It is the Nordic nRF8001 BLE library for RedBearLab BLE Shield v2.1 or above. It is compatible with the platforms those soldered Arduino compatible form factor. The library is ported to mbed from Sandeep's arduino-BLEPeripheral library for Arduino: https://github.com/sandeepmistry/arduino-BLEPeripheral. We have tested it on KL05, KL25, MK20 and SeeedStudio Arch platforms and it works well.
Dependents: nRF8001_SimpleChat nRF8001_SimpleControls mbed_BLE2 mbed_BLEtry2 ... more
BLETypedCharacteristic.h
00001 #ifndef _BLE_TYPED_CHARACTERISTIC_H_ 00002 #define _BLE_TYPED_CHARACTERISTIC_H_ 00003 00004 #include "Arduino.h" 00005 00006 #include "BLECharacteristic.h" 00007 00008 template<typename T> class BLETypedCharacteristic : public BLECharacteristic 00009 { 00010 public: 00011 BLETypedCharacteristic(const char* uuid, unsigned char properties); 00012 00013 bool setValue(T value); 00014 T value(); 00015 00016 bool setValueLE(T value); 00017 T valueLE(); 00018 00019 bool setValueBE(T value); 00020 T valueBE(); 00021 00022 private: 00023 T byteSwap(T value); 00024 }; 00025 00026 template<typename T> BLETypedCharacteristic<T>::BLETypedCharacteristic(const char* uuid, unsigned char properties) : 00027 BLECharacteristic(uuid, properties, sizeof(T), true) 00028 { 00029 T value; 00030 memset(&value, 0x00, sizeof(value)); 00031 00032 this->setValue(value); 00033 } 00034 00035 template<typename T> bool BLETypedCharacteristic<T>::setValue(T value) { 00036 return this->BLECharacteristic::setValue((unsigned char*)&value, sizeof(T)); 00037 } 00038 00039 template<typename T> T BLETypedCharacteristic<T>::value() { 00040 T value; 00041 00042 memcpy(&value, (unsigned char*)this->BLECharacteristic::value(), this->BLECharacteristic::valueSize()); 00043 00044 return value; 00045 } 00046 00047 template<typename T> bool BLETypedCharacteristic<T>::setValueLE(T value) { 00048 return this->setValue(value); 00049 } 00050 00051 template<typename T> T BLETypedCharacteristic<T>::valueLE() { 00052 return this->getValue(); 00053 } 00054 00055 template<typename T> bool BLETypedCharacteristic<T>::setValueBE(T value) { 00056 return this->setValue(this->byteSwap(value)); 00057 } 00058 00059 template<typename T> T BLETypedCharacteristic<T>::valueBE() { 00060 return this->byteSwap(this->value()); 00061 } 00062 00063 template<typename T> T BLETypedCharacteristic<T>::byteSwap(T value) { 00064 T result; 00065 unsigned char* src = (unsigned char*)&value; 00066 unsigned char* dst = (unsigned char*)&result; 00067 00068 for (int i = 0; i < sizeof(T); i++) { 00069 dst[i] = src[sizeof(T) - i - 1]; 00070 } 00071 00072 return result; 00073 } 00074 00075 #endif
Generated on Tue Jul 12 2022 18:13:45 by
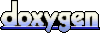