
A simple mbed OS application providing an example of asynchronous access to the X-NUCLEO_NFC01A1 Dynamic NFC Tag board.
Dependencies: NDefLib X_NUCLEO_NFC01A1
Fork of mbed-os-example-NFC01A1 by
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @date 22/01/2016 00005 * @brief Test the async comunication api 00006 ****************************************************************************** 00007 * 00008 * COPYRIGHT(c) 2015 STMicroelectronics 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 1. Redistributions of source code must retain the above copyright notice, 00013 * this list of conditions and the following disclaimer. 00014 * 2. Redistributions in binary form must reproduce the above copyright notice, 00015 * this list of conditions and the following disclaimer in the documentation 00016 * and/or other materials provided with the distribution. 00017 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00018 * may be used to endorse or promote products derived from this software 00019 * without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00022 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00023 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00025 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00026 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00027 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00028 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00030 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 * 00032 ****************************************************************************** 00033 */ 00034 00035 #include "mbed.h" 00036 #include "XNucleoNFC01A1.h" 00037 #include "ReadUriCallbacks.h" 00038 #include "WriteUriCallbacks.h" 00039 #include "NDefNfcTagM24SR.h" 00040 #include "NDefLib/RecordType/RecordURI.h" 00041 00042 Thread thread; 00043 00044 /** flag to signal an interrupt from the nfc component*/ 00045 #define NFC_INTERRUPT_FLAG 0x1 00046 00047 /** flag to signal user button pressure*/ 00048 #define BUTTON_PRESSED_FLAG 0x2 00049 00050 00051 NDefLib::NDefNfcTag *tag; 00052 00053 XNucleoNFC01A1 *nfcNucleo; 00054 00055 /** Nfc ISR called when the nfc component has a message ready*/ 00056 static void nfc_interrupt_callback() { 00057 thread.signal_set(NFC_INTERRUPT_FLAG); 00058 }//nfcInterruptCallback 00059 00060 /** ISR handling button pressure **/ 00061 static void set_button_press() { 00062 thread.signal_set(BUTTON_PRESSED_FLAG); 00063 }//buttonPressed event 00064 00065 00066 void handleEvents() { 00067 //create the callback to read a tag 00068 ReadUriCallbacks NDefReadCallback(nfcNucleo->get_led1(),nfcNucleo->get_led2(),nfcNucleo->get_led3()); 00069 while (true) { 00070 osEvent event = thread.signal_wait(0); // wait for any event 00071 if (event.value.v == NFC_INTERRUPT_FLAG) { 00072 nfcNucleo->get_M24SR().manage_event(); 00073 } else if (event.value.v == BUTTON_PRESSED_FLAG) { 00074 printf("Button pressed! Reading the Tag.\n\r"); 00075 tag->set_callback(&NDefReadCallback); 00076 tag->open_session(); 00077 } 00078 } 00079 } 00080 00081 int main() { 00082 00083 #if defined(TARGET_STM) 00084 InterruptIn userButton(USER_BUTTON); 00085 userButton.fall(set_button_press); 00086 #endif 00087 00088 //create the nfc component 00089 I2C i2cChannel(XNucleoNFC01A1::DEFAULT_SDA_PIN,XNucleoNFC01A1::DEFAULT_SDL_PIN); 00090 00091 nfcNucleo = XNucleoNFC01A1::instance(i2cChannel,&nfc_interrupt_callback, 00092 XNucleoNFC01A1::DEFAULT_GPO_PIN,XNucleoNFC01A1::DEFAULT_RF_DISABLE_PIN, 00093 XNucleoNFC01A1::DEFAULT_LED1_PIN,XNucleoNFC01A1::DEFAULT_LED2_PIN, 00094 XNucleoNFC01A1::DEFAULT_LED3_PIN); 00095 00096 //No call back needed since default behavior is sync 00097 nfcNucleo->get_M24SR().get_session(); 00098 nfcNucleo->get_M24SR().manage_I2C_GPO(M24SR::I2C_ANSWER_READY); //switch to async mode 00099 00100 tag = &(nfcNucleo->get_M24SR().get_NDef_tag()); 00101 printf("System Init done!\n\r"); 00102 00103 //Start the event handling thread 00104 thread.start(handleEvents); 00105 00106 //create the callback to write a tag 00107 WriteUriCallbacks NDefWriteCallback(nfcNucleo->get_led1(),nfcNucleo->get_led2(),nfcNucleo->get_led3()); 00108 // write the tag 00109 tag->set_callback(&NDefWriteCallback); //set the callback 00110 printf("Writing Tag\n\r"); 00111 tag->open_session(); 00112 00113 while (true) { 00114 __WFE(); 00115 } 00116 00117 }
Generated on Sun Jul 17 2022 19:57:24 by
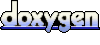