Updates to follow mbed SDK coding style guidelines.
Dependencies: ST_INTERFACES X_NUCLEO_COMMON
Dependents: 53L0A1_Satellites_with_Interrupts_OS5 Display_53L0A1_OS5
Fork of X_NUCLEO_53L0A1 by
vl53l0x_platform_log.h
00001 /******************************************************************************* 00002 Copyright © 2015, STMicroelectronics International N.V. 00003 All rights reserved. 00004 00005 Redistribution and use in source and binary forms, with or without 00006 modification, are permitted provided that the following conditions are met: 00007 * Redistributions of source code must retain the above copyright 00008 notice, this list of conditions and the following disclaimer. 00009 * Redistributions in binary form must reproduce the above copyright 00010 notice, this list of conditions and the following disclaimer in the 00011 documentation and/or other materials provided with the distribution. 00012 * Neither the name of STMicroelectronics nor the 00013 names of its contributors may be used to endorse or promote products 00014 derived from this software without specific prior written permission. 00015 00016 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00017 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, AND 00019 NON-INFRINGEMENT OF INTELLECTUAL PROPERTY RIGHTS ARE DISCLAIMED. 00020 IN NO EVENT SHALL STMICROELECTRONICS INTERNATIONAL N.V. BE LIABLE FOR ANY 00021 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00022 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00023 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00024 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00025 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00026 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00027 ********************************************************************************/ 00028 00029 00030 #ifndef _VL53L0X_PLATFORM_LOG_H_ 00031 #define _VL53L0X_PLATFORM_LOG_H_ 00032 00033 #include <stdio.h> 00034 #include <string.h> 00035 /* LOG Functions */ 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 /** 00042 * @file vl53l0_platform_log.h 00043 * 00044 * @brief platform log function definition 00045 */ 00046 00047 //#define VL53L0X_LOG_ENABLE 0 00048 00049 enum { 00050 TRACE_LEVEL_NONE, 00051 TRACE_LEVEL_ERRORS, 00052 TRACE_LEVEL_WARNING, 00053 TRACE_LEVEL_INFO, 00054 TRACE_LEVEL_DEBUG, 00055 TRACE_LEVEL_ALL, 00056 TRACE_LEVEL_IGNORE 00057 }; 00058 00059 enum { 00060 TRACE_FUNCTION_NONE = 0, 00061 TRACE_FUNCTION_I2C = 1, 00062 TRACE_FUNCTION_ALL = 0x7fffffff //all bits except sign 00063 }; 00064 00065 enum { 00066 TRACE_MODULE_NONE = 0x0, 00067 TRACE_MODULE_API = 0x1, 00068 TRACE_MODULE_PLATFORM = 0x2, 00069 TRACE_MODULE_ALL = 0x7fffffff //all bits except sign 00070 }; 00071 00072 00073 #ifdef VL53L0X_LOG_ENABLE 00074 00075 #include <sys/time.h> 00076 00077 extern uint32_t _trace_level; 00078 00079 00080 00081 int32_t VL53L0X_trace_config(char *filename, uint32_t modules, uint32_t level, uint32_t functions); 00082 00083 void trace_print_module_function(uint32_t module, uint32_t level, uint32_t function, const char *format, ...); 00084 00085 00086 //extern FILE * log_file; 00087 00088 #define LOG_GET_TIME() (int)clock() 00089 00090 #define _LOG_FUNCTION_START(module, fmt, ... ) \ 00091 trace_print_module_function(module, _trace_level, TRACE_FUNCTION_ALL, "%ld <START> %s "fmt"\n", LOG_GET_TIME(), __FUNCTION__, ##__VA_ARGS__); 00092 00093 #define _LOG_FUNCTION_END(module, status, ... )\ 00094 trace_print_module_function(module, _trace_level, TRACE_FUNCTION_ALL, "%ld <END> %s %d\n", LOG_GET_TIME(), __FUNCTION__, (int)status, ##__VA_ARGS__) 00095 00096 #define _LOG_FUNCTION_END_FMT(module, status, fmt, ... )\ 00097 trace_print_module_function(module, _trace_level, TRACE_FUNCTION_ALL, "%ld <END> %s %d "fmt"\n", LOG_GET_TIME(), __FUNCTION__, (int)status,##__VA_ARGS__) 00098 00099 // __func__ is gcc only 00100 #define VL53L0X_ErrLog( fmt, ...) fprintf(stderr, "VL53L0X_ErrLog %s" fmt "\n", __func__, ##__VA_ARGS__) 00101 00102 #else /* VL53L0X_LOG_ENABLE no logging */ 00103 #define VL53L0X_ErrLog(...) (void)0 00104 #define _LOG_FUNCTION_START(module, fmt, ... ) (void)0 00105 #define _LOG_FUNCTION_END(module, status, ... ) (void)0 00106 #define _LOG_FUNCTION_END_FMT(module, status, fmt, ... ) (void)0 00107 #endif /* else */ 00108 00109 #define VL53L0X_COPYSTRING(str, ...) strcpy(str, ##__VA_ARGS__) 00110 00111 #ifdef __cplusplus 00112 } 00113 #endif 00114 00115 #endif /* _VL53L0X_PLATFORM_LOG_H_ */ 00116 00117 00118 00119
Generated on Wed Jul 13 2022 19:35:41 by
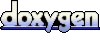