NSDL C library
Dependents: NSDL_HelloWorld_WiFi UbloxModemNanoServiceClient IOT-NSDL_HelloWorld LWM2M_NanoService_Ethernet ... more
Fork of nsdl_lib by
sn_coap_header.h
00001 /** 00002 * \file sn_coap_header.h 00003 * 00004 * \brief CoAP C-library User header interface header file 00005 * 00006 * Created on: Jun 30, 2011 00007 * Author: tero 00008 * 00009 * \note Supports draft-ietf-core-coap-18 00010 */ 00011 00012 #ifndef SN_COAP_HEADER_H_ 00013 #define SN_COAP_HEADER_H_ 00014 00015 #ifdef __cplusplus 00016 extern "C" { 00017 #endif 00018 00019 /* * * * * * * * * * * * * * */ 00020 /* * * * ENUMERATIONS * * * */ 00021 /* * * * * * * * * * * * * * */ 00022 00023 /** 00024 * \brief Enumeration for CoAP Version 00025 */ 00026 typedef enum coap_version_ 00027 { 00028 COAP_VERSION_1 = 0x40, 00029 COAP_VERSION_UNKNOWN = 0xFF 00030 } coap_version_e; 00031 00032 /** 00033 * \brief Enumeration for CoAP Message type, used in CoAP Header 00034 */ 00035 typedef enum sn_coap_msg_type_ 00036 { 00037 COAP_MSG_TYPE_CONFIRMABLE = 0x00, /**< Reliable Request messages */ 00038 COAP_MSG_TYPE_NON_CONFIRMABLE = 0x10, /**< Non-reliable Request and Response messages */ 00039 COAP_MSG_TYPE_ACKNOWLEDGEMENT = 0x20, /**< Response to a Confirmable Request */ 00040 COAP_MSG_TYPE_RESET = 0x30 /**< Answer a Bad Request */ 00041 } sn_coap_msg_type_e; 00042 00043 /** 00044 * \brief Enumeration for CoAP Message code, used in CoAP Header 00045 */ 00046 typedef enum sn_coap_msg_code_ 00047 { 00048 COAP_MSG_CODE_EMPTY = 0, 00049 COAP_MSG_CODE_REQUEST_GET = 1, 00050 COAP_MSG_CODE_REQUEST_POST = 2, 00051 COAP_MSG_CODE_REQUEST_PUT = 3, 00052 COAP_MSG_CODE_REQUEST_DELETE = 4, 00053 00054 COAP_MSG_CODE_RESPONSE_CREATED = 65, 00055 COAP_MSG_CODE_RESPONSE_DELETED = 66, 00056 COAP_MSG_CODE_RESPONSE_VALID = 67, 00057 COAP_MSG_CODE_RESPONSE_CHANGED = 68, 00058 COAP_MSG_CODE_RESPONSE_CONTENT = 69, 00059 COAP_MSG_CODE_RESPONSE_BAD_REQUEST = 128, 00060 COAP_MSG_CODE_RESPONSE_UNAUTHORIZED = 129, 00061 COAP_MSG_CODE_RESPONSE_BAD_OPTION = 130, 00062 COAP_MSG_CODE_RESPONSE_FORBIDDEN = 131, 00063 COAP_MSG_CODE_RESPONSE_NOT_FOUND = 132, 00064 COAP_MSG_CODE_RESPONSE_METHOD_NOT_ALLOWED = 133, 00065 COAP_MSG_CODE_RESPONSE_NOT_ACCEPTABLE = 134, 00066 COAP_MSG_CODE_RESPONSE_REQUEST_ENTITY_INCOMPLETE = 136, 00067 COAP_MSG_CODE_RESPONSE_PRECONDITION_FAILED = 140, 00068 COAP_MSG_CODE_RESPONSE_REQUEST_ENTITY_TOO_LARGE = 141, 00069 COAP_MSG_CODE_RESPONSE_UNSUPPORTED_CONTENT_FORMAT = 143, 00070 COAP_MSG_CODE_RESPONSE_INTERNAL_SERVER_ERROR = 160, 00071 COAP_MSG_CODE_RESPONSE_NOT_IMPLEMENTED = 161, 00072 COAP_MSG_CODE_RESPONSE_BAD_GATEWAY = 162, 00073 COAP_MSG_CODE_RESPONSE_SERVICE_UNAVAILABLE = 163, 00074 COAP_MSG_CODE_RESPONSE_GATEWAY_TIMEOUT = 164, 00075 COAP_MSG_CODE_RESPONSE_PROXYING_NOT_SUPPORTED = 165 00076 } sn_coap_msg_code_e; 00077 00078 /** 00079 * \brief Enumeration for CoAP Option number, used in CoAP Header 00080 */ 00081 typedef enum sn_coap_option_numbers_ 00082 { 00083 COAP_OPTION_IF_MATCH = 1, 00084 COAP_OPTION_URI_HOST = 3, 00085 COAP_OPTION_ETAG = 4, 00086 COAP_OPTION_IF_NONE_MATCH = 5, 00087 COAP_OPTION_OBSERVE = 6, 00088 COAP_OPTION_URI_PORT = 7, 00089 COAP_OPTION_LOCATION_PATH = 8, 00090 COAP_OPTION_URI_PATH = 11, 00091 COAP_OPTION_CONTENT_FORMAT = 12, 00092 COAP_OPTION_MAX_AGE = 14, 00093 COAP_OPTION_URI_QUERY = 15, 00094 COAP_OPTION_ACCEPT = 17, 00095 COAP_OPTION_LOCATION_QUERY = 20, 00096 COAP_OPTION_BLOCK2 = 23, 00097 COAP_OPTION_BLOCK1 = 27, 00098 COAP_OPTION_PROXY_URI = 35, 00099 COAP_OPTION_PROXY_SCHEME = 39, 00100 COAP_OPTION_SIZE1 = 60 00101 // 128 = (Reserved) 00102 // 132 = (Reserved) 00103 // 136 = (Reserved) 00104 } sn_coap_option_numbers_e; 00105 00106 /** 00107 * \brief Enumeration for CoAP Content Format codes 00108 */ 00109 typedef enum sn_coap_content_format_ 00110 { 00111 COAP_CT_NONE = -1, 00112 COAP_CT_TEXT_PLAIN = 0, 00113 COAP_CT_LINK_FORMAT = 40, 00114 COAP_CT_XML = 41, 00115 COAP_CT_OCTET_STREAM = 42, 00116 COAP_CT_EXI = 47, 00117 COAP_CT_JSON = 50, 00118 } sn_coap_content_format_e; 00119 00120 /** 00121 * \brief Enumeration for CoAP status, used in CoAP Header 00122 */ 00123 typedef enum sn_coap_status_ 00124 { 00125 COAP_STATUS_OK = 0, /**< Default value is OK */ 00126 COAP_STATUS_PARSER_ERROR_IN_HEADER = 1, /**< CoAP will send Reset message to invalid message sender */ 00127 COAP_STATUS_PARSER_DUPLICATED_MSG = 2, /**< CoAP will send Acknowledgement message to duplicated message sender */ 00128 COAP_STATUS_PARSER_BLOCKWISE_MSG_RECEIVING = 3, /**< User will get whole message after all message blocks received. 00129 User must release messages with this status. */ 00130 COAP_STATUS_PARSER_BLOCKWISE_ACK = 4, /**< Acknowledgement for sent Blockwise message received */ 00131 COAP_STATUS_PARSER_BLOCKWISE_MSG_REJECTED = 5, /**< Blockwise message received but not supported by compiling switch */ 00132 COAP_STATUS_PARSER_BLOCKWISE_MSG_RECEIVED = 6 /**< Blockwise message fully received and returned to app. 00133 User must take care of releasing whole payload of the blockwise messages */ 00134 } sn_coap_status_e; 00135 00136 00137 /* * * * * * * * * * * * * */ 00138 /* * * * STRUCTURES * * * */ 00139 /* * * * * * * * * * * * * */ 00140 00141 /** 00142 * \brief Structure for CoAP Options 00143 */ 00144 typedef struct sn_coap_options_list_ 00145 { 00146 00147 uint8_t max_age_len; /**< 0-4 bytes. */ 00148 uint8_t *max_age_ptr; /**< Must be set to NULL if not used */ 00149 00150 uint16_t proxy_uri_len; /**< 1-1034 bytes. */ 00151 uint8_t *proxy_uri_ptr; /**< Must be set to NULL if not used */ 00152 00153 uint8_t etag_len; /**< 1-8 bytes. Repeatable */ 00154 uint8_t *etag_ptr; /**< Must be set to NULL if not used */ 00155 00156 uint16_t uri_host_len; /**< 1-255 bytes. */ 00157 uint8_t *uri_host_ptr; /**< Must be set to NULL if not used */ 00158 00159 uint16_t location_path_len; /**< 0-255 bytes. Repeatable */ 00160 uint8_t *location_path_ptr; /**< Must be set to NULL if not used */ 00161 00162 uint8_t uri_port_len; /**< 0-2 bytes. */ 00163 uint8_t *uri_port_ptr; /**< Must be set to NULL if not used */ 00164 00165 uint16_t location_query_len; /**< 0-255 bytes. Repeatable */ 00166 uint8_t *location_query_ptr; /**< Must be set to NULL if not used */ 00167 00168 uint8_t observe; 00169 uint8_t observe_len; /**< 0-2 bytes. */ 00170 uint8_t *observe_ptr; /**< Must be set to NULL if not used */ 00171 00172 uint8_t accept_len; /**< 0-2 bytes. Repeatable */ 00173 uint8_t *accept_ptr; /**< Must be set to NULL if not used */ 00174 00175 uint16_t uri_query_len; /**< 1-255 bytes. Repeatable */ 00176 uint8_t *uri_query_ptr; /**< Must be set to NULL if not used */ 00177 00178 uint8_t block1_len; /**< 0-3 bytes. */ 00179 uint8_t *block1_ptr; /**< Not for User */ 00180 00181 uint8_t block2_len; /**< 0-3 bytes. */ 00182 uint8_t *block2_ptr; /**< Not for User */ 00183 } sn_coap_options_list_s; 00184 00185 00186 /* !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! */ 00187 /* !!! Main CoAP message struct !!! */ 00188 /* !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! */ 00189 00190 /** 00191 * \brief Main CoAP message struct 00192 */ 00193 typedef struct sn_coap_hdr_ 00194 { 00195 sn_coap_status_e coap_status; /**< Used for telling to User special cases when parsing message */ 00196 00197 /* * * * * * * * * * * */ 00198 /* * * * Header * * * */ 00199 /* * * * * * * * * * * */ 00200 00201 sn_coap_msg_type_e msg_type; /**< Confirmable, Non-Confirmable, Acknowledgement or Reset */ 00202 sn_coap_msg_code_e msg_code; /**< Empty: 0; Requests: 1-31; Responses: 64-191 */ 00203 uint16_t msg_id; /**< Message ID. Parser sets parsed message ID, builder sets message ID of built coap message */ 00204 00205 /* * * * * * * * * * * */ 00206 /* * * * Options * * * */ 00207 /* * * * * * * * * * * */ 00208 00209 /* Here are most often used Options */ 00210 00211 uint16_t uri_path_len; /**< 0-255 bytes. Repeatable. */ 00212 uint8_t *uri_path_ptr; /**< Must be set to NULL if not used. E.g: temp1/temp2 */ 00213 00214 uint8_t token_len; /**< 1-8 bytes. */ 00215 uint8_t *token_ptr; /**< Must be set to NULL if not used */ 00216 00217 uint8_t content_type_len; /**< 0-2 bytes. */ 00218 uint8_t *content_type_ptr; /**< Must be set to NULL if not used */ 00219 00220 /* Here are not so often used Options */ 00221 sn_coap_options_list_s *options_list_ptr; /**< Must be set to NULL if not used */ 00222 00223 /* * * * * * * * * * * */ 00224 /* * * * Payload * * * */ 00225 /* * * * * * * * * * * */ 00226 00227 uint16_t payload_len; /**< Must be set to zero if not used */ 00228 uint8_t *payload_ptr; /**< Must be set to NULL if not used */ 00229 } sn_coap_hdr_s; 00230 00231 /* * * * * * * * * * * * * * * * * * * * * * */ 00232 /* * * * EXTERNAL FUNCTION PROTOTYPES * * * */ 00233 /* * * * * * * * * * * * * * * * * * * * * * */ 00234 00235 /** 00236 * \brief Sets the memory allocation and deallocation functions the library will use, and must be called first. 00237 */ 00238 extern void sn_coap_builder_and_parser_init(void* (*used_malloc_func_ptr)(uint16_t), void (*used_free_func_ptr)(void*)); 00239 00240 /** 00241 * \brief Parses an incoming message buffer to a CoAP header structure. 00242 */ 00243 extern sn_coap_hdr_s *sn_coap_parser(uint16_t packet_data_len, uint8_t *packet_data_ptr, coap_version_e *coap_version_ptr); 00244 00245 /** 00246 * \brief Releases any memory allocated by a CoAP message structure. 00247 */ 00248 extern void sn_coap_parser_release_allocated_coap_msg_mem(sn_coap_hdr_s *freed_coap_msg_ptr); 00249 00250 /** 00251 * \brief Builds an outgoing message buffer from a CoAP header structure. 00252 */ 00253 extern int16_t sn_coap_builder(uint8_t *dst_packet_data_ptr, sn_coap_hdr_s *src_coap_msg_ptr); 00254 00255 /** 00256 * \brief Calculates the needed message buffer size from a CoAP message structure. 00257 */ 00258 extern uint16_t sn_coap_builder_calc_needed_packet_data_size(sn_coap_hdr_s *src_coap_msg_ptr); 00259 00260 /** 00261 * \brief Automates the building of a response to an incoming request. 00262 */ 00263 extern sn_coap_hdr_s *sn_coap_build_response(sn_coap_hdr_s *coap_packet_ptr, uint8_t msg_code); 00264 00265 /** 00266 * \brief CoAP packet debugging. 00267 */ 00268 extern void sn_coap_packet_debug(sn_coap_hdr_s *coap_packet_ptr); 00269 00270 #ifdef __cplusplus 00271 } 00272 #endif 00273 00274 #endif /* SN_COAP_HEADER_H_ */
Generated on Tue Jul 12 2022 18:09:19 by
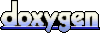