It is the Nordic nRF8001 BLE library for RedBearLab BLE Shield v2.1 or above. It is compatible with the platforms those soldered Arduino compatible form factor. The library is ported to mbed from Sandeep's arduino-BLEPeripheral library for Arduino: https://github.com/sandeepmistry/arduino-BLEPeripheral. We have tested it on KL05, KL25, MK20 and SeeedStudio Arch platforms and it works well.
Dependents: nRF8001_SimpleChat nRF8001_SimpleControls mbed_BLE2 mbed_BLEtry2 ... more
BLEPeripheral.h
00001 #ifndef _BLE_PERIPHERAL_H_ 00002 #define _BLE_PERIPHERAL_H_ 00003 00004 #include "Arduino.h" 00005 00006 #include "nRF8001.h" 00007 00008 #include "BLEAttribute.h" 00009 #include "BLECentral.h" 00010 #include "BLEDescriptor.h" 00011 #include "BLEService.h" 00012 #include "BLETypedCharacteristics.h" 00013 00014 enum BLEPeripheralEvent { 00015 BLEConnected = 0, 00016 BLEDisconnected = 1 00017 }; 00018 00019 typedef void (*BLEPeripheralEventHandler)(BLECentral& central); 00020 00021 class BLEPeripheral : public nRF8001EventListener, public BLECharacteristicValueChangeListener 00022 { 00023 public: 00024 BLEPeripheral(DigitalInOut *req, DigitalInOut *rdy, DigitalInOut *rst); 00025 virtual ~BLEPeripheral(); 00026 00027 void begin(); 00028 void poll(); 00029 00030 void setAdvertisedServiceUuid(const char* advertisedServiceUuid); 00031 void setManufacturerData(const unsigned char manufacturerData[], unsigned char manufacturerDataLength); 00032 void setLocalName(const char *localName); 00033 00034 void setDeviceName(const char* deviceName); 00035 void setAppearance(unsigned short appearance); 00036 00037 void addAttribute(BLEAttribute& attribute); 00038 00039 void disconnect(); 00040 00041 BLECentral central(); 00042 bool connected(); 00043 00044 void setEventHandler(BLEPeripheralEvent event, BLEPeripheralEventHandler eventHandler); 00045 00046 protected: 00047 bool characteristicValueChanged(BLECharacteristic& characteristic); 00048 bool canNotifyCharacteristic(BLECharacteristic& characteristic); 00049 bool canIndicateCharacteristic(BLECharacteristic& characteristic); 00050 00051 virtual void nRF8001Connected(nRF8001& nRF8001, const unsigned char* address); 00052 virtual void nRF8001Disconnected(nRF8001& nRF8001); 00053 00054 virtual void nRF8001CharacteristicValueChanged(nRF8001& nRF8001, BLECharacteristic& characteristic, const unsigned char* value, unsigned char valueLength); 00055 virtual void nRF8001CharacteristicSubscribedChanged(nRF8001& nRF8001, BLECharacteristic& characteristic, bool subscribed); 00056 00057 virtual void nRF8001AddressReceived(nRF8001& nRF8001, const unsigned char* address); 00058 virtual void nRF8001TemperatureReceived(nRF8001& nRF8001, float temperature); 00059 virtual void nRF8001BatteryLevelReceived(nRF8001& nRF8001, float batteryLevel); 00060 00061 private: 00062 nRF8001 _nRF8001; 00063 00064 const char* _advertisedServiceUuid; 00065 const unsigned char* _manufacturerData; 00066 unsigned char _manufacturerDataLength; 00067 const char* _localName; 00068 00069 BLEAttribute** _attributes; 00070 unsigned char _numAttributes; 00071 00072 BLEService _genericAccessService; 00073 BLECharacteristic _deviceNameCharacteristic; 00074 BLECharacteristic _appearanceCharacteristic; 00075 BLEService _genericAttributeService; 00076 BLECharacteristic _servicesChangedCharacteristic; 00077 00078 BLECentral _central; 00079 BLEPeripheralEventHandler _eventHandlers[2]; 00080 }; 00081 00082 #endif
Generated on Tue Jul 12 2022 18:13:45 by
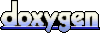