Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
JPEG_Converter Class Reference
A class to communicate a JCU. More...
#include <JPEG_Converter.h>
Data Structures | |
struct | bitmap_buff_info_t |
Bitmap data setting struct. More... | |
struct | encode_options_t |
Encode option setting. More... | |
Public Types | |
enum | jpeg_conv_error_t { JPEG_CONV_OK = 0, JPEG_CONV_JCU_ERR = -1, JPEG_CONV_FORMA_ERR = -2, JPEG_CONV_PARAM_ERR = -3, JPEG_CONV_BUSY = -4, JPEG_CONV_PARAM_RANGE_ERR = -7 } |
Error codes. More... | |
enum | wr_rd_swa_t { WR_RD_WRSWA_NON = 0, WR_RD_WRSWA_8BIT = 1, WR_RD_WRSWA_16BIT = 2, WR_RD_WRSWA_16_8BIT = 3, WR_RD_WRSWA_32BIT = 4, WR_RD_WRSWA_32_8BIT = 5, WR_RD_WRSWA_32_16BIT = 6, WR_RD_WRSWA_32_16_8BIT = 7 } |
Write/Read image pixcel frame buffer swap setting. More... | |
enum | wr_rd_format_t { WR_RD_YCbCr422 = 0x00, WR_RD_ARGB8888 = 0x01, WR_RD_RGB565 = 0x02 } |
Write/Read image pixcel format selects. More... | |
enum | sub_sampling_t { SUB_SAMPLING_1_1 = 0x00, SUB_SAMPLING_1_2 = 0x01, SUB_SAMPLING_1_4 = 0x02, SUB_SAMPLING_1_8 = 0x03 } |
Thinning output image selects. More... | |
enum | cbcr_offset_t { CBCR_OFFSET_0 = 0x00, CBCR_OFFSET_128 = 0x01 } |
Cb/Cr range selects for decode. More... | |
Public Member Functions | |
JPEG_Converter () | |
Constructor method of JPEG converter(encode/decode) | |
virtual | ~JPEG_Converter () |
Destructor method of JPEG converter(encode/decode) | |
JPEG_Converter::jpeg_conv_error_t | decode (void *pJpegBuff, bitmap_buff_info_t *psOutputBuff) |
Decode JPEG to rinear data. | |
JPEG_Converter::jpeg_conv_error_t | decode (void *pJpegBuff, bitmap_buff_info_t *psOutputBuff, decode_options_t *pOptions) |
JPEG data decode to bitmap. | |
JPEG_Converter::jpeg_conv_error_t | encode (bitmap_buff_info_t *psInputBuff, void *pJpegBuff, size_t *pEncodeSize) |
Encode rinear data to JPEG. | |
JPEG_Converter::jpeg_conv_error_t | encode (bitmap_buff_info_t *psInputBuff, void *pJpegBuff, size_t *pEncodeSize, encode_options_t *pOptions) |
Bitmap data encode to JPEG. | |
JPEG_Converter::jpeg_conv_error_t | SetQuality (const uint8_t qual) |
Set encode quality. |
Detailed Description
A class to communicate a JCU.
Definition at line 42 of file JPEG_Converter.h.
Member Enumeration Documentation
enum cbcr_offset_t |
Cb/Cr range selects for decode.
- Enumerator:
CBCR_OFFSET_0 Cb/Cr range -128 to 127 (all format used)
CBCR_OFFSET_128 Cb/Cr range 0 to 255 (Default and YCbCr format only used)
Definition at line 93 of file JPEG_Converter.h.
enum jpeg_conv_error_t |
Error codes.
- Enumerator:
Definition at line 48 of file JPEG_Converter.h.
enum sub_sampling_t |
Thinning output image selects.
- Enumerator:
SUB_SAMPLING_1_1 Thinning output image to 1/1
SUB_SAMPLING_1_2 Thinning output image to 1/2
SUB_SAMPLING_1_4 Thinning output image to 1/4
SUB_SAMPLING_1_8 Thinning output image to 1/8
Definition at line 83 of file JPEG_Converter.h.
enum wr_rd_format_t |
Write/Read image pixcel format selects.
- Enumerator:
WR_RD_YCbCr422 YCbCr422 (2byte / px)
WR_RD_ARGB8888 ARGB8888 (4byte / px)
WR_RD_RGB565 RGB565 (2byte / px)
Definition at line 74 of file JPEG_Converter.h.
enum wr_rd_swa_t |
Write/Read image pixcel frame buffer swap setting.
- Enumerator:
Definition at line 60 of file JPEG_Converter.h.
Constructor & Destructor Documentation
JPEG_Converter | ( | void | ) |
Constructor method of JPEG converter(encode/decode)
Constructor of the JPEG_Converter class.
- Parameters:
-
[in] None
- Return values:
-
None
Definition at line 227 of file JPEG_Coverter.cpp.
~JPEG_Converter | ( | void | ) | [virtual] |
Destructor method of JPEG converter(encode/decode)
Destructor of the JPEG_Converter class.
- Parameters:
-
[in] None
- Return values:
-
None
Definition at line 247 of file JPEG_Coverter.cpp.
Member Function Documentation
JPEG_Converter::jpeg_conv_error_t decode | ( | void * | pJpegBuff, |
bitmap_buff_info_t * | psOutputBuff | ||
) |
Decode JPEG to rinear data.
JPEG data decode to bitmap.
- Parameters:
-
[in] void* pJpegBuff : Input JPEG data address in/out] bitmap_buff_info_t* psOutputBuff : Output bitmap data address [in] decode_options_t* pOptions : Decode option(Optional)
- Returns:
- JPEG_CONV_OK = success JPEG_CONV_JCU_ERR = failure (JCU error) JPEG_CONV_FORMA_ERR = failure (data format error) JPEG_CONV_PARAM_ERR = failure (input parameter error) JPEG_CONV_PARAM_RANGE_ERR = failure (input parameter range error)
- Parameters:
-
[in] void* pJpegBuff : Input JPEG data address in/out] bitmap_buff_info_t* psOutputBuff : Output bitmap data address
- Return values:
-
error code
Definition at line 263 of file JPEG_Coverter.cpp.
JPEG_Converter::jpeg_conv_error_t decode | ( | void * | pJpegBuff, |
bitmap_buff_info_t * | psOutputBuff, | ||
decode_options_t * | pOptions | ||
) |
JPEG data decode to bitmap.
- Parameters:
-
[in] void* pJpegBuff : Input JPEG data address in/out] bitmap_buff_info_t* psOutputBuff : Output bitmap data address [in] decode_options_t* pOptions : Decode option(Optional)
- Return values:
-
error code
Definition at line 277 of file JPEG_Coverter.cpp.
JPEG_Converter::jpeg_conv_error_t encode | ( | bitmap_buff_info_t * | psInputBuff, |
void * | pJpegBuff, | ||
size_t * | pEncodeSize, | ||
encode_options_t * | pOptions | ||
) |
Bitmap data encode to JPEG.
- Parameters:
-
[in] bitmap_buff_info_t* psInputBuff : Input bitmap data address [out] void* pJpegBuff : Output JPEG data address [out] size_t* pEncodeSize : Encode size address [in] encode_options_t* pOptions : Encode option(Optional)
- Return values:
-
error code
Definition at line 453 of file JPEG_Coverter.cpp.
JPEG_Converter::jpeg_conv_error_t encode | ( | bitmap_buff_info_t * | psInputBuff, |
void * | pJpegBuff, | ||
size_t * | pEncodeSize | ||
) |
Encode rinear data to JPEG.
Bitmap data encode to JPEG.
- Parameters:
-
[in] bitmap_buff_info_t* psInputBuff : Input bitmap data address [out] void* pJpegBuff : Output JPEG data address [out] size_t* pEncodeSize : Encode size address [in] encode_options_t* pOptions[IN] : Encode option(Optional)
- Returns:
- JPEG_CONV_OK = success JPEG_CONV_JCU_ERR = failure (JCU error) JPEG_CONV_FORMA_ERR = failure (data format error) JPEG_CONV_PARAM_ERR = failure (input parameter error) JPEG_CONV_PARAM_RANGE_ERR = failure (input parameter range error)
- Parameters:
-
[in] bitmap_buff_info_t* psInputBuff : Input bitmap data address [out] void* pJpegBuff : Output JPEG data address [out] size_t* pEncodeSize : Encode size address
- Return values:
-
error code
Definition at line 437 of file JPEG_Coverter.cpp.
JPEG_Converter::jpeg_conv_error_t SetQuality | ( | const uint8_t | qual ) |
Set encode quality.
- Parameters:
-
[in] uint8_t qual : Encode quality (1 <= qual <= 100)
- Returns:
- JPEG_CONV_OK = success JPEG_CONV_PARAM_RANGE_ERR = failure (input parameter range error)
- Parameters:
-
[in] uint8_t qual : Encode quality (1 <= qual <= 100)
- Return values:
-
error code
Definition at line 132 of file JPEG_Coverter.cpp.
Generated on Tue Jul 12 2022 11:15:12 by
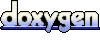