a library to use GPRS like ethernet or wifi, which makes it possible to connect to the internet with your GPRS module
Dependencies: BufferedSerial
Dependents: ThinkSpeak_Test roam_v1 roam_v2 finalv3
Fork of GPRSInterface by
modem.cpp
00001 /* 00002 modem.cpp 00003 2015 Copyright (c) Seeed Technology Limited. All right reserved. 00004 */ 00005 00006 #include "modem.h" 00007 #include <stdarg.h> 00008 00009 #define DEBUG 00010 00011 #ifdef DEBUG 00012 #include "USBSerial.h" 00013 extern USBSerial pc; 00014 #define LOG(args...) pc.printf(args) 00015 #else 00016 #define LOG(args...) 00017 #endif 00018 00019 int Modem::readline(char *buf, int len, uint32_t timeout) 00020 { 00021 int bytes = 0; 00022 uint32_t start = us_ticker_read(); 00023 timeout = timeout * 1000; // ms to us 00024 00025 while (bytes < len) { 00026 if (readable()) { 00027 char ch = getc(); 00028 if (ch == '\n') { 00029 if (bytes > 0 && buf[bytes - 1] == '\r') { 00030 bytes--; 00031 } 00032 00033 if (bytes > 0) { 00034 buf[bytes] = '\0'; 00035 00036 return bytes; 00037 } 00038 } else { 00039 buf[bytes] = ch; 00040 bytes++; 00041 } 00042 } else { 00043 if ((uint32_t)(us_ticker_read() - start) > timeout) { 00044 LOG("wait for a line - timeout\r\n"); 00045 return -1; 00046 } 00047 } 00048 } 00049 00050 // buffer is not enough 00051 LOG("%s %d line buffer is not enough (max_buf_size: %d)!\r\n", __FILE__, __LINE__, len); 00052 00053 return 0; 00054 } 00055 00056 int Modem::command(const char* format, ...) 00057 { 00058 00059 char buffer[64]; 00060 memset(buffer, 0, sizeof(buffer)); 00061 int r = 0; 00062 00063 va_list arg; 00064 va_start(arg, format); 00065 r = vsprintf(buffer, format, arg); 00066 // this may not hit the heap but should alert the user anyways 00067 if(r > sizeof(buffer)) { 00068 error("%s %d buffer overwrite (max_buf_size: %d exceeded: %d)!\r\n", __FILE__, __LINE__, sizeof(buffer), r); 00069 va_end(arg); 00070 return 0; 00071 } 00072 va_end(arg); 00073 00074 00075 while (!writeable()) { 00076 } 00077 flush(); 00078 00079 r = BufferedSerial::write(buffer, r); // send command 00080 00081 char response[64] = {0,}; 00082 readline(response, sizeof(response)); // echo enabled 00083 00084 readline(response, sizeof(response)); 00085 00086 if (strcmp(response, "OK") == 0) { 00087 return 0; 00088 } 00089 00090 LOG("cmd failed - w(%s), r(%s)\r\n", buffer, response); 00091 00092 return -1; 00093 } 00094 00095 int Modem::match(const char *str, uint32_t timeout) 00096 { 00097 const char *ptr = str; 00098 uint32_t start = us_ticker_read(); 00099 timeout = timeout * 1000; // ms to us 00100 00101 while(*ptr) { 00102 if(readable()) { 00103 char c = getc(); 00104 if (*ptr == c) { 00105 ptr++; 00106 } else { 00107 ptr = str; 00108 } 00109 } else { 00110 if ((uint32_t)(us_ticker_read() - start) > timeout) { 00111 LOG("wait for [%s] - timeout\r\n", str); 00112 return -1; 00113 } 00114 } 00115 } 00116 00117 return 0; 00118 } 00119 00120 void Modem::flush() 00121 { 00122 while (readable()) { // flush 00123 getc(); 00124 } 00125 } 00126 00127 uint8_t Modem::read() 00128 { 00129 while (!readable()) { 00130 } 00131 00132 return getc(); 00133 } 00134
Generated on Tue Jul 12 2022 16:57:21 by
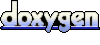