Human body temp sensor library
Dependents: MAX30205_Human_Body_Temperature_Example_Program_Hello_World MAX32630HSP3_IMU_Hello_World MAX30205_Human_Body_Temperature_Sensor MAX30205_Human_Body_Temperature_IoT ... more
MAX30205.h
00001 /******************************************************************************* 00002 * Copyright (C) 2017 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #ifndef __MAX30205_H_ 00034 #define __MAX30205_H_ 00035 00036 #include "mbed.h" 00037 00038 /** 00039 * @brief Library for the MAX30205\n 00040 * The MAX30205 temperature sensor accurately measures temperature and provide 00041 * an overtemperature alarm/interrupt/shutdown output. This device converts the 00042 * temperature measurements to digital form using a high-resolution, 00043 * sigma-delta, analog-to-digital converter (ADC). Accuracy meets clinical 00044 * thermometry specification of the ASTM E1112 when soldered on the final PCB. 00045 * Communication is through an I2C-compatible 2-wire serial interface. 00046 * 00047 * @code 00048 * #include "mbed.h" 00049 * #include "max32630fthr.h" 00050 * #include "MAX30205.h" 00051 * 00052 * MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00053 * 00054 * //Get I2C instance 00055 * I2C i2cBus(I2C1_SDA, I2C1_SCL); 00056 * 00057 * //Get temp sensor instance 00058 * MAX30205 bodyTempSensor(i2cBus, 0x4D); //Constructor takes 7-bit slave adrs 00059 * 00060 * int main(void) 00061 * { 00062 * //use sensor 00063 * } 00064 * @endcode 00065 */ 00066 00067 class MAX30205 00068 { 00069 00070 public: 00071 /// MAX30205 Register Addresses 00072 enum Registers_e 00073 { 00074 Temperature = 0x00, 00075 Configuration = 0x01, 00076 THYST = 0x02, 00077 TOS = 0x03 00078 }; 00079 00080 ///MAX30205 Configuration register bitfields 00081 union Configuration_u 00082 { 00083 uint8_t all; 00084 struct BitField_s 00085 { 00086 uint8_t shutdown : 1; 00087 uint8_t comp_int : 1; 00088 uint8_t os_polarity : 1; 00089 uint8_t fault_queue : 2; 00090 uint8_t data_format : 1; 00091 uint8_t timeout : 1; 00092 uint8_t one_shot : 1; 00093 }bits; 00094 }; 00095 00096 /** 00097 * @brief Constructor using reference to I2C object 00098 * @param i2c - Reference to I2C object 00099 * @param slaveAddress - 7-bit I2C address 00100 */ 00101 MAX30205(I2C &i2c, uint8_t slaveAddress); 00102 00103 /** @brief Destructor */ 00104 ~MAX30205(void); 00105 00106 /** 00107 * @brief Read the temperature from the device into a 16 bit value 00108 * @param[out] value - Raw temperature data on success 00109 * @return 0 on success, non-zero on failure 00110 */ 00111 int32_t readTemperature(uint16_t &value); 00112 00113 /** 00114 * @brief Read the configuration register 00115 * @param config - Reference to Configuration type 00116 * @return 0 on success, non-zero on failure 00117 */ 00118 int32_t readConfiguration(Configuration_u &config); 00119 00120 /** 00121 * @brief Write the configuration register with given configuration 00122 * @param config - Configuration to write 00123 * @return 0 on success, non-zero on failure 00124 */ 00125 int32_t writeConfiguration(const Configuration_u config); 00126 00127 /** 00128 * @brief Read the THYST value from a specified device instance 00129 * @param[out] value - THYST register value on success 00130 * @return 0 on success, non-zero on failure 00131 */ 00132 int32_t readTHYST(uint16_t &value); 00133 00134 /** 00135 * @brief Write the THYST to a device instance 00136 * @param value - 16-bit value to write 00137 * @return 0 on success, non-zero on failure 00138 */ 00139 int32_t writeTHYST(const uint16_t value); 00140 00141 /** 00142 * @brief Read the TOS value from device 00143 * @param[out] value - TOS register value on success 00144 * @return 0 on success, non-zero on failure 00145 */ 00146 int32_t readTOS(uint16_t &value); 00147 00148 /** 00149 * @brief Write the TOS register 00150 * @param value - 16-bit value to write 00151 * @return 0 on success, non-zero on failure 00152 */ 00153 int32_t writeTOS(const uint16_t value); 00154 00155 /** 00156 * @brief Convert a raw temperature value into a float 00157 * @param rawTemp - raw temperature value to convert 00158 * @return the convereted value in degrees C 00159 */ 00160 float toCelsius(uint32_t rawTemp); 00161 00162 /** 00163 * @brief Convert the passed in temperature in C to Fahrenheit 00164 * @param temperatureC Temperature in C to convert 00165 * @returns Returns the converted Fahrenheit value 00166 */ 00167 float toFahrenheit(float temperatureC); 00168 00169 protected: 00170 00171 /** 00172 * @brief Write register of device at slave address 00173 * @param reg - Register address 00174 * @param value - Value to write 00175 * @return 0 on success, non-zero on failure 00176 */ 00177 int32_t writeRegister(Registers_e reg, uint16_t value); 00178 00179 /** 00180 * @brief Read register of device at slave address 00181 * @param reg - Register address 00182 * @param[out] value - Read data on success 00183 * @return 0 on success, non-zero on failure 00184 */ 00185 int32_t readRegister(Registers_e reg, uint16_t &value); 00186 00187 private: 00188 /// I2C object 00189 I2C & m_i2c; 00190 /// Device slave addresses 00191 uint8_t m_writeAddress, m_readAddress; 00192 }; 00193 00194 #endif /* __MAX30205_H_ */
Generated on Thu Jul 14 2022 02:10:03 by
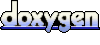