Speed profile working
Fork of Easyspin_lib by
Easyspin Class Reference
Easyspin library class. More...
#include <easyspin.h>
Public Member Functions | |
Easyspin () | |
Constructor. | |
void | AttachFlagInterrupt (void(*callback)(void)) |
Attaches a user callback to the flag Interrupt The call back will be then called each time the status flag pin will be pulled down due to the occurrence of a programmed alarms ( OCD, thermal pre-warning or shutdown, UVLO, wrong command, non-performable command) | |
void | Begin (uint8_t nbShields) |
Starts the Easyspin library. | |
uint16_t | GetAcceleration (uint8_t shieldId) |
Returns the acceleration of the specified shield. | |
uint16_t | GetCurrentSpeed (uint8_t shieldId) |
Returns the current speed of the specified shield. | |
uint16_t | GetDeceleration (uint8_t shieldId) |
Returns the deceleration of the specified shield. | |
shieldState_t | GetShieldState (uint8_t shieldId) |
Returns the shield state. | |
uint8_t | GetFwVersion (void) |
Returns the FW version of the library. | |
int32_t | GetMark (uint8_t shieldId) |
Returns the mark position of the specified shield. | |
uint16_t | GetMaxSpeed (uint8_t shieldId) |
Returns the max speed of the specified shield. | |
uint16_t | GetMinSpeed (uint8_t shieldId) |
Returns the min speed of the specified shield. | |
int32_t | GetPosition (uint8_t shieldId) |
Returns the ABS_POSITION of the specified shield. | |
void | GoHome (uint8_t shieldId) |
Requests the motor to move to the home position (ABS_POSITION = 0) | |
void | GoMark (uint8_t shieldId) |
Requests the motor to move to the mark position. | |
void | GoTo (uint8_t shieldId, int32_t targetPosition) |
Requests the motor to move to the specified position. | |
void | HardStop (uint8_t shieldId) |
Immediatly stops the motor and disable the power bridge. | |
void | Move (uint8_t shieldId, dir_t direction, uint32_t stepCount) |
Moves the motor of the specified number of steps. | |
void | ResetAllShields (void) |
Resets all Easyspin shields. | |
void | Run (uint8_t shieldId, dir_t direction) |
Runs the motor. | |
bool | SetAcceleration (uint8_t shieldId, uint16_t newAcc) |
Changes the acceleration of the specified shield. | |
bool | SetDeceleration (uint8_t shieldId, uint16_t newDec) |
Changes the deceleration of the specified shield. | |
void | SetHome (uint8_t shieldId) |
Set current position to be the Home position (ABS pos set to 0) | |
void | SetMark (uint8_t shieldId) |
Sets current position to be the Mark position. | |
bool | SetMaxSpeed (uint8_t shieldId, uint16_t newMaxSpeed) |
Changes the max speed of the specified shield. | |
bool | SetMinSpeed (uint8_t shieldId, uint16_t newMinSpeed) |
Changes the min speed of the specified shield. | |
bool | SoftStop (uint8_t shieldId) |
Stops the motor by using the shield deceleration. | |
void | WaitWhileActive (uint8_t shieldId) |
Locks until the shield state becomes Inactive. | |
void | Turn (rot_t rotation, uint16_t angle) |
Turn left or right with the specified angle. | |
void | Move_cm (dir_t direction, uint16_t distance) |
Move forward or backward with the specified distance. | |
void | CmdDisable (uint8_t shieldId) |
Issue the Disable command to the Easyspin of the specified shield. | |
void | CmdEnable (uint8_t shieldId) |
Issues the Enable command to the Easyspin of the specified shield. | |
uint32_t | CmdGetParam (uint8_t shieldId, Easyspin_Registers_t param) |
Issues the GetParam command to the Easyspin of the specified shield. | |
uint16_t | CmdGetStatus (uint8_t shieldId) |
Issues the GetStatus command to the Easyspin of the specified shield. | |
void | CmdNop (uint8_t shieldId) |
Issues the Nop command to the Easyspin of the specified shield. | |
void | CmdSetParam (uint8_t shieldId, Easyspin_Registers_t param, uint32_t value) |
Issues the SetParam command to the Easyspin of the specified shield. | |
uint16_t | ReadStatusRegister (uint8_t shieldId) |
Reads the Status Register value. | |
void | Reset (void) |
Resets the Easyspin (reset pin set to low) of all shields. | |
void | ReleaseReset (void) |
Releases the Easyspin reset (pin set to High) of all shields. | |
void | SelectStepMode (uint8_t shieldId, Easyspin_STEP_SEL_t stepMod) |
Set the stepping mode. | |
void | SetDirection (uint8_t shieldId, dir_t direction) |
Specifies the direction. | |
void | StepClockHandler (uint8_t shieldId) |
Handles the shield state machine at each ste. | |
Static Public Member Functions | |
static class Easyspin * | GetInstancePtr (void) |
Gets the pointer to the Easyspin instance. |
Detailed Description
Easyspin library class.
Definition at line 409 of file easyspin.h.
Constructor & Destructor Documentation
Easyspin | ( | ) |
Generated on Tue Jul 12 2022 21:33:48 by
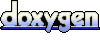