C code and C++ library, driver software for Maxim Integrated DS1775, DS75 thermometer and thermostat temperature sensor. Code supports continuous or shut-down/standby, hysteresis, alarm limits, comparator or interrupt mode, fault filtering, and active low/high. Compact 5-pin SOT23 packaging
Dependents: DS1775_Digital_Thermostat_Temperature
ds1775_cpp.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2019 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "ds1775.h" 00034 #include "ds1775_cpp.h" 00035 #include "mbed.h" 00036 // #include "USBSerial.h" 00037 00038 /****************************************************************************** 00039 * C++ version for DS1775 driver * 00040 ****************************************************************************** 00041 */ 00042 00043 /******************************************************************************/ 00044 DS1775::DS1775(I2C &i2c_bus, uint8_t slave_address): 00045 m_i2c(i2c_bus), 00046 m_write_address(slave_address <<1), 00047 m_read_address ((slave_address << 1) | 1) 00048 { 00049 } 00050 00051 /******************************************************************************/ 00052 DS1775::~DS1775(void) 00053 { 00054 /** empty block */ 00055 } 00056 00057 /******************************************************************************/ 00058 int DS1775::read_cfg_reg(uint8_t *value) 00059 { 00060 int32_t ret; 00061 char data[1] = {0}; 00062 char reg = DS1775_REG_CONFIGURATION; 00063 00064 /* write to the Register Select, true is for repeated start */ 00065 ret = m_i2c.write(m_write_address, ®, 1, true); 00066 if (ret == 0) { 00067 ret = m_i2c.read(m_read_address, data, 1, false); 00068 if (ret == 0) { 00069 *value = data[0]; 00070 return DS1775_NO_ERROR; 00071 } else { 00072 printf( 00073 "%s: failed to read data: ret: %d\r\n", __func__, ret); 00074 } 00075 } else { 00076 printf("%s: failed to write to Register Select: ret: %d\r\n", 00077 __func__, ret); 00078 } 00079 return DS1775_ERROR; 00080 } 00081 00082 /******************************************************************************/ 00083 int DS1775::read_reg16(int16_t *value, char reg) 00084 { 00085 int32_t ret; 00086 char data[2] = {0, 0}; 00087 ds1775_raw_data tmp; 00088 00089 if (reg == DS1775_REG_TEMPERATURE || 00090 reg == DS1775_REG_THYST_LOW_TRIP || reg == DS1775_REG_TOS_HIGH_TRIP) { 00091 /* write to the Register Select, true is for repeated start */ 00092 ret = m_i2c.write(m_write_address, ®, 1, true); 00093 /* read the two bytes of data */ 00094 if (ret == 0) { 00095 ret = m_i2c.read(m_read_address, data, 2, false); 00096 if (ret == 0) { 00097 tmp.msb = data[0]; 00098 tmp.lsb = data[1]; 00099 *value = tmp.swrd; 00100 return DS1775_NO_ERROR; 00101 } else { 00102 printf( 00103 "%s: failed to read data: ret: %d\r\n", __func__, ret); 00104 } 00105 } else { 00106 printf("%s: failed to write to Register Select: ret: %d\r\n", 00107 __func__, ret); 00108 } 00109 } else { 00110 printf("%s: register address is not correct: register: %d\r\n", 00111 __func__, reg); 00112 } 00113 return DS1775_ERROR; 00114 } 00115 00116 /******************************************************************************/ 00117 float DS1775::read_reg_as_temperature(uint8_t reg) 00118 { 00119 ds1775_raw_data tmp; 00120 float temperature; 00121 if (reg == DS1775_REG_TEMPERATURE || 00122 reg == DS1775_REG_THYST_LOW_TRIP || reg == DS1775_REG_TOS_HIGH_TRIP) { 00123 read_reg16(&tmp.swrd, reg); 00124 temperature = (float)tmp.swrd; /* values are 2's complement */ 00125 temperature *= DS1775_CF_LSB; 00126 return temperature; 00127 } else { 00128 printf("%s: register is invalid, %d r\n", __func__, reg); 00129 return 0; 00130 } 00131 } 00132 00133 /******************************************************************************/ 00134 int DS1775::write_reg16(int16_t value, char reg) 00135 { 00136 int32_t ret; 00137 char cmd[3]; 00138 ds1775_raw_data tmp; 00139 00140 if (reg >= DS1775_REG_THYST_LOW_TRIP && reg <= DS1775_REG_MAX) { 00141 cmd[0] = reg; 00142 tmp.swrd = value; 00143 cmd[1] = tmp.msb; 00144 cmd[2] = tmp.lsb; 00145 ret = m_i2c.write(m_write_address, cmd, 3, false); 00146 if (ret == 0) { 00147 return DS1775_NO_ERROR; 00148 } else { 00149 printf("%s: I2C write error %d\r\n",__func__, ret); 00150 return DS1775_ERROR; 00151 } 00152 } else { 00153 printf("%s: register value invalid %x\r\n",__func__, reg); 00154 return DS1775_ERROR; 00155 } 00156 } 00157 00158 00159 /******************************************************************************/ 00160 int DS1775::write_cfg_reg(uint8_t cfg) 00161 { 00162 int32_t ret; 00163 char cmd[2]; 00164 00165 cmd[0] = DS1775_REG_CONFIGURATION; 00166 cmd[1] = cfg; 00167 ret = m_i2c.write(m_write_address, cmd, 2, false); 00168 if (ret == 0) { 00169 return DS1775_NO_ERROR; 00170 } else { 00171 printf("%s: I2C write error %d\r\n",__func__, ret); 00172 return DS1775_ERROR; 00173 } 00174 } 00175 00176 /******************************************************************************/ 00177 int DS1775::write_trip_low_thyst(float temperature) 00178 { 00179 ds1775_raw_data raw; 00180 temperature /= DS1775_CF_LSB; 00181 raw.swrd = int16_t(temperature); 00182 return write_reg16(raw.swrd, DS1775_REG_THYST_LOW_TRIP); 00183 } 00184 00185 /******************************************************************************/ 00186 int DS1775::write_trip_high_tos(float temperature) 00187 { 00188 ds1775_raw_data raw; 00189 temperature /= DS1775_CF_LSB; 00190 raw.swrd = int16_t(temperature); 00191 return write_reg16(raw.uwrd, DS1775_REG_TOS_HIGH_TRIP); 00192 } 00193 00194 /******************************************************************************/ 00195 float DS1775::celsius_to_fahrenheit(float temp_c) 00196 { 00197 float temp_f; 00198 temp_f = ((temp_c * 9)/5) + 32; 00199 return temp_f; 00200 }
Generated on Fri Jul 15 2022 10:27:39 by
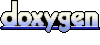