
Updated version of RenBuggy Servo that can accept instructions based on time or distance.
Fork of RenBuggyServo by
Car.h
00001 /******************************************************************************* 00002 * RenBED Car used to drive RenBuggy with servo and 1 motor * 00003 * Copyright (c) 2014 Mark Jones * 00004 * * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy * 00006 * of this software and associated documentation files (the "Software"), to deal* 00007 * in the Software without restriction, including without limitation the rights * 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell * 00009 * copies of the Software, and to permit persons to whom the Software is * 00010 * furnished to do so, subject to the following conditions: * 00011 * * 00012 * The above copyright notice and this permission notice shall be included in * 00013 * all copies or substantial portions of the Software. * 00014 * * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR * 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, * 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE * 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER * 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,* 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN * 00021 * THE SOFTWARE. * 00022 * * 00023 * Car.h * 00024 * * 00025 * V1.1 31/03/2014 Mark Jones * 00026 *******************************************************************************/ 00027 00028 #ifndef CAR_H 00029 #define CAR_H 00030 00031 #include "mbed.h" 00032 #include "PinDetect.h" 00033 00034 /** 00035 * RenBuggyServo Example: 00036 * @code 00037 * 00038 * 00039 #include "mbed.h" 00040 #include "Car.h" 00041 00042 Car myCar(P1_25, P1_24); 00043 00044 // Main entry point of application. 00045 int main() { 00046 00047 // Drive the RenBuggy! 00048 myCar.setSpeed(20000); 00049 myCar.setDirection(0); 00050 00051 myCar.forwards(); 00052 wait(1); 00053 myCar.setDirection(45); 00054 wait(1); 00055 myCar.setDirection(-45); 00056 wait(1); 00057 myCar.setDirection(0); 00058 myCar.stop(); 00059 00060 myCar.forwards(10); // Move the car a final 10cm. 00061 myCar.stop(); 00062 } 00063 // End programme. 00064 @endcode 00065 */ 00066 00067 /** 00068 ** The car class for controlling the RenBuggy_Servo. 00069 */ 00070 class Car { 00071 private: 00072 00073 PwmOut m_servo; 00074 PwmOut m_motor; 00075 00076 int m_speed; 00077 00078 int m_encoderCount; 00079 00080 int m_servoRange; // Pulsewidth range to full left/right from centre (1.5ms) 00081 float m_servoDegrees; // Angle to full right/left turn from centre (0). 00082 00083 float m_wheelCircumference; // The circumference of the wheel with stripes. 00084 int m_countsPerRevolution; // The number of stripes on the wheel. 00085 00086 PinDetect m_sensor; // For debouncing. 00087 00088 void updateEncoderCount(); 00089 00090 public: 00091 /** Constructs the car with PwmOut objects for servo and motor. 00092 * 00093 * @param servoPin is the pin used for pwm output for driving the servo. 00094 * @param motorPin is the pin used for pwm output for driving the motor. 00095 */ 00096 Car(PinName servoPin, PinName motorPin); 00097 00098 /** Constructs the car with PwmOut objects for servo and motor, and configures 00099 * the encoder. 00100 * 00101 * @param servoPin is the pin used for pwm output for driving the servo. 00102 * @param motorPin is the pin used for pwm output for driving the motor. 00103 * @param countsPerRevolution is the number of counts the encoder 00104 * makes in one full cycle of the wheel. 00105 * @param wheelCircumference is the circumference of the wheel being 00106 * read by the encoder. 00107 * @param sensorPin is the pin required for the encoder for debouncing. 00108 */ 00109 Car(PinName servoPin, PinName motorPin, int countsPerRevolution, float wheelCircumference, PinName sensorPin); 00110 00111 /** 00112 * Deconstructs the car. 00113 */ 00114 ~Car(); 00115 00116 /** 00117 * Sets the speed the buggy will move at. 00118 * @param speed_us is the speed the car will move at, in microseconds. 00119 */ 00120 void setSpeed(int speed_us); 00121 00122 /** 00123 * Moves the car in the direction it is facing, for a specified 00124 * distance. 00125 * @param distance is how far the car will travel, in cm. 00126 */ 00127 void forwards_measured(float distance); 00128 00129 /** 00130 * Moves the car in the direction it is facing, until a user 00131 * tells it to stop. 00132 * @param Time is the time in seconds during which it will drive forwards, then stop. 00133 */ 00134 void forwards_timed(float Time); 00135 00136 /** 00137 * Moves the car in the direction it is facing, for a specified 00138 * distance. 00139 * @param distance is how far the car will travel, in cm. 00140 */ 00141 void forwards(float distance); 00142 00143 /** 00144 * Stops the car from moving. 00145 */ 00146 void stop(); 00147 00148 /** 00149 * Sets the direction the car will face. This is used to navigate the car. 00150 * @param degrees is the angle of the servo, where -45 is full 00151 * left, 0 is centre and +45 is full right. 00152 */ 00153 void setDirection(int degrees); 00154 00155 /** 00156 * Configures the servo with a pulsewidth, period, range and degrees. Pulsewidth and 00157 * period is accepted in microsecond format. 00158 * @param pulsewidth_us is pwm pulsewidth for the servo, in mircoseconds. 00159 * @param period_us is the pwm period for the servo, in mircoseconds. 00160 * @param range is the pulsewidth range to full left/right turn of the servo from centre (1500us). 00161 * @param degrees is the angle to full right/left turn of the servo from centre (0). 00162 */ 00163 void configureServo_us(int pulsewidth_us, int period_us, int range, float degrees); 00164 00165 /** 00166 * Configures the servo with a pulsewidth, period, range and degrees. Pulsewidth and 00167 * period is accepted in millisecond format. 00168 * @param pulsewidth_ms is pwm pulsewidth for the servo, in millisecond. 00169 * @param period_ms is the pwm period for the servo, in millisecond. 00170 * @param range is the pulsewidth range to full left/right turn of the servo from centre (1.5ms). 00171 * @param degrees is the angle to full right/left turn of the servo from centre (0). 00172 */ 00173 void configureServo_ms(int pulsewidth_ms, int period_ms, int range, float degrees); 00174 00175 /** 00176 * Configures the pulsewidth and period for the motor, in microseconds. 00177 * @param pulsewidth_us is the pwm pulsewidth for the motor, in mircoseconds. 00178 * @param period_us is the pwm period for the motor, in microseconds. 00179 */ 00180 void configureMotor_us(int pulsewidth_us, int period_us); 00181 00182 /** 00183 * Configures the pulsewidth and period for the motor, in milliseconds. 00184 * @param pulsewidth_ms is the pwm pulsewidth for the motor, in milliseconds. 00185 * @param period_ms is the pwm period for the motor, in milliseconds. 00186 */ 00187 void configureMotor_ms(int pulsewidth_ms, int period_ms); 00188 00189 /** 00190 * Provides information required to make use of an encoder for 00191 * specifying distance. 00192 * @param countsPerRevolution is the number of counts the encoder 00193 * makes in one full cycle of the wheel. 00194 * @param wheelCircumference is the circumference of the wheel being 00195 * read by the encoder. 00196 */ 00197 void configureEncoder(int countsPerRevolution, float wheelCircumference); 00198 }; 00199 00200 #endif // CAR_H
Generated on Tue Jul 12 2022 21:16:14 by
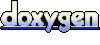