STM32L476G-Discovery board drivers V1.0.0
Dependents: DiscoLogger DISCO_L476VG_GlassLCD DISCO_L476VG_MicrophoneRecorder DISCO_L476VG_UART ... more
mfxstm32l152.c
00001 /** 00002 ****************************************************************************** 00003 * @file mfxstm32l152.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the MFXSTM32L152 00006 * IO Expander devices. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© Copyright (c) 2015 STMicroelectronics. 00011 * All rights reserved.</center></h2> 00012 * 00013 * This software component is licensed by ST under BSD 3-Clause license, 00014 * the "License"; You may not use this file except in compliance with the 00015 * License. You may obtain a copy of the License at: 00016 * opensource.org/licenses/BSD-3-Clause 00017 * 00018 ****************************************************************************** 00019 */ 00020 00021 /* Includes ------------------------------------------------------------------*/ 00022 #include "mfxstm32l152.h" 00023 00024 /** @addtogroup BSP 00025 * @{ 00026 */ 00027 00028 /** @addtogroup Component 00029 * @{ 00030 */ 00031 00032 /** @defgroup MFXSTM32L152 00033 * @{ 00034 */ 00035 00036 /* Private typedef -----------------------------------------------------------*/ 00037 00038 /** @defgroup MFXSTM32L152_Private_Types_Definitions 00039 * @{ 00040 */ 00041 00042 /* Private define ------------------------------------------------------------*/ 00043 00044 /** @defgroup MFXSTM32L152_Private_Defines 00045 * @{ 00046 */ 00047 #define MFXSTM32L152_MAX_INSTANCE 3 00048 00049 /* Private macro -------------------------------------------------------------*/ 00050 00051 /** @defgroup MFXSTM32L152_Private_Macros 00052 * @{ 00053 */ 00054 00055 /* Private variables ---------------------------------------------------------*/ 00056 00057 /** @defgroup MFXSTM32L152_Private_Variables 00058 * @{ 00059 */ 00060 00061 /* Touch screen driver structure initialization */ 00062 TS_DrvTypeDef mfxstm32l152_ts_drv = 00063 { 00064 mfxstm32l152_Init, 00065 mfxstm32l152_ReadID, 00066 mfxstm32l152_Reset, 00067 00068 mfxstm32l152_TS_Start, 00069 mfxstm32l152_TS_DetectTouch, 00070 mfxstm32l152_TS_GetXY, 00071 00072 mfxstm32l152_TS_EnableIT, 00073 mfxstm32l152_TS_ClearIT, 00074 mfxstm32l152_TS_ITStatus, 00075 mfxstm32l152_TS_DisableIT, 00076 }; 00077 00078 /* IO driver structure initialization */ 00079 IO_DrvTypeDef mfxstm32l152_io_drv = 00080 { 00081 mfxstm32l152_Init, 00082 mfxstm32l152_ReadID, 00083 mfxstm32l152_Reset, 00084 00085 mfxstm32l152_IO_Start, 00086 mfxstm32l152_IO_Config, 00087 mfxstm32l152_IO_WritePin, 00088 mfxstm32l152_IO_ReadPin, 00089 00090 mfxstm32l152_IO_EnableIT, 00091 mfxstm32l152_IO_DisableIT, 00092 mfxstm32l152_IO_ITStatus, 00093 mfxstm32l152_IO_ClearIT, 00094 }; 00095 00096 /* IDD driver structure initialization */ 00097 IDD_DrvTypeDef mfxstm32l152_idd_drv = 00098 { 00099 mfxstm32l152_Init, 00100 mfxstm32l152_DeInit, 00101 mfxstm32l152_ReadID, 00102 mfxstm32l152_Reset, 00103 mfxstm32l152_LowPower, 00104 mfxstm32l152_WakeUp, 00105 00106 mfxstm32l152_IDD_Start, 00107 mfxstm32l152_IDD_Config, 00108 mfxstm32l152_IDD_GetValue, 00109 00110 mfxstm32l152_IDD_EnableIT, 00111 mfxstm32l152_IDD_ClearIT, 00112 mfxstm32l152_IDD_GetITStatus, 00113 mfxstm32l152_IDD_DisableIT, 00114 00115 mfxstm32l152_Error_EnableIT, 00116 mfxstm32l152_Error_ClearIT, 00117 mfxstm32l152_Error_GetITStatus, 00118 mfxstm32l152_Error_DisableIT, 00119 mfxstm32l152_Error_ReadSrc, 00120 mfxstm32l152_Error_ReadMsg 00121 }; 00122 00123 00124 /* mfxstm32l152 instances by address */ 00125 uint8_t mfxstm32l152[MFXSTM32L152_MAX_INSTANCE] = {0}; 00126 /** 00127 * @} 00128 */ 00129 00130 /* Private function prototypes -----------------------------------------------*/ 00131 00132 /** @defgroup MFXSTM32L152_Private_Function_Prototypes 00133 * @{ 00134 */ 00135 static uint8_t mfxstm32l152_GetInstance(uint16_t DeviceAddr); 00136 static uint8_t mfxstm32l152_ReleaseInstance(uint16_t DeviceAddr); 00137 static void mfxstm32l152_reg24_setPinValue(uint16_t DeviceAddr, uint8_t RegisterAddr, uint32_t PinPosition, uint8_t PinValue ); 00138 00139 /* Private functions ---------------------------------------------------------*/ 00140 00141 /** @defgroup MFXSTM32L152_Private_Functions 00142 * @{ 00143 */ 00144 00145 /** 00146 * @brief Initialize the mfxstm32l152 and configure the needed hardware resources 00147 * @param DeviceAddr: Device address on communication Bus. 00148 * @retval None 00149 */ 00150 void mfxstm32l152_Init(uint16_t DeviceAddr) 00151 { 00152 uint8_t instance; 00153 uint8_t empty; 00154 00155 /* Check if device instance already exists */ 00156 instance = mfxstm32l152_GetInstance(DeviceAddr); 00157 00158 /* To prevent double initialization */ 00159 if(instance == 0xFF) 00160 { 00161 /* Look for empty instance */ 00162 empty = mfxstm32l152_GetInstance(0); 00163 00164 if(empty < MFXSTM32L152_MAX_INSTANCE) 00165 { 00166 /* Register the current device instance */ 00167 mfxstm32l152[empty] = DeviceAddr; 00168 00169 /* Initialize IO BUS layer */ 00170 MFX_IO_Init(); 00171 } 00172 } 00173 00174 mfxstm32l152_SetIrqOutPinPolarity(DeviceAddr, MFXSTM32L152_OUT_PIN_POLARITY_HIGH); 00175 mfxstm32l152_SetIrqOutPinType(DeviceAddr, MFXSTM32L152_OUT_PIN_TYPE_PUSHPULL); 00176 } 00177 00178 /** 00179 * @brief DeInitialize the mfxstm32l152 and unconfigure the needed hardware resources 00180 * @param DeviceAddr: Device address on communication Bus. 00181 * @retval None 00182 */ 00183 void mfxstm32l152_DeInit(uint16_t DeviceAddr) 00184 { 00185 uint8_t instance; 00186 00187 /* release existing instance */ 00188 instance = mfxstm32l152_ReleaseInstance(DeviceAddr); 00189 00190 /* De-Init only if instance was previously registered */ 00191 if(instance != 0xFF) 00192 { 00193 /* De-Initialize IO BUS layer */ 00194 MFX_IO_DeInit(); 00195 } 00196 } 00197 00198 /** 00199 * @brief Reset the mfxstm32l152 by Software. 00200 * @param DeviceAddr: Device address on communication Bus. 00201 * @retval None 00202 */ 00203 void mfxstm32l152_Reset(uint16_t DeviceAddr) 00204 { 00205 /* Soft Reset */ 00206 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, MFXSTM32L152_SWRST); 00207 00208 /* Wait for a delay to ensure registers erasing */ 00209 MFX_IO_Delay(10); 00210 } 00211 00212 /** 00213 * @brief Put mfxstm32l152 Device in Low Power standby mode 00214 * @param DeviceAddr: Device address on communication Bus. 00215 * @retval None 00216 */ 00217 void mfxstm32l152_LowPower(uint16_t DeviceAddr) 00218 { 00219 /* Enter standby mode */ 00220 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, MFXSTM32L152_STANDBY); 00221 00222 /* enable wakeup pin */ 00223 MFX_IO_EnableWakeupPin(); 00224 } 00225 00226 /** 00227 * @brief WakeUp mfxstm32l152 from standby mode 00228 * @param DeviceAddr: Device address on communication Bus. 00229 * @retval None 00230 */ 00231 void mfxstm32l152_WakeUp(uint16_t DeviceAddr) 00232 { 00233 uint8_t instance; 00234 00235 /* Check if device instance already exists */ 00236 instance = mfxstm32l152_GetInstance(DeviceAddr); 00237 00238 /* if instance does not exist, first initialize pins*/ 00239 if(instance == 0xFF) 00240 { 00241 /* enable wakeup pin */ 00242 MFX_IO_EnableWakeupPin(); 00243 } 00244 00245 /* toggle wakeup pin */ 00246 MFX_IO_Wakeup(); 00247 } 00248 00249 /** 00250 * @brief Read the MFXSTM32L152 IO Expander device ID. 00251 * @param DeviceAddr: Device address on communication Bus. 00252 * @retval The Device ID (two bytes). 00253 */ 00254 uint16_t mfxstm32l152_ReadID(uint16_t DeviceAddr) 00255 { 00256 uint8_t id; 00257 00258 /* Wait for a delay to ensure the state of registers */ 00259 MFX_IO_Delay(1); 00260 00261 /* Initialize IO BUS layer */ 00262 MFX_IO_Init(); 00263 00264 id = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_ID); 00265 00266 /* Return the device ID value */ 00267 return (id); 00268 } 00269 00270 /** 00271 * @brief Read the MFXSTM32L152 device firmware version. 00272 * @param DeviceAddr: Device address on communication Bus. 00273 * @retval The Device FW version (two bytes). 00274 */ 00275 uint16_t mfxstm32l152_ReadFwVersion(uint16_t DeviceAddr) 00276 { 00277 uint8_t data[2]; 00278 00279 MFX_IO_ReadMultiple((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_FW_VERSION_MSB, data, sizeof(data)) ; 00280 00281 /* Recompose MFX firmware value */ 00282 return ((data[0] << 8) | data[1]); 00283 } 00284 00285 /** 00286 * @brief Enable the interrupt mode for the selected IT source 00287 * @param DeviceAddr: Device address on communication Bus. 00288 * @param Source: The interrupt source to be configured, could be: 00289 * @arg MFXSTM32L152_IRQ_GPIO: IO interrupt 00290 * @arg MFXSTM32L152_IRQ_IDD : IDD interrupt 00291 * @arg MFXSTM32L152_IRQ_ERROR : Error interrupt 00292 * @arg MFXSTM32L152_IRQ_TS_DET : Touch Screen Controller Touch Detected interrupt 00293 * @arg MFXSTM32L152_IRQ_TS_NE : Touch Screen FIFO Not Empty 00294 * @arg MFXSTM32L152_IRQ_TS_TH : Touch Screen FIFO threshold triggered 00295 * @arg MFXSTM32L152_IRQ_TS_FULL : Touch Screen FIFO Full 00296 * @arg MFXSTM32L152_IRQ_TS_OVF : Touch Screen FIFO Overflow 00297 * @retval None 00298 */ 00299 void mfxstm32l152_EnableITSource(uint16_t DeviceAddr, uint8_t Source) 00300 { 00301 uint8_t tmp = 0; 00302 00303 /* Get the current value of the INT_EN register */ 00304 tmp = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_SRC_EN); 00305 00306 /* Set the interrupts to be Enabled */ 00307 tmp |= Source; 00308 00309 /* Set the register */ 00310 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_SRC_EN, tmp); 00311 } 00312 00313 /** 00314 * @brief Disable the interrupt mode for the selected IT source 00315 * @param DeviceAddr: Device address on communication Bus. 00316 * @param Source: The interrupt source to be configured, could be: 00317 * @arg MFXSTM32L152_IRQ_GPIO: IO interrupt 00318 * @arg MFXSTM32L152_IRQ_IDD : IDD interrupt 00319 * @arg MFXSTM32L152_IRQ_ERROR : Error interrupt 00320 * @arg MFXSTM32L152_IRQ_TS_DET : Touch Screen Controller Touch Detected interrupt 00321 * @arg MFXSTM32L152_IRQ_TS_NE : Touch Screen FIFO Not Empty 00322 * @arg MFXSTM32L152_IRQ_TS_TH : Touch Screen FIFO threshold triggered 00323 * @arg MFXSTM32L152_IRQ_TS_FULL : Touch Screen FIFO Full 00324 * @arg MFXSTM32L152_IRQ_TS_OVF : Touch Screen FIFO Overflow 00325 * @retval None 00326 */ 00327 void mfxstm32l152_DisableITSource(uint16_t DeviceAddr, uint8_t Source) 00328 { 00329 uint8_t tmp = 0; 00330 00331 /* Get the current value of the INT_EN register */ 00332 tmp = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_SRC_EN); 00333 00334 /* Set the interrupts to be Enabled */ 00335 tmp &= ~Source; 00336 00337 /* Set the register */ 00338 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_SRC_EN, tmp); 00339 } 00340 00341 00342 /** 00343 * @brief Returns the selected Global interrupt source pending bit value 00344 * @param DeviceAddr: Device address on communication Bus. 00345 * @param Source: the Global interrupt source to be checked, could be: 00346 * @arg MFXSTM32L152_IRQ_GPIO: IO interrupt 00347 * @arg MFXSTM32L152_IRQ_IDD : IDD interrupt 00348 * @arg MFXSTM32L152_IRQ_ERROR : Error interrupt 00349 * @arg MFXSTM32L152_IRQ_TS_DET : Touch Screen Controller Touch Detected interrupt 00350 * @arg MFXSTM32L152_IRQ_TS_NE : Touch Screen FIFO Not Empty 00351 * @arg MFXSTM32L152_IRQ_TS_TH : Touch Screen FIFO threshold triggered 00352 * @arg MFXSTM32L152_IRQ_TS_FULL : Touch Screen FIFO Full 00353 * @arg MFXSTM32L152_IRQ_TS_OVF : Touch Screen FIFO Overflow 00354 * @retval The value of the checked Global interrupt source status. 00355 */ 00356 uint8_t mfxstm32l152_GlobalITStatus(uint16_t DeviceAddr, uint8_t Source) 00357 { 00358 /* Return the global IT source status (pending or not)*/ 00359 return((MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_PENDING) & Source)); 00360 } 00361 00362 /** 00363 * @brief Clear the selected Global interrupt pending bit(s) 00364 * @param DeviceAddr: Device address on communication Bus. 00365 * @param Source: the Global interrupt source to be cleared, could be any combination 00366 * of the below values. The acknowledge signal for MFXSTM32L152_GPIOs configured in input 00367 * with interrupt is not on this register but in IRQ_GPI_ACK1, IRQ_GPI_ACK2 registers. 00368 * @arg MFXSTM32L152_IRQ_IDD : IDD interrupt 00369 * @arg MFXSTM32L152_IRQ_ERROR : Error interrupt 00370 * @arg MFXSTM32L152_IRQ_TS_DET : Touch Screen Controller Touch Detected interrupt 00371 * @arg MFXSTM32L152_IRQ_TS_NE : Touch Screen FIFO Not Empty 00372 * @arg MFXSTM32L152_IRQ_TS_TH : Touch Screen FIFO threshold triggered 00373 * @arg MFXSTM32L152_IRQ_TS_FULL : Touch Screen FIFO Full 00374 * @arg MFXSTM32L152_IRQ_TS_OVF : Touch Screen FIFO Overflow 00375 * /\/\ IMPORTANT NOTE /\/\ must not use MFXSTM32L152_IRQ_GPIO as argument, see IRQ_GPI_ACK1 and IRQ_GPI_ACK2 registers 00376 * @retval None 00377 */ 00378 void mfxstm32l152_ClearGlobalIT(uint16_t DeviceAddr, uint8_t Source) 00379 { 00380 /* Write 1 to the bits that have to be cleared */ 00381 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_ACK, Source); 00382 } 00383 00384 /** 00385 * @brief Set the global interrupt Polarity of IRQ_OUT_PIN. 00386 * @param DeviceAddr: Device address on communication Bus. 00387 * @param Polarity: the IT mode polarity, could be one of the following values: 00388 * @arg MFXSTM32L152_OUT_PIN_POLARITY_LOW: Interrupt output line is active Low edge 00389 * @arg MFXSTM32L152_OUT_PIN_POLARITY_HIGH: Interrupt line output is active High edge 00390 * @retval None 00391 */ 00392 void mfxstm32l152_SetIrqOutPinPolarity(uint16_t DeviceAddr, uint8_t Polarity) 00393 { 00394 uint8_t tmp = 0; 00395 00396 /* Get the current register value */ 00397 tmp = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_MFX_IRQ_OUT); 00398 00399 /* Mask the polarity bits */ 00400 tmp &= ~(uint8_t)0x02; 00401 00402 /* Modify the Interrupt Output line configuration */ 00403 tmp |= Polarity; 00404 00405 /* Set the new register value */ 00406 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_MFX_IRQ_OUT, tmp); 00407 00408 /* Wait for 1 ms for MFX to change IRQ_out pin config, before activate it */ 00409 MFX_IO_Delay(1); 00410 00411 } 00412 00413 /** 00414 * @brief Set the global interrupt Type of IRQ_OUT_PIN. 00415 * @param DeviceAddr: Device address on communication Bus. 00416 * @param Type: Interrupt line activity type, could be one of the following values: 00417 * @arg MFXSTM32L152_OUT_PIN_TYPE_OPENDRAIN: Open Drain output Interrupt line 00418 * @arg MFXSTM32L152_OUT_PIN_TYPE_PUSHPULL: Push Pull output Interrupt line 00419 * @retval None 00420 */ 00421 void mfxstm32l152_SetIrqOutPinType(uint16_t DeviceAddr, uint8_t Type) 00422 { 00423 uint8_t tmp = 0; 00424 00425 /* Get the current register value */ 00426 tmp = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_MFX_IRQ_OUT); 00427 00428 /* Mask the type bits */ 00429 tmp &= ~(uint8_t)0x01; 00430 00431 /* Modify the Interrupt Output line configuration */ 00432 tmp |= Type; 00433 00434 /* Set the new register value */ 00435 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_MFX_IRQ_OUT, tmp); 00436 00437 /* Wait for 1 ms for MFX to change IRQ_out pin config, before activate it */ 00438 MFX_IO_Delay(1); 00439 00440 } 00441 00442 00443 /* ------------------------------------------------------------------ */ 00444 /* ----------------------- GPIO ------------------------------------- */ 00445 /* ------------------------------------------------------------------ */ 00446 00447 00448 /** 00449 * @brief Start the IO functionality used and enable the AF for selected IO pin(s). 00450 * @param DeviceAddr: Device address on communication Bus. 00451 * @param AF_en: 0 to disable, else enabled. 00452 * @retval None 00453 */ 00454 void mfxstm32l152_IO_Start(uint16_t DeviceAddr, uint32_t IO_Pin) 00455 { 00456 uint8_t mode; 00457 00458 /* Get the current register value */ 00459 mode = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL); 00460 00461 /* Set the IO Functionalities to be Enabled */ 00462 mode |= MFXSTM32L152_GPIO_EN; 00463 00464 /* Enable ALTERNATE functions */ 00465 /* AGPIO[0..3] can be either IDD or GPIO */ 00466 /* AGPIO[4..7] can be either TS or GPIO */ 00467 /* if IDD or TS are enabled no matter the value this bit GPIO are not available for those pins */ 00468 /* however the MFX will waste some cycles to to handle these potential GPIO (pooling, etc) */ 00469 /* so if IDD and TS are both active it is better to let ALTERNATE off (0) */ 00470 /* if however IDD or TS are not connected then set it on gives more GPIOs availability */ 00471 /* remind that AGPIO are less efficient then normal GPIO (They use pooling rather then EXTI */ 00472 if (IO_Pin > 0xFFFF) 00473 { 00474 mode |= MFXSTM32L152_ALTERNATE_GPIO_EN; 00475 } 00476 else 00477 { 00478 mode &= ~MFXSTM32L152_ALTERNATE_GPIO_EN; 00479 } 00480 00481 /* Write the new register value */ 00482 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, mode); 00483 00484 /* Wait for 1 ms for MFX to change IRQ_out pin config, before activate it */ 00485 MFX_IO_Delay(1); 00486 } 00487 00488 /** 00489 * @brief Configures the IO pin(s) according to IO mode structure value. 00490 * @param DeviceAddr: Device address on communication Bus. 00491 * @param IO_Pin: The output pin to be set or reset. This parameter can be one 00492 * of the following values: 00493 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00494 * @param IO_Mode: The IO pin mode to configure, could be one of the following values: 00495 * @arg IO_MODE_INPUT 00496 * @arg IO_MODE_OUTPUT 00497 * @arg IO_MODE_IT_RISING_EDGE 00498 * @arg IO_MODE_IT_FALLING_EDGE 00499 * @arg IO_MODE_IT_LOW_LEVEL 00500 * @arg IO_MODE_IT_HIGH_LEVEL 00501 * @arg IO_MODE_INPUT_PU, 00502 * @arg IO_MODE_INPUT_PD, 00503 * @arg IO_MODE_OUTPUT_OD_PU, 00504 * @arg IO_MODE_OUTPUT_OD_PD, 00505 * @arg IO_MODE_OUTPUT_PP_PU, 00506 * @arg IO_MODE_OUTPUT_PP_PD, 00507 * @arg IO_MODE_IT_RISING_EDGE_PU 00508 * @arg IO_MODE_IT_FALLING_EDGE_PU 00509 * @arg IO_MODE_IT_LOW_LEVEL_PU 00510 * @arg IO_MODE_IT_HIGH_LEVEL_PU 00511 * @arg IO_MODE_IT_RISING_EDGE_PD 00512 * @arg IO_MODE_IT_FALLING_EDGE_PD 00513 * @arg IO_MODE_IT_LOW_LEVEL_PD 00514 * @arg IO_MODE_IT_HIGH_LEVEL_PD 00515 * @retval None 00516 */ 00517 uint8_t mfxstm32l152_IO_Config(uint16_t DeviceAddr, uint32_t IO_Pin, IO_ModeTypedef IO_Mode) 00518 { 00519 uint8_t error_code = 0; 00520 00521 /* Configure IO pin according to selected IO mode */ 00522 switch(IO_Mode) 00523 { 00524 case IO_MODE_OFF: /* Off or analog mode */ 00525 case IO_MODE_ANALOG: /* Off or analog mode */ 00526 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00527 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00528 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITHOUT_PULL_RESISTOR); 00529 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00530 break; 00531 00532 case IO_MODE_INPUT: /* Input mode */ 00533 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00534 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00535 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITHOUT_PULL_RESISTOR); 00536 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00537 break; 00538 00539 case IO_MODE_INPUT_PU: /* Input mode */ 00540 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00541 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00542 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00543 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00544 break; 00545 00546 case IO_MODE_INPUT_PD: /* Input mode */ 00547 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00548 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00549 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00550 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00551 break; 00552 00553 case IO_MODE_OUTPUT: /* Output mode */ 00554 case IO_MODE_OUTPUT_PP_PD: /* Output mode */ 00555 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00556 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_OUT); 00557 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPO_PUSH_PULL); 00558 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00559 break; 00560 00561 case IO_MODE_OUTPUT_PP_PU: /* Output mode */ 00562 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00563 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_OUT); 00564 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPO_PUSH_PULL); 00565 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00566 break; 00567 00568 case IO_MODE_OUTPUT_OD_PD: /* Output mode */ 00569 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00570 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_OUT); 00571 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPO_OPEN_DRAIN); 00572 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00573 break; 00574 00575 case IO_MODE_OUTPUT_OD_PU: /* Output mode */ 00576 mfxstm32l152_IO_DisablePinIT(DeviceAddr, IO_Pin); /* first disable IT */ 00577 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_OUT); 00578 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPO_OPEN_DRAIN); 00579 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00580 break; 00581 00582 case IO_MODE_IT_RISING_EDGE: /* Interrupt rising edge mode */ 00583 mfxstm32l152_IO_EnableIT(DeviceAddr); 00584 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00585 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITHOUT_PULL_RESISTOR); 00586 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00587 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_EDGE); 00588 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_HLRE); 00589 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00590 break; 00591 00592 case IO_MODE_IT_RISING_EDGE_PU: /* Interrupt rising edge mode */ 00593 mfxstm32l152_IO_EnableIT(DeviceAddr); 00594 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00595 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00596 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00597 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_EDGE); 00598 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_HLRE); 00599 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00600 break; 00601 00602 case IO_MODE_IT_RISING_EDGE_PD: /* Interrupt rising edge mode */ 00603 mfxstm32l152_IO_EnableIT(DeviceAddr); 00604 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00605 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00606 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00607 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_EDGE); 00608 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_HLRE); 00609 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00610 break; 00611 00612 case IO_MODE_IT_FALLING_EDGE: /* Interrupt falling edge mode */ 00613 mfxstm32l152_IO_EnableIT(DeviceAddr); 00614 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00615 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITHOUT_PULL_RESISTOR); 00616 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00617 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_EDGE); 00618 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_LLFE); 00619 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00620 break; 00621 00622 case IO_MODE_IT_FALLING_EDGE_PU: /* Interrupt falling edge mode */ 00623 mfxstm32l152_IO_EnableIT(DeviceAddr); 00624 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00625 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00626 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00627 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_EDGE); 00628 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_LLFE); 00629 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00630 break; 00631 00632 case IO_MODE_IT_FALLING_EDGE_PD: /* Interrupt falling edge mode */ 00633 mfxstm32l152_IO_EnableIT(DeviceAddr); 00634 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00635 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00636 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00637 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_EDGE); 00638 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_LLFE); 00639 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00640 break; 00641 00642 case IO_MODE_IT_LOW_LEVEL: /* Low level interrupt mode */ 00643 mfxstm32l152_IO_EnableIT(DeviceAddr); 00644 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00645 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITHOUT_PULL_RESISTOR); 00646 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00647 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_LEVEL); 00648 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_LLFE); 00649 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00650 break; 00651 00652 case IO_MODE_IT_LOW_LEVEL_PU: /* Low level interrupt mode */ 00653 mfxstm32l152_IO_EnableIT(DeviceAddr); 00654 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00655 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00656 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00657 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_LEVEL); 00658 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_LLFE); 00659 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00660 break; 00661 00662 case IO_MODE_IT_LOW_LEVEL_PD: /* Low level interrupt mode */ 00663 mfxstm32l152_IO_EnableIT(DeviceAddr); 00664 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00665 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00666 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00667 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_LEVEL); 00668 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_LLFE); 00669 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00670 break; 00671 00672 case IO_MODE_IT_HIGH_LEVEL: /* High level interrupt mode */ 00673 mfxstm32l152_IO_EnableIT(DeviceAddr); 00674 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00675 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITHOUT_PULL_RESISTOR); 00676 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00677 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_LEVEL); 00678 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_HLRE); 00679 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00680 break; 00681 00682 case IO_MODE_IT_HIGH_LEVEL_PU: /* High level interrupt mode */ 00683 mfxstm32l152_IO_EnableIT(DeviceAddr); 00684 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00685 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00686 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_UP); 00687 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_LEVEL); 00688 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_HLRE); 00689 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00690 break; 00691 00692 case IO_MODE_IT_HIGH_LEVEL_PD: /* High level interrupt mode */ 00693 mfxstm32l152_IO_EnableIT(DeviceAddr); 00694 mfxstm32l152_IO_InitPin(DeviceAddr, IO_Pin, MFXSTM32L152_GPIO_DIR_IN); 00695 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_TYPE1, IO_Pin, MFXSTM32L152_GPI_WITH_PULL_RESISTOR); 00696 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_PUPD1, IO_Pin, MFXSTM32L152_GPIO_PULL_DOWN); 00697 mfxstm32l152_IO_SetIrqEvtMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_EVT_LEVEL); 00698 mfxstm32l152_IO_SetIrqTypeMode(DeviceAddr, IO_Pin, MFXSTM32L152_IRQ_GPI_TYPE_HLRE); 00699 mfxstm32l152_IO_EnablePinIT(DeviceAddr, IO_Pin); /* last to do: enable IT */ 00700 break; 00701 00702 default: 00703 error_code = (uint8_t) IO_Mode; 00704 break; 00705 } 00706 00707 return error_code; 00708 } 00709 00710 /** 00711 * @brief Initialize the selected IO pin direction. 00712 * @param DeviceAddr: Device address on communication Bus. 00713 * @param IO_Pin: The IO pin to be configured. This parameter could be any 00714 * combination of the following values: 00715 * @arg MFXSTM32L152_GPIO_PIN_x: Where x can be from 0 to 23. 00716 * @param Direction: could be MFXSTM32L152_GPIO_DIR_IN or MFXSTM32L152_GPIO_DIR_OUT. 00717 * @retval None 00718 */ 00719 void mfxstm32l152_IO_InitPin(uint16_t DeviceAddr, uint32_t IO_Pin, uint8_t Direction) 00720 { 00721 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_DIR1, IO_Pin, Direction); 00722 } 00723 00724 /** 00725 * @brief Set the global interrupt Type. 00726 * @param DeviceAddr: Device address on communication Bus. 00727 * @param IO_Pin: The IO pin to be configured. This parameter could be any 00728 * combination of the following values: 00729 * @arg MFXSTM32L152_GPIO_PIN_x: Where x can be from 0 to 23. 00730 * @param Evt: Interrupt line activity type, could be one of the following values: 00731 * @arg MFXSTM32L152_IRQ_GPI_EVT_LEVEL: Interrupt line is active in level model 00732 * @arg MFXSTM32L152_IRQ_GPI_EVT_EDGE: Interrupt line is active in edge model 00733 * @retval None 00734 */ 00735 void mfxstm32l152_IO_SetIrqEvtMode(uint16_t DeviceAddr, uint32_t IO_Pin, uint8_t Evt) 00736 { 00737 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_EVT1, IO_Pin, Evt); 00738 MFX_IO_Delay(1); 00739 } 00740 00741 /** 00742 * @brief Configure the Edge for which a transition is detectable for the 00743 * selected pin. 00744 * @param DeviceAddr: Device address on communication Bus. 00745 * @param IO_Pin: The IO pin to be configured. This parameter could be any 00746 * combination of the following values: 00747 * @arg MFXSTM32L152_GPIO_PIN_x: Where x can be from 0 to 23. 00748 * @param Evt: Interrupt line activity type, could be one of the following values: 00749 * @arg MFXSTM32L152_IRQ_GPI_TYPE_LLFE: Interrupt line is active in Low Level or Falling Edge 00750 * @arg MFXSTM32L152_IRQ_GPI_TYPE_HLRE: Interrupt line is active in High Level or Rising Edge 00751 * @retval None 00752 */ 00753 void mfxstm32l152_IO_SetIrqTypeMode(uint16_t DeviceAddr, uint32_t IO_Pin, uint8_t Type) 00754 { 00755 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_TYPE1, IO_Pin, Type); 00756 MFX_IO_Delay(1); 00757 } 00758 00759 /** 00760 * @brief When GPIO is in output mode, puts the corresponding GPO in High (1) or Low (0) level. 00761 * @param DeviceAddr: Device address on communication Bus. 00762 * @param IO_Pin: The output pin to be set or reset. This parameter can be one 00763 * of the following values: 00764 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00765 * @param PinState: The new IO pin state. 00766 * @retval None 00767 */ 00768 void mfxstm32l152_IO_WritePin(uint16_t DeviceAddr, uint32_t IO_Pin, uint8_t PinState) 00769 { 00770 /* Apply the bit value to the selected pin */ 00771 if (PinState != 0) 00772 { 00773 /* Set the SET register */ 00774 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPO_SET1, IO_Pin, 1); 00775 } 00776 else 00777 { 00778 /* Set the CLEAR register */ 00779 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_GPO_CLR1, IO_Pin, 1); 00780 } 00781 } 00782 00783 /** 00784 * @brief Return the state of the selected IO pin(s). 00785 * @param DeviceAddr: Device address on communication Bus. 00786 * @param IO_Pin: The output pin to be set or reset. This parameter can be one 00787 * of the following values: 00788 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00789 * @retval IO pin(s) state. 00790 */ 00791 uint32_t mfxstm32l152_IO_ReadPin(uint16_t DeviceAddr, uint32_t IO_Pin) 00792 { 00793 uint32_t tmp1 = 0; 00794 uint32_t tmp2 = 0; 00795 uint32_t tmp3 = 0; 00796 00797 if(IO_Pin & 0x000000FF) 00798 { 00799 tmp1 = (uint32_t) MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_STATE1); 00800 } 00801 if(IO_Pin & 0x0000FF00) 00802 { 00803 tmp2 = (uint32_t) MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_STATE2); 00804 } 00805 if(IO_Pin & 0x00FF0000) 00806 { 00807 tmp3 = (uint32_t) MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_GPIO_STATE3); 00808 } 00809 00810 tmp3 = tmp1 + (tmp2 << 8) + (tmp3 << 16); 00811 00812 return(tmp3 & IO_Pin); 00813 } 00814 00815 /** 00816 * @brief Enable the global IO interrupt source. 00817 * @param DeviceAddr: Device address on communication Bus. 00818 * @retval None 00819 */ 00820 void mfxstm32l152_IO_EnableIT(uint16_t DeviceAddr) 00821 { 00822 MFX_IO_ITConfig(); 00823 00824 /* Enable global IO IT source */ 00825 mfxstm32l152_EnableITSource(DeviceAddr, MFXSTM32L152_IRQ_GPIO); 00826 } 00827 00828 /** 00829 * @brief Disable the global IO interrupt source. 00830 * @param DeviceAddr: Device address on communication Bus. 00831 * @retval None 00832 */ 00833 void mfxstm32l152_IO_DisableIT(uint16_t DeviceAddr) 00834 { 00835 /* Disable global IO IT source */ 00836 mfxstm32l152_DisableITSource(DeviceAddr, MFXSTM32L152_IRQ_GPIO); 00837 } 00838 00839 /** 00840 * @brief Enable interrupt mode for the selected IO pin(s). 00841 * @param DeviceAddr: Device address on communication Bus. 00842 * @param IO_Pin: The IO interrupt to be enabled. This parameter could be any 00843 * combination of the following values: 00844 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00845 * @retval None 00846 */ 00847 void mfxstm32l152_IO_EnablePinIT(uint16_t DeviceAddr, uint32_t IO_Pin) 00848 { 00849 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_SRC1, IO_Pin, 1); 00850 } 00851 00852 /** 00853 * @brief Disable interrupt mode for the selected IO pin(s). 00854 * @param DeviceAddr: Device address on communication Bus. 00855 * @param IO_Pin: The IO interrupt to be disabled. This parameter could be any 00856 * combination of the following values: 00857 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00858 * @retval None 00859 */ 00860 void mfxstm32l152_IO_DisablePinIT(uint16_t DeviceAddr, uint32_t IO_Pin) 00861 { 00862 mfxstm32l152_reg24_setPinValue(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_SRC1, IO_Pin, 0); 00863 } 00864 00865 00866 /** 00867 * @brief Check the status of the selected IO interrupt pending bit 00868 * @param DeviceAddr: Device address on communication Bus. 00869 * @param IO_Pin: The IO interrupt to be checked could be: 00870 * @arg MFXSTM32L152_GPIO_PIN_x Where x can be from 0 to 23. 00871 * @retval Status of the checked IO pin(s). 00872 */ 00873 uint32_t mfxstm32l152_IO_ITStatus(uint16_t DeviceAddr, uint32_t IO_Pin) 00874 { 00875 /* Get the Interrupt status */ 00876 uint8_t tmp1 = 0; 00877 uint16_t tmp2 = 0; 00878 uint32_t tmp3 = 0; 00879 00880 if(IO_Pin & 0xFF) 00881 { 00882 tmp1 = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_PENDING1); 00883 } 00884 if(IO_Pin & 0xFFFF00) 00885 { 00886 tmp2 = (uint16_t) MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_PENDING2); 00887 } 00888 if(IO_Pin & 0xFFFF0000) 00889 { 00890 tmp3 = (uint32_t) MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_PENDING3); 00891 } 00892 00893 tmp3 = tmp1 + (tmp2 << 8) + (tmp3 << 16); 00894 00895 return(tmp3 & IO_Pin); 00896 } 00897 00898 /** 00899 * @brief Clear the selected IO interrupt pending bit(s). It clear automatically also the general MFXSTM32L152_REG_ADR_IRQ_PENDING 00900 * @param DeviceAddr: Device address on communication Bus. 00901 * @param IO_Pin: the IO interrupt to be cleared, could be: 00902 * @arg MFXSTM32L152_GPIO_PIN_x: Where x can be from 0 to 23. 00903 * @retval None 00904 */ 00905 void mfxstm32l152_IO_ClearIT(uint16_t DeviceAddr, uint32_t IO_Pin) 00906 { 00907 /* Clear the IO IT pending bit(s) by acknowledging */ 00908 /* it cleans automatically also the Global IRQ_GPIO */ 00909 /* normally this function is called under interrupt */ 00910 uint8_t pin_0_7, pin_8_15, pin_16_23; 00911 00912 pin_0_7 = IO_Pin & 0x0000ff; 00913 pin_8_15 = IO_Pin >> 8; 00914 pin_8_15 = pin_8_15 & 0x00ff; 00915 pin_16_23 = IO_Pin >> 16; 00916 00917 if (pin_0_7) 00918 { 00919 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_ACK1, pin_0_7); 00920 } 00921 if (pin_8_15) 00922 { 00923 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_ACK2, pin_8_15); 00924 } 00925 if (pin_16_23) 00926 { 00927 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_IRQ_GPI_ACK3, pin_16_23); 00928 } 00929 } 00930 00931 00932 /** 00933 * @brief Enable the AF for aGPIO. 00934 * @param DeviceAddr: Device address on communication Bus. 00935 * @retval None 00936 */ 00937 void mfxstm32l152_IO_EnableAF(uint16_t DeviceAddr) 00938 { 00939 uint8_t mode; 00940 00941 /* Get the current register value */ 00942 mode = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL); 00943 00944 /* Enable ALTERNATE functions */ 00945 /* AGPIO[0..3] can be either IDD or GPIO */ 00946 /* AGPIO[4..7] can be either TS or GPIO */ 00947 /* if IDD or TS are enabled no matter the value this bit GPIO are not available for those pins */ 00948 /* however the MFX will waste some cycles to to handle these potential GPIO (pooling, etc) */ 00949 /* so if IDD and TS are both active it is better to let ALTERNATE disabled (0) */ 00950 /* if however IDD or TS are not connected then set it on gives more GPIOs availability */ 00951 /* remind that AGPIO are less efficient then normal GPIO (they use pooling rather then EXTI) */ 00952 mode |= MFXSTM32L152_ALTERNATE_GPIO_EN; 00953 00954 /* Write the new register value */ 00955 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, mode); 00956 } 00957 00958 /** 00959 * @brief Disable the AF for aGPIO. 00960 * @param DeviceAddr: Device address on communication Bus. 00961 * @retval None 00962 */ 00963 void mfxstm32l152_IO_DisableAF(uint16_t DeviceAddr) 00964 { 00965 uint8_t mode; 00966 00967 /* Get the current register value */ 00968 mode = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL); 00969 00970 /* Enable ALTERNATE functions */ 00971 /* AGPIO[0..3] can be either IDD or GPIO */ 00972 /* AGPIO[4..7] can be either TS or GPIO */ 00973 /* if IDD or TS are enabled no matter the value this bit GPIO are not available for those pins */ 00974 /* however the MFX will waste some cycles to to handle these potential GPIO (pooling, etc) */ 00975 /* so if IDD and TS are both active it is better to let ALTERNATE disabled (0) */ 00976 /* if however IDD or TS are not connected then set it on gives more GPIOs availability */ 00977 /* remind that AGPIO are less efficient then normal GPIO (they use pooling rather then EXTI) */ 00978 mode &= ~MFXSTM32L152_ALTERNATE_GPIO_EN; 00979 00980 /* Write the new register value */ 00981 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, mode); 00982 00983 } 00984 00985 00986 /* ------------------------------------------------------------------ */ 00987 /* --------------------- TOUCH SCREEN ------------------------------- */ 00988 /* ------------------------------------------------------------------ */ 00989 00990 /** 00991 * @brief Configures the touch Screen Controller (Single point detection) 00992 * @param DeviceAddr: Device address on communication Bus. 00993 * @retval None. 00994 */ 00995 void mfxstm32l152_TS_Start(uint16_t DeviceAddr) 00996 { 00997 uint8_t mode; 00998 00999 /* Get the current register value */ 01000 mode = MFX_IO_Read(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL); 01001 01002 /* Set the Functionalities to be Enabled */ 01003 mode |= MFXSTM32L152_TS_EN; 01004 01005 /* Set the new register value */ 01006 MFX_IO_Write(DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, mode); 01007 01008 /* Wait for 2 ms */ 01009 MFX_IO_Delay(2); 01010 01011 /* Select 2 nF filter capacitor */ 01012 /* Configuration: 01013 - Touch average control : 4 samples 01014 - Touch delay time : 500 uS 01015 - Panel driver setting time: 500 uS 01016 */ 01017 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_SETTLING, 0x32); 01018 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_TOUCH_DET_DELAY, 0x5); 01019 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_AVE, 0x04); 01020 01021 /* Configure the Touch FIFO threshold: single point reading */ 01022 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_FIFO_TH, 0x01); 01023 01024 /* Clear the FIFO memory content. */ 01025 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_FIFO_TH, MFXSTM32L152_TS_CLEAR_FIFO); 01026 01027 /* Touch screen control configuration : 01028 - No window tracking index 01029 */ 01030 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_TRACK, 0x00); 01031 01032 01033 /* Clear all the IT status pending bits if any */ 01034 mfxstm32l152_IO_ClearIT(DeviceAddr, 0xFFFFFF); 01035 01036 /* Wait for 1 ms delay */ 01037 MFX_IO_Delay(1); 01038 } 01039 01040 /** 01041 * @brief Return if there is touch detected or not. 01042 * @param DeviceAddr: Device address on communication Bus. 01043 * @retval Touch detected state. 01044 */ 01045 uint8_t mfxstm32l152_TS_DetectTouch(uint16_t DeviceAddr) 01046 { 01047 uint8_t state; 01048 uint8_t ret = 0; 01049 01050 state = MFX_IO_Read(DeviceAddr, MFXSTM32L152_TS_FIFO_STA); 01051 state = ((state & (uint8_t)MFXSTM32L152_TS_CTRL_STATUS) == (uint8_t)MFXSTM32L152_TS_CTRL_STATUS); 01052 01053 if(state > 0) 01054 { 01055 if(MFX_IO_Read(DeviceAddr, MFXSTM32L152_TS_FIFO_LEVEL) > 0) 01056 { 01057 ret = 1; 01058 } 01059 } 01060 01061 return ret; 01062 } 01063 01064 /** 01065 * @brief Get the touch screen X and Y positions values 01066 * @param DeviceAddr: Device address on communication Bus. 01067 * @param X: Pointer to X position value 01068 * @param Y: Pointer to Y position value 01069 * @retval None. 01070 */ 01071 void mfxstm32l152_TS_GetXY(uint16_t DeviceAddr, uint16_t *X, uint16_t *Y) 01072 { 01073 uint8_t data_xy[3]; 01074 01075 MFX_IO_ReadMultiple(DeviceAddr, MFXSTM32L152_TS_XY_DATA, data_xy, sizeof(data_xy)) ; 01076 01077 /* Calculate positions values */ 01078 *X = (data_xy[1]<<4) + (data_xy[0]>>4); 01079 *Y = (data_xy[2]<<4) + (data_xy[0]&4); 01080 01081 /* Reset the FIFO memory content. */ 01082 MFX_IO_Write(DeviceAddr, MFXSTM32L152_TS_FIFO_TH, MFXSTM32L152_TS_CLEAR_FIFO); 01083 } 01084 01085 /** 01086 * @brief Configure the selected source to generate a global interrupt or not 01087 * @param DeviceAddr: Device address on communication Bus. 01088 * @retval None 01089 */ 01090 void mfxstm32l152_TS_EnableIT(uint16_t DeviceAddr) 01091 { 01092 MFX_IO_ITConfig(); 01093 01094 /* Enable global TS IT source */ 01095 mfxstm32l152_EnableITSource(DeviceAddr, MFXSTM32L152_IRQ_TS_DET); 01096 } 01097 01098 /** 01099 * @brief Configure the selected source to generate a global interrupt or not 01100 * @param DeviceAddr: Device address on communication Bus. 01101 * @retval None 01102 */ 01103 void mfxstm32l152_TS_DisableIT(uint16_t DeviceAddr) 01104 { 01105 /* Disable global TS IT source */ 01106 mfxstm32l152_DisableITSource(DeviceAddr, MFXSTM32L152_IRQ_TS_DET); 01107 } 01108 01109 /** 01110 * @brief Configure the selected source to generate a global interrupt or not 01111 * @param DeviceAddr: Device address on communication Bus. 01112 * @retval TS interrupts status 01113 */ 01114 uint8_t mfxstm32l152_TS_ITStatus(uint16_t DeviceAddr) 01115 { 01116 /* Return TS interrupts status */ 01117 return(mfxstm32l152_GlobalITStatus(DeviceAddr, MFXSTM32L152_IRQ_TS)); 01118 } 01119 01120 /** 01121 * @brief Configure the selected source to generate a global interrupt or not 01122 * @param DeviceAddr: Device address on communication Bus. 01123 * @retval None 01124 */ 01125 void mfxstm32l152_TS_ClearIT(uint16_t DeviceAddr) 01126 { 01127 /* Clear the global TS IT source */ 01128 mfxstm32l152_ClearGlobalIT(DeviceAddr, MFXSTM32L152_IRQ_TS); 01129 } 01130 01131 /* ------------------------------------------------------------------ */ 01132 /* --------------------- IDD MEASUREMENT ---------------------------- */ 01133 /* ------------------------------------------------------------------ */ 01134 01135 /** 01136 * @brief Launch IDD current measurement 01137 * @param DeviceAddr: Device address on communication Bus 01138 * @retval None. 01139 */ 01140 void mfxstm32l152_IDD_Start(uint16_t DeviceAddr) 01141 { 01142 uint8_t mode = 0; 01143 01144 /* Get the current register value */ 01145 mode = MFX_IO_Read((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_CTRL); 01146 01147 /* Set the Functionalities to be enabled */ 01148 mode |= MFXSTM32L152_IDD_CTRL_REQ; 01149 01150 /* Start measurement campaign */ 01151 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_CTRL, mode); 01152 } 01153 01154 /** 01155 * @brief Configures the IDD current measurement 01156 * @param DeviceAddr: Device address on communication Bus. 01157 * @param MfxIddConfig: Parameters depending on hardware config. 01158 * @retval None 01159 */ 01160 void mfxstm32l152_IDD_Config(uint16_t DeviceAddr, IDD_ConfigTypeDef MfxIddConfig) 01161 { 01162 uint8_t value = 0; 01163 uint8_t mode = 0; 01164 01165 /* Get the current register value */ 01166 mode = MFX_IO_Read((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL); 01167 01168 if((mode & MFXSTM32L152_IDD_EN) != MFXSTM32L152_IDD_EN) 01169 { 01170 /* Set the Functionalities to be enabled */ 01171 mode |= MFXSTM32L152_IDD_EN; 01172 01173 /* Set the new register value */ 01174 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_SYS_CTRL, mode); 01175 } 01176 01177 /* Control register setting: number of shunts */ 01178 value = ((MfxIddConfig.ShuntNbUsed << 1) & MFXSTM32L152_IDD_CTRL_SHUNT_NB); 01179 value |= (MfxIddConfig.VrefMeasurement & MFXSTM32L152_IDD_CTRL_VREF_DIS); 01180 value |= (MfxIddConfig.Calibration & MFXSTM32L152_IDD_CTRL_CAL_DIS); 01181 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_CTRL, value); 01182 01183 /* Idd pre delay configuration: unit and value*/ 01184 value = (MfxIddConfig.PreDelayUnit & MFXSTM32L152_IDD_PREDELAY_UNIT) | 01185 (MfxIddConfig.PreDelayValue & MFXSTM32L152_IDD_PREDELAY_VALUE); 01186 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_PRE_DELAY, value); 01187 01188 /* Shunt 0 register value: MSB then LSB */ 01189 value = (uint8_t) (MfxIddConfig.Shunt0Value >> 8); 01190 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT0_MSB, value); 01191 value = (uint8_t) (MfxIddConfig.Shunt0Value); 01192 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT0_LSB, value); 01193 01194 /* Shunt 1 register value: MSB then LSB */ 01195 value = (uint8_t) (MfxIddConfig.Shunt1Value >> 8); 01196 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT1_MSB, value); 01197 value = (uint8_t) (MfxIddConfig.Shunt1Value); 01198 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT1_LSB, value); 01199 01200 /* Shunt 2 register value: MSB then LSB */ 01201 value = (uint8_t) (MfxIddConfig.Shunt2Value >> 8); 01202 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT2_MSB, value); 01203 value = (uint8_t) (MfxIddConfig.Shunt2Value); 01204 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT2_LSB, value); 01205 01206 /* Shunt 3 register value: MSB then LSB */ 01207 value = (uint8_t) (MfxIddConfig.Shunt3Value >> 8); 01208 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT3_MSB, value); 01209 value = (uint8_t) (MfxIddConfig.Shunt3Value); 01210 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT3_LSB, value); 01211 01212 /* Shunt 4 register value: MSB then LSB */ 01213 value = (uint8_t) (MfxIddConfig.Shunt4Value >> 8); 01214 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT4_MSB, value); 01215 value = (uint8_t) (MfxIddConfig.Shunt4Value); 01216 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT4_LSB, value); 01217 01218 /* Shunt 0 stabilization delay */ 01219 value = MfxIddConfig.Shunt0StabDelay; 01220 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SH0_STABILIZATION, value); 01221 01222 /* Shunt 1 stabilization delay */ 01223 value = MfxIddConfig.Shunt1StabDelay; 01224 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SH1_STABILIZATION, value); 01225 01226 /* Shunt 2 stabilization delay */ 01227 value = MfxIddConfig.Shunt2StabDelay; 01228 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SH2_STABILIZATION, value); 01229 01230 /* Shunt 3 stabilization delay */ 01231 value = MfxIddConfig.Shunt3StabDelay; 01232 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SH3_STABILIZATION, value); 01233 01234 /* Shunt 4 stabilization delay */ 01235 value = MfxIddConfig.Shunt4StabDelay; 01236 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SH4_STABILIZATION, value); 01237 01238 /* Idd ampli gain value: MSB then LSB */ 01239 value = (uint8_t) (MfxIddConfig.AmpliGain >> 8); 01240 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_GAIN_MSB, value); 01241 value = (uint8_t) (MfxIddConfig.AmpliGain); 01242 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_GAIN_LSB, value); 01243 01244 /* Idd VDD min value: MSB then LSB */ 01245 value = (uint8_t) (MfxIddConfig.VddMin >> 8); 01246 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_VDD_MIN_MSB, value); 01247 value = (uint8_t) (MfxIddConfig.VddMin); 01248 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_VDD_MIN_LSB, value); 01249 01250 /* Idd number of measurements */ 01251 value = MfxIddConfig.MeasureNb; 01252 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_NBR_OF_MEAS, value); 01253 01254 /* Idd delta delay configuration: unit and value */ 01255 value = (MfxIddConfig.DeltaDelayUnit & MFXSTM32L152_IDD_DELTADELAY_UNIT) | 01256 (MfxIddConfig.DeltaDelayValue & MFXSTM32L152_IDD_DELTADELAY_VALUE); 01257 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_MEAS_DELTA_DELAY, value); 01258 01259 /* Idd number of shut on board */ 01260 value = MfxIddConfig.ShuntNbOnBoard; 01261 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNTS_ON_BOARD, value); 01262 } 01263 01264 /** 01265 * @brief This function allows to modify number of shunt used for a measurement 01266 * @param DeviceAddr: Device address on communication Bus 01267 * @retval None. 01268 */ 01269 void mfxstm32l152_IDD_ConfigShuntNbLimit(uint16_t DeviceAddr, uint8_t ShuntNbLimit) 01270 { 01271 uint8_t mode = 0; 01272 01273 /* Get the current register value */ 01274 mode = MFX_IO_Read((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_CTRL); 01275 01276 /* Clear number of shunt limit */ 01277 mode &= ~(MFXSTM32L152_IDD_CTRL_SHUNT_NB); 01278 01279 /* Clear number of shunt limit */ 01280 mode |= ((ShuntNbLimit << 1) & MFXSTM32L152_IDD_CTRL_SHUNT_NB); 01281 01282 /* Write noewx desired limit */ 01283 MFX_IO_Write((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_CTRL, mode); 01284 } 01285 01286 /** 01287 * @brief Get Idd current value 01288 * @param DeviceAddr: Device address on communication Bus 01289 * @param ReadValue: Pointer on value to be read 01290 * @retval Idd value in 10 nA. 01291 */ 01292 void mfxstm32l152_IDD_GetValue(uint16_t DeviceAddr, uint32_t *ReadValue) 01293 { 01294 uint8_t data[3]; 01295 01296 MFX_IO_ReadMultiple((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_VALUE_MSB, data, sizeof(data)) ; 01297 01298 /* Recompose Idd current value */ 01299 *ReadValue = (data[0] << 16) | (data[1] << 8) | data[2]; 01300 01301 } 01302 01303 /** 01304 * @brief Get Last shunt used for measurement 01305 * @param DeviceAddr: Device address on communication Bus 01306 * @retval Last shunt used 01307 */ 01308 uint8_t mfxstm32l152_IDD_GetShuntUsed(uint16_t DeviceAddr) 01309 { 01310 return(MFX_IO_Read((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_IDD_SHUNT_USED)); 01311 } 01312 01313 /** 01314 * @brief Configure mfx to enable Idd interrupt 01315 * @param DeviceAddr: Device address on communication Bus. 01316 * @retval None 01317 */ 01318 void mfxstm32l152_IDD_EnableIT(uint16_t DeviceAddr) 01319 { 01320 MFX_IO_ITConfig(); 01321 01322 /* Enable global IDD interrupt source */ 01323 mfxstm32l152_EnableITSource(DeviceAddr, MFXSTM32L152_IRQ_IDD); 01324 } 01325 01326 /** 01327 * @brief Clear Idd global interrupt 01328 * @param DeviceAddr: Device address on communication Bus. 01329 * @retval None 01330 */ 01331 void mfxstm32l152_IDD_ClearIT(uint16_t DeviceAddr) 01332 { 01333 /* Clear the global IDD interrupt source */ 01334 mfxstm32l152_ClearGlobalIT(DeviceAddr, MFXSTM32L152_IRQ_IDD); 01335 } 01336 01337 /** 01338 * @brief get Idd interrupt status 01339 * @param DeviceAddr: Device address on communication Bus. 01340 * @retval IDD interrupts status 01341 */ 01342 uint8_t mfxstm32l152_IDD_GetITStatus(uint16_t DeviceAddr) 01343 { 01344 /* Return IDD interrupt status */ 01345 return(mfxstm32l152_GlobalITStatus(DeviceAddr, MFXSTM32L152_IRQ_IDD)); 01346 } 01347 01348 /** 01349 * @brief disable Idd interrupt 01350 * @param DeviceAddr: Device address on communication Bus. 01351 * @retval None. 01352 */ 01353 void mfxstm32l152_IDD_DisableIT(uint16_t DeviceAddr) 01354 { 01355 /* Disable global IDD interrupt source */ 01356 mfxstm32l152_DisableITSource(DeviceAddr, MFXSTM32L152_IRQ_IDD); 01357 } 01358 01359 01360 /* ------------------------------------------------------------------ */ 01361 /* --------------------- ERROR MANAGEMENT --------------------------- */ 01362 /* ------------------------------------------------------------------ */ 01363 01364 /** 01365 * @brief Read Error Source. 01366 * @param DeviceAddr: Device address on communication Bus. 01367 * @retval Error message code with error source 01368 */ 01369 uint8_t mfxstm32l152_Error_ReadSrc(uint16_t DeviceAddr) 01370 { 01371 /* Get the current source register value */ 01372 return(MFX_IO_Read((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_ERROR_SRC)); 01373 } 01374 01375 /** 01376 * @brief Read Error Message 01377 * @param DeviceAddr: Device address on communication Bus. 01378 * @retval Error message code with error source 01379 */ 01380 uint8_t mfxstm32l152_Error_ReadMsg(uint16_t DeviceAddr) 01381 { 01382 /* Get the current message register value */ 01383 return(MFX_IO_Read((uint8_t) DeviceAddr, MFXSTM32L152_REG_ADR_ERROR_MSG)); 01384 } 01385 01386 /** 01387 * @brief Enable Error global interrupt 01388 * @param DeviceAddr: Device address on communication Bus. 01389 * @retval None 01390 */ 01391 01392 void mfxstm32l152_Error_EnableIT(uint16_t DeviceAddr) 01393 { 01394 MFX_IO_ITConfig(); 01395 01396 /* Enable global Error interrupt source */ 01397 mfxstm32l152_EnableITSource(DeviceAddr, MFXSTM32L152_IRQ_ERROR); 01398 } 01399 01400 /** 01401 * @brief Clear Error global interrupt 01402 * @param DeviceAddr: Device address on communication Bus. 01403 * @retval None 01404 */ 01405 void mfxstm32l152_Error_ClearIT(uint16_t DeviceAddr) 01406 { 01407 /* Clear the global Error interrupt source */ 01408 mfxstm32l152_ClearGlobalIT(DeviceAddr, MFXSTM32L152_IRQ_ERROR); 01409 } 01410 01411 /** 01412 * @brief get Error interrupt status 01413 * @param DeviceAddr: Device address on communication Bus. 01414 * @retval Error interrupts status 01415 */ 01416 uint8_t mfxstm32l152_Error_GetITStatus(uint16_t DeviceAddr) 01417 { 01418 /* Return Error interrupt status */ 01419 return(mfxstm32l152_GlobalITStatus(DeviceAddr, MFXSTM32L152_IRQ_ERROR)); 01420 } 01421 01422 /** 01423 * @brief disable Error interrupt 01424 * @param DeviceAddr: Device address on communication Bus. 01425 * @retval None. 01426 */ 01427 void mfxstm32l152_Error_DisableIT(uint16_t DeviceAddr) 01428 { 01429 /* Disable global Error interrupt source */ 01430 mfxstm32l152_DisableITSource(DeviceAddr, MFXSTM32L152_IRQ_ERROR); 01431 } 01432 01433 /** 01434 * @brief FOR DEBUG ONLY 01435 */ 01436 uint8_t mfxstm32l152_ReadReg(uint16_t DeviceAddr, uint8_t RegAddr) 01437 { 01438 /* Get the current register value */ 01439 return(MFX_IO_Read((uint8_t) DeviceAddr, RegAddr)); 01440 } 01441 01442 void mfxstm32l152_WriteReg(uint16_t DeviceAddr, uint8_t RegAddr, uint8_t Value) 01443 { 01444 /* set the current register value */ 01445 MFX_IO_Write((uint8_t) DeviceAddr, RegAddr, Value); 01446 } 01447 01448 /* ------------------------------------------------------------------ */ 01449 /* ----------------------- Private functions ------------------------ */ 01450 /* ------------------------------------------------------------------ */ 01451 /** 01452 * @brief Check if the device instance of the selected address is already registered 01453 * and return its index 01454 * @param DeviceAddr: Device address on communication Bus. 01455 * @retval Index of the device instance if registered, 0xFF if not. 01456 */ 01457 static uint8_t mfxstm32l152_GetInstance(uint16_t DeviceAddr) 01458 { 01459 uint8_t idx = 0; 01460 01461 /* Check all the registered instances */ 01462 for(idx = 0; idx < MFXSTM32L152_MAX_INSTANCE ; idx ++) 01463 { 01464 if(mfxstm32l152[idx] == DeviceAddr) 01465 { 01466 return idx; 01467 } 01468 } 01469 01470 return 0xFF; 01471 } 01472 01473 /** 01474 * @brief Release registered device instance 01475 * @param DeviceAddr: Device address on communication Bus. 01476 * @retval Index of released device instance, 0xFF if not. 01477 */ 01478 static uint8_t mfxstm32l152_ReleaseInstance(uint16_t DeviceAddr) 01479 { 01480 uint8_t idx = 0; 01481 01482 /* Check for all the registered instances */ 01483 for(idx = 0; idx < MFXSTM32L152_MAX_INSTANCE ; idx ++) 01484 { 01485 if(mfxstm32l152[idx] == DeviceAddr) 01486 { 01487 mfxstm32l152[idx] = 0; 01488 return idx; 01489 } 01490 } 01491 return 0xFF; 01492 } 01493 01494 /** 01495 * @brief Internal routine 01496 * @param DeviceAddr: Device address on communication Bus. 01497 * @param RegisterAddr: Register Address 01498 * @param PinPosition: Pin [0:23] 01499 * @param PinValue: 0/1 01500 * @retval None 01501 */ 01502 void mfxstm32l152_reg24_setPinValue(uint16_t DeviceAddr, uint8_t RegisterAddr, uint32_t PinPosition, uint8_t PinValue ) 01503 { 01504 uint8_t tmp = 0; 01505 uint8_t pin_0_7, pin_8_15, pin_16_23; 01506 01507 pin_0_7 = PinPosition & 0x0000ff; 01508 pin_8_15 = PinPosition >> 8; 01509 pin_8_15 = pin_8_15 & 0x00ff; 01510 pin_16_23 = PinPosition >> 16; 01511 01512 if (pin_0_7) 01513 { 01514 /* Get the current register value */ 01515 tmp = MFX_IO_Read(DeviceAddr, RegisterAddr); 01516 01517 /* Set the selected pin direction */ 01518 if (PinValue != 0) 01519 { 01520 tmp |= (uint8_t)pin_0_7; 01521 } 01522 else 01523 { 01524 tmp &= ~(uint8_t)pin_0_7; 01525 } 01526 01527 /* Set the new register value */ 01528 MFX_IO_Write(DeviceAddr, RegisterAddr, tmp); 01529 } 01530 01531 if (pin_8_15) 01532 { 01533 /* Get the current register value */ 01534 tmp = MFX_IO_Read(DeviceAddr, RegisterAddr+1); 01535 01536 /* Set the selected pin direction */ 01537 if (PinValue != 0) 01538 { 01539 tmp |= (uint8_t)pin_8_15; 01540 } 01541 else 01542 { 01543 tmp &= ~(uint8_t)pin_8_15; 01544 } 01545 01546 /* Set the new register value */ 01547 MFX_IO_Write(DeviceAddr, RegisterAddr+1, tmp); 01548 } 01549 01550 if (pin_16_23) 01551 { 01552 /* Get the current register value */ 01553 tmp = MFX_IO_Read(DeviceAddr, RegisterAddr+2); 01554 01555 /* Set the selected pin direction */ 01556 if (PinValue != 0) 01557 { 01558 tmp |= (uint8_t)pin_16_23; 01559 } 01560 else 01561 { 01562 tmp &= ~(uint8_t)pin_16_23; 01563 } 01564 01565 /* Set the new register value */ 01566 MFX_IO_Write(DeviceAddr, RegisterAddr+2, tmp); 01567 } 01568 } 01569 01570 01571 /** 01572 * @} 01573 */ 01574 01575 /** 01576 * @} 01577 */ 01578 01579 /** 01580 * @} 01581 */ 01582 01583 /** 01584 * @} 01585 */ 01586 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 18:37:21 by
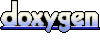