Capacitive digital sensor for relative humidity and temperature.
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: HelloWorld_ST_Sensors MOTENV_Mbed mbed-os-mqtt-client HTS221_JS ... more
HTS221_driver.h
00001 /** 00002 ****************************************************************************** 00003 * @file HTS221_driver.h 00004 * @author HESA Application Team 00005 * @version V1.1 00006 * @date 10-August-2016 00007 * @brief HTS221 driver header file 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __HTS221_DRIVER__H 00040 #define __HTS221_DRIVER__H 00041 00042 #include <stdint.h> 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* Uncomment the line below to expanse the "assert_param" macro in the drivers code */ 00049 #define USE_FULL_ASSERT_HTS221 00050 00051 /* Exported macro ------------------------------------------------------------*/ 00052 #ifdef USE_FULL_ASSERT_HTS221 00053 00054 /** 00055 * @brief The assert_param macro is used for function's parameters check. 00056 * @param expr: If expr is false, it calls assert_failed function which reports 00057 * the name of the source file and the source line number of the call 00058 * that failed. If expr is true, it returns no value. 00059 * @retval None 00060 */ 00061 #define HTS221_assert_param(expr) ((expr) ? (void)0 : HTS221_assert_failed((uint8_t *)__FILE__, __LINE__)) 00062 /* Exported functions ------------------------------------------------------- */ 00063 void HTS221_assert_failed(uint8_t *file, uint32_t line); 00064 #else 00065 #define HTS221_assert_param(expr) ((void)0) 00066 #endif /* USE_FULL_ASSERT_HTS221 */ 00067 00068 /** @addtogroup Environmental_Sensor 00069 * @{ 00070 */ 00071 00072 /** @addtogroup HTS221_DRIVER 00073 * @{ 00074 */ 00075 00076 /* Exported Types -------------------------------------------------------------*/ 00077 /** @defgroup HTS221_Exported_Types 00078 * @{ 00079 */ 00080 00081 00082 /** 00083 * @brief Error code type. 00084 */ 00085 typedef enum {HTS221_OK = (uint8_t)0, HTS221_ERROR = !HTS221_OK} HTS221_Error_et; 00086 00087 /** 00088 * @brief State type. 00089 */ 00090 typedef enum {HTS221_DISABLE = (uint8_t)0, HTS221_ENABLE = !HTS221_DISABLE} HTS221_State_et; 00091 #define IS_HTS221_State(MODE) ((MODE == HTS221_ENABLE) || (MODE == HTS221_DISABLE)) 00092 00093 /** 00094 * @brief Bit status type. 00095 */ 00096 typedef enum {HTS221_RESET = (uint8_t)0, HTS221_SET = !HTS221_RESET} HTS221_BitStatus_et; 00097 #define IS_HTS221_BitStatus(MODE) ((MODE == HTS221_RESET) || (MODE == HTS221_SET)) 00098 00099 /** 00100 * @brief Humidity average. 00101 */ 00102 typedef enum { 00103 HTS221_AVGH_4 = (uint8_t)0x00, /*!< Internal average on 4 samples */ 00104 HTS221_AVGH_8 = (uint8_t)0x01, /*!< Internal average on 8 samples */ 00105 HTS221_AVGH_16 = (uint8_t)0x02, /*!< Internal average on 16 samples */ 00106 HTS221_AVGH_32 = (uint8_t)0x03, /*!< Internal average on 32 samples */ 00107 HTS221_AVGH_64 = (uint8_t)0x04, /*!< Internal average on 64 samples */ 00108 HTS221_AVGH_128 = (uint8_t)0x05, /*!< Internal average on 128 samples */ 00109 HTS221_AVGH_256 = (uint8_t)0x06, /*!< Internal average on 256 samples */ 00110 HTS221_AVGH_512 = (uint8_t)0x07 /*!< Internal average on 512 samples */ 00111 } HTS221_Avgh_et; 00112 #define IS_HTS221_AVGH(AVGH) ((AVGH == HTS221_AVGH_4) || (AVGH == HTS221_AVGH_8) || \ 00113 (AVGH == HTS221_AVGH_16) || (AVGH == HTS221_AVGH_32) || \ 00114 (AVGH == HTS221_AVGH_64) || (AVGH == HTS221_AVGH_128) || \ 00115 (AVGH == HTS221_AVGH_256) || (AVGH == HTS221_AVGH_512)) 00116 00117 /** 00118 * @brief Temperature average. 00119 */ 00120 typedef enum { 00121 HTS221_AVGT_2 = (uint8_t)0x00, /*!< Internal average on 2 samples */ 00122 HTS221_AVGT_4 = (uint8_t)0x08, /*!< Internal average on 4 samples */ 00123 HTS221_AVGT_8 = (uint8_t)0x10, /*!< Internal average on 8 samples */ 00124 HTS221_AVGT_16 = (uint8_t)0x18, /*!< Internal average on 16 samples */ 00125 HTS221_AVGT_32 = (uint8_t)0x20, /*!< Internal average on 32 samples */ 00126 HTS221_AVGT_64 = (uint8_t)0x28, /*!< Internal average on 64 samples */ 00127 HTS221_AVGT_128 = (uint8_t)0x30, /*!< Internal average on 128 samples */ 00128 HTS221_AVGT_256 = (uint8_t)0x38 /*!< Internal average on 256 samples */ 00129 } HTS221_Avgt_et; 00130 #define IS_HTS221_AVGT(AVGT) ((AVGT == HTS221_AVGT_2) || (AVGT == HTS221_AVGT_4) || \ 00131 (AVGT == HTS221_AVGT_8) || (AVGT == HTS221_AVGT_16) || \ 00132 (AVGT == HTS221_AVGT_32) || (AVGT == HTS221_AVGT_64) || \ 00133 (AVGT == HTS221_AVGT_128) || (AVGT == HTS221_AVGT_256)) 00134 00135 /** 00136 * @brief Output data rate configuration. 00137 */ 00138 typedef enum { 00139 HTS221_ODR_ONE_SHOT = (uint8_t)0x00, /*!< Output Data Rate: one shot */ 00140 HTS221_ODR_1HZ = (uint8_t)0x01, /*!< Output Data Rate: 1Hz */ 00141 HTS221_ODR_7HZ = (uint8_t)0x02, /*!< Output Data Rate: 7Hz */ 00142 HTS221_ODR_12_5HZ = (uint8_t)0x03, /*!< Output Data Rate: 12.5Hz */ 00143 } HTS221_Odr_et; 00144 #define IS_HTS221_ODR(ODR) ((ODR == HTS221_ODR_ONE_SHOT) || (ODR == HTS221_ODR_1HZ) || \ 00145 (ODR == HTS221_ODR_7HZ) || (ODR == HTS221_ODR_12_5HZ)) 00146 00147 00148 /** 00149 * @brief Push-pull/Open Drain selection on DRDY pin. 00150 */ 00151 typedef enum { 00152 HTS221_PUSHPULL = (uint8_t)0x00, /*!< DRDY pin in push pull */ 00153 HTS221_OPENDRAIN = (uint8_t)0x40 /*!< DRDY pin in open drain */ 00154 } HTS221_OutputType_et; 00155 #define IS_HTS221_OutputType(MODE) ((MODE == HTS221_PUSHPULL) || (MODE == HTS221_OPENDRAIN)) 00156 00157 /** 00158 * @brief Active level of DRDY pin. 00159 */ 00160 typedef enum { 00161 HTS221_HIGH_LVL = (uint8_t)0x00, /*!< HIGH state level for DRDY pin */ 00162 HTS221_LOW_LVL = (uint8_t)0x80 /*!< LOW state level for DRDY pin */ 00163 } HTS221_DrdyLevel_et; 00164 #define IS_HTS221_DrdyLevelType(MODE) ((MODE == HTS221_HIGH_LVL) || (MODE == HTS221_LOW_LVL)) 00165 00166 /** 00167 * @brief Driver Version Info structure definition. 00168 */ 00169 typedef struct { 00170 uint8_t Major; 00171 uint8_t Minor; 00172 uint8_t Point; 00173 } HTS221_DriverVersion_st; 00174 00175 00176 /** 00177 * @brief HTS221 Init structure definition. 00178 */ 00179 typedef struct { 00180 HTS221_Avgh_et avg_h ; /*!< Humidity average */ 00181 HTS221_Avgt_et avg_t ; /*!< Temperature average */ 00182 HTS221_Odr_et odr ; /*!< Output data rate */ 00183 HTS221_State_et bdu_status ; /*!< HTS221_ENABLE/HTS221_DISABLE the block data update */ 00184 HTS221_State_et heater_status ; /*!< HTS221_ENABLE/HTS221_DISABLE the internal heater */ 00185 00186 HTS221_DrdyLevel_et irq_level ; /*!< HTS221_HIGH_LVL/HTS221_LOW_LVL the level for DRDY pin */ 00187 HTS221_OutputType_et irq_output_type ; /*!< Output configuration for DRDY pin */ 00188 HTS221_State_et irq_enable ; /*!< HTS221_ENABLE/HTS221_DISABLE interrupt on DRDY pin */ 00189 } HTS221_Init_st; 00190 00191 /** 00192 * @} 00193 */ 00194 00195 00196 /* Exported Constants ---------------------------------------------------------*/ 00197 /** @defgroup HTS221_Exported_Constants 00198 * @{ 00199 */ 00200 00201 /** 00202 * @brief Bitfield positioning. 00203 */ 00204 #define HTS221_BIT(x) ((uint8_t)x) 00205 00206 /** 00207 * @brief I2C address. 00208 */ 00209 #define HTS221_I2C_ADDRESS (uint8_t)0xBE 00210 00211 /** 00212 * @brief Driver version. 00213 */ 00214 #define HTS221_DRIVER_VERSION_MAJOR (uint8_t)1 00215 #define HTS221_DRIVER_VERSION_MINOR (uint8_t)1 00216 #define HTS221_DRIVER_VERSION_POINT (uint8_t)0 00217 00218 /** 00219 * @addtogroup HTS221_Registers 00220 * @{ 00221 */ 00222 00223 00224 /** 00225 * @brief Device Identification register. 00226 * \code 00227 * Read 00228 * Default value: 0xBC 00229 * 7:0 This read-only register contains the device identifier for HTS221. 00230 * \endcode 00231 */ 00232 #define HTS221_WHO_AM_I_REG (uint8_t)0x0F 00233 00234 /** 00235 * @brief Device Identification value. 00236 */ 00237 #define HTS221_WHO_AM_I_VAL (uint8_t)0xBC 00238 00239 00240 /** 00241 * @brief Humidity and temperature average mode register. 00242 * \code 00243 * Read/write 00244 * Default value: 0x1B 00245 * 7:6 Reserved. 00246 * 5:3 AVGT2-AVGT1-AVGT0: Select the temperature internal average. 00247 * 00248 * AVGT2 | AVGT1 | AVGT0 | Nr. Internal Average 00249 * ---------------------------------------------------- 00250 * 0 | 0 | 0 | 2 00251 * 0 | 0 | 1 | 4 00252 * 0 | 1 | 0 | 8 00253 * 0 | 1 | 1 | 16 00254 * 1 | 0 | 0 | 32 00255 * 1 | 0 | 1 | 64 00256 * 1 | 1 | 0 | 128 00257 * 1 | 1 | 1 | 256 00258 * 00259 * 2:0 AVGH2-AVGH1-AVGH0: Select humidity internal average. 00260 * AVGH2 | AVGH1 | AVGH0 | Nr. Internal Average 00261 * ------------------------------------------------------ 00262 * 0 | 0 | 0 | 4 00263 * 0 | 0 | 1 | 8 00264 * 0 | 1 | 0 | 16 00265 * 0 | 1 | 1 | 32 00266 * 1 | 0 | 0 | 64 00267 * 1 | 0 | 1 | 128 00268 * 1 | 1 | 0 | 256 00269 * 1 | 1 | 1 | 512 00270 * 00271 * \endcode 00272 */ 00273 #define HTS221_AV_CONF_REG (uint8_t)0x10 00274 00275 #define HTS221_AVGT_BIT HTS221_BIT(3) 00276 #define HTS221_AVGH_BIT HTS221_BIT(0) 00277 00278 #define HTS221_AVGH_MASK (uint8_t)0x07 00279 #define HTS221_AVGT_MASK (uint8_t)0x38 00280 00281 /** 00282 * @brief Control register 1. 00283 * \code 00284 * Read/write 00285 * Default value: 0x00 00286 * 7 PD: power down control. 0 - power down mode; 1 - active mode. 00287 * 6:3 Reserved. 00288 * 2 BDU: block data update. 0 - continuous update; 1 - output registers not updated until MSB and LSB reading. 00289 * 1:0 ODR1, ODR0: output data rate selection. 00290 * 00291 * ODR1 | ODR0 | Humidity output data-rate(Hz) | Pressure output data-rate(Hz) 00292 * ---------------------------------------------------------------------------------- 00293 * 0 | 0 | one shot | one shot 00294 * 0 | 1 | 1 | 1 00295 * 1 | 0 | 7 | 7 00296 * 1 | 1 | 12.5 | 12.5 00297 * 00298 * \endcode 00299 */ 00300 #define HTS221_CTRL_REG1 (uint8_t)0x20 00301 00302 #define HTS221_PD_BIT HTS221_BIT(7) 00303 #define HTS221_BDU_BIT HTS221_BIT(2) 00304 #define HTS221_ODR_BIT HTS221_BIT(0) 00305 00306 #define HTS221_PD_MASK (uint8_t)0x80 00307 #define HTS221_BDU_MASK (uint8_t)0x04 00308 #define HTS221_ODR_MASK (uint8_t)0x03 00309 00310 /** 00311 * @brief Control register 2. 00312 * \code 00313 * Read/write 00314 * Default value: 0x00 00315 * 7 BOOT: Reboot memory content. 0: normal mode; 1: reboot memory content. Self-cleared upon completation. 00316 * 6:2 Reserved. 00317 * 1 HEATHER: 0: heater enable; 1: heater disable. 00318 * 0 ONE_SHOT: 0: waiting for start of conversion; 1: start for a new dataset. Self-cleared upon completation. 00319 * \endcode 00320 */ 00321 #define HTS221_CTRL_REG2 (uint8_t)0x21 00322 00323 #define HTS221_BOOT_BIT HTS221_BIT(7) 00324 #define HTS221_HEATHER_BIT HTS221_BIT(1) 00325 #define HTS221_ONESHOT_BIT HTS221_BIT(0) 00326 00327 #define HTS221_BOOT_MASK (uint8_t)0x80 00328 #define HTS221_HEATHER_MASK (uint8_t)0x02 00329 #define HTS221_ONE_SHOT_MASK (uint8_t)0x01 00330 00331 /** 00332 * @brief Control register 3. 00333 * \code 00334 * Read/write 00335 * Default value: 0x00 00336 * 7 DRDY_H_L: Interrupt edge. 0: active high, 1: active low. 00337 * 6 PP_OD: Push-Pull/OpenDrain selection on interrupt pads. 0: push-pull; 1: open drain. 00338 * 5:3 Reserved. 00339 * 2 DRDY: interrupt config. 0: disable, 1: enable. 00340 * \endcode 00341 */ 00342 #define HTS221_CTRL_REG3 (uint8_t)0x22 00343 00344 #define HTS221_DRDY_H_L_BIT HTS221_BIT(7) 00345 #define HTS221_PP_OD_BIT HTS221_BIT(6) 00346 #define HTS221_DRDY_BIT HTS221_BIT(2) 00347 00348 #define HTS221_DRDY_H_L_MASK (uint8_t)0x80 00349 #define HTS221_PP_OD_MASK (uint8_t)0x40 00350 #define HTS221_DRDY_MASK (uint8_t)0x04 00351 00352 /** 00353 * @brief Status register. 00354 * \code 00355 * Read 00356 * Default value: 0x00 00357 * 7:2 Reserved. 00358 * 1 H_DA: Humidity data available. 0: new data for humidity is not yet available; 1: new data for humidity is available. 00359 * 0 T_DA: Temperature data available. 0: new data for temperature is not yet available; 1: new data for temperature is available. 00360 * \endcode 00361 */ 00362 #define HTS221_STATUS_REG (uint8_t)0x27 00363 00364 #define HTS221_H_DA_BIT HTS221_BIT(1) 00365 #define HTS221_T_DA_BIT HTS221_BIT(0) 00366 00367 #define HTS221_HDA_MASK (uint8_t)0x02 00368 #define HTS221_TDA_MASK (uint8_t)0x01 00369 00370 /** 00371 * @brief Humidity data (LSB). 00372 * \code 00373 * Read 00374 * Default value: 0x00. 00375 * HOUT7 - HOUT0: Humidity data LSB (2's complement). 00376 * \endcode 00377 */ 00378 #define HTS221_HR_OUT_L_REG (uint8_t)0x28 00379 00380 /** 00381 * @brief Humidity data (MSB). 00382 * \code 00383 * Read 00384 * Default value: 0x00. 00385 * HOUT15 - HOUT8: Humidity data MSB (2's complement). 00386 * \endcode 00387 */ 00388 #define HTS221_HR_OUT_H_REG (uint8_t)0x29 00389 00390 00391 /** 00392 * @brief Temperature data (LSB). 00393 * \code 00394 * Read 00395 * Default value: 0x00. 00396 * TOUT7 - TOUT0: temperature data LSB. 00397 * \endcode 00398 */ 00399 #define HTS221_TEMP_OUT_L_REG (uint8_t)0x2A 00400 00401 /** 00402 * @brief Temperature data (MSB). 00403 * \code 00404 * Read 00405 * Default value: 0x00. 00406 * TOUT15 - TOUT8: temperature data MSB. 00407 * \endcode 00408 */ 00409 #define HTS221_TEMP_OUT_H_REG (uint8_t)0x2B 00410 00411 /** 00412 * @brief Calibration registers. 00413 * \code 00414 * Read 00415 * \endcode 00416 */ 00417 #define HTS221_H0_RH_X2 (uint8_t)0x30 00418 #define HTS221_H1_RH_X2 (uint8_t)0x31 00419 #define HTS221_T0_DEGC_X8 (uint8_t)0x32 00420 #define HTS221_T1_DEGC_X8 (uint8_t)0x33 00421 #define HTS221_T0_T1_DEGC_H2 (uint8_t)0x35 00422 #define HTS221_H0_T0_OUT_L (uint8_t)0x36 00423 #define HTS221_H0_T0_OUT_H (uint8_t)0x37 00424 #define HTS221_H1_T0_OUT_L (uint8_t)0x3A 00425 #define HTS221_H1_T0_OUT_H (uint8_t)0x3B 00426 #define HTS221_T0_OUT_L (uint8_t)0x3C 00427 #define HTS221_T0_OUT_H (uint8_t)0x3D 00428 #define HTS221_T1_OUT_L (uint8_t)0x3E 00429 #define HTS221_T1_OUT_H (uint8_t)0x3F 00430 00431 00432 /** 00433 * @} 00434 */ 00435 00436 00437 /** 00438 * @} 00439 */ 00440 00441 00442 /* Exported Functions -------------------------------------------------------------*/ 00443 /** @defgroup HTS221_Exported_Functions 00444 * @{ 00445 */ 00446 00447 HTS221_Error_et HTS221_read_reg(void *handle, uint8_t RegAddr, uint16_t NumByteToRead, uint8_t *Data); 00448 HTS221_Error_et HTS221_write_reg(void *handle, uint8_t RegAddr, uint16_t NumByteToWrite, uint8_t *Data); 00449 00450 HTS221_Error_et HTS221_Get_DriverVersion(HTS221_DriverVersion_st *version); 00451 HTS221_Error_et HTS221_Get_DeviceID(void *handle, uint8_t *deviceid); 00452 00453 HTS221_Error_et HTS221_Set_InitConfig(void *handle, HTS221_Init_st *pxInit); 00454 HTS221_Error_et HTS221_Get_InitConfig(void *handle, HTS221_Init_st *pxInit); 00455 HTS221_Error_et HTS221_DeInit(void *handle); 00456 HTS221_Error_et HTS221_IsMeasurementCompleted(void *handle, HTS221_BitStatus_et *Is_Measurement_Completed); 00457 00458 HTS221_Error_et HTS221_Get_Measurement(void *handle, uint16_t *humidity, int16_t *temperature); 00459 HTS221_Error_et HTS221_Get_RawMeasurement(void *handle, int16_t *humidity, int16_t *temperature); 00460 HTS221_Error_et HTS221_Get_Humidity(void *handle, uint16_t *value); 00461 HTS221_Error_et HTS221_Get_HumidityRaw(void *handle, int16_t *value); 00462 HTS221_Error_et HTS221_Get_TemperatureRaw(void *handle, int16_t *value); 00463 HTS221_Error_et HTS221_Get_Temperature(void *handle, int16_t *value); 00464 HTS221_Error_et HTS221_Get_DataStatus(void *handle, HTS221_BitStatus_et *humidity, HTS221_BitStatus_et *temperature); 00465 HTS221_Error_et HTS221_Activate(void *handle); 00466 HTS221_Error_et HTS221_DeActivate(void *handle); 00467 00468 HTS221_Error_et HTS221_Set_AvgHT(void *handle, HTS221_Avgh_et avgh, HTS221_Avgt_et avgt); 00469 HTS221_Error_et HTS221_Set_AvgH(void *handle, HTS221_Avgh_et avgh); 00470 HTS221_Error_et HTS221_Set_AvgT(void *handle, HTS221_Avgt_et avgt); 00471 HTS221_Error_et HTS221_Get_AvgHT(void *handle, HTS221_Avgh_et *avgh, HTS221_Avgt_et *avgt); 00472 HTS221_Error_et HTS221_Set_BduMode(void *handle, HTS221_State_et status); 00473 HTS221_Error_et HTS221_Get_BduMode(void *handle, HTS221_State_et *status); 00474 HTS221_Error_et HTS221_Set_PowerDownMode(void *handle, HTS221_BitStatus_et status); 00475 HTS221_Error_et HTS221_Get_PowerDownMode(void *handle, HTS221_BitStatus_et *status); 00476 HTS221_Error_et HTS221_Set_Odr(void *handle, HTS221_Odr_et odr); 00477 HTS221_Error_et HTS221_Get_Odr(void *handle, HTS221_Odr_et *odr); 00478 HTS221_Error_et HTS221_MemoryBoot(void *handle); 00479 HTS221_Error_et HTS221_Set_HeaterState(void *handle, HTS221_State_et status); 00480 HTS221_Error_et HTS221_Get_HeaterState(void *handle, HTS221_State_et *status); 00481 HTS221_Error_et HTS221_StartOneShotMeasurement(void *handle); 00482 HTS221_Error_et HTS221_Set_IrqActiveLevel(void *handle, HTS221_DrdyLevel_et status); 00483 HTS221_Error_et HTS221_Get_IrqActiveLevel(void *handle, HTS221_DrdyLevel_et *status); 00484 HTS221_Error_et HTS221_Set_IrqOutputType(void *handle, HTS221_OutputType_et value); 00485 HTS221_Error_et HTS221_Get_IrqOutputType(void *handle, HTS221_OutputType_et *value); 00486 HTS221_Error_et HTS221_Set_IrqEnable(void *handle, HTS221_State_et status); 00487 HTS221_Error_et HTS221_Get_IrqEnable(void *handle, HTS221_State_et *status); 00488 00489 /** 00490 * @} 00491 */ 00492 00493 /** 00494 * @} 00495 */ 00496 00497 /** 00498 * @} 00499 */ 00500 00501 #ifdef __cplusplus 00502 } 00503 #endif 00504 00505 #endif /* __HTS221_DRIVER__H */ 00506 00507 /******************* (C) COPYRIGHT 2013 STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 20:43:31 by
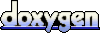