Human body temp sensor library
Dependents: MAX30205_Human_Body_Temperature_Example_Program_Hello_World MAX32630HSP3_IMU_Hello_World MAX30205_Human_Body_Temperature_Sensor MAX30205_Human_Body_Temperature_IoT ... more
MAX30205.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2017 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 00035 #include "MAX30205.h" 00036 00037 00038 //****************************************************************************** 00039 MAX30205::MAX30205(I2C &i2c, uint8_t slaveAddress): 00040 m_i2c(i2c), m_writeAddress(slaveAddress << 1), 00041 m_readAddress((slaveAddress << 1) | 1) 00042 { 00043 } 00044 00045 00046 //****************************************************************************** 00047 MAX30205::~MAX30205(void) 00048 { 00049 //empty block 00050 } 00051 00052 00053 //****************************************************************************** 00054 int32_t MAX30205::readTemperature(uint16_t &value) 00055 { 00056 return readRegister(MAX30205::Temperature, value); 00057 } 00058 00059 00060 //****************************************************************************** 00061 int32_t MAX30205::readConfiguration(Configuration_u &config) 00062 { 00063 uint16_t data; 00064 00065 int32_t result = readRegister(MAX30205::Configuration, data); 00066 if(result == 0) 00067 { 00068 config.all = (0x00FF & data); 00069 } 00070 00071 return result; 00072 00073 } 00074 00075 00076 //****************************************************************************** 00077 int32_t MAX30205::writeConfiguration(const Configuration_u config) 00078 { 00079 uint16_t local_config = (0x00FF & config.all); 00080 00081 return writeRegister(MAX30205::Configuration, local_config); 00082 } 00083 00084 00085 //****************************************************************************** 00086 int32_t MAX30205::readTHYST(uint16_t &value) 00087 { 00088 return readRegister(MAX30205::THYST, value); 00089 } 00090 00091 00092 //****************************************************************************** 00093 int32_t MAX30205::writeTHYST(uint16_t value) 00094 { 00095 return writeRegister(MAX30205::THYST, value); 00096 } 00097 00098 00099 //****************************************************************************** 00100 int32_t MAX30205::readTOS(uint16_t &value) 00101 { 00102 return readRegister(MAX30205::TOS, value); 00103 } 00104 00105 00106 //****************************************************************************** 00107 int32_t MAX30205::writeTOS(const uint16_t value) 00108 { 00109 return writeRegister(MAX30205::TOS, value); 00110 } 00111 00112 00113 //****************************************************************************** 00114 float MAX30205::toCelsius(uint32_t rawTemp) 00115 { 00116 uint8_t val1, val2; 00117 float result; 00118 00119 val1 = (rawTemp >> 8); 00120 val2 = (rawTemp & 0xFF); 00121 00122 result = static_cast<float>(val1 + (val2/ 256.0F)); 00123 00124 return result; 00125 } 00126 00127 00128 //****************************************************************************** 00129 float MAX30205::toFahrenheit(float temperatureC) 00130 { 00131 return((temperatureC * 1.8F) + 32.0f); 00132 } 00133 00134 00135 //****************************************************************************** 00136 int32_t MAX30205::writeRegister(Registers_e reg, uint16_t value) 00137 { 00138 int32_t result; 00139 00140 uint8_t hi = ((value >> 8) & 0xFF); 00141 uint8_t lo = (value & 0xFF); 00142 char cmdData[3] = {reg, hi, lo}; 00143 00144 result = m_i2c.write(m_writeAddress, cmdData, 3); 00145 00146 return result; 00147 } 00148 00149 00150 //****************************************************************************** 00151 int32_t MAX30205::readRegister(Registers_e reg, uint16_t &value) 00152 { 00153 int32_t result; 00154 00155 char data[2]; 00156 char cmdData[1] = {reg}; 00157 00158 result = m_i2c.write(m_writeAddress, cmdData, 1); 00159 if(result == 0) 00160 { 00161 result = m_i2c.read(m_readAddress, data, 2); 00162 if (result == 0) 00163 { 00164 value = (data[0] << 8) + data[1]; 00165 } 00166 } 00167 00168 return result; 00169 }
Generated on Thu Jul 14 2022 02:10:03 by
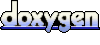