It is the Nordic nRF8001 BLE library for RedBearLab BLE Shield v2.1 or above. It is compatible with the platforms those soldered Arduino compatible form factor. The library is ported to mbed from Sandeep's arduino-BLEPeripheral library for Arduino: https://github.com/sandeepmistry/arduino-BLEPeripheral. We have tested it on KL05, KL25, MK20 and SeeedStudio Arch platforms and it works well.
Dependents: nRF8001_SimpleChat nRF8001_SimpleControls mbed_BLE2 mbed_BLEtry2 ... more
aci_queue.h
00001 /* Copyright (c) 2014, Nordic Semiconductor ASA 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy 00004 * of this software and associated documentation files (the "Software"), to deal 00005 * in the Software without restriction, including without limitation the rights 00006 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00007 * copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all 00011 * copies or substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00014 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00015 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00016 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00017 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00019 * SOFTWARE. 00020 */ 00021 00022 /** @file 00023 * @brief Interface for buffer. 00024 */ 00025 00026 /** @defgroup aci_queue aci_queue 00027 @{ 00028 @ingroup aci_queue 00029 00030 */ 00031 00032 #ifndef ACI_QUEUE_H__ 00033 #define ACI_QUEUE_H__ 00034 00035 #include "aci.h " 00036 #include "hal_aci_tl.h" 00037 00038 /*********************************************************************** */ 00039 /* The ACI_QUEUE_SIZE determines the memory usage of the system. */ 00040 /* Successfully tested to a ACI_QUEUE_SIZE of 4 (interrupt) and 4 (polling) */ 00041 /*********************************************************************** */ 00042 #define ACI_QUEUE_SIZE 4 00043 00044 /** Data type for queue of data packets to send/receive from radio. 00045 * 00046 * A FIFO queue is maintained for packets. New packets are added (enqueued) 00047 * at the tail and taken (dequeued) from the head. The head variable is the 00048 * index of the next packet to dequeue while the tail variable is the index of 00049 * where the next packet should be queued. 00050 */ 00051 00052 typedef struct { 00053 hal_aci_data_t aci_data[ACI_QUEUE_SIZE]; 00054 uint8_t head; 00055 uint8_t tail; 00056 } aci_queue_t; 00057 00058 void aci_queue_init(aci_queue_t *aci_q); 00059 00060 bool aci_queue_dequeue(aci_queue_t *aci_q, hal_aci_data_t *p_data); 00061 bool aci_queue_dequeue_from_isr(aci_queue_t *aci_q, hal_aci_data_t *p_data); 00062 00063 bool aci_queue_enqueue(aci_queue_t *aci_q, hal_aci_data_t *p_data); 00064 bool aci_queue_enqueue_from_isr(aci_queue_t *aci_q, hal_aci_data_t *p_data); 00065 00066 bool aci_queue_is_empty(aci_queue_t *aci_q); 00067 bool aci_queue_is_empty_from_isr(aci_queue_t *aci_q); 00068 00069 bool aci_queue_is_full(aci_queue_t *aci_q); 00070 bool aci_queue_is_full_from_isr(aci_queue_t *aci_q); 00071 00072 bool aci_queue_peek(aci_queue_t *aci_q, hal_aci_data_t *p_data); 00073 bool aci_queue_peek_from_isr(aci_queue_t *aci_q, hal_aci_data_t *p_data); 00074 00075 #endif /* ACI_QUEUE_H__ */ 00076 /** @} */
Generated on Tue Jul 12 2022 18:13:45 by
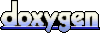