
Updated version of RenBuggy Servo that can accept instructions based on time or distance.
Fork of RenBuggyServo by
Car Class Reference
RenBuggyServo Example: More...
#include <Car.h>
Public Member Functions | |
Car (PinName servoPin, PinName motorPin) | |
Constructs the car with PwmOut objects for servo and motor. | |
Car (PinName servoPin, PinName motorPin, int countsPerRevolution, float wheelCircumference, PinName sensorPin) | |
Constructs the car with PwmOut objects for servo and motor, and configures the encoder. | |
~Car () | |
Deconstructs the car. | |
void | setSpeed (int speed_us) |
Sets the speed the buggy will move at. | |
void | forwards_measured (float distance) |
Moves the car in the direction it is facing, for a specified distance. | |
void | forwards_timed (float Time) |
Moves the car in the direction it is facing, until a user tells it to stop. | |
void | forwards (float distance) |
Moves the car in the direction it is facing, for a specified distance. | |
void | stop () |
Stops the car from moving. | |
void | setDirection (int degrees) |
Sets the direction the car will face. | |
void | configureServo_us (int pulsewidth_us, int period_us, int range, float degrees) |
Configures the servo with a pulsewidth, period, range and degrees. | |
void | configureServo_ms (int pulsewidth_ms, int period_ms, int range, float degrees) |
Configures the servo with a pulsewidth, period, range and degrees. | |
void | configureMotor_us (int pulsewidth_us, int period_us) |
Configures the pulsewidth and period for the motor, in microseconds. | |
void | configureMotor_ms (int pulsewidth_ms, int period_ms) |
Configures the pulsewidth and period for the motor, in milliseconds. | |
void | configureEncoder (int countsPerRevolution, float wheelCircumference) |
Provides information required to make use of an encoder for specifying distance. |
Detailed Description
RenBuggyServo Example:
#include "mbed.h" #include "Car.h" Car myCar(P1_25, P1_24); // Main entry point of application. int main() { // Drive the RenBuggy! myCar.setSpeed(20000); myCar.setDirection(0); myCar.forwards(); wait(1); myCar.setDirection(45); wait(1); myCar.setDirection(-45); wait(1); myCar.setDirection(0); myCar.stop(); myCar.forwards(10); // Move the car a final 10cm. myCar.stop(); } // End programme.
The car class for controlling the RenBuggy_Servo.
Definition at line 70 of file Car.h.
Constructor & Destructor Documentation
Car | ( | PinName | servoPin, |
PinName | motorPin | ||
) |
Car | ( | PinName | servoPin, |
PinName | motorPin, | ||
int | countsPerRevolution, | ||
float | wheelCircumference, | ||
PinName | sensorPin | ||
) |
Constructs the car with PwmOut objects for servo and motor, and configures the encoder.
- Parameters:
-
servoPin is the pin used for pwm output for driving the servo. motorPin is the pin used for pwm output for driving the motor. countsPerRevolution is the number of counts the encoder makes in one full cycle of the wheel. wheelCircumference is the circumference of the wheel being read by the encoder. sensorPin is the pin required for the encoder for debouncing.
Member Function Documentation
void configureEncoder | ( | int | countsPerRevolution, |
float | wheelCircumference | ||
) |
Provides information required to make use of an encoder for specifying distance.
- Parameters:
-
countsPerRevolution is the number of counts the encoder makes in one full cycle of the wheel. wheelCircumference is the circumference of the wheel being read by the encoder.
void configureMotor_ms | ( | int | pulsewidth_ms, |
int | period_ms | ||
) |
void configureMotor_us | ( | int | pulsewidth_us, |
int | period_us | ||
) |
void configureServo_ms | ( | int | pulsewidth_ms, |
int | period_ms, | ||
int | range, | ||
float | degrees | ||
) |
Configures the servo with a pulsewidth, period, range and degrees.
Pulsewidth and period is accepted in millisecond format.
- Parameters:
-
pulsewidth_ms is pwm pulsewidth for the servo, in millisecond. period_ms is the pwm period for the servo, in millisecond. range is the pulsewidth range to full left/right turn of the servo from centre (1.5ms). degrees is the angle to full right/left turn of the servo from centre (0).
void configureServo_us | ( | int | pulsewidth_us, |
int | period_us, | ||
int | range, | ||
float | degrees | ||
) |
Configures the servo with a pulsewidth, period, range and degrees.
Pulsewidth and period is accepted in microsecond format.
- Parameters:
-
pulsewidth_us is pwm pulsewidth for the servo, in mircoseconds. period_us is the pwm period for the servo, in mircoseconds. range is the pulsewidth range to full left/right turn of the servo from centre (1500us). degrees is the angle to full right/left turn of the servo from centre (0).
void forwards | ( | float | distance ) |
void forwards_measured | ( | float | distance ) |
void forwards_timed | ( | float | Time ) |
void setDirection | ( | int | degrees ) |
void setSpeed | ( | int | speed_us ) |
Generated on Tue Jul 12 2022 21:16:14 by
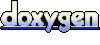