a library to use GPRS like ethernet or wifi, which makes it possible to connect to the internet with your GPRS module
Dependencies: BufferedSerial
Dependents: ThinkSpeak_Test roam_v1 roam_v2 finalv3
Fork of GPRSInterface by
GPRSInterface Class Reference
Interface using GPRS to connect to an IP-based network. More...
#include <GPRSInterface.h>
Inherits GPRS.
Public Member Functions | |
GPRSInterface (PinName tx, PinName rx, const char *apn, const char *userName=NULL, const char *passWord=NULL) | |
Constructor. | |
int | init () |
Initialize the interface(no connection at this point). | |
int | connect () |
Connect to the network and get IP address. | |
int | disconnect () |
Disconnect with the network. | |
char * | getIPAddress () |
Get IP address. | |
bool | join (void) |
Connect the GPRS module to the network. | |
bool | close (int socket) |
Close a tcp connection. | |
bool | connect (int socket, Protocol ptl, const char *host, int port, int timeout=DEFAULT_TIMEOUT_MS) |
Open a tcp/udp connection with the specified host on the specified port. | |
bool | setProtocol (int socket, Protocol p) |
Set the protocol (UDP or TCP) | |
void | reset () |
Reset the GPRS module. | |
int | wait_readable (int socket, int wait_time) |
wait a few time to check if GPRS module is readable or not | |
int | wait_writeable (int socket, int req_size) |
wait a few time to check if GPRS module is writeable or not | |
bool | is_connected (int socket) |
Check if a tcp link is active. | |
int | sock_send (int socket, const char *str, int len) |
send data to socket | |
int | sock_recv (int socket, char *buf, int len) |
read data from socket | |
bool | gethostbyname (const char *host, uint32_t *ip) |
convert the host to ip | |
Static Public Member Functions | |
static GPRS * | getInstance () |
get instance of GPRS class | |
Protected Member Functions | |
int | readline (char *buf, int len, uint32_t timeout=DEFAULT_TIMEOUT_MS) |
Read a line. |
Detailed Description
Interface using GPRS to connect to an IP-based network.
Definition at line 32 of file GPRSInterface.h.
Constructor & Destructor Documentation
GPRSInterface | ( | PinName | tx, |
PinName | rx, | ||
const char * | apn, | ||
const char * | userName = NULL , |
||
const char * | passWord = NULL |
||
) |
Constructor.
- Parameters:
-
tx mbed pin to use for tx line of Serial interface rx mbed pin to use for rx line of Serial interface baudRate serial communicate baud rate apn name of the gateway for GPRS to connect to the network userName apn's username, usually is NULL passWord apn's password, usually is NULL
Definition at line 25 of file GPRSInterface.cpp.
Member Function Documentation
bool close | ( | int | socket ) | [inherited] |
bool connect | ( | int | socket, |
Protocol | ptl, | ||
const char * | host, | ||
int | port, | ||
int | timeout = DEFAULT_TIMEOUT_MS |
||
) | [inherited] |
Open a tcp/udp connection with the specified host on the specified port.
- Parameters:
-
socket an endpoint of an inter-process communication flow of GPRS module,for SIM900 module, it is in [0,6] ptl protocol for socket, TCP/UDP can be choosen host host (can be either an ip address or a name. If a name is provided, a dns request will be established) port port timeout wait time (ms)
- Returns:
- true if successful
int connect | ( | ) |
Connect to the network and get IP address.
- Returns:
- 0 on success, a negative number on failure
Definition at line 35 of file GPRSInterface.cpp.
int disconnect | ( | void | ) |
Disconnect with the network.
- Returns:
- 0 on success, a negative number on failure
Definition at line 40 of file GPRSInterface.cpp.
bool gethostbyname | ( | const char * | host, |
uint32_t * | ip | ||
) | [inherited] |
static GPRS* getInstance | ( | ) | [static, inherited] |
char * getIPAddress | ( | ) |
int init | ( | ) |
Initialize the interface(no connection at this point).
- Returns:
- 0 on success, a negative number on failure
Definition at line 30 of file GPRSInterface.cpp.
bool is_connected | ( | int | socket ) | [inherited] |
bool join | ( | void | ) | [inherited] |
int readline | ( | char * | buf, |
int | len, | ||
uint32_t | timeout = DEFAULT_TIMEOUT_MS |
||
) | [protected, inherited] |
bool setProtocol | ( | int | socket, |
Protocol | p | ||
) | [inherited] |
int sock_recv | ( | int | socket, |
char * | buf, | ||
int | len | ||
) | [inherited] |
int sock_send | ( | int | socket, |
const char * | str, | ||
int | len | ||
) | [inherited] |
int wait_readable | ( | int | socket, |
int | wait_time | ||
) | [inherited] |
wait a few time to check if GPRS module is readable or not
- Parameters:
-
socket socket wait_time time of waiting
int wait_writeable | ( | int | socket, |
int | req_size | ||
) | [inherited] |
wait a few time to check if GPRS module is writeable or not
- Parameters:
-
socket socket wait_time time of waiting
Generated on Tue Jul 12 2022 16:57:21 by
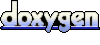