
MAX32620HSP (MAXREFDES100) RPC Example for Graphical User Interface
Dependencies: USBDevice
Fork of HSP_Release by
ServiceNonInterrupt.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "ServiceNonInterrupt.h" 00034 #include "Streaming.h" 00035 #include "BMP280.h" 00036 #include "PacketFifo.h" 00037 #include "MAX30205.h" 00038 #include "Stream.h" 00039 #include "BMP280.h" 00040 #include "PacketFifo.h" 00041 #include "Peripherals.h" 00042 #include "Device_Logging.h" 00043 00044 /// timer used for devices that do not have interrupt capability 00045 static Timer nonInterruptTimer; 00046 00047 /// reference to logging object for the BMP280 00048 extern Device_Logging *bmp280_Logging; 00049 /// reference to logging object for the MAX30205 (instance 0) 00050 extern Device_Logging *MAX30205_0_Logging; 00051 /// reference to logging object for the MAX30205 (instance 1) 00052 extern Device_Logging *MAX30205_1_Logging; 00053 00054 /** 00055 * @brief Initialize the book keeping variables for non interrupt devices 00056 */ 00057 void ServiceNonInterrupt_Init(void) { 00058 // clear out the next time member in the device logging class 00059 // this is so that the devices are sampled the very first go-around 00060 bmp280_Logging->setNextSampleTime(0); 00061 MAX30205_0_Logging->setNextSampleTime(0); 00062 MAX30205_1_Logging->setNextSampleTime(0); 00063 } 00064 00065 /** 00066 * @brief Stop the 1 second timer 00067 */ 00068 void ServiceNonInterrupt_StartTimer(void) { 00069 nonInterruptTimer.start(); 00070 nonInterruptTimer.reset(); 00071 } 00072 00073 /** 00074 * @brief Stop the 1 second timer 00075 */ 00076 void ServiceNonInterrupt_StopTimer(void) { nonInterruptTimer.stop(); } 00077 00078 /** 00079 * @brief Get the current count of the timer 00080 * @return timer count in seconds 00081 */ 00082 static int ServiceNonInterrupt_GetTimerCount(void) { 00083 return nonInterruptTimer.read(); 00084 } 00085 00086 /** 00087 * @brief Log the BMP280 sensor value if it is time 00088 * @param deviceLogging pointer to object that keeps track of logging for a 00089 * device 00090 */ 00091 void ServiceNonInterrupt_BMP280(Device_Logging *deviceLogging) { 00092 int currentTime; 00093 uint32_t bmp280_data[2]; // index 0 - Temp (deg C), index 1 - Press (Pa). 00094 // Divide the result by 10 to get the correct answer. 00095 float temp_C, press_P; 00096 int nextTime; 00097 00098 if (deviceLogging->isLoggingEnabled() == 0) 00099 return; 00100 currentTime = ServiceNonInterrupt_GetTimerCount(); 00101 nextTime = deviceLogging->getNextSampleTime(); 00102 if ((nextTime == 0) || (currentTime >= nextTime)) { 00103 nextTime = currentTime + deviceLogging->getLoggingSampleRate(); 00104 deviceLogging->setNextSampleTime(nextTime); 00105 Peripherals::bmp280()->ReadCompData( 00106 &temp_C, &press_P); // Read the Temp (index 0) and Pressure (index 1) 00107 bmp280_data[0] = (int32_t)(temp_C * 10); 00108 bmp280_data[1] = (int32_t)(press_P * 10); 00109 PacketFifo_InsertPacket(PACKET_BMP280_PRESSURE, &bmp280_data[0], 00110 2); // Read it and insert it into the FIFO 00111 } 00112 } 00113 00114 /** 00115 * @brief Log the BMP280 sensor value if it is time 00116 * @param deviceLogging pointer to object that keeps track of logging for a 00117 * device 00118 * @param device pointer to the device instance (MAX30205 instance 0 or MAX30205 00119 * instance 1) 00120 * @param packetId packet id that is used when building a packet 00121 */ 00122 void ServiceNonInterrupt_MAX30205(Device_Logging *deviceLogging, 00123 MAX30205 *device, uint32_t packetId) { 00124 int currentTime; 00125 uint32_t data; 00126 uint16_t tempData; 00127 int nextTime; 00128 00129 if (deviceLogging->isLoggingEnabled() == 0) 00130 return; 00131 currentTime = ServiceNonInterrupt_GetTimerCount(); 00132 nextTime = deviceLogging->getNextSampleTime(); 00133 if ((nextTime == 0) || (currentTime >= nextTime)) { 00134 nextTime = currentTime + deviceLogging->getLoggingSampleRate(); 00135 deviceLogging->setNextSampleTime(nextTime); 00136 device->readTemperature(&tempData); 00137 // assemble this in the correct order 00138 data = (uint32_t)((tempData >> 8) + ((tempData & 0xFF) << 8)); 00139 PacketFifo_InsertPacket(packetId, &data, 00140 1); // Read it and insert it into the FIFO 00141 } 00142 }
Generated on Tue Jul 12 2022 17:59:19 by
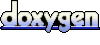