Speed profile working
Fork of Easyspin_lib by
Embed:
(wiki syntax)
Show/hide line numbers
easyspin.h
Go to the documentation of this file.
00001 /******************************************************//** 00002 * @file easyspin.h 00003 * @version V1.0 00004 * @date June 29, 2015 00005 * @brief Header for easyspin library for mbed 00006 * 00007 * This file is free software; you can redistribute it and/or modify 00008 * it under the terms of either the GNU General Public License version 2 00009 * or the GNU Lesser General Public License version 2.1, both as 00010 * published by the Free Software Foundation. 00011 **********************************************************/ 00012 00013 #ifndef __Easyspin_H_INCLUDED 00014 #define __Easyspin_H_INCLUDED 00015 00016 #include "easyspin_config.h" 00017 #include "mbed.h" 00018 00019 /// Define to print debug logs via the UART 00020 #ifndef _DEBUG_Easyspin 00021 //#define _DEBUG_Easyspin 00022 #endif 00023 00024 00025 /// Clear bit Macro 00026 #ifndef cbi 00027 #define cbi(sfr, bit) (_SFR_BYTE(sfr) &= ~_BV(bit)) 00028 #endif 00029 00030 /// Set bit Macro 00031 #ifndef sbi 00032 #define sbi(sfr, bit) (_SFR_BYTE(sfr) |= _BV(bit)) 00033 #endif 00034 00035 /// Current FW version 00036 #define Easyspin_FW_VERSION (1) 00037 00038 /// Digital Pins used for the Easyspin MOSI pin 00039 #define Easyspin_MOSI_Pin PA_7 00040 /// Digital Pins used for the Easyspin MISO pin 00041 #define Easyspin_MISO_Pin PA_6 00042 /// Digital Pins used for the Easyspin SCK pin 00043 #define Easyspin_SCK_Pin PA_5 00044 /// Digital Pins used for the Easyspin flag pin 00045 #define Easyspin_FLAG_Pin PA_10 //(2) 00046 /// Digital Pins used for the Easyspin chip select pin 00047 #define Easyspin_CS_Pin PB_6 00048 /// Digital Pins used for the Easyspin step clock pin of shield 0 00049 #define Easyspin_PWM_1_Pin PC_7 //(9) 00050 /// Digital Pins used for the Easyspin step clock pin of shield 1 00051 #define Easyspin_PWM_2_Pin PB_3 //(3) 00052 /// Digital Pins used for the Easyspin step clock pin of shield 2 00053 #define Easyspin_PWM_3_Pin PB_10 //(6) 00054 /// Digital Pins used for the Easyspin direction pin of shield 0 00055 #define Easyspin_DIR_1_Pin PA_8 //(7) 00056 /// Digital Pins used for the Easyspin direction pin of shield 1 00057 #define Easyspin_DIR_2_Pin PB_5 //(4) 00058 /// Digital Pins used for the Easyspin direction pin of shield 2 00059 #define Easyspin_DIR_3_Pin PB_4 //(5) 00060 /// Digital Pins used for the Easyspin reset pin 00061 #define Easyspin_Reset_Pin PA_9 //(8) 00062 /// Maximum number of steps 00063 #define MAX_STEPS (0x7FFFFFFF) 00064 /// uint8_t max value 00065 #define UINT8_MAX (uint8_t)(0XFF) 00066 /// uint16_t max value 00067 #define UINT16_MAX (uint16_t)(0XFFFF) 00068 00069 /// Pwm prescaler array size for timer 0 & 1 00070 #define PRESCALER_ARRAY_TIMER0_1_SIZE (6) 00071 /// Pwm prescaler array size for timer 2 00072 #define PRESCALER_ARRAY_TIMER2_SIZE (8) 00073 00074 /// Maximum frequency of the PWMs 00075 #define Easyspin_MAX_PWM_FREQ (10000) 00076 /// Minimum frequency of the PWMs 00077 #define Easyspin_MIN_PWM_FREQ (30) 00078 00079 /// Easyspin max number of bytes of command & arguments to set a parameter 00080 #define Easyspin_CMD_ARG_MAX_NB_BYTES (4) 00081 00082 /// Easyspin command + argument bytes number for NOP command 00083 #define Easyspin_CMD_ARG_NB_BYTES_NOP (1) 00084 /// Easyspin command + argument bytes number for ENABLE command 00085 #define Easyspin_CMD_ARG_NB_BYTES_ENABLE (1) 00086 /// Easyspin command + argument bytes number for DISABLE command 00087 #define Easyspin_CMD_ARG_NB_BYTES_DISABLE (1) 00088 /// Easyspin command + argument bytes number for GET_STATUS command 00089 #define Easyspin_CMD_ARG_NB_BYTES_GET_STATUS (1) 00090 00091 /// Easyspin response bytes number 00092 #define Easyspin_RSP_NB_BYTES_GET_STATUS (2) 00093 00094 /// Daisy chain command mask 00095 #define DAISY_CHAIN_COMMAND_MASK (0xFA) 00096 00097 /// Easyspin value mask for ABS_POS register 00098 #define Easyspin_ABS_POS_VALUE_MASK ((uint32_t) 0x003FFFFF) 00099 /// Easyspin sign bit mask for ABS_POS register 00100 #define Easyspin_ABS_POS_SIGN_BIT_MASK ((uint32_t) 0x00200000) 00101 00102 /// Easyspin step mask for electrical position (EL_POS) register 00103 #define Easyspin_ELPOS_STEP_MASK ((uint8_t)0xC0) 00104 /// Easyspin microstep mask for electrical position (EL_POS) register 00105 #define Easyspin_ELPOS_MICROSTEP_MASK ((uint8_t)0x3F) 00106 00107 /// Easyspin fast decay time option (TOFF_FAST values for T_FAST register ) 00108 typedef enum { 00109 Easyspin_TOFF_FAST_2us = ((uint8_t) 0x00 << 4), 00110 Easyspin_TOFF_FAST_4us = ((uint8_t) 0x01 << 4), 00111 Easyspin_TOFF_FAST_6us = ((uint8_t) 0x02 << 4), 00112 Easyspin_TOFF_FAST_8us = ((uint8_t) 0x03 << 4), 00113 Easyspin_TOFF_FAST_10us = ((uint8_t) 0x04 << 4), 00114 Easyspin_TOFF_FAST_12us = ((uint8_t) 0x05 << 4), 00115 Easyspin_TOFF_FAST_14us = ((uint8_t) 0x06 << 4), 00116 Easyspin_TOFF_FAST_16us = ((uint8_t) 0x07 << 4), 00117 Easyspin_TOFF_FAST_18us = ((uint8_t) 0x08 << 4), 00118 Easyspin_TOFF_FAST_20us = ((uint8_t) 0x09 << 4), 00119 Easyspin_TOFF_FAST_22us = ((uint8_t) 0x0A << 4), 00120 Easyspin_TOFF_FAST_24us = ((uint8_t) 0x0B << 4), 00121 Easyspin_TOFF_FAST_26us = ((uint8_t) 0x0C << 4), 00122 Easyspin_TOFF_FAST_28us = ((uint8_t) 0x0D << 4), 00123 Easyspin_TOFF_FAST_30us = ((uint8_t) 0x0E << 4), 00124 Easyspin_TOFF_FAST_32us = ((uint8_t) 0x0F << 4) 00125 } Easyspin_TOFF_FAST_t; 00126 00127 /// Easyspin fall step time options (FAST_STEP values for T_FAST register ) 00128 typedef enum { 00129 Easyspin_FAST_STEP_2us = ((uint8_t) 0x00), 00130 Easyspin_FAST_STEP_4us = ((uint8_t) 0x01), 00131 Easyspin_FAST_STEP_6us = ((uint8_t) 0x02), 00132 Easyspin_FAST_STEP_8us = ((uint8_t) 0x03), 00133 Easyspin_FAST_STEP_10us = ((uint8_t) 0x04), 00134 Easyspin_FAST_STEP_12us = ((uint8_t) 0x05), 00135 Easyspin_FAST_STEP_14us = ((uint8_t) 0x06), 00136 Easyspin_FAST_STEP_16us = ((uint8_t) 0x07), 00137 Easyspin_FAST_STEP_18us = ((uint8_t) 0x08), 00138 Easyspin_FAST_STEP_20us = ((uint8_t) 0x09), 00139 Easyspin_FAST_STEP_22us = ((uint8_t) 0x0A), 00140 Easyspin_FAST_STEP_24us = ((uint8_t) 0x0B), 00141 Easyspin_FAST_STEP_26us = ((uint8_t) 0x0C), 00142 Easyspin_FAST_STEP_28us = ((uint8_t) 0x0D), 00143 Easyspin_FAST_STEP_30us = ((uint8_t) 0x0E), 00144 Easyspin_FAST_STEP_32us = ((uint8_t) 0x0F) 00145 } Easyspin_FAST_STEP_t; 00146 00147 /// Easyspin overcurrent threshold options (OCD_TH register) 00148 typedef enum { 00149 Easyspin_OCD_TH_375mA = ((uint8_t) 0x00), 00150 Easyspin_OCD_TH_750mA = ((uint8_t) 0x01), 00151 Easyspin_OCD_TH_1125mA = ((uint8_t) 0x02), 00152 Easyspin_OCD_TH_1500mA = ((uint8_t) 0x03), 00153 Easyspin_OCD_TH_1875mA = ((uint8_t) 0x04), 00154 Easyspin_OCD_TH_2250mA = ((uint8_t) 0x05), 00155 Easyspin_OCD_TH_2625mA = ((uint8_t) 0x06), 00156 Easyspin_OCD_TH_3000mA = ((uint8_t) 0x07), 00157 Easyspin_OCD_TH_3375mA = ((uint8_t) 0x08), 00158 Easyspin_OCD_TH_3750mA = ((uint8_t) 0x09), 00159 Easyspin_OCD_TH_4125mA = ((uint8_t) 0x0A), 00160 Easyspin_OCD_TH_4500mA = ((uint8_t) 0x0B), 00161 Easyspin_OCD_TH_4875mA = ((uint8_t) 0x0C), 00162 Easyspin_OCD_TH_5250mA = ((uint8_t) 0x0D), 00163 Easyspin_OCD_TH_5625mA = ((uint8_t) 0x0E), 00164 Easyspin_OCD_TH_6000mA = ((uint8_t) 0x0F) 00165 } Easyspin_OCD_TH_t; 00166 00167 /// Easyspin STEP_MODE register masks 00168 typedef enum { 00169 Easyspin_STEP_MODE_STEP_SEL = ((uint8_t) 0x07), 00170 Easyspin_STEP_MODE_SYNC_SEL = ((uint8_t) 0x70) 00171 } Easyspin_STEP_MODE_Masks_t; 00172 00173 /// Easyspin STEP_SEL options for STEP_MODE register 00174 typedef enum { 00175 Easyspin_STEP_SEL_1 = ((uint8_t) 0x08), //full step 00176 Easyspin_STEP_SEL_1_2 = ((uint8_t) 0x09), //half step 00177 Easyspin_STEP_SEL_1_4 = ((uint8_t) 0x0A), //1/4 microstep 00178 Easyspin_STEP_SEL_1_8 = ((uint8_t) 0x0B), //1/8 microstep 00179 Easyspin_STEP_SEL_1_16 = ((uint8_t) 0x0C) //1/16 microstep 00180 } Easyspin_STEP_SEL_t; 00181 00182 /// Easyspin SYNC_SEL options for STEP_MODE register 00183 typedef enum { 00184 Easyspin_SYNC_SEL_1_2 = ((uint8_t) 0x80), 00185 Easyspin_SYNC_SEL_1 = ((uint8_t) 0x90), 00186 Easyspin_SYNC_SEL_2 = ((uint8_t) 0xA0), 00187 Easyspin_SYNC_SEL_4 = ((uint8_t) 0xB0), 00188 Easyspin_SYNC_SEL_8 = ((uint8_t) 0xC0), 00189 Easyspin_SYNC_SEL_UNUSED = ((uint8_t) 0xD0) 00190 } Easyspin_SYNC_SEL_t; 00191 00192 /// Easyspin ALARM_EN register options 00193 typedef enum { 00194 Easyspin_ALARM_EN_OVERCURRENT = ((uint8_t) 0x01), 00195 Easyspin_ALARM_EN_THERMAL_SHUTDOWN = ((uint8_t) 0x02), 00196 Easyspin_ALARM_EN_THERMAL_WARNING = ((uint8_t) 0x04), 00197 Easyspin_ALARM_EN_UNDERVOLTAGE = ((uint8_t) 0x08), 00198 Easyspin_ALARM_EN_SW_TURN_ON = ((uint8_t) 0x40), 00199 Easyspin_ALARM_EN_WRONG_NPERF_CMD = ((uint8_t) 0x80) 00200 } Easyspin_ALARM_EN_t; 00201 00202 /// Easyspin CONFIG register masks 00203 typedef enum { 00204 Easyspin_CONFIG_OSC_SEL = ((uint16_t) 0x0007), 00205 Easyspin_CONFIG_EXT_CLK = ((uint16_t) 0x0008), 00206 Easyspin_CONFIG_EN_TQREG = ((uint16_t) 0x0020), 00207 Easyspin_CONFIG_OC_SD = ((uint16_t) 0x0080), 00208 Easyspin_CONFIG_POW_SR = ((uint16_t) 0x0300), 00209 Easyspin_CONFIG_TOFF = ((uint16_t) 0x7C00) 00210 } Easyspin_CONFIG_Masks_t; 00211 00212 /// Easyspin clock source options for CONFIG register 00213 typedef enum { 00214 Easyspin_CONFIG_INT_16MHZ = ((uint16_t) 0x0000), 00215 Easyspin_CONFIG_INT_16MHZ_OSCOUT_2MHZ = ((uint16_t) 0x0008), 00216 Easyspin_CONFIG_INT_16MHZ_OSCOUT_4MHZ = ((uint16_t) 0x0009), 00217 Easyspin_CONFIG_INT_16MHZ_OSCOUT_8MHZ = ((uint16_t) 0x000A), 00218 Easyspin_CONFIG_INT_16MHZ_OSCOUT_16MHZ = ((uint16_t) 0x000B), 00219 Easyspin_CONFIG_EXT_8MHZ_XTAL_DRIVE = ((uint16_t) 0x0004), 00220 Easyspin_CONFIG_EXT_16MHZ_XTAL_DRIVE = ((uint16_t) 0x0005), 00221 Easyspin_CONFIG_EXT_24MHZ_XTAL_DRIVE = ((uint16_t) 0x0006), 00222 Easyspin_CONFIG_EXT_32MHZ_XTAL_DRIVE = ((uint16_t) 0x0007), 00223 Easyspin_CONFIG_EXT_8MHZ_OSCOUT_INVERT = ((uint16_t) 0x000C), 00224 Easyspin_CONFIG_EXT_16MHZ_OSCOUT_INVERT = ((uint16_t) 0x000D), 00225 Easyspin_CONFIG_EXT_24MHZ_OSCOUT_INVERT = ((uint16_t) 0x000E), 00226 Easyspin_CONFIG_EXT_32MHZ_OSCOUT_INVERT = ((uint16_t) 0x000F) 00227 } Easyspin_CONFIG_OSC_MGMT_t; 00228 00229 /// Easyspin external torque regulation options for CONFIG register 00230 typedef enum { 00231 Easyspin_CONFIG_EN_TQREG_TVAL_USED = ((uint16_t) 0x0000), 00232 Easyspin_CONFIG_EN_TQREG_ADC_OUT = ((uint16_t) 0x0020) 00233 } Easyspin_CONFIG_EN_TQREG_t; 00234 00235 /// Easyspin over current shutdown options for CONFIG register 00236 typedef enum { 00237 Easyspin_CONFIG_OC_SD_DISABLE = ((uint16_t) 0x0000), 00238 Easyspin_CONFIG_OC_SD_ENABLE = ((uint16_t) 0x0080) 00239 } Easyspin_CONFIG_OC_SD_t; 00240 00241 /// Easyspin power bridge output slew_rates options (POW_SR values for CONFIG register) 00242 typedef enum { 00243 Easyspin_CONFIG_SR_320V_us =((uint16_t)0x0000), 00244 Easyspin_CONFIG_SR_075V_us =((uint16_t)0x0100), 00245 Easyspin_CONFIG_SR_110V_us =((uint16_t)0x0200), 00246 Easyspin_CONFIG_SR_260V_us =((uint16_t)0x0300) 00247 } Easyspin_CONFIG_POW_SR_t; 00248 00249 /// Easyspin Off time options (TOFF values for CONFIG register) 00250 typedef enum { 00251 Easyspin_CONFIG_TOFF_004us = (((uint16_t) 0x01) << 10), 00252 Easyspin_CONFIG_TOFF_008us = (((uint16_t) 0x02) << 10), 00253 Easyspin_CONFIG_TOFF_012us = (((uint16_t) 0x03) << 10), 00254 Easyspin_CONFIG_TOFF_016us = (((uint16_t) 0x04) << 10), 00255 Easyspin_CONFIG_TOFF_020us = (((uint16_t) 0x05) << 10), 00256 Easyspin_CONFIG_TOFF_024us = (((uint16_t) 0x06) << 10), 00257 Easyspin_CONFIG_TOFF_028us = (((uint16_t) 0x07) << 10), 00258 Easyspin_CONFIG_TOFF_032us = (((uint16_t) 0x08) << 10), 00259 Easyspin_CONFIG_TOFF_036us = (((uint16_t) 0x09) << 10), 00260 Easyspin_CONFIG_TOFF_040us = (((uint16_t) 0x0A) << 10), 00261 Easyspin_CONFIG_TOFF_044us = (((uint16_t) 0x0B) << 10), 00262 Easyspin_CONFIG_TOFF_048us = (((uint16_t) 0x0C) << 10), 00263 Easyspin_CONFIG_TOFF_052us = (((uint16_t) 0x0D) << 10), 00264 Easyspin_CONFIG_TOFF_056us = (((uint16_t) 0x0E) << 10), 00265 Easyspin_CONFIG_TOFF_060us = (((uint16_t) 0x0F) << 10), 00266 Easyspin_CONFIG_TOFF_064us = (((uint16_t) 0x10) << 10), 00267 Easyspin_CONFIG_TOFF_068us = (((uint16_t) 0x11) << 10), 00268 Easyspin_CONFIG_TOFF_072us = (((uint16_t) 0x12) << 10), 00269 Easyspin_CONFIG_TOFF_076us = (((uint16_t) 0x13) << 10), 00270 Easyspin_CONFIG_TOFF_080us = (((uint16_t) 0x14) << 10), 00271 Easyspin_CONFIG_TOFF_084us = (((uint16_t) 0x15) << 10), 00272 Easyspin_CONFIG_TOFF_088us = (((uint16_t) 0x16) << 10), 00273 Easyspin_CONFIG_TOFF_092us = (((uint16_t) 0x17) << 10), 00274 Easyspin_CONFIG_TOFF_096us = (((uint16_t) 0x18) << 10), 00275 Easyspin_CONFIG_TOFF_100us = (((uint16_t) 0x19) << 10), 00276 Easyspin_CONFIG_TOFF_104us = (((uint16_t) 0x1A) << 10), 00277 Easyspin_CONFIG_TOFF_108us = (((uint16_t) 0x1B) << 10), 00278 Easyspin_CONFIG_TOFF_112us = (((uint16_t) 0x1C) << 10), 00279 Easyspin_CONFIG_TOFF_116us = (((uint16_t) 0x1D) << 10), 00280 Easyspin_CONFIG_TOFF_120us = (((uint16_t) 0x1E) << 10), 00281 Easyspin_CONFIG_TOFF_124us = (((uint16_t) 0x1F) << 10) 00282 } Easyspin_CONFIG_TOFF_t; 00283 00284 /// Easyspin STATUS register bit masks 00285 typedef enum { 00286 Easyspin_STATUS_HIZ = (((uint16_t) 0x0001)), 00287 Easyspin_STATUS_DIR = (((uint16_t) 0x0010)), 00288 Easyspin_STATUS_NOTPERF_CMD = (((uint16_t) 0x0080)), 00289 Easyspin_STATUS_WRONG_CMD = (((uint16_t) 0x0100)), 00290 Easyspin_STATUS_UVLO = (((uint16_t) 0x0200)), 00291 Easyspin_STATUS_TH_WRN = (((uint16_t) 0x0400)), 00292 Easyspin_STATUS_TH_SD = (((uint16_t) 0x0800)), 00293 Easyspin_STATUS_OCD = (((uint16_t) 0x1000)) 00294 } Easyspin_STATUS_Masks_t; 00295 00296 /// Easyspin STATUS register options 00297 typedef enum { 00298 Easyspin_STATUS_DIR_FORWARD = (((uint16_t) 0x0001) << 4), 00299 Easyspin_STATUS_DIR_REVERSE = (((uint16_t) 0x0000) << 4) 00300 } Easyspin_STATUS_DIR_t; 00301 00302 /// Easyspin internal register addresses 00303 typedef enum { 00304 Easyspin_ABS_POS = ((uint8_t) 0x01), 00305 Easyspin_EL_POS = ((uint8_t) 0x02), 00306 Easyspin_MARK = ((uint8_t) 0x03), 00307 Easyspin_RESERVED_REG01 = ((uint8_t) 0x04), 00308 Easyspin_RESERVED_REG02 = ((uint8_t) 0x05), 00309 Easyspin_RESERVED_REG03 = ((uint8_t) 0x06), 00310 Easyspin_RESERVED_REG04 = ((uint8_t) 0x07), 00311 Easyspin_RESERVED_REG05 = ((uint8_t) 0x08), 00312 Easyspin_RESERVED_REG06 = ((uint8_t) 0x15), 00313 Easyspin_TVAL = ((uint8_t) 0x09), 00314 Easyspin_RESERVED_REG07 = ((uint8_t) 0x0A), 00315 Easyspin_RESERVED_REG08 = ((uint8_t) 0x0B), 00316 Easyspin_RESERVED_REG09 = ((uint8_t) 0x0C), 00317 Easyspin_RESERVED_REG10 = ((uint8_t) 0x0D), 00318 Easyspin_T_FAST = ((uint8_t) 0x0E), 00319 Easyspin_TON_MIN = ((uint8_t) 0x0F), 00320 Easyspin_TOFF_MIN = ((uint8_t) 0x10), 00321 Easyspin_RESERVED_REG11 = ((uint8_t) 0x11), 00322 Easyspin_ADC_OUT = ((uint8_t) 0x12), 00323 Easyspin_OCD_TH = ((uint8_t) 0x13), 00324 Easyspin_RESERVED_REG12 = ((uint8_t) 0x14), 00325 Easyspin_STEP_MODE = ((uint8_t) 0x16), 00326 Easyspin_ALARM_EN = ((uint8_t) 0x17), 00327 Easyspin_CONFIG = ((uint8_t) 0x18), 00328 Easyspin_STATUS = ((uint8_t) 0x19), 00329 Easyspin_RESERVED_REG13 = ((uint8_t) 0x1A), 00330 Easyspin_RESERVED_REG14 = ((uint8_t) 0x1B), 00331 Easyspin_INEXISTENT_REG = ((uint8_t) 0x1F) 00332 } Easyspin_Registers_t; 00333 00334 /// Easyspin command set 00335 typedef enum { 00336 Easyspin_NOP = ((uint8_t) 0x00), 00337 Easyspin_SET_PARAM = ((uint8_t) 0x00), 00338 Easyspin_GET_PARAM = ((uint8_t) 0x20), 00339 Easyspin_ENABLE = ((uint8_t) 0xB8), 00340 Easyspin_DISABLE = ((uint8_t) 0xA8), 00341 Easyspin_GET_STATUS = ((uint8_t) 0xD0), 00342 Easyspin_RESERVED_CMD1 = ((uint8_t) 0xEB), 00343 Easyspin_RESERVED_CMD2 = ((uint8_t) 0xF8) 00344 } Easyspin_Commands_t; 00345 00346 /// Direction options 00347 typedef enum { 00348 FORWARD = 1, 00349 BACKWARD = 0 00350 } dir_t; 00351 00352 /// Rotation options 00353 typedef enum { 00354 RIGHT = 1, 00355 LEFT = 0 00356 } rot_t; 00357 00358 /// Shield state 00359 typedef enum { 00360 ACCELERATING = 0, 00361 DECELERATING = 1, 00362 STEADY = 2, 00363 INACTIVE= 3 00364 } shieldState_t; 00365 00366 /// Shield Commands 00367 typedef enum { 00368 RUN_CMD, 00369 MOVE_CMD, 00370 SOFT_STOP_CMD, 00371 NO_CMD 00372 } shieldCommand_t; 00373 00374 /// Easyspin shield parameters 00375 typedef struct { 00376 /// accumulator used to store speed increase smaller than 1 pps 00377 volatile uint32_t accu; 00378 /// Position in steps at the start of the goto or move commands 00379 volatile int32_t currentPosition; 00380 /// position in step at the end of the accelerating phase 00381 volatile uint32_t endAccPos; 00382 /// nb steps performed from the beggining of the goto or the move command 00383 volatile uint32_t relativePos; 00384 /// position in step at the start of the decelerating phase 00385 volatile uint32_t startDecPos; 00386 /// nb steps to perform for the goto or move commands 00387 volatile uint32_t stepsToTake; 00388 00389 /// acceleration in pps^2 00390 volatile uint16_t acceleration; 00391 /// deceleration in pps^2 00392 volatile uint16_t deceleration; 00393 /// max speed in pps (speed use for goto or move command) 00394 volatile uint16_t maxSpeed; 00395 /// min speed in pps 00396 volatile uint16_t minSpeed; 00397 /// current speed in pps 00398 volatile uint16_t speed; 00399 00400 /// command under execution 00401 volatile shieldCommand_t commandExecuted; 00402 /// FORWARD or BACKWARD direction 00403 volatile dir_t direction; 00404 /// Current State of the shield 00405 volatile shieldState_t motionState; 00406 }shieldParams_t; 00407 00408 /// Easyspin library class 00409 class Easyspin { 00410 public: 00411 // constructor: 00412 Easyspin(); 00413 00414 /// @defgroup group1 Shield control functions 00415 ///@{ 00416 void AttachFlagInterrupt(void (*callback)(void)); //Attach a user callback to the flag Interrupt 00417 void Begin(uint8_t nbShields); //Start the Easyspin library 00418 uint16_t GetAcceleration(uint8_t shieldId); //Return the acceleration in pps^2 00419 uint16_t GetCurrentSpeed(uint8_t shieldId); //Return the current speed in pps 00420 uint16_t GetDeceleration(uint8_t shieldId); //Return the deceleration in pps^2 00421 shieldState_t GetShieldState(uint8_t shieldId); //Return the shield state 00422 uint8_t GetFwVersion(void); //Return the FW version 00423 int32_t GetMark(uint8_t shieldId); //Return the mark position 00424 uint16_t GetMaxSpeed(uint8_t shieldId); //Return the max speed in pps 00425 uint16_t GetMinSpeed(uint8_t shieldId); //Return the min speed in pps 00426 int32_t GetPosition(uint8_t shieldId); //Return the ABS_POSITION (32b signed) 00427 void GoHome(uint8_t shieldId); //Move to the home position 00428 void GoMark(uint8_t shieldId); //Move to the Mark position 00429 void GoTo(uint8_t shieldId, int32_t targetPosition); //Go to the specified position 00430 void HardStop(uint8_t shieldId); //Stop the motor and disable the power bridge 00431 void Move(uint8_t shieldId, //Move the motor of the specified number of steps 00432 dir_t direction, 00433 uint32_t stepCount); 00434 void ResetAllShields(void); //Reset all Easyspin shields 00435 void Run(uint8_t shieldId, dir_t direction); //Run the motor 00436 bool SetAcceleration(uint8_t shieldId,uint16_t newAcc); //Set the acceleration in pps^2 00437 bool SetDeceleration(uint8_t shieldId,uint16_t newDec); //Set the deceleration in pps^2 00438 void SetHome(uint8_t shieldId); //Set current position to be the home position 00439 void SetMark(uint8_t shieldId); //Set current position to be the Markposition 00440 bool SetMaxSpeed(uint8_t shieldId,uint16_t newMaxSpeed); //Set the max speed in pps 00441 bool SetMinSpeed(uint8_t shieldId,uint16_t newMinSpeed); //Set the min speed in pps 00442 bool SoftStop(uint8_t shieldId); //Progressively stops the motor 00443 void WaitWhileActive(uint8_t shieldId); //Wait for the shield state becomes Inactive 00444 void Turn(rot_t rotation, uint16_t angle); //Turn left or right with the specified angle 00445 void Move_cm(dir_t direction, uint16_t distance); //Go forward or backward with the specified distance 00446 ///@} 00447 00448 /// @defgroup group2 Easyspin control functions 00449 ///@{ 00450 void CmdDisable(uint8_t shieldId); //Send the Easyspin_DISABLE command 00451 void CmdEnable(uint8_t shieldId); //Send the Easyspin_ENABLE command 00452 uint32_t CmdGetParam(uint8_t shieldId, //Send the Easyspin_GET_PARAM command 00453 Easyspin_Registers_t param); 00454 uint16_t CmdGetStatus(uint8_t shieldId); // Send the Easyspin_GET_STATUS command 00455 void CmdNop(uint8_t shieldId); //Send the Easyspin_NOP command 00456 void CmdSetParam(uint8_t shieldId, //Send the Easyspin_SET_PARAM command 00457 Easyspin_Registers_t param, 00458 uint32_t value); 00459 uint16_t ReadStatusRegister(uint8_t shieldId); // Read the Easyspin_STATUS register without 00460 // clearing the flags 00461 void Reset(void); //Set the Easyspin reset pin 00462 void ReleaseReset(void); //Release the Easyspin reset pin 00463 void SelectStepMode(uint8_t shieldId, // Step mode selection 00464 Easyspin_STEP_SEL_t stepMod); 00465 void SetDirection(uint8_t shieldId, //Set the Easyspin direction pin 00466 dir_t direction); 00467 ///@} 00468 00469 /// @defgroup group4 Functions for timer ISRs only 00470 /// @brief To be used inside the library by the timer ISRs only 00471 /// Must not be used elsewhere. 00472 ///@{ 00473 static class Easyspin *GetInstancePtr(void); 00474 void StepClockHandler(uint8_t shieldId); 00475 ///@} 00476 00477 private: 00478 void ApplySpeed(uint8_t pwmId, uint16_t newSpeed); 00479 void ComputeSpeedProfile(uint8_t shieldId, uint32_t nbSteps); 00480 int32_t ConvertPosition(uint32_t abs_position_reg); 00481 static void FlagInterruptHandler(void); 00482 void SendCommand(uint8_t shieldId, uint8_t param); 00483 void SetRegisterToPredefinedValues(uint8_t shieldId); 00484 void WriteBytes(uint8_t *pByteToTransmit, uint8_t *pReceivedByte); 00485 void PwmInit(uint8_t pwmId); 00486 void Pwm1SetFreq(uint16_t newFreq); 00487 void Pwm2SetFreq(uint16_t newFreq); 00488 void Pwm3SetFreq(uint16_t newFreq); 00489 void PwmStop(uint8_t pwmId); 00490 void SetShieldParamsToPredefinedValues(void); 00491 void StartMovement(uint8_t shieldId); 00492 uint8_t Tval_Current_to_Par(double Tval); 00493 uint8_t Tmin_Time_to_Par(double Tmin); 00494 void tick1(); 00495 void tick2(); 00496 00497 // variable members 00498 DigitalOut dir1; 00499 DigitalOut dir2; 00500 DigitalOut dir3; 00501 DigitalOut pwm1; 00502 DigitalOut pwm2; 00503 DigitalOut pwm3; 00504 DigitalOut reset; 00505 DigitalOut CS; 00506 Ticker ticker1; 00507 Ticker ticker2; 00508 Ticker ticker3; 00509 InterruptIn flag; 00510 SPI spi; 00511 shieldParams_t shieldPrm[MAX_NUMBER_OF_SHIELDS]; 00512 static volatile class Easyspin *instancePtr; 00513 static volatile void(*flagInterruptCallback)(void); 00514 static volatile bool isrFlag; 00515 static volatile bool spiPreemtionByIsr; 00516 static volatile uint8_t numberOfShields; 00517 static const uint16_t prescalerArrayTimer0_1[PRESCALER_ARRAY_TIMER0_1_SIZE]; 00518 static const uint16_t prescalerArrayTimer2[PRESCALER_ARRAY_TIMER2_SIZE]; 00519 static uint8_t spiTxBursts[Easyspin_CMD_ARG_MAX_NB_BYTES][MAX_NUMBER_OF_SHIELDS]; 00520 static uint8_t spiRxBursts[Easyspin_CMD_ARG_MAX_NB_BYTES][MAX_NUMBER_OF_SHIELDS]; 00521 }; 00522 00523 #ifdef _DEBUG_Easyspin 00524 uint16_t GetFreeRam (void); 00525 #endif 00526 00527 #endif /* #ifndef __Easyspin_H_INCLUDED */
Generated on Tue Jul 12 2022 21:33:48 by
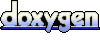