Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
r_ospl.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_ospl.h 00025 * @brief OS Porting Layer. Main Header. Functions. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 #ifndef R_OSPL_H 00033 #define R_OSPL_H 00034 00035 00036 /****************************************************************************** 00037 Includes <System Includes> , "Project Includes" 00038 ******************************************************************************/ 00039 #include "Project_Config.h" 00040 #include "platform.h" 00041 #include "r_ospl_typedef.h" 00042 #include "r_multi_compiler_typedef.h" 00043 #include "locking.h" 00044 #include "r_static_an_tag.h" 00045 #include "r_ospl_debug.h" 00046 #if ! R_OSPL_IS_PREEMPTION 00047 #include "r_ospl_os_less.h" 00048 #endif 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif /* __cplusplus */ 00053 00054 00055 /****************************************************************************** 00056 Typedef definitions 00057 ******************************************************************************/ 00058 /* In "r_ospl_typedef.h" */ 00059 00060 /****************************************************************************** 00061 Macro definitions 00062 ******************************************************************************/ 00063 /* In "r_ospl_typedef.h" */ 00064 00065 /****************************************************************************** 00066 Variable Externs 00067 ******************************************************************************/ 00068 /* In "r_ospl_typedef.h" */ 00069 00070 /****************************************************************************** 00071 Functions Prototypes 00072 ******************************************************************************/ 00073 00074 00075 /* Section: Version and initialize */ 00076 /** 00077 * @brief Returns version number of OSPL 00078 * 00079 * @par Parameters 00080 * None 00081 * @return Version number of OSPL 00082 * 00083 * @par Description 00084 * Return value is same as "R_OSPL_VERSION" macro. 00085 */ 00086 int32_t R_OSPL_GetVersion(void); 00087 00088 00089 /** 00090 * @brief Returns whether the environment is supported preemption 00091 * 00092 * @par Parameters 00093 * None 00094 * @return Whether the environment is RTOS supported preemption 00095 * 00096 * @par Description 00097 * Return value is same as "R_OSPL_IS_PREEMPTION" macro. 00098 */ 00099 bool_t R_OSPL_IsPreemption(void); 00100 00101 00102 /** 00103 * @brief Initializes the internal of OSPL 00104 * 00105 * @param NullConfig Specify NULL 00106 * @return None 00107 * 00108 * @par Description 00109 * Initializes internal mutual exclusion objects. 00110 * However, "R_OSPL_Initialize" function does not have to be called for 00111 * OSPL of "R_OSPL_IS_PREEMPTION = 0". 00112 * "E_ACCESS_DENIED" error is raised, when the OSPL API that it is 00113 * necessary to call "R_OSPL_Initialize" before calling the API was called. 00114 */ 00115 errnum_t R_OSPL_Initialize( const void *const NullConfig ); 00116 00117 00118 /* Section: Standard functions */ 00119 /** 00120 * @brief No operation from C++ specification 00121 * 00122 * @par Parameters 00123 * None 00124 * @return None 00125 * 00126 * @par Description 00127 * Compatible with __noop (MS C++). But our naming rule is not match. 00128 */ 00129 INLINE void R_NOOP(void) {} 00130 00131 00132 /** 00133 * @brief Returns element count of the array 00134 * 00135 * @param Array An array 00136 * @return Count of specified array's element 00137 * 00138 * @par Description 00139 * Compatible with _countof (MS C++) and ARRAY_SIZE (Linux). 00140 * But our naming rule is not match. 00141 * 00142 * @par Example 00143 * @code 00144 * uint32_t array[10]; 00145 * R_COUNT_OF( array ) // = 10 00146 * @endcode 00147 * 00148 * @par Example 00149 * Array argument must not be specified the pointer using like array. 00150 * @code 00151 * uint32_t array[10]; 00152 * func( array ); 00153 * 00154 * void func( uint32_t array[] ) // "array" is a pointer 00155 * { 00156 * R_COUNT_OF( array ) // NG 00157 * } 00158 * @endcode 00159 */ 00160 /* ->MISRA 19.7 : Cannot function */ /* ->SEC M5.1.3 */ 00161 #define R_COUNT_OF( Array ) ( sizeof( Array ) / sizeof( *(Array) ) ) 00162 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 00163 00164 00165 /* Section: Error handling and debugging (1) */ 00166 00167 00168 /** 00169 * @def IF 00170 * @brief Breaks and transits to error state, if condition expression is not 0 00171 * @param Condition Condition expression 00172 * @return None 00173 * 00174 * @par Example 00175 * @code 00176 * e= TestFunction(); IF(e){goto fin;} 00177 * @endcode 00178 * 00179 * @par Description 00180 * "IF" is as same as general "if", if "R_OSPL_ERROR_BREAK" macro was 00181 * defined to be 0. The following descriptions are available, 00182 * if "R_OSPL_ERROR_BREAK" macro was defined to be 1. 00183 * 00184 * "IF" macro supports to find the code raising an error. 00185 * 00186 * If the "if statement" that is frequently seen in guard condition and 00187 * after calling functions was changed to "IF" macro, the CPU breaks 00188 * at raising an error. Then the status (values of variables) can be 00189 * looked immediately and the code (call stack) can be looked. Thus, 00190 * debug work grows in efficiency. 00191 * 00192 * "IF" macro promotes recognizing normal code and exceptional code. 00193 * Reading speed will grow up by skipping exceptional code. 00194 * 00195 * Call "R_OSPL_SET_BREAK_ERROR_ID" function, if set to break at the code 00196 * raising an error. 00197 * 00198 * Whether the state was error state or the error raised count is stored 00199 * in the thread local storage. In Release configuration, the variable 00200 * of error state and the error raised count is deleted. Manage the error 00201 * code using auto variable and so on at out of OSPL. 00202 * 00203 * The error state is resolved by calling "R_OSPL_CLEAR_ERROR" function. 00204 * If "R_DEBUG_BREAK_IF_ERROR" macro was called with any error state, 00205 * the process breaks at the macro. 00206 */ 00207 #if R_OSPL_ERROR_BREAK 00208 00209 /* ->MISRA 19.4 : Abnormal termination. Compliant with C language syntax. */ /* ->SEC M1.8.2 */ 00210 #define IF( Condition ) \ 00211 if ( IS( R_OSPL_OnRaisingErrorForMISRA( \ 00212 IS( (int_fast32_t)( Condition ) ), __FILE__, __LINE__ ) ) ) 00213 /* (int_fast32_t) cast is for QAC warning of implicit cast unsigned to signed */ 00214 /* != 0 is for QAC warning of MISRA 13.2 Advice */ 00215 /* <-MISRA 19.4 */ /* <-SEC M1.8.2 */ 00216 00217 #else /* ! R_OSPL_ERROR_BREAK */ 00218 00219 /* ->MISRA 19.4 : Abnormal termination. Compliant with C language syntax. */ /* ->SEC M1.8.2 */ 00220 #define IF if 00221 /* <-MISRA 19.4 */ /* <-SEC M1.8.2 */ 00222 #endif 00223 00224 00225 /** 00226 * @def IF_D 00227 * @brief It is same as "IF" (for Debug configuration only) 00228 * @param Condition Condition expression 00229 * @return None 00230 * 00231 * @par Description 00232 * In Release configuration, the result of condition expression is always "false". 00233 * The release configuration is the configuration defined "R_OSPL_NDEBUG". 00234 */ 00235 /* ->MISRA 19.4 : Compliant with C language syntax. */ /* ->SEC M1.8.2 */ 00236 /* ->MISRA 19.7 : Cannot function */ /* ->SEC M5.1.3 */ 00237 #ifndef R_OSPL_NDEBUG 00238 #define IF_D( Condition ) IF ( Condition ) 00239 #else 00240 #define IF_D( Condition ) if ( false ) 00241 #endif 00242 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 00243 /* <-MISRA 19.4 */ /* <-SEC M1.8.2 */ 00244 00245 00246 /** 00247 * @def ASSERT_R 00248 * @brief Assertion (Programming By Contract) 00249 * @param Condition The condition expression expected true 00250 * @param goto_fin_Statement The operation doing at condition is false 00251 * @return None 00252 * 00253 * @par Description 00254 * It is possible to write complex sentence divided by ";" in 00255 * "goto_fin_Statement" argument. 00256 * 00257 * @par - Case of defined "R_OSPL_ERROR_BREAK" to be 0 00258 * If the result of condition expression is 0(false), do "StatementsForError". 00259 * If operations did nothing, write "R_NOOP()" at "StatementsForError" argument. 00260 * 00261 * @par - Case of defined "R_OSPL_ERROR_BREAK" to be 1 00262 * If the result of condition expression is 0(false), the error state 00263 * will become active and the operation of "StatementForError" argument 00264 * will be done. 00265 */ 00266 #ifndef __cplusplus 00267 #define ASSERT_R( Condition, goto_fin_Statement ) \ 00268 do{ IF(!(Condition)) { goto_fin_Statement; } } while(0) /* do-while is CERT standard PRE10-C */ 00269 #else 00270 #define ASSERT_R( Condition, goto_fin_Statement ) \ 00271 { IF(!(Condition)) { goto_fin_Statement; } } /* no C5236(I) */ 00272 #endif 00273 00274 00275 /** 00276 * @def ASSERT_D 00277 * @brief Assertion (Programming By Contract) (for Debug configuration only) 00278 * @param Condition The condition expression expected true 00279 * @param goto_fin_Statement The operation doing at condition is false 00280 * @return None 00281 * 00282 * @par Description 00283 * This does nothing in Release configuration. 00284 * Release configuration is the configuration defined "R_OSPL_NDEBUG" 00285 * as same as standard library. 00286 */ 00287 #ifndef R_OSPL_NDEBUG 00288 #define ASSERT_D ASSERT_R 00289 #else 00290 /* ->MISRA 19.7 : Function's argument can not get "goto_fin_Statement" */ /* ->SEC M5.1.3 */ 00291 #define ASSERT_D( Condition, goto_fin_Statement ) R_NOOP() 00292 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 00293 #endif 00294 00295 00296 /** 00297 * @brief Sub routine of IF macro 00298 * 00299 * @param Condition Condition in IF macro 00300 * @param File File name 00301 * @param Line Line number 00302 * @return "Condition" argument 00303 * 00304 * @par Description 00305 * - This part is for compliant to MISRA 2004 - 19.7. 00306 */ 00307 bool_t R_OSPL_OnRaisingErrorForMISRA( bool_t const Condition, const char_t *const File, 00308 int_t const Line ); 00309 00310 00311 /*********************************************************************** 00312 * Class: r_ospl_thread_id_t 00313 ************************************************************************/ 00314 00315 /** 00316 * @brief Get running thread ID (for OS less and OS-using environment) 00317 * 00318 * @par Parameters 00319 * None 00320 * @return The current running thread ID 00321 * 00322 * @par Description 00323 * - It is possible to use this function for both OS less and OS - using environment. 00324 * For OS less, returns thread ID passed to "R_OSPL_THREAD_SetCurrentId" function. 00325 * "NULL" is returned, if in interrupt context. 00326 */ 00327 r_ospl_thread_id_t R_OSPL_THREAD_GetCurrentId(void); 00328 00329 00330 /** 00331 * @brief Set one or some bits to 1 00332 * 00333 * @param ThreadId The thread ID attached the target event 00334 * @param SetFlags The value of bit flags that target bit is 1 00335 * @return None 00336 * 00337 * @par Description 00338 * For OS less, there is the area disabled all interrupts. 00339 * 00340 * - For OS - using environment, the thread waiting in "R_OSPL_EVENT_Wait" 00341 * function might wake up soon. 00342 * 00343 * Do nothing, when "ThreadId" = "NULL" 00344 */ 00345 void R_OSPL_EVENT_Set( r_ospl_thread_id_t const ThreadId, bit_flags32_t const SetFlags ); 00346 00347 00348 /** 00349 * @brief Set one or some bits to 0 00350 * 00351 * @param ThreadId The thread ID attached the target event 00352 * @param ClearFlags1 The value of bit flags that clearing bit is 1 00353 * @return None 00354 * 00355 * @par Description 00356 * It is not necessary to call this function after called "R_OSPL_EVENT_Wait" 00357 * function. 00358 * 00359 * The way that all bit flags is cleared is setting "R_OSPL_EVENT_ALL_BITS" 00360 * (=0x0000FFFF) at "ClearFlags1" argument. 00361 * 00362 * When other thread was nofied by calling "R_OSPL_EVENT_Set", "R_OSPL_EVENT_Clear" 00363 * must not be called from caller (notifier) thread. 00364 * 00365 * For OS less, there is the area disabled all interrupts. 00366 * 00367 * Do nothing, when "ThreadId" = "NULL" 00368 */ 00369 void R_OSPL_EVENT_Clear( r_ospl_thread_id_t const ThreadId, bit_flags32_t const ClearFlags1 ); 00370 00371 00372 /** 00373 * @brief Get 16bit flags value 00374 * 00375 * @param ThreadId The thread ID attached the target event 00376 * @return The value of 16bit flags 00377 * 00378 * @par Description 00379 * This API cannot be used in newest specification. 00380 * 00381 * In receiving the event, call "R_OSPL_EVENT_Wait" function instead of 00382 * "R_OSPL_EVENT_Get" function or call "R_OSPL_EVENT_Clear" function 00383 * passed the NOT operated value of flags got by "R_OSPL_EVENT_Get" function. 00384 */ 00385 #if ( ! defined( osCMSIS ) || osCMSIS <= 0x10001 ) && R_OSPL_VERSION < 85 00386 bit_flags32_t R_OSPL_EVENT_Get( r_ospl_thread_id_t const ThreadId ); 00387 #endif 00388 00389 00390 /** 00391 * @brief Waits for setting the flags in 16bit and clear received flags 00392 * 00393 * @param WaigingFlags The bit flags set to 1 waiting or "R_OSPL_ANY_FLAG" 00394 * @param out_GotFlags NULL is permitted. Output: 16 bit flags or "R_OSPL_TIMEOUT" 00395 * @param Timeout_msec Time out (millisecond) or "R_OSPL_INFINITE" 00396 * @return Error code. If there is no error, the return value is 0. 00397 * 00398 * @par Description 00399 * Waits in this function until the flags become passed flags pattern 00400 * by "R_OSPL_EVENT_Set" function. 00401 * 00402 * Check "r_ospl_async_t::ReturnValue", when the asynchronous operation 00403 * was ended. 00404 */ 00405 errnum_t R_OSPL_EVENT_Wait( bit_flags32_t const WaigingFlags, bit_flags32_t *const out_GotFlags, 00406 uint32_t const Timeout_msec ); 00407 /* Unsigned flag (bit_flags32_t) is for QAC 4130 */ 00408 00409 00410 /*********************************************************************** 00411 * Class: r_ospl_flag32_t 00412 ************************************************************************/ 00413 00414 /** 00415 * @brief Clears all flags in 32bit to 0 00416 * 00417 * @param self The value of 32bit flags 00418 * @return None 00419 * 00420 * @par Description 00421 * Operates following operation. 00422 * @code 00423 * volatile bit_flags32_t self->flags; 00424 * self->flags = 0; 00425 * @endcode 00426 */ 00427 void R_OSPL_FLAG32_InitConst( volatile r_ospl_flag32_t *const self ); 00428 00429 00430 /** 00431 * @brief Set one or some bits to 1 00432 * 00433 * @param self The value of 32bit flags 00434 * @param SetFlags The value of bit flags that target bit is 1 00435 * @return None 00436 * 00437 * @par Description 00438 * Operates following operation. 00439 * @code 00440 * volatile bit_flags32_t self->Flags; 00441 * bit_flags32_t SetFlags; 00442 * self->Flags |= SetFlags; 00443 * @endcode 00444 * This function is not atomic because "|=" operator is "Read Modify Write" operation. 00445 */ 00446 void R_OSPL_FLAG32_Set( volatile r_ospl_flag32_t *const self, bit_flags32_t const SetFlags ); 00447 00448 00449 /** 00450 * @brief Set one or some bits to 0 00451 * 00452 * @param self The value of 32bit flags 00453 * @param ClearFlags1 The value of bit flags that clearing bit is 1 00454 * @return None 00455 * 00456 * @par Description 00457 * Operates following operation. 00458 * @code 00459 * volatile bit_flags32_t self->Flags; 00460 * bit_flags32_t ClearFlags1; 00461 * 00462 * self->Flags &= ~ClearFlags1; 00463 * @endcode 00464 * 00465 * Set "R_OSPL_FLAG32_ALL_BITS", if you wanted to clear all bits. 00466 * 00467 * This function is not atomic because "&=" operator is "Read Modify Write" operation. 00468 */ 00469 void R_OSPL_FLAG32_Clear( volatile r_ospl_flag32_t *const self, bit_flags32_t const ClearFlags1 ); 00470 00471 00472 /** 00473 * @brief Get 32bit flags value 00474 * 00475 * @param self The value of 32bit flags 00476 * @return The value of 32bit flags 00477 * 00478 * @par Description 00479 * In receiving the event, call "R_OSPL_FLAG32_GetAndClear" function 00480 * instead of "R_OSPL_FLAG32_Get" function or call "R_OSPL_FLAG32_Clear" 00481 * function passed the NOT operated value of flags got by "R_OSPL_FLAG32_Get" 00482 * function. 00483 * 00484 * @code 00485 * Operates following operation. 00486 * volatile bit_flags32_t self->Flags; 00487 * bit_flags32_t return_flags; 00488 * 00489 * return_flags = self->Flags; 00490 * 00491 * return return_flags; 00492 * @endcode 00493 */ 00494 bit_flags32_t R_OSPL_FLAG32_Get( volatile const r_ospl_flag32_t *const self ); 00495 00496 00497 /** 00498 * @brief Get 32bit flags value 00499 * 00500 * @param self The value of 32bit flags 00501 * @return The value of 32bit flags 00502 * 00503 * @par Description 00504 * Operates following operation. 00505 * @code 00506 * volatile bit_flags32_t self->Flags; 00507 * bit_flags32_t return_flags; 00508 * 00509 * return_flags = self->Flags; 00510 * self->Flags = 0; 00511 * 00512 * return return_flags; 00513 * @endcode 00514 * 00515 * This function is not atomic because the value might be set before clearing to 0. 00516 */ 00517 bit_flags32_t R_OSPL_FLAG32_GetAndClear( volatile r_ospl_flag32_t *const self ); 00518 00519 00520 /*********************************************************************** 00521 * Class: r_ospl_queue_t 00522 ************************************************************************/ 00523 00524 /** 00525 * @brief Initializes a queue 00526 * 00527 * @param out_self Output: Address of initialized queue object 00528 * @param QueueDefine Initial attributes of queue and work area 00529 * @return Error code. If there is no error, the return value is 0 00530 * 00531 * @par Description 00532 * It is not possible to call this function from the library. 00533 * This function is called from porting layer of the driver and send 00534 * created queue to the driver. 00535 * 00536 * OSPL does not have finalizing function (portabled with CMSIS). 00537 * An object specified "QueueDefine" argument can be specified to the 00538 * create function 1 times only. Some OS does not have this limitation. 00539 * 00540 * The address of a variable as "r_ospl_queue_t*" type is set at 00541 * "out_self" argument. 00542 * Internal variables of the queue are stored in the variable specified 00543 * with "QueueDefine" argument. 00544 */ 00545 errnum_t R_OSPL_QUEUE_Create( r_ospl_queue_t **out_self, r_ospl_queue_def_t *QueueDefine ); 00546 00547 00548 /** 00549 * @brief Gets status of the queue 00550 * 00551 * @param self A queue object 00552 * @param out_Status Output: Pointer to the status structure 00553 * @return Error code. If there is no error, the return value is 0 00554 * 00555 * @par Description 00556 * Got status are the information at calling moment. 00557 * If ohter threads were run, the status will be changed. 00558 * See "R_DRIVER_GetAsyncStatus" function about pointer type of 00559 * "out_Status" argument 00560 */ 00561 errnum_t R_OSPL_QUEUE_GetStatus( r_ospl_queue_t *self, const r_ospl_queue_status_t **out_Status ); 00562 00563 00564 /** 00565 * @brief Allocates an element from the queue object 00566 * 00567 * @param self A queue object 00568 * @param out_Address Output: Address of allocated element 00569 * @param Timeout_msec Timeout (msec) or R_OSPL_INFINITE 00570 * @return Error code. If there is no error, the return value is 0 00571 * 00572 * @par Description 00573 * An error will be raised, if "Timeout_msec != 0" in interrupt context. 00574 * It becomes "*out_Address = NULL", when it was timeout and 00575 * "Timeout_msec = 0". 00576 * E_TIME_OUT error is raised, when it was timeout and "Timeout_msec != 0". 00577 */ 00578 errnum_t R_OSPL_QUEUE_Allocate( r_ospl_queue_t *self, void *out_Address, uint32_t Timeout_msec ); 00579 00580 00581 /** 00582 * @brief Sends the element to the queue 00583 * 00584 * @param self A queue object 00585 * @param Address Address of element to put 00586 * @return Error code. If there is no error, the return value is 0 00587 * 00588 * @par Description 00589 * It is correct, even if other thread put to the queue or get from 00590 * the queue from calling "R_OSPL_QUEUE_Allocate" to calling 00591 * "R_OSPL_QUEUE_Put". 00592 * 00593 * The message put to the queue by this function receives the thread 00594 * calling "R_OSPL_QUEUE_Get" function. 00595 */ 00596 errnum_t R_OSPL_QUEUE_Put( r_ospl_queue_t *self, void *Address ); 00597 00598 00599 /** 00600 * @brief Receives the element from the queue 00601 * 00602 * @param self A queue object 00603 * @param out_Address Output: Address of received element 00604 * @param Timeout_msec Timeout (msec) or R_OSPL_INFINITE 00605 * @return Error code. If there is no error, the return value is 0 00606 * 00607 * @par Description 00608 * Call "R_OSPL_QUEUE_Free" function after finishing to access to 00609 * the element. Don't access the memory area of the element after 00610 * calling "R_OSPL_QUEUE_Free". 00611 * 00612 * "E_NOT_THREAD" error is raised, if "Timeout_msec = 0" was specified 00613 * from interrupt context. It is not possible to wait for put data to 00614 * the queue in interrupt context. 00615 * 00616 * "*out_Address" is NULL and any errors are not raised, if it becomed 00617 * to timeout and "Timeout_msec = 0". "E_TIME_OUT" is raised, 00618 * if "Timeout_msec != 0". 00619 * 00620 * Specify "Timeout_msec = 0", call the following functions by the following 00621 * order and use an event for preventing to block to receive other events 00622 * by the thread having waited for the queue. 00623 * 00624 * Sending Side 00625 * - R_OSPL_QUEUE_Allocate 00626 * - R_OSPL_QUEUE_Put 00627 * - R_OSPL_EVENT_Set 00628 * 00629 * Receiving Side 00630 * - R_OSPL_EVENT_Wait 00631 * - R_OSPL_QUEUE_Get 00632 * - R_OSPL_QUEUE_Free 00633 * 00634 * In OS less environment, "R_OSPL_QUEUE_Get" supports pseudo multi 00635 * threading. See "R_OSPL_THREAD_GetIsWaiting" function. 00636 */ 00637 errnum_t R_OSPL_QUEUE_Get( r_ospl_queue_t *self, void *out_Address, uint32_t Timeout_msec ); 00638 00639 00640 /** 00641 * @brief Releases the element to the queue object 00642 * 00643 * @param self A queue object 00644 * @param Address Address of received element 00645 * @return Error code. If there is no error, the return value is 0 00646 * 00647 * @par Description 00648 * It is correct, even if other thread put to the queue or get from 00649 * the queue from calling "R_OSPL_QUEUE_Get" to calling "R_OSPL_QUEUE_Free". 00650 */ 00651 errnum_t R_OSPL_QUEUE_Free( r_ospl_queue_t *self, void *Address ); 00652 00653 00654 /** 00655 * @brief Print status of the queue object 00656 * 00657 * @param self A queue object 00658 * @return Error code. If there is no error, the return value is 0 00659 */ 00660 #ifndef R_OSPL_NDEBUG 00661 errnum_t R_OSPL_QUEUE_Print( r_ospl_queue_t *self ); 00662 #endif 00663 00664 00665 /*********************************************************************** 00666 * Class: r_ospl_async_t 00667 ************************************************************************/ 00668 00669 /** 00670 * @brief CopyExceptAThread 00671 * 00672 * @param Source Source 00673 * @param Destination Destination 00674 * @return None 00675 */ 00676 void R_OSPL_ASYNC_CopyExceptAThread( const r_ospl_async_t *const Source, 00677 r_ospl_async_t *const Destination ); 00678 00679 00680 /*********************************************************************** 00681 * Class: r_ospl_caller_t 00682 ************************************************************************/ 00683 00684 /** 00685 * @brief Calls the interrupt callback function. It is called from OS porting layer in the driver 00686 * 00687 * @param self The internal parameters about interrupt operations 00688 * @param InterruptSource The source of the interrupt 00689 * @return None 00690 */ 00691 void R_OSPL_CallInterruptCallback( const r_ospl_caller_t *const self, 00692 const r_ospl_interrupt_t *const InterruptSource ); 00693 00694 00695 /** 00696 * @brief Initialize <r_ospl_caller_t>. 00697 * 00698 * @param self The internal parameters about interrupt operations 00699 * @param Async <r_ospl_async_t> 00700 * @return None 00701 */ 00702 void R_OSPL_CALLER_Initialize( r_ospl_caller_t *const self, r_ospl_async_t *const Async, 00703 volatile void *const PointerToState, int_t const StateValueOfOnInterrupting, 00704 void *const I_Lock, const r_ospl_i_lock_vtable_t *const I_LockVTable ); 00705 00706 00707 /** 00708 * @brief GetRootChannelNum. 00709 * 00710 * @param self The internal parameters about interrupt operations 00711 * @return RootChannelNum 00712 */ 00713 INLINE int_fast32_t R_OSPL_CALLER_GetRootChannelNum( const r_ospl_caller_t *const self ); 00714 00715 00716 /* Section: Interrupt */ 00717 /** 00718 * @brief Interrupt callback function for unregisterd interrupt. 00719 * 00720 * @param int_sense (See INTC driver) 00721 * @return None 00722 */ 00723 void R_OSPL_OnInterruptForUnregistered( uint32_t const int_sense ); 00724 00725 00726 /** 00727 * @brief Releases all disabled interrupts 00728 * 00729 * @par Parameters 00730 * None 00731 * @return None 00732 * 00733 * @par Description 00734 * Driver user should not call this function. 00735 * Call this function at the end of area of all interrupts disabled. 00736 * Do not release, if all interrupts was already disabled by caller function. 00737 * This function does not release disabled NMI. 00738 */ 00739 void R_OSPL_EnableAllInterrupt(void); 00740 00741 00742 /** 00743 * @brief Disables all interrupts 00744 * 00745 * @par Parameters 00746 * None 00747 * @return None 00748 * 00749 * @par Description 00750 * Driver user should not call this function. 00751 * Call this function at begin of area of all interrupts disabled. 00752 * This function does not disable NMI. 00753 * 00754 * @par Example 00755 * @code 00756 * void Func() 00757 * { 00758 * bool_t was_all_enabled = false; 00759 * 00760 * was_all_enabled = R_OSPL_DisableAllInterrupt(); 00761 * 00762 * // All interrupt disabled 00763 * 00764 * if ( was_all_enabled ) 00765 * { R_OSPL_EnableAllInterrupt(); } 00766 * } 00767 * @endcode 00768 */ 00769 bool_t R_OSPL_DisableAllInterrupt(void); 00770 00771 00772 /** 00773 * @brief Sets the priority of the interrupt line. 00774 * 00775 * @param IRQ_Num Interrupt request number 00776 * @param Priority Priority. The less the prior. 00777 * @return Error code. If there is no error, the return value is 0 00778 */ 00779 errnum_t R_OSPL_SetInterruptPriority( bsp_int_src_t const IRQ_Num, int_fast32_t const Priority ); 00780 00781 00782 /* Section: Locking channel */ 00783 /** 00784 * @brief Locks by channel number. 00785 * 00786 * @param ChannelNum Locking channel number or "R_OSPL_UNLOCKED_CHANNEL" 00787 * @param out_ChannelNum Output: Locked channel number, (in) NULL is permitted 00788 * @param HardwareIndexMin Hardware index of channel number = 0 00789 * @param HardwareIndexMax Hardware index of max channel number 00790 * @return Error code. If there is no error, the return value is 0 00791 * 00792 * @par Description 00793 * This function is called from the internal of "R_DRIVER_Initialize" 00794 * function or "R_DRIVER_LockChannel" function. 00795 * This function calls "R_BSP_HardwareLock". 00796 */ 00797 errnum_t R_OSPL_LockChannel( int_fast32_t ChannelNum, int_fast32_t *out_ChannelNum, 00798 mcu_lock_t HardwareIndexMin, mcu_lock_t HardwareIndexMax ); 00799 00800 00801 /** 00802 * @brief Unlocks by channel number. 00803 * 00804 * @param ChannelNum Channel number 00805 * @param e Raising error code, If there is no error, 0 00806 * @param HardwareIndexMin Hardware index of channel number = 0 00807 * @param HardwareIndexMax Hardware index of max channel number 00808 * @return Error code. If there is no error, the return value is 0 00809 * 00810 * @par Description 00811 * This function is called from the internal of "R_DRIVER_Finalize" 00812 * function or "R_DRIVER_UnlockChannel" function. 00813 * This function calls "R_BSP_HardwareUnlock". 00814 */ 00815 errnum_t R_OSPL_UnlockChannel( int_fast32_t ChannelNum, errnum_t e, 00816 mcu_lock_t HardwareIndexMin, mcu_lock_t HardwareIndexMax ); 00817 00818 00819 /*********************************************************************** 00820 * Class: r_ospl_c_lock_t 00821 ************************************************************************/ 00822 00823 /** 00824 * @brief Initializes the C-lock object 00825 * 00826 * @param self C-lock object 00827 * @return None 00828 * 00829 * @par Description 00830 * If *self is global variable or static variable initialized 0, 00831 * this function does not have to be called. 00832 */ 00833 void R_OSPL_C_LOCK_InitConst( r_ospl_c_lock_t *const self ); 00834 00835 00836 /** 00837 * @brief Locks the target, if lockable state. 00838 * 00839 * @param self C-lock object 00840 * @return Error code. If there is no error, the return value is 0. 00841 * 00842 * @par Description 00843 * Even if lock owner called this function, if lock object was already 00844 * locked, E_ACCESS_DENIED error is raised. 00845 * 00846 * "R_OSPL_C_LOCK_Lock" does not do exclusive control. 00847 */ 00848 errnum_t R_OSPL_C_LOCK_Lock( r_ospl_c_lock_t *const self ); 00849 00850 00851 /** 00852 * @brief Unlocks the target. 00853 * 00854 * @param self C-lock object 00855 * @return Error code. If there is no error, the return value is 0. 00856 * 00857 * @par Description 00858 * If this function was called with unlocked object, this function 00859 * does nothing and raises "E_ACCESS_DENIED" error. 00860 * 00861 * If self == NULL, this function does nothing and raises no error. 00862 * E_NOT_THREAD error is raised, if this function was called from the 00863 * interrupt context. 00864 * 00865 * - I - lock does not do in this function. 00866 * 00867 * "R_OSPL_C_LOCK_Unlock" does not do exclusive control. 00868 */ 00869 errnum_t R_OSPL_C_LOCK_Unlock( r_ospl_c_lock_t *const self ); 00870 00871 00872 /*********************************************************************** 00873 * Class: r_ospl_i_lock_vtable_t 00874 ************************************************************************/ 00875 00876 /** 00877 * @brief Do nothing. This is registered to r_ospl_i_lock_vtable_t::Lock. 00878 * 00879 * @param self_ I-lock object 00880 * @return false 00881 */ 00882 bool_t R_OSPL_I_LOCK_LockStub( void *const self_ ); 00883 00884 00885 /** 00886 * @brief Do nothing. This is registered to r_ospl_i_lock_vtable_t::Unlock. 00887 * 00888 * @param self_ I-lock object 00889 * @return None 00890 */ 00891 void R_OSPL_I_LOCK_UnlockStub( void *const self_ ); 00892 00893 00894 /** 00895 * @brief Do nothing. This is registered to r_ospl_i_lock_vtable_t::RequestFinalize. 00896 * 00897 * @param self_ I-lock object 00898 * @return None 00899 */ 00900 void R_OSPL_I_LOCK_RequestFinalizeStub( void *const self_ ); 00901 00902 00903 /** 00904 * @brief Get root channel number 00905 * 00906 * @param self <r_ospl_caller_t> object 00907 * @return Root channel number 00908 */ 00909 INLINE int_fast32_t R_OSPL_CALLER_GetRootChannelNum( const r_ospl_caller_t *const self ) 00910 { 00911 int_fast32_t root_channel_num; 00912 00913 IF_DQ( self == NULL ) { 00914 root_channel_num = 0; 00915 } 00916 else { 00917 root_channel_num = self->I_LockVTable->GetRootChannelNum( self->I_Lock ); 00918 } 00919 00920 return root_channel_num; 00921 } 00922 00923 00924 /* Section: Memory Operation */ 00925 /** 00926 * @brief Flushes cache memory 00927 * 00928 * @param FlushType The operation of flush 00929 * @return None 00930 * 00931 * @par Description 00932 * Call the function of the driver after flushing input output buffer 00933 * in the cache memory, If the data area accessing by the hardware is 00934 * on cache and the driver did not manage the cache memory. 00935 * Whether the driver manages the cache memory is depend on the driver 00936 * specification. 00937 */ 00938 void R_OSPL_MEMORY_Flush( r_ospl_flush_t const FlushType ); 00939 00940 00941 /** 00942 * @brief Flushes cache memory with the range of virtual address. 00943 * 00944 * @param FlushType The operation of flush 00945 * @return None 00946 * 00947 * @par Description 00948 * Align "StartAddress" argument and "Length" argument to cache line size. 00949 * If not aligned, E_OTHERS error is raised. 00950 * Refer to : R_OSPL_MEMORY_GetSpecification 00951 * 00952 * If the data area written by the hardware and read from CPU was in cache 00953 * rea, when the hardware started without invalidate 00954 * ("R_OSPL_FLUSH_WRITEBACK_INVALIDATE" or "R_OSPL_FLUSH_INVALIDATE"), 00955 * invalidate the data area and read it after finished to write by hardware. 00956 * (If the driver does not manage the cache memory.) 00957 */ 00958 errnum_t R_OSPL_MEMORY_RangeFlush( r_ospl_flush_t const FlushType, 00959 const void *const StartAddress, size_t const Length ); 00960 00961 00962 /** 00963 * @brief Gets the specification about memory and cache memory. 00964 * 00965 * @param out_MemorySpec The specification about memory and cache memory 00966 * @return None 00967 */ 00968 void R_OSPL_MEMORY_GetSpecification( r_ospl_memory_spec_t *const out_MemorySpec ); 00969 00970 00971 /** 00972 * @brief Set a memory barrier. 00973 * 00974 * @par Parameters 00975 * None 00976 * @return None 00977 * 00978 * @par Description 00979 * In ARM, This function calls DSB assembler operation. 00980 * This effects to L1 cache only. 00981 */ 00982 void R_OSPL_MEMORY_Barrier(void); 00983 00984 00985 /** 00986 * @brief Set a instruction barrier. 00987 * 00988 * @par Parameters 00989 * None 00990 * @return None 00991 * 00992 * @par Description 00993 * In ARM, This function calls ISB assembler operation. 00994 */ 00995 void R_OSPL_InstructionSyncBarrier(void); 00996 00997 00998 /** 00999 * @brief Changes to physical address 01000 * 01001 * @param Address Virtual address 01002 * @param out_PhysicalAddress Output: Physical address 01003 * @return Error code. If there is no error, the return value is 0. 01004 * 01005 * @par Description 01006 * This function must be modified by MMU setting. 01007 */ 01008 errnum_t R_OSPL_ToPhysicalAddress( const volatile void *const Address, uintptr_t *const out_PhysicalAddress ); 01009 01010 01011 /** 01012 * @brief Changes to the address in the L1 cache area 01013 * 01014 * @param Address Virtual address 01015 * @param out_CachedAddress Output: Virtual address for cached area 01016 * @return Error code. If there is no error, the return value is 0. 01017 * 01018 * @par Description 01019 * This function must be modified by MMU setting. 01020 * If "E_ACCESS_DENIED" error was raised, you may know the variable by 01021 * looking at value of "Address" argument and map file. 01022 */ 01023 errnum_t R_OSPL_ToCachedAddress( const volatile void *const Address, void *const out_CachedAddress ); 01024 01025 01026 /** 01027 * @brief Changes to the address in the L1 uncached area 01028 * 01029 * @param Address Virtual address 01030 * @param out_UncachedAddress Output: Virtual address for uncached area 01031 * @return Error code. If there is no error, the return value is 0. 01032 * 01033 * @par Description 01034 * This function must be modified by MMU setting. 01035 * If "E_ACCESS_DENIED" error was raised, you may know the variable by 01036 * looking at value of "Address" argument and map file. 01037 */ 01038 errnum_t R_OSPL_ToUncachedAddress( const volatile void *const Address, void *const out_UncachedAddress ); 01039 01040 01041 /** 01042 * @brief Gets the level of cache for flushing the memory indicated by the address. 01043 * 01044 * @param Address The address in flushing memory 01045 * @param out_Level Output: 0=Not need to flush, 1=L1 cache only, 2=both of L1 and L2 cache 01046 * @return Error code. If there is no error, the return value is 0. 01047 */ 01048 errnum_t R_OSPL_MEMORY_GetLevelOfFlush( const void *Address, int_fast32_t *out_Level ); 01049 01050 01051 /** 01052 * @brief Get 2nd cache attribute of AXI bus for peripheral (not CPU) from physical address. 01053 * 01054 * @param PhysicalAddress The physical address in the memory area 01055 * @param out_CacheAttribute Output: Cache_attribute, AWCACHE[3:0], ARCACHE[3:0] 01056 * @return Error code. If there is no error, the return value is 0. 01057 */ 01058 errnum_t R_OSPL_AXI_Get2ndCacheAttribute( uintptr_t const PhysicalAddress, 01059 r_ospl_axi_cache_attribute_t *const out_CacheAttribute ); 01060 01061 01062 /** 01063 * @brief Gets protection attribute of AXI bus from the address 01064 * 01065 * @param PhysicalAddress The physical address in the memory area 01066 * @param out_CacheAttribute Output: The protection attribute of AXI bus AWPROT[2:0], ARPROT[2:0] 01067 * @return Error code. If there is no error, the return value is 0. 01068 */ 01069 errnum_t R_OSPL_AXI_GetProtection( uintptr_t const physical_address, 01070 r_ospl_axi_protection_t *const out_protection ); 01071 01072 01073 /* Section: Timer */ 01074 /** 01075 * @brief Waits for a while until passed time 01076 * 01077 * @param DelayTime_msec Time of waiting (millisecond) 01078 * @return Error code. If there is no error, the return value is 0. 01079 * 01080 * @par Description 01081 * Maximum value is "R_OSPL_MAX_TIME_OUT" (=65533). 01082 */ 01083 errnum_t R_OSPL_Delay( uint32_t const DelayTime_msec ); 01084 01085 01086 /** 01087 * @brief Set up the free running timer 01088 * 01089 * @param out_Specification NULL is permitted. Output: The precision of the free run timer 01090 * @return Error code. If there is no error, the return value is 0. 01091 * 01092 * @par Description 01093 * The free running timer does not stop. 01094 * 01095 * If the counter of the free running timer was overflow, the counter returns to 0. 01096 * Even in interrupt handler, the counter does count up. 01097 * OSPL free running timer does not use any interrupt. 01098 * 01099 * Using timer can be selected by "R_OSPL_FTIMER_IS" macro. 01100 * 01101 * If the free running timer was already set up, this function does not set up it, 01102 * outputs to "out_Specification" argument and does not raise any error. 01103 * 01104 * When OSPL API function with timeout or "R_OSPL_Delay" function was called, 01105 * "R_OSPL_FTIMER_InitializeIfNot" function is callbacked from these functions. 01106 * 01107 * There is all interrupt disabled area inside. 01108 */ 01109 errnum_t R_OSPL_FTIMER_InitializeIfNot( r_ospl_ftimer_spec_t *const out_Specification ); 01110 01111 01112 /** 01113 * @brief Gets the specification of free running timer. 01114 * 01115 * @param out_Specification Output: The precision of the free run timer 01116 * @return None 01117 */ 01118 void R_OSPL_FTIMER_GetSpecification( r_ospl_ftimer_spec_t *const out_Specification ); 01119 01120 01121 /** 01122 * @brief Get current time of free running timer. 01123 * 01124 * @par Parameters 01125 * None 01126 * @return The current clock count of free run timer 01127 * 01128 * @par Description 01129 * Call "R_OSPL_FTIMER_InitializeIfNot" function before calling this function. 01130 * Call "R_OSPL_FTIMER_IsPast" function, when it is determined whether time passed. 01131 * 01132 * @par Example 01133 * @code 01134 * errnum_t e; 01135 * r_ospl_ftimer_spec_t ts; 01136 * uint32_t start; 01137 * uint32_t end; 01138 * 01139 * e= R_OSPL_FTIMER_InitializeIfNot( &ts ); IF(e){goto fin;} 01140 * start = R_OSPL_FTIMER_Get(); 01141 * 01142 * // The section of measuring 01143 * 01144 * end = R_OSPL_FTIMER_Get(); 01145 * printf( "%d msec\n", R_OSPL_FTIMER_CountToTime( 01146 * &ts, end - start ) ); 01147 * @endcode 01148 */ 01149 uint32_t R_OSPL_FTIMER_Get(void); 01150 01151 01152 /** 01153 * @brief Returns whether specified time was passed 01154 * 01155 * @param ts Precision of the free running timer 01156 * @param Now Count of current time 01157 * @param TargetTime Count of target time 01158 * @param out_IsPast Output: Whether the target time was past or not 01159 * @return Error code. If there is no error, the return value is 0. 01160 */ 01161 errnum_t R_OSPL_FTIMER_IsPast( const r_ospl_ftimer_spec_t *const ts, 01162 uint32_t const Now, uint32_t const TargetTime, bool_t *const out_IsPast ); 01163 01164 01165 /** 01166 * @brief Change from mili-second unit to free running timer unit 01167 * 01168 * @param ts Precision of the free running timer 01169 * @param msec The value of mili-second unit 01170 * @return The value of free running timer unit 01171 * 01172 * @par Description 01173 * The fractional part is been round up. (For waiting time must be more 01174 * than specified time.) 01175 * 01176 * This function calculates like the following formula. 01177 * @code 01178 * ( msec * ts->msec_Denominator + ts->msec_Numerator - 1 ) / ts->msec_Numerator 01179 * @endcode 01180 * 01181 * - Attention: If "ts - >msec_Denominator" was more than "ts->msec_Numerator", 01182 * take care of overflow. 01183 */ 01184 INLINE uint32_t R_OSPL_FTIMER_TimeToCount( const r_ospl_ftimer_spec_t *const ts, 01185 uint32_t const msec ) 01186 { 01187 uint32_t count; 01188 01189 IF_DQ( ts == NULL ) { 01190 count = 0; 01191 } 01192 else { 01193 count = ( ((msec * ts->msec_Denominator) + ts->msec_Numerator) - 1u ) / ts->msec_Numerator; 01194 } 01195 return count; 01196 } 01197 01198 01199 /** 01200 * @brief Change from free running timer unit to mili-second unit 01201 * 01202 * @param ts Precision of the free running timer 01203 * @param Count The value of free running timer unit 01204 * @return The value of mili-second unit 01205 * 01206 * @par Description 01207 * The fractional part is been round down. (Because overflow does not 01208 * occur, when "Count = r_ospl_ftimer_spec_t::MaxCount" ) 01209 * 01210 * This function calculates like the following formula. 01211 * @code 01212 * ( Count * ts->msec_Numerator ) / ts->msec_Denominator 01213 * @endcode 01214 */ 01215 INLINE uint32_t R_OSPL_FTIMER_CountToTime( const r_ospl_ftimer_spec_t *const ts, 01216 uint32_t const Count ) 01217 { 01218 uint32_t time; 01219 01220 IF_DQ( ts == NULL ) { 01221 time = 0; 01222 } 01223 else { 01224 time = ( Count * ts->msec_Numerator ) / ts->msec_Denominator; 01225 } 01226 return time; 01227 } 01228 01229 01230 /*********************************************************************** 01231 * Class: r_ospl_table_t 01232 ************************************************************************/ 01233 01234 /** 01235 * @brief Initializes an index table 01236 * 01237 * @param self Index table object 01238 * @param Area First address of the index table 01239 * @param AreaByteSize Size of the index table. See <R_OSPL_TABLE_SIZE> 01240 * @param Is_T_Lock Whether to call <R_OSPL_Start_T_Lock> 01241 * @return None 01242 */ 01243 void R_OSPL_TABLE_InitConst( r_ospl_table_t *const self, 01244 void *const Area, size_t const AreaByteSize, bool_t const Is_T_Lock ); 01245 01246 01247 /** 01248 * @brief Returns index from related key 01249 * 01250 * @param self Index table object 01251 * @param Key Key number 01252 * @param out_Index Output: Related index 01253 * @param TypeOfIfNot Behavior when key was not registerd. See <r_ospl_if_not_t> 01254 * @return Error code. If there is no error, the return value is 0. 01255 */ 01256 errnum_t R_OSPL_TABLE_GetIndex( r_ospl_table_t *const self, const void *const Key, 01257 int_fast32_t *const out_Index, r_ospl_if_not_t const TypeOfIfNot ); 01258 01259 01260 /** 01261 * @brief Separates relationship of specified key and related index 01262 * 01263 * @param self Index table object 01264 * @param Key Key number 01265 * @return None 01266 * 01267 * @par Description 01268 * Error is not raised, even if specified key was already separated. 01269 */ 01270 void R_OSPL_TABLE_Free( r_ospl_table_t *const self, const void *const Key ); 01271 01272 01273 /** 01274 * @brief Print status of specified index table object (for debug) 01275 * 01276 * @param self Index table object 01277 * @return None 01278 */ 01279 #if R_OSPL_DEBUG_TOOL 01280 void R_OSPL_TABLE_Print( r_ospl_table_t *const self ); 01281 #endif 01282 01283 01284 /* Section: Bit flags */ 01285 /** 01286 * @brief Evaluate whether any passed bits are 1 or not 01287 * 01288 * @param Variable The value of target bit flags 01289 * @param ConstValue The value that investigating bits are 1 01290 * @return Whether the any passed bit are 1 01291 */ 01292 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01293 #define IS_BIT_SET( Variable, ConstValue ) \ 01294 ( BIT_And_Sub( Variable, ConstValue ) != 0u ) 01295 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01296 01297 01298 /** 01299 * @brief Evaluate whether any passed bits are 1 or not 01300 * 01301 * @param Variable The value of target bit flags 01302 * @param OrConstValue The value that investigating bits are 1 01303 * @return Whether the any passed bit are 1 01304 */ 01305 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01306 #define IS_ANY_BITS_SET( Variable, OrConstValue ) \ 01307 ( BIT_And_Sub( Variable, OrConstValue ) != 0u ) 01308 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01309 01310 01311 /** 01312 * @brief Evaluate whether all passed bits are 1 or not 01313 * 01314 * @param Variable The value of target bit flags 01315 * @param OrConstValue The value that investigating bits are 1 01316 * @return Whether the all passed bit are 1 01317 */ 01318 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01319 #define IS_ALL_BITS_SET( Variable, OrConstValue ) \ 01320 ( BIT_And_Sub( Variable, OrConstValue ) == (OrConstValue) ) 01321 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01322 01323 01324 /** 01325 * @brief Evaluate whether the passed bit is 0 or not 01326 * 01327 * @param Variable The value of target bit flags 01328 * @param ConstValue The value that investigating bit is 1 01329 * @return Whether the passed bit is 0 01330 */ 01331 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01332 #define IS_BIT_NOT_SET( Variable, ConstValue ) \ 01333 ( BIT_And_Sub( Variable, ConstValue ) == 0u ) 01334 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01335 01336 01337 /** 01338 * @brief Evaluate whether any passed bits are 0 or not 01339 * 01340 * @param Variable The value of target bit flags 01341 * @param OrConstValue The value that investigating bits are 1 01342 * @return Whether the any passed bit are 0 01343 */ 01344 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01345 #define IS_ANY_BITS_NOT_SET( Variable, OrConstValue ) \ 01346 ( BIT_And_Sub( Variable, OrConstValue ) != (OrConstValue) ) 01347 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01348 01349 01350 /** 01351 * @brief Evaluate whether all passed bits are 0 or not 01352 * 01353 * @param Variable The value of target bit flags 01354 * @param OrConstValue The value that investigating bits are 1 01355 * @return Whether the all passed bit are 0 01356 */ 01357 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01358 #define IS_ALL_BITS_NOT_SET( Variable, OrConstValue ) \ 01359 ( BIT_And_Sub( Variable, OrConstValue ) == 0u ) 01360 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01361 01362 01363 /** 01364 * @brief Sub routine of bitwise operation 01365 * 01366 * @param Variable The value of target bit flags 01367 * @param ConstValue The value that investigating bits are 1 01368 * @return Whether the all passed bit are 0 01369 * 01370 * @par Description 01371 * - This part is for compliant to MISRA 2004 - 19.7. 01372 */ 01373 INLINE uint_fast32_t BIT_And_Sub( bit_flags_fast32_t const Variable, 01374 bit_flags_fast32_t const ConstValue ) 01375 { 01376 return ((Variable) & (ConstValue)); 01377 } 01378 01379 01380 /*********************************************************************** 01381 * About: IS_BIT_SET__Warning 01382 * 01383 * - This is for QAC-3344 warning : MISRA 13.2 Advice : Tests of a value against 01384 * zero should be made explicit, unless the operand is effectively Boolean. 01385 * - This is for QAC-1253 warning : SEC M1.2.2 : A "U" suffix shall be applied 01386 * to all constants of unsigned type. 01387 ************************************************************************/ 01388 01389 01390 /* Section: Error handling and debugging (2) */ 01391 /** 01392 * @brief Breaks here 01393 * 01394 * @par Parameters 01395 * None 01396 * @return None 01397 * 01398 * @par Description 01399 * Does break by calling "R_DebugBreak" function. 01400 * This macro is not influenced the setting of "R_OSPL_ERROR_BREAK" macro. 01401 */ 01402 #define R_DEBUG_BREAK() R_DebugBreak(__FILE__,__LINE__) 01403 01404 01405 /** 01406 * @brief The function callbacked from OSPL for breaking 01407 * 01408 * @param Variable The value of target bit flags 01409 * @param ConstValue The value that investigating bits are 1 01410 * @return Whether the all passed bit are 0 01411 * 01412 * @par Description 01413 * Set a break point at this function. 01414 * In Release configuration, "File = NULL, Line = 0". 01415 * If "File = NULL", "Line" argument is error code. 01416 * This function can be customized by application developer. 01417 */ 01418 void R_DebugBreak( const char_t *const File, int_fast32_t const Line ); 01419 01420 01421 /** 01422 * @brief Breaks here, if it is error state 01423 * 01424 * @par Parameters 01425 * None 01426 * @return None 01427 * 01428 * @par Description 01429 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01430 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01431 * macro was defined to be 1. 01432 * 01433 * Checks the error state of the current thread. 01434 * Call this macro from the last of each thread. 01435 * Does break by calling "R_DebugBreak" function. 01436 * 01437 * If an error was raised, this function calls "printf" with following message. 01438 * Set "error_ID" to "R_OSPL_SET_BREAK_ERROR_ID" 01439 * @code 01440 * <ERROR error_ID="0x1" file="../src/api.c(336)"/> 01441 * @endcode 01442 */ 01443 #if R_OSPL_ERROR_BREAK 01444 #define R_DEBUG_BREAK_IF_ERROR() R_OSPL_DebugBreakIfError(__FILE__,__LINE__) 01445 void R_OSPL_DebugBreakIfError( const char_t *const File, int_fast32_t const Line ); 01446 #else 01447 INLINE void R_DEBUG_BREAK_IF_ERROR(void) {} 01448 #endif 01449 01450 01451 /** 01452 * @brief Raises the error of system unrecoverable 01453 * 01454 * @param e Error code 01455 * @return None 01456 * 01457 * @par Description 01458 * The error of system unrecoverable is the error of impossible to 01459 * - self - recover by process or main system. Example, the heap area was 01460 * broken or there are not any responses from hardware. This error can 01461 * be recoverable by OS or the system controller(e.g. Software reset) 01462 * 01463 * Example, when an error of recovery process was raised, 01464 * "R_OSPL_RaiseUnrecoverable" function must be called. 01465 * 01466 * "R_OSPL_RaiseUnrecoverable" function can be customized by the 01467 * application. By default, it calls "R_DebugBreak" function and falls 01468 * into the infinite loop. 01469 */ 01470 void R_OSPL_RaiseUnrecoverable( errnum_t const e ); 01471 01472 01473 /** 01474 * @brief Merge the error code raised in the finalizing operation 01475 * 01476 * @param CurrentError Current error code 01477 * @param AppendError New append error code 01478 * @return Merged error code 01479 * 01480 * @par Description 01481 * When the state was error state, if other new error was raised, 01482 * new error code is ignored. 01483 * - If "CurrentError != 0", this function returns "CurrentError" argument. 01484 * - If "CurrentError == 0", this function returns "AppendError" argument. 01485 * 01486 * This function can be modify by user. 01487 * 01488 * @par Example 01489 * @code 01490 * ee= Sample(); 01491 * e= R_OSPL_MergeErrNum( e, ee ); 01492 * return e; 01493 * @endcode 01494 */ 01495 INLINE errnum_t R_OSPL_MergeErrNum( errnum_t const CurrentError, errnum_t const AppendError ) 01496 { 01497 errnum_t e; 01498 01499 if ( CurrentError != 0 ) { 01500 e = CurrentError; 01501 } else { 01502 e = AppendError; 01503 } 01504 return e; 01505 } 01506 01507 01508 /** 01509 * @brief Sets an error code to TLS (Thread Local Storage). 01510 * 01511 * @param e Raising error code 01512 * @return None 01513 * 01514 * @par Description 01515 * Usually error code is returned. If API function cannot return any 01516 * error code, API function can have the specification of setting error 01517 * code by "R_OSPL_SetErrNum". 01518 * 01519 * There is this function, if "R_OSPL_TLS_ERROR_CODE" macro was defined 01520 * to be 1. 01521 * This function does nothing, if any error code was stored already in TLS. 01522 * The state does not change to error state, if "R_OSPL_SetErrNum" function 01523 * was called only. See "R_OSPL_GET_ERROR_ID". 01524 */ 01525 #if R_OSPL_TLS_ERROR_CODE 01526 void R_OSPL_SetErrNum( errnum_t const e ); 01527 #endif 01528 01529 01530 /** 01531 * @brief Returns the error code from TLS (Thread Local Storage). 01532 * 01533 * @par Parameters 01534 * None 01535 * @return Error code 01536 * 01537 * @par Description 01538 * Usually error code is returned. If API function cannot return any 01539 * error code, API function may have the specification of getting error 01540 * code by "R_OSPL_GetErrNum". 01541 * 01542 * There is this function, if "R_OSPL_TLS_ERROR_CODE" macro was defined 01543 * to be 1. This function returns 0 after called "R_OSPL_CLEAR_ERROR" 01544 * function. 01545 */ 01546 #if R_OSPL_TLS_ERROR_CODE 01547 errnum_t R_OSPL_GetErrNum(void); 01548 #endif 01549 01550 01551 /** 01552 * @brief Clears the error state 01553 * 01554 * @par Parameters 01555 * None 01556 * @return None 01557 * 01558 * @par Description 01559 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro and 01560 * "R_OSPL_TLS_ERROR_CODE" macro were defined to be 0. The following 01561 * descriptions are available, if "R_OSPL_ERROR_BREAK" macro was 01562 * defined to be 1. 01563 * 01564 * Whether the state is the error state is stored in thread local 01565 * storage. "R_OSPL_GetErrNum" function returns 0 after called this 01566 * function. 01567 * 01568 * If the error state was not cleared, the following descriptions were caused. 01569 * - Breaks at "R_DEBUG_BREAK_IF_ERROR" macro 01570 * - "R_OSPL_SET_BREAK_ERROR_ID" function behaves not expected behavior 01571 * because the count of error is not counted up. 01572 */ 01573 #if R_OSPL_ERROR_BREAK || R_OSPL_TLS_ERROR_CODE 01574 void R_OSPL_CLEAR_ERROR(void); 01575 #else 01576 INLINE void R_OSPL_CLEAR_ERROR(void) {} /* QAC 3138 */ 01577 #endif 01578 01579 01580 /** 01581 * @brief Returns the number of current error 01582 * 01583 * @par Parameters 01584 * None 01585 * @return The number of current error 01586 * 01587 * @par Description 01588 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01589 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01590 * macro was defined to be 1. 01591 * 01592 * This function returns 0, if any errors were not raised. 01593 * 01594 * This function returns 1, if first error was raised. 01595 * 01596 * After that, this function returns 2, if second error was raised after 01597 * calling "R_OSPL_CLEAR_ERROR" function. 01598 * This function does not return 0 after that the error was cleared by 01599 * calling "R_OSPL_CLEAR_ERROR". 01600 * The number of current error is running number in the whole of system 01601 * (all threads). 01602 * 01603 * Error is raised by following macros. 01604 * @code 01605 * IF, IF_D, ASSERT_R, ASSERT_D 01606 * @endcode 01607 * The process breaks at a moment of error raised, if the number of current 01608 * error was set to "R_OSPL_SET_BREAK_ERROR_ID" macro. 01609 */ 01610 #if R_OSPL_ERROR_BREAK 01611 int_fast32_t R_OSPL_GET_ERROR_ID(void); 01612 #else 01613 INLINE int_fast32_t R_OSPL_GET_ERROR_ID(void) 01614 { 01615 return -1; 01616 } 01617 #endif 01618 01619 01620 /** 01621 * @brief Register to break at raising error at the moment 01622 * 01623 * @param ID Breaking number of error 01624 * @return None 01625 * 01626 * @par Description 01627 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01628 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01629 * macro was defined to be 1. 01630 * 01631 * Set a break point at "R_DebugBreak" function, when the process breaks 01632 * at the error raised code. 01633 * 01634 * The number of "ErrorID" argument can be known by "R_DEBUG_BREAK_IF_ERROR" 01635 * macro or "R_OSPL_GET_ERROR_ID" macro. 01636 * - In multi - threading environment, the number of "ErrorID" argument is the 01637 * number of raised errors in all threads. But when "ErrorID" argument was 01638 * set to be over 2, call "R_OSPL_SET_DEBUG_WORK" function before calling 01639 * "R_OSPL_SET_BREAK_ERROR_ID" function. 01640 * 01641 * The following code breaks at first error. 01642 * @code 01643 * R_OSPL_SET_BREAK_ERROR_ID( 1 ); 01644 * @endcode 01645 * 01646 * The following code breaks at next error after resuming from meny errors. 01647 * @code 01648 * R_OSPL_SET_BREAK_ERROR_ID( R_OSPL_GET_ERROR_ID() + 1 ); 01649 * @endcode 01650 */ 01651 #if R_OSPL_ERROR_BREAK 01652 void R_OSPL_SET_BREAK_ERROR_ID( int_fast32_t ID ); 01653 #else 01654 INLINE void R_OSPL_SET_BREAK_ERROR_ID( int_fast32_t const ID ) 01655 { 01656 R_UNREFERENCED_VARIABLE( ID ); 01657 } 01658 #endif 01659 01660 01661 /** 01662 * @brief Set the debug work area 01663 * 01664 * @param WorkArea Start address of work area 01665 * @param WorkAreaSize Size of work area (byte). See. <R_OSPL_DEBUG_WORK_SIZE> 01666 * @return None 01667 * 01668 * @par Description 01669 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01670 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01671 * macro was defined to be 1. 01672 * 01673 * Set the debug work area, when "R_OSPL_SET_BREAK_ERROR_ID" function 01674 * supports multi thread. "E_NO_DEBUG_TLS" error is raised, if the debug 01675 * work area was not set, when errors was raised in 2 or more threads. 01676 * It is not necessary to call this function, if error handling did by one 01677 * thread only. 01678 * 01679 * @par Example 01680 * @code 01681 * #if R_OSPL_ERROR_BREAK 01682 * #define GS_MAX_THREAD 10 01683 * static uint8_t gs_DebugWorkArea[ R_OSPL_DEBUG_WORK_SIZE( GS_MAX_THREAD ) ]; 01684 * #endif 01685 * 01686 * R_OSPL_SET_DEBUG_WORK( gs_DebugWorkArea, sizeof(gs_DebugWorkArea) ); 01687 * @endcode 01688 */ 01689 #if R_OSPL_ERROR_BREAK 01690 void R_OSPL_SET_DEBUG_WORK( void *WorkArea, uint32_t WorkAreaSize ); 01691 #else 01692 INLINE void R_OSPL_SET_DEBUG_WORK( const void *const WorkArea, uint32_t const WorkAreaSize ) 01693 { 01694 R_UNREFERENCED_VARIABLE_2( WorkArea, WorkAreaSize ); 01695 } 01696 #endif 01697 01698 01699 /** 01700 * @brief Returns debbug information of current thread. 01701 * 01702 * @par Parameters 01703 * None 01704 * @return Debbug information of current thread. 01705 */ 01706 #if R_OSPL_ERROR_BREAK 01707 r_ospl_error_t *R_OSPL_GetCurrentThreadError(void); 01708 #endif 01709 01710 01711 /** 01712 * @brief Modifies count of objects that current thread has locked. 01713 * 01714 * @param Plus The value of adding to the counter. 01715 * @return None 01716 * 01717 * @par Description 01718 * The counter is subtracted, if this argument was minus. 01719 * 01720 * Drivers calls this function. 01721 * This function is not called from OSPL. 01722 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro is 0. 01723 */ 01724 #if R_OSPL_ERROR_BREAK 01725 #if R_OSPL_IS_PREEMPTION 01726 void R_OSPL_MODIFY_THREAD_LOCKED_COUNT( int_fast32_t Plus ); 01727 #else 01728 INLINE void R_OSPL_MODIFY_THREAD_LOCKED_COUNT( int_fast32_t Plus ) {} 01729 #endif 01730 #else 01731 INLINE void R_OSPL_MODIFY_THREAD_LOCKED_COUNT( int_fast32_t Plus ) {} 01732 #endif 01733 01734 01735 /** 01736 * @brief Returns count of objects that current thread has locked. 01737 * 01738 * @par Parameters 01739 * None 01740 * @return Count of objects that current thread has locked 01741 * 01742 * @par Description 01743 * This function returns 0, if "R_OSPL_ERROR_BREAK" macro is 0. 01744 */ 01745 #if R_OSPL_ERROR_BREAK 01746 #if R_OSPL_IS_PREEMPTION 01747 int_fast32_t R_OSPL_GET_THREAD_LOCKED_COUNT(void); 01748 #else 01749 INLINE int_fast32_t R_OSPL_GET_THREAD_LOCKED_COUNT(void) 01750 { 01751 return 0; 01752 } 01753 #endif 01754 #else 01755 INLINE int_fast32_t R_OSPL_GET_THREAD_LOCKED_COUNT(void) 01756 { 01757 return 0; 01758 } 01759 #endif 01760 01761 01762 /* Section: Accessing to register bit field */ 01763 /** 01764 * @brief Reads modifies writes for bit field of 32bit register. 01765 * 01766 * @param in_out_Register Address of accessing register 01767 * @param Mask Mask of accessing bit field 01768 * @param Shift Shift count. Lowest bit number 01769 * @param Value Writing value before shift to the bit field 01770 * @return None 01771 */ 01772 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01773 01774 /* ->SEC R3.6.2(QAC-3345) */ 01775 /* Volatile access at left of "=" and right of "=". But this is not depend on compiler spcifications. */ 01776 /* ->SEC M1.2.2(QAC-1259) */ 01777 /* If "Value" is signed, this is depend on CPU bit width. This expects 32bit CPU. But driver code is no problem. */ 01778 01779 #define R_OSPL_SET_TO_32_BIT_REGISTER( in_out_Register, Mask, Shift, Value ) \ 01780 ( *(volatile uint32_t*)(in_out_Register) = (uint32_t)( \ 01781 ( ((uint32_t) *(volatile uint32_t*)(in_out_Register)) & \ 01782 ~(Mask) ) | ( (Mask) & ( ( (uint_fast32_t)(Value) << (Shift) ) & (Mask) ) ) ) ) 01783 /* This code is optimized well. */ 01784 01785 /* <-SEC M1.2.2(QAC-1259) */ 01786 /* <-SEC R3.6.2(QAC-3345) */ 01787 01788 #else 01789 01790 INLINE void R_OSPL_SET_TO_32_BIT_REGISTER( volatile uint32_t *const Register, 01791 uint32_t const Mask, int_fast32_t const Shift, uint32_t const Value ) 01792 { 01793 uint32_t reg_value; 01794 01795 IF_DQ ( Register == NULL ) {} 01796 else { 01797 reg_value = *Register; 01798 reg_value = ( reg_value & ~Mask ) | ( ( Value << Shift ) & Mask ); 01799 *Register = reg_value; 01800 } 01801 } 01802 01803 #endif 01804 01805 01806 /** 01807 * @brief Reads modifies writes for bit field of 16bit register. 01808 * 01809 * @param in_out_Register Address of accessing register 01810 * @param Mask Mask of accessing bit field 01811 * @param Shift Shift count. Lowest bit number 01812 * @param Value Writing value before shift to the bit field 01813 * @return None 01814 */ 01815 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01816 01817 /* ->SEC R3.6.2(QAC-3345) */ 01818 /* Volatile access at left of "=" and right of "=". But this is not depend on compiler spcifications. */ 01819 /* ->SEC M1.2.2(QAC-1259) */ 01820 /* If "Value" is signed, this is depend on CPU bit width. This expects 32bit CPU. But driver code is no problem. */ 01821 01822 #define R_OSPL_SET_TO_16_BIT_REGISTER( in_out_Register, Mask, Shift, Value ) \ 01823 ( *(volatile uint16_t*)(in_out_Register) = (uint16_t)( \ 01824 ( ((uint16_t) *(volatile uint16_t*)(in_out_Register)) & \ 01825 ~(Mask) ) | ( (Mask) & ( ( (uint_fast16_t)(Value) << (Shift) ) & (Mask) ) ) ) ) 01826 /* This code is optimized well. */ 01827 01828 01829 /* <-SEC M1.2.2(QAC-1259) */ 01830 /* <-SEC R3.6.2(QAC-3345) */ 01831 01832 #else 01833 01834 INLINE void R_OSPL_SET_TO_16_BIT_REGISTER( volatile uint16_t *const Register, 01835 uint16_t const Mask, int_fast32_t const Shift, uint16_t const Value ) 01836 { 01837 uint16_t reg_value; 01838 01839 IF_DQ ( Register == NULL ) {} 01840 else { 01841 reg_value = *Register; 01842 reg_value = (uint16_t)( ( (uint_fast32_t) reg_value & ~(uint_fast32_t) Mask ) | 01843 ( ( (uint_fast32_t) Value << Shift ) & (uint_fast32_t) Mask ) ); 01844 /* Cast is for SEC R2.4.2 */ 01845 *Register = reg_value; 01846 } 01847 } 01848 01849 #endif 01850 01851 01852 /** 01853 * @brief Reads modifies writes for bit field of 8bit register. 01854 * 01855 * @param in_out_Register Address of accessing register 01856 * @param Mask Mask of accessing bit field 01857 * @param Shift Shift count. Lowest bit number 01858 * @param Value Writing value before shift to the bit field 01859 * @return None 01860 */ 01861 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01862 01863 /* ->SEC R3.6.2(QAC-3345) */ 01864 /* Volatile access at left of "=" and right of "=". But this is not depend on compiler spcifications. */ 01865 /* ->SEC M1.2.2(QAC-1259) */ 01866 /* If "Value" is signed, this is depend on CPU bit width. This expects 32bit CPU. But driver code is no problem. */ 01867 01868 01869 #define R_OSPL_SET_TO_8_BIT_REGISTER( in_out_Register, Mask, Shift, Value ) \ 01870 ( *(volatile uint8_t*)(in_out_Register) = (uint8_t)( \ 01871 ( ((uint8_t) *(volatile uint8_t*)(in_out_Register)) & \ 01872 ~(Mask) ) | ( (Mask) & ( ( (uint_fast8_t)(Value) << (Shift) ) & (Mask) ) ) ) ) 01873 /* This code is optimized well. */ 01874 01875 /* <-SEC M1.2.2(QAC-1259) */ 01876 /* <-SEC R3.6.2(QAC-3345) */ 01877 01878 #else 01879 01880 INLINE void R_OSPL_SET_TO_8_BIT_REGISTER( volatile uint8_t *const Register, 01881 uint8_t const Mask, int_fast32_t const Shift, uint8_t const Value ) 01882 { 01883 uint8_t reg_value; 01884 01885 IF_DQ ( Register == NULL ) {} 01886 else { 01887 reg_value = *Register; 01888 reg_value = (uint8_t)( ( (uint_fast32_t) reg_value & ~(uint_fast32_t) Mask ) | 01889 ( ( (uint_fast32_t) Value << Shift ) & (uint_fast32_t) Mask ) ); 01890 /* Cast is for SEC R2.4.2 */ 01891 *Register = reg_value; 01892 } 01893 } 01894 01895 #endif 01896 01897 01898 /** 01899 * @brief Reads for bit field of 32bit register. 01900 * 01901 * @param RegisterValueAddress Address of accessing register 01902 * @param Mask Mask of accessing bit field 01903 * @param Shift Shift count. Lowest bit number 01904 * @return Read value after shift 01905 */ 01906 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01907 01908 /* ->SEC R3.6.2(QAC-3345) */ 01909 /* Volatile access at &(get address), cast and *(memory load). But this is not double volatile access. */ 01910 /* RegisterValueAddress is for avoid QAC-0310,QAC-3345 by cast code at caller. */ 01911 01912 #define R_OSPL_GET_FROM_32_BIT_REGISTER( RegisterValueAddress, Mask, Shift ) \ 01913 ( (uint32_t)( ( (uint32_t)*(volatile const uint32_t*) (RegisterValueAddress) \ 01914 & (uint_fast32_t)(Mask) ) >> (Shift) ) ) 01915 /* This code is optimized well. */ 01916 01917 /* <-SEC R3.6.2(QAC-3345) */ 01918 01919 #else /* __QAC_ARM_H__ */ /* This code must be tested defined "__QAC_ARM_H__" */ 01920 01921 01922 /* This inline functions is not expanded on __CC_ARM 5.15 */ 01923 INLINE uint32_t R_OSPL_GET_FROM_32_BIT_REGISTER( volatile const uint32_t *const RegisterAddress, 01924 uint32_t const Mask, int_fast32_t const Shift ) 01925 { 01926 uint32_t reg_value; 01927 01928 IF_DQ ( RegisterAddress == NULL ) { 01929 enum { num = 0x0EDEDEDE }; /* SEC M1.10.1 */ 01930 reg_value = num; 01931 } 01932 else { 01933 reg_value = *RegisterAddress; 01934 reg_value = ( reg_value & Mask ) >> Shift; 01935 } 01936 return reg_value; 01937 } 01938 01939 #endif 01940 01941 01942 /** 01943 * @brief Reads for bit field of 16bit register. 01944 * 01945 * @param RegisterValueAddress Address of accessing register 01946 * @param Mask Mask of accessing bit field 01947 * @param Shift Shift count. Lowest bit number 01948 * @return Read value after shift 01949 */ 01950 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01951 01952 /* ->SEC R3.6.2(QAC-3345) */ 01953 /* Volatile access at &(get address), cast and *(memory load). But this is not double volatile access. */ 01954 /* RegisterValueAddress is for avoid QAC-0310,QAC-3345 by cast code at caller. */ 01955 01956 #define R_OSPL_GET_FROM_16_BIT_REGISTER( RegisterValueAddress, Mask, Shift ) \ 01957 ( (uint16_t)( ( (uint_fast32_t)*(volatile const uint16_t*) (RegisterValueAddress) \ 01958 & (uint_fast16_t)(Mask) ) >> (Shift) ) ) 01959 /* This code is optimized well. */ 01960 01961 /* <-SEC R3.6.2(QAC-3345) */ 01962 01963 #else /* __QAC_ARM_H__ */ /* This code must be tested defined "__QAC_ARM_H__" */ 01964 01965 /* This inline functions is not expanded on __CC_ARM 5.15 */ 01966 INLINE uint16_t R_OSPL_GET_FROM_16_BIT_REGISTER( volatile const uint16_t *const RegisterAddress, 01967 uint16_t const Mask, int_fast32_t const Shift ) 01968 { 01969 uint16_t reg_value; 01970 01971 IF_DQ ( RegisterAddress == NULL ) { 01972 enum { num = 0xDEDE }; /* SEC M1.10.1 */ 01973 reg_value = num; 01974 } 01975 else { 01976 reg_value = *RegisterAddress; 01977 reg_value = (uint16_t)( ( (uint_fast32_t) reg_value & (uint_fast32_t) Mask ) >> Shift ); 01978 /* Cast is for SEC R2.4.2 */ 01979 } 01980 return reg_value; 01981 } 01982 01983 #endif 01984 01985 01986 /** 01987 * @brief Reads for bit field of 8bit register. 01988 * 01989 * @param RegisterValueAddress Address of accessing register 01990 * @param Mask Mask of accessing bit field 01991 * @param Shift Shift count. Lowest bit number 01992 * @return Read value after shift 01993 */ 01994 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01995 01996 /* ->SEC R3.6.2(QAC-3345) */ 01997 /* Volatile access at &(get address), cast and *(memory load). But this is not double volatile access. */ 01998 /* RegisterValueAddress is for avoid QAC-0310,QAC-3345 by cast code at caller. */ 01999 02000 #define R_OSPL_GET_FROM_8_BIT_REGISTER( RegisterValueAddress, Mask, Shift ) \ 02001 ( (uint8_t)( ( (uint_fast32_t)*(volatile const uint8_t*) (RegisterValueAddress) \ 02002 & (uint_fast8_t)(Mask) ) >> (Shift) ) ) 02003 /* This code is optimized well. */ 02004 02005 /* <-SEC R3.6.2(QAC-3345) */ 02006 02007 #else /* __QAC_ARM_H__ */ /* This code must be tested defined "__QAC_ARM_H__" */ 02008 02009 /* This inline functions is not expanded on __CC_ARM 5.15 */ 02010 INLINE uint8_t R_OSPL_GET_FROM_8_BIT_REGISTER( volatile const uint8_t *const RegisterAddress, 02011 uint8_t const Mask, int_fast32_t const Shift ) 02012 { 02013 uint8_t reg_value; 02014 02015 IF_DQ ( RegisterAddress == NULL ) { 02016 enum { num = 0xDE }; /* SEC M1.10.1 */ 02017 reg_value = num; 02018 } 02019 else { 02020 reg_value = *RegisterAddress; 02021 reg_value = (uint8_t)( ( (uint_fast32_t) reg_value & (uint_fast32_t) Mask ) >> Shift ); 02022 /* Cast is for SEC R2.4.2 */ 02023 } 02024 return reg_value; 02025 } 02026 02027 #endif 02028 02029 02030 /*********************************************************************** 02031 * End of File: 02032 ************************************************************************/ 02033 02034 #ifdef __cplusplus 02035 } /* extern "C" */ 02036 #endif /* __cplusplus */ 02037 02038 #endif /* R_OSPL_H */ 02039
Generated on Tue Jul 12 2022 11:15:03 by
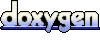