C code and C++ library, driver software for Maxim Integrated DS1775, DS75 thermometer and thermostat temperature sensor. Code supports continuous or shut-down/standby, hysteresis, alarm limits, comparator or interrupt mode, fault filtering, and active low/high. Compact 5-pin SOT23 packaging
Dependents: DS1775_Digital_Thermostat_Temperature
DS1775 Class Reference
Digital thermometer, thermostat, temperature sensor. More...
#include <ds1775_cpp.h>
Public Member Functions | |
DS1775 (I2C &i2c_bus, uint8_t slave_address) | |
Constructor for DS1775 Class. | |
~DS1775 () | |
Default destructor for DS1775 Class. | |
int | read_cfg_reg (uint8_t *value) |
Read register of device at slave address. | |
int | read_reg16 (int16_t *value, char reg) |
Read register of device at slave address. | |
float | read_reg_as_temperature (uint8_t reg) |
Reads the temperature registers. | |
int | write_cfg_reg (uint8_t cfg) |
Writes to the configuration register. | |
int | write_trip_low_thyst (float temperature) |
Writes to the THYST register. | |
int | write_trip_high_tos (float temperature) |
Writes to the TOS register. | |
float | celsius_to_fahrenheit (float temp_c) |
Converts Celsius degrees to Fahrenheit. | |
Protected Member Functions | |
int | write_reg16 (int16_t value, char reg) |
Write a value to a register. |
Detailed Description
Digital thermometer, thermostat, temperature sensor.
- Version:
- 1.0000.0002
The DS1775 reports the device's temperature over the I2C bus. It supports high, low triggers stored in EEPROM for hystersis or limit alarms using comparator or interrupt mode. The DS1775 can operate in shutdown and one-shot mode for extremely low power applications. The software is compatible with the DS75. Five pin SOT23 package Accuracy is +-2.0°C from -55°C to +125°C (-67°F to +257°),
#include "mbed.h" #include "max32630fthr.h" #include "ds1775.h" #include "USBSerial.h" MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); I2C i2cBus(P3_4, P3_5); int main() { float temperature; DigitalOut rLED(LED1, LED_OFF); DigitalOut gLED(LED2, LED_OFF); DigitalOut bLED(LED3, LED_OFF); gLED = LED_ON; DS1775 temp_sensor(i2cBus, DS1775_I2C_SLAVE_ADR_R0); i2cBus.frequency(400000); temperature = temp_sensor.read_reg_as_temperature(DS1775_REG_TEMPERATURE); printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", temperature, temp_sensor.celsius_to_fahrenheit(temperature)); }
Definition at line 78 of file ds1775_cpp.h.
Constructor & Destructor Documentation
DS1775 | ( | I2C & | i2c_bus, |
uint8_t | slave_address | ||
) |
Constructor for DS1775 Class.
Allows user to use existing I2C object
On Entry:
- Parameters:
-
[in] i2c_bus - pointer to existing I2C object [in] i2c_adrs - 7-bit slave address of DS1775
On Exit:
- Returns:
- None
Definition at line 44 of file ds1775_cpp.cpp.
~DS1775 | ( | void | ) |
Default destructor for DS1775 Class.
Destroys I2C object if owner
On Entry:
On Exit:
- Returns:
- None
empty block
Definition at line 52 of file ds1775_cpp.cpp.
Member Function Documentation
float celsius_to_fahrenheit | ( | float | temp_c ) |
Converts Celsius degrees to Fahrenheit.
- Parameters:
-
temp_c - the temperature in Celsius degrees
- Returns:
- temperature in Celsius degrees
Definition at line 195 of file ds1775_cpp.cpp.
int read_cfg_reg | ( | uint8_t * | value ) |
Read register of device at slave address.
- Parameters:
-
[out] value - Read data on success
- Returns:
- 0 on success, negative number on failure
Definition at line 58 of file ds1775_cpp.cpp.
int read_reg16 | ( | int16_t * | value, |
char | reg | ||
) |
Read register of device at slave address.
- Parameters:
-
[out] value - Read data on success reg - Register address
- Returns:
- 0 on success, negative number on failure
Definition at line 83 of file ds1775_cpp.cpp.
float read_reg_as_temperature | ( | uint8_t | reg ) |
Reads the temperature registers.
- Parameters:
-
reg - the address of the temperature register
- Returns:
- temperature in degrees Celsius
Definition at line 117 of file ds1775_cpp.cpp.
int write_cfg_reg | ( | uint8_t | cfg ) |
Writes to the configuration register.
- Parameters:
-
cfg - configurate word
- Returns:
- 0 on success, negative number on failure
Definition at line 160 of file ds1775_cpp.cpp.
int write_reg16 | ( | int16_t | value, |
char | reg | ||
) | [protected] |
Write a value to a register.
- Parameters:
-
value - value to write to the register reg - register address
- Returns:
- 0 on success, negative number on failure
Definition at line 134 of file ds1775_cpp.cpp.
int write_trip_high_tos | ( | float | temperature ) |
Writes to the TOS register.
- Parameters:
-
temperature - the temperature in Celsius degrees
- Returns:
- 0 on success, negative number on failure
Definition at line 186 of file ds1775_cpp.cpp.
int write_trip_low_thyst | ( | float | temperature ) |
Writes to the THYST register.
- Parameters:
-
temperature - the temperature in Celsius degrees
- Returns:
- 0 on success, negative number on failure
Definition at line 177 of file ds1775_cpp.cpp.
Generated on Fri Jul 15 2022 10:27:39 by
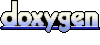