 0x15, /**< Captured Sensor Data */ 00133 REG_HOSTOUT3 = 0x16, /**< Captured Sensor Data */ 00134 REG_HOSTOUT4 = 0x17, /**< Captured Sensor Data */ 00135 REG_HOSTOUT5 = 0x18, /**< Captured Sensor Data */ 00136 REG_HOSTOUT6 = 0x19, /**< Captured Sensor Data */ 00137 REG_HOSTOUT7 = 0x1A, /**< Captured Sensor Data */ 00138 REG_HOSTOUT8 = 0x1B, /**< Captured Sensor Data */ 00139 REG_HOSTOUT9 = 0x1C, /**< Captured Sensor Data */ 00140 REG_HOSTOUT10 = 0x1D, /**< Captured Sensor Data */ 00141 REG_HOSTOUT11 = 0x1E, /**< Captured Sensor Data */ 00142 REG_HOSTOUT12 = 0x1F, /**< Captured Sensor Data */ 00143 REG_HOSTOUT13 = 0x20, /**< Captured Sensor Data */ 00144 REG_HOSTOUT14 = 0x21, /**< Captured Sensor Data */ 00145 REG_HOSTOUT15 = 0x22, /**< Captured Sensor Data */ 00146 REG_HOSTOUT16 = 0x23, /**< Captured Sensor Data */ 00147 REG_HOSTOUT17 = 0x24, /**< Captured Sensor Data */ 00148 REG_HOSTOUT18 = 0x25, /**< Captured Sensor Data */ 00149 REG_HOSTOUT19 = 0x26, /**< Captured Sensor Data */ 00150 REG_HOSTOUT20 = 0x27, /**< Captured Sensor Data */ 00151 REG_HOSTOUT21 = 0x28, /**< Captured Sensor Data */ 00152 REG_HOSTOUT22 = 0x29, /**< Captured Sensor Data */ 00153 REG_HOSTOUT23 = 0x2A, /**< Captured Sensor Data */ 00154 REG_HOSTOUT24 = 0x2B, /**< Captured Sensor Data */ 00155 REG_HOSTOUT25 = 0x2C, /**< Captured Sensor Data */ 00156 }; 00157 /**@}*/ 00158 00159 /** 00160 * @name Parameters 00161 * @{ 00162 */ 00163 enum Parameter { 00164 PARAM_I2C_ADDR = 0x00, /**< I2C address */ 00165 PARAM_CH_LIST = 0x01, /**< Channel list */ 00166 PARAM_ADCCONFIG0 = 0x02, /**< ADC config for Channel 0 */ 00167 PARAM_ADCSENS0 = 0x03, /**< ADC sensitivity setting for Channel 0 */ 00168 PARAM_ADCPOST0 = 0x04, /**< ADC resolution, shift and threshold settings for Channel 0 */ 00169 PARAM_MEASCONFIG0 = 0x05, /**< ADC measurement counter selection for Channel 0 */ 00170 PARAM_ADCCONFIG1 = 0x06, /**< ADC config for Channel 1 */ 00171 PARAM_ADCSENS1 = 0x07, /**< ADC sensitivity setting for Channel 1 */ 00172 PARAM_ADCPOST1 = 0x08, /**< ADC resolution, shift and threshold settings for Channel 1 */ 00173 PARAM_MEASCONFIG1 = 0x09, /**< ADC measurement counter selection for Channel 1 */ 00174 PARAM_ADCCONFIG2 = 0x0A, /**< ADC config for Channel 2 */ 00175 PARAM_ADCSENS2 = 0x0B, /**< ADC sensitivity setting for Channel 2 */ 00176 PARAM_ADCPOST2 = 0x0C, /**< ADC resolution, shift and threshold settings for Channel 2 */ 00177 PARAM_MEASCONFIG2 = 0x0D, /**< ADC measurement counter selection for Channel 2 */ 00178 PARAM_ADCCONFIG3 = 0x0E, /**< ADC config for Channel 3 */ 00179 PARAM_ADCSENS3 = 0x0F, /**< ADC sensitivity setting for Channel 3 */ 00180 PARAM_ADCPOST3 = 0x10, /**< ADC resolution, shift and threshold settings for Channel 3 */ 00181 PARAM_MEASCONFIG3 = 0x11, /**< ADC measurement counter selection for Channel 3 */ 00182 PARAM_ADCCONFIG4 = 0x12, /**< ADC config for Channel 4 */ 00183 PARAM_ADCSENS4 = 0x13, /**< ADC sensitivity setting for Channel 4 */ 00184 PARAM_ADCPOST4 = 0x14, /**< ADC resolution, shift and threshold settings for Channel 4 */ 00185 PARAM_MEASCONFIG4 = 0x15, /**< ADC measurement counter selection for Channel 4 */ 00186 PARAM_ADCCONFIG5 = 0x16, /**< ADC config for Channel 5 */ 00187 PARAM_ADCSENS5 = 0x17, /**< ADC sensitivity setting for Channel 5 */ 00188 PARAM_ADCPOST5 = 0x18, /**< ADC resolution, shift and threshold settings for Channel 5 */ 00189 PARAM_MEASCONFIG5 = 0x19, /**< ADC measurement counter selection for Channel 5 */ 00190 PARAM_MEASRATE_H = 0x1A, /**< Main measurement rate counter MSB */ 00191 PARAM_MEASRATE_L = 0x1B, /**< Main measurement rate counter LSB */ 00192 PARAM_MEASCOUNT0 = 0x1C, /**< Measurement rate extension counter 0 */ 00193 PARAM_MEASCOUNT1 = 0x1D, /**< Measurement rate extension counter 1 */ 00194 PARAM_MEASCOUNT2 = 0x1E, /**< Measurement rate extension counter 2 */ 00195 PARAM_THRESHOLD0_H = 0x25, /**< Threshold level 0 MSB */ 00196 PARAM_THRESHOLD0_L = 0x26, /**< Threshold level 0 LSB */ 00197 PARAM_THRESHOLD1_H = 0x27, /**< Threshold level 1 MSB */ 00198 PARAM_THRESHOLD1_L = 0x28, /**< Threshold level 1 LSB */ 00199 PARAM_THRESHOLD2_H = 0x29, /**< Threshold level 2 MSB */ 00200 PARAM_THRESHOLD2_L = 0x2A, /**< Threshold level 2 LSB */ 00201 PARAM_BURST = 0x2B, /**< Burst enable and burst count */ 00202 }; 00203 /**@}*/ 00204 00205 /** 00206 * @name Commands 00207 * @{ 00208 */ 00209 enum Command { 00210 CMD_RESET_CMD_CTR = 0x00, /**< Resets the command counter */ 00211 CMD_RESET = 0x01, /**< Forces a Reset */ 00212 CMD_NEW_ADDR = 0x02, /**< Stores the new I2C address */ 00213 CMD_FORCE_CH = 0x11, /**< Initiates a set of measurements specified in CHAN_LIST parameter */ 00214 CMD_PAUSE_CH = 0x12, /**< Pauses autonomous measurements */ 00215 CMD_START = 0x13, /**< Starts autonomous measurements */ 00216 CMD_PARAM_SET = 0x80, /**< Sets a parameter */ 00217 CMD_PARAM_QUERY = 0x40, /**< Reads a parameter */ 00218 }; 00219 /**@}*/ 00220 00221 /** 00222 * @name Responses 00223 * @{ 00224 */ 00225 enum Response { 00226 RSP0_CHIPSTAT_MASK = 0xE0, /**< Chip state mask in Response0 register */ 00227 RSP0_COUNTER_MASK = 0x1F, /**< Command counter and error indicator mask in Response0 register */ 00228 RSP0_SLEEP = 0x20, /**< Sleep state indicator bit mask in Response0 register */ 00229 }; 00230 /**@}*/ 00231 00232 /** 00233 * @brief 00234 * Structure to store the data measured by the Si1133 00235 */ 00236 typedef struct { 00237 uint8_t irq_status; /**< Interrupt status of the device */ 00238 int32_t ch0; /**< Channel 0 measurement data */ 00239 int32_t ch1; /**< Channel 1 measurement data */ 00240 int32_t ch2; /**< Channel 2 measurement data */ 00241 int32_t ch3; /**< Channel 3 measurement data */ 00242 } Samples_t; 00243 00244 /** 00245 * @brief 00246 * Structure to store the calculation coefficients 00247 */ 00248 typedef struct { 00249 int16_t info; /**< Info */ 00250 uint16_t mag; /**< Magnitude */ 00251 } Coeff_t; 00252 00253 /** 00254 * @brief 00255 * Structure to store the coefficients used for Lux calculation 00256 */ 00257 typedef struct { 00258 Coeff_t coeff_high[4]; /**< High amplitude coeffs */ 00259 Coeff_t coeff_low[9]; /**< Low amplitude coeffs */ 00260 } LuxCoeff_t; 00261 00262 /* Forward-declare constant coefficient table */ 00263 static const LuxCoeff_t lk; 00264 static const Coeff_t uk[]; 00265 00266 /* Private functions */ 00267 uint32_t read_register(enum Register reg, uint8_t *data); 00268 uint32_t write_register(enum Register reg, uint8_t data); 00269 uint32_t write_register_block(enum Register reg, uint8_t length, uint8_t *data); 00270 uint32_t read_register_block(enum Register reg, uint8_t length, uint8_t *data); 00271 uint32_t get_irq_status(uint8_t *irq_status); 00272 uint32_t wait_until_sleep(void); 00273 uint32_t reset(void); 00274 uint32_t reset_cmd_counter (void); 00275 uint32_t send_cmd(enum Command command); 00276 uint32_t force_measurement (void); 00277 uint32_t pause_measurement (void); 00278 uint32_t start_measurement (void); 00279 uint32_t set_parameter (enum Parameter address, uint8_t value); 00280 uint32_t read_parameter (enum Parameter address); 00281 uint32_t init (void); 00282 uint32_t deinit (void); 00283 uint32_t measure (Samples_t *samples); 00284 int32_t get_uv (int32_t uv); 00285 int32_t get_lux (int32_t vis_high, int32_t vis_low, int32_t ir); 00286 uint32_t measure_lux_uv (float *lux, float *uvi); 00287 uint32_t get_measurement (float *lux, float *uvi); 00288 uint32_t get_hardware_id (uint8_t *hardware_id); 00289 00290 int32_t calculate_polynomial_helper (int32_t input, int8_t fraction, uint16_t mag, int8_t shift); 00291 int32_t calculate_polynomial (int32_t x, int32_t y, uint8_t input_fraction, uint8_t output_fraction, uint8_t num_coeff, const Coeff_t *kp); 00292 00293 /* Member variables */ 00294 I2C m_I2C; 00295 }; 00296 00297 #endif
Generated on Sat Jul 16 2022 18:44:29 by
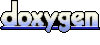