Expansion SW library to control a bipolar stepper motor using X-NUCLEO-IHM05A1 expansion board based on L6208.
Dependencies: ST_INTERFACES
Dependents: HelloWorld_IHM05A1 TAU_ROTATING_PLATFORM_IHM05A1 Amaldi_13_Exercise_IHM05A1 Amaldi_13_Exercise_IHM05A1motore ... more
Fork of X-NUCLEO-IHM05A1 by
L6208_def.h
00001 /******************************************************//** 00002 * @file L6208_def.h 00003 * @author IPC Rennes 00004 * @version V1.1.0 00005 * @date February 11th, 2016 00006 * @brief Header for l6208.c module 00007 * @note (C) COPYRIGHT 2016 STMicroelectronics 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef _L6208_H_INCLUDED 00040 #define _L6208_H_INCLUDED 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "L6208_config.h" 00048 #include "motor_def.h" 00049 00050 /* Definitions ---------------------------------------------------------------*/ 00051 00052 /** @addtogroup Components 00053 * @{ 00054 */ 00055 00056 /** @defgroup L6208 00057 * @{ 00058 */ 00059 00060 /** @defgroup L6208_Exported_Defines L6208 Exported Defines 00061 * @{ 00062 */ 00063 /// Current FW major version 00064 #define L6208_FW_MAJOR_VERSION (uint8_t)(1) 00065 /// Current FW minor version 00066 #define L6208_FW_MINOR_VERSION (uint8_t)(1) 00067 /// Current FW patch version 00068 #define L6208_FW_PATCH_VERSION (uint8_t)(0) 00069 /// Current FW version 00070 #define L6208_FW_VERSION (uint32_t)((L6208_FW_MAJOR_VERSION<<16)|\ 00071 (L6208_FW_MINOR_VERSION<<8)|\ 00072 (L6208_FW_PATCH_VERSION)) 00073 00074 /// Exponent used to scale the sine function for the RefMicroTable 00075 #define L6208_SINE_WAVEFORM_POWER_OF_TWO_MAX_VALUE (15) 00076 00077 /// Tick frequency (Hz) 00078 #define TIMER_TICK_FREQUENCY (10000) 00079 00080 /// MCU wait time after power bridges are enabled 00081 #define BRIDGE_TURN_ON_DELAY (10) 00082 00083 /// The maximum number of devices 00084 #define MAX_NUMBER_OF_DEVICES (1) 00085 00086 /// Max position 00087 #define L6208_MAX_POSITION (0x7FFFFFFF) 00088 00089 /// Min position 00090 #define L6208_MIN_POSITION (0x80000000) 00091 00092 /// Position range 00093 #define L6208_POSITION_RANGE ((uint32_t)(L6208_MAX_POSITION -\ 00094 L6208_MIN_POSITION)) 00095 /// micro step samples per period/4 00096 #define L6208_USTEPS_PER_QUARTER_PERIOD (16) 00097 00098 /// minimum speed 00099 #define L6208_MIN_SPEED (16) 00100 00101 /// minimum acceleration and deceleration rate 00102 #define L6208_MIN_ACC_DEC_RATE (24) 00103 00104 /// Mask for HiZ bit in motorDecayMode_t enum 00105 #define L6208_FAST_DECAY_MODE_MASK (0x1) 00106 00107 /// L6208 error base number 00108 #define L6208_ERROR_BASE (0x9000) 00109 /** 00110 * @} 00111 */ 00112 00113 /* Types ---------------------------------------------------------------------*/ 00114 00115 /** @defgroup L6208_Exported_Types L6208 Exported Types 00116 * @{ 00117 */ 00118 00119 /** @defgroup Error_Types Error Types 00120 * @{ 00121 */ 00122 /// Errors 00123 typedef enum { 00124 L6208_ERROR_SET_HOME = L6208_ERROR_BASE, /// Error while setting home position 00125 L6208_ERROR_SET_MAX_SPEED = L6208_ERROR_BASE + 1, /// Error while setting max speed 00126 L6208_ERROR_SET_MIN_SPEED = L6208_ERROR_BASE + 2, /// Error while setting min speed 00127 L6208_ERROR_SET_ACCELERATION = L6208_ERROR_BASE + 3, /// Error while setting acceleration 00128 L6208_ERROR_SET_DECELERATION = L6208_ERROR_BASE + 4, /// Error while setting decelaration 00129 L6208_ERROR_MCU_OSC_CONFIG = L6208_ERROR_BASE + 5, /// Error while configuring mcu oscillator 00130 L6208_ERROR_MCU_CLOCK_CONFIG = L6208_ERROR_BASE + 6, /// Error while configuring mcu clock 00131 L6208_ERROR_POSITION = L6208_ERROR_BASE + 7, /// Unexpected current position (wrong number of steps) 00132 L6208_ERROR_SPEED = L6208_ERROR_BASE + 8, /// Unexpected current speed 00133 L6208_ERROR_INIT = L6208_ERROR_BASE + 9, /// Unexpected number of devices 00134 L6208_ERROR_SET_DIRECTION = L6208_ERROR_BASE + 10,/// Error while setting direction 00135 L6208_ERROR_SET_STEP_MODE = L6208_ERROR_BASE + 11,/// Attempt to set an unsupported step mode 00136 L6208_ERROR_SET_PWM = L6208_ERROR_BASE + 12,/// Error while setting a PWM parameter 00137 }errorTypes_t; 00138 /** 00139 * @} 00140 */ 00141 00142 /** @defgroup Device_Parameters Device Parameters 00143 * @{ 00144 */ 00145 /// Device Parameters Structure Type 00146 typedef struct 00147 { 00148 /// dwelling waiting time counter (tick) 00149 volatile uint32_t dwellCounter; 00150 /// motor position indicator (tick) 00151 uint32_t ticks; 00152 /// LSByte copy of the previous position (tick) 00153 uint8_t lsbOldTicks; 00154 /// LSByte copy of the previous position (tick) ( micro stepping ) 00155 uint8_t lsbOldUSteppingTicks; 00156 /// LSByte copy of the current position (tick) 00157 uint8_t lsbTicks; 00158 /// P1 = acceleration phase steps number (motor position control mode) 00159 uint32_t positionTarget1; 00160 /// P2 = constant speed steps number (motor position control mode) 00161 uint32_t positionTarget2; 00162 /// P3 = deceleration phase steps number (motor position control mode) 00163 uint32_t positionTarget3; 00164 /// P = total move distance in steps (motor position control mode) 00165 uint32_t positionTarget; 00166 /// absolute motor position in microsteps (motor position control mode) 00167 volatile int32_t absolutePos; 00168 /// mark position in microsteps (motor position control mode) 00169 volatile int32_t markPos; 00170 /// motor position in microsteps (motor position control mode) 00171 volatile uint32_t step; 00172 /// dwelling time after position got (ms) 00173 volatile uint16_t moveDwellTime; 00174 /// number of micro stepping waveform samples to be rescaled according to selected torque value 00175 volatile uint8_t uStepsample2scale; 00176 /// number of micro stepping waveform samples to be updated into the waveform scanning table 00177 volatile uint8_t uStepsample2update; 00178 /// microstepping waveform sample index 00179 volatile uint8_t uStepSample; 00180 /// system status flags 00181 volatile uint32_t flags; 00182 /// current stepper state machine index 00183 volatile motorState_t motionState; 00184 /// current step mode 00185 volatile motorStepMode_t stepMode; 00186 /// micro stepping waveform scanning sample index increment 00187 uint8_t uStepInc; 00188 /// frequency of the VREFA and VREFB PWM 00189 uint32_t vrefPwmFreq; 00190 /// period of the VREFA and VREFB PWM in 1/256th of a microsecond 00191 uint16_t vrefPwmPeriod; 00192 /// pulse width target of the VREFA PWM in 1/256th of a microsecond 00193 volatile uint16_t vrefPwmPulseWidthTargetA; 00194 /// pulse width target of the VREFB PWM in 1/256th of a microsecond 00195 volatile uint16_t vrefPwmPulseWidthTargetB; 00196 /// pulse width to be generated for VREFA PWM in 1/256th of a microsecond 00197 volatile int16_t vrefPwmPulseWidthToBeGeneratedA; 00198 /// pulse width to be generated for VREFB PWM in 1/256th of a microsecond 00199 volatile int16_t vrefPwmPulseWidthToBeGeneratedB; 00200 /// current selected torque value 00201 volatile uint16_t curTorqueScaler; 00202 /// selected VREFA value (%) 00203 volatile uint16_t vRefAVal; 00204 /// selected VREFB value (%) 00205 volatile uint16_t vRefBVal; 00206 /// constant speed phase torque value (%) 00207 volatile uint8_t runTorque; 00208 /// acceleration phase torque value (%) 00209 volatile uint8_t accelTorque; 00210 /// deceleration phase torque value (%) 00211 volatile uint8_t decelTorque; 00212 /// holding phase torque value (%) 00213 volatile uint8_t holdTorque; 00214 /// acceleration (steps/s^2) 00215 volatile uint16_t accelerationSps2; 00216 /// deceleration (steps/s^2) 00217 volatile uint16_t decelerationSps2; 00218 /// acceleration (steps/tick^2) 00219 volatile uint16_t accelerationSpt2; 00220 /// deceleration (steps/tick^2) 00221 volatile uint16_t decelerationSpt2; 00222 /// maximum speed (steps/s) 00223 volatile uint16_t maxSpeedSps; 00224 /// minimum speed (steps/s) 00225 volatile uint16_t minSpeedSps; 00226 /// current speed (steps/s) 00227 volatile uint16_t speedSps; 00228 /// maximum speed (steps/tick) 00229 volatile uint32_t maxSpeedSpt; 00230 /// minimum speed (steps/tick) 00231 volatile uint32_t minSpeedSpt; 00232 /// current speed (steps/tick) 00233 volatile uint32_t speedSpt; 00234 }deviceParams_t; 00235 /** 00236 * @} 00237 */ 00238 00239 /// Motor driver initialization structure definition 00240 typedef struct 00241 { 00242 /// acceleration (steps/s^2) 00243 uint16_t accelerationSps2; 00244 /// acceleration phase torque value (%) 00245 uint8_t accelTorque; 00246 /// deceleration (steps/s^2) 00247 uint16_t decelerationSps2; 00248 /// deceleration phase torque value (%) 00249 uint8_t decelTorque; 00250 /// maximum speed (steps/s) 00251 uint16_t maxSpeedSps; 00252 /// constant speed phase torque value (%) 00253 uint8_t runTorque; 00254 /// holding phase torque value (%) 00255 uint8_t holdTorque; 00256 /// current step mode 00257 motorStepMode_t stepMode; 00258 /// current decay mode (SLOW_DECAY or FAST_DECAY) 00259 motorDecayMode_t decayMode; 00260 /// dwelling time after position got (ms) 00261 uint16_t moveDwellTime; 00262 /// automatic HiZ on stop 00263 bool autoHiZstop; 00264 /// frequency of the VREFA and VREFB PWM 00265 uint32_t vrefPwmFreq; 00266 } l6208_init_t; 00267 /** 00268 * @} 00269 */ 00270 00271 /* Functions --------------------------------------------------------*/ 00272 00273 /** @defgroup MotorControl_Board_Linked_Functions MotorControl Board Linked Functions 00274 * @{ 00275 */ 00276 ///Delay of the requested number of milliseconds 00277 extern void L6208_Board_Delay(uint32_t delay); 00278 ///Enable Irq 00279 extern void L6208_Board_EnableIrq(void); 00280 ///Disable Irq 00281 extern void L6208_Board_DisableIrq(void); 00282 //Initialize the VREFA or VREFB PWM 00283 extern bool L6208_Board_VrefPwmInit(uint8_t bridgeId, uint32_t pwmFreq); 00284 ///Initialize the tick 00285 extern void L6208_Board_TickInit(void); 00286 ///Release the reset pin 00287 extern void L6208_Board_Releasereset(void); 00288 ///Set the reset pin 00289 extern void L6208_Board_reset(void); 00290 ///Set the control pin 00291 extern void L6208_Board_CONTROL_PIN_Set(void); 00292 ///Reset the control pin 00293 extern void L6208_Board_CONTROL_PIN_reset(void); 00294 ///Set the clock pin 00295 extern void L6208_Board_CLOCK_PIN_Set(void); 00296 ///Reset the clock pin 00297 extern void L6208_Board_CLOCK_PIN_reset(void); 00298 ///Set the half full pin 00299 extern void L6208_Board_HALF_FULL_PIN_Set(void); 00300 ///Reset the half full pin 00301 extern void L6208_Board_HALF_FULL_PIN_reset(void); 00302 ///Set the dir pin 00303 extern void L6208_Board_DIR_PIN_Set(void); 00304 ///Reset the dir pin 00305 extern void L6208_Board_DIR_PIN_reset(void); 00306 ///Enable the power bridges (leave the output bridges HiZ) 00307 extern void L6208_Board_enable(void); 00308 ///Disable the power bridges (leave the output bridges HiZ) 00309 extern void L6208_Board_disable(void); 00310 /** 00311 * @} 00312 */ 00313 00314 /** 00315 * @} 00316 */ 00317 00318 /** 00319 * @} 00320 */ 00321 00322 #ifdef __cplusplus 00323 } 00324 #endif 00325 00326 #endif /* __L6208_H */ 00327 00328 /******************* (C) COPYRIGHT 2016 STMicroelectronics *****END OF FILE****/
Generated on Thu Jul 14 2022 21:58:52 by
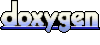