
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
GenericValue< Encoding, Allocator > Class Template Reference
Represents a JSON value. Use Value for UTF8 encoding and default allocator. More...
#include <document.h>
Inherited by GenericDocument< Encoding, Allocator, StackAllocator >.
Public Types | |
typedef GenericMember < Encoding, Allocator > | Member |
Name-value pair in an object. | |
typedef Encoding | EncodingType |
Encoding type from template parameter. | |
typedef Allocator | AllocatorType |
Allocator type from template parameter. | |
typedef Encoding::Ch | Ch |
Character type derived from Encoding. | |
typedef GenericStringRef< Ch > | StringRefType |
Reference to a constant string. | |
typedef GenericMemberIterator < false, Encoding, Allocator > ::Iterator | MemberIterator |
Member iterator for iterating in object. | |
typedef GenericMemberIterator < true, Encoding, Allocator > ::Iterator | ConstMemberIterator |
Constant member iterator for iterating in object. | |
typedef GenericValue * | ValueIterator |
Value iterator for iterating in array. | |
typedef const GenericValue * | ConstValueIterator |
Constant value iterator for iterating in array. | |
typedef GenericValue< Encoding, Allocator > | ValueType |
Value type of itself. | |
Public Member Functions | |
template<typename T > | |
RAPIDJSON_DISABLEIF_RETURN ((internal::IsPointer< T >),(GenericValue &)) operator | |
Assignment with primitive types. | |
void | SetObjectRaw (Member *members, SizeType count, Allocator &allocator) |
Initialize this value as object with initial data, without calling destructor. | |
void | SetStringRaw (StringRefType s) RAPIDJSON_NOEXCEPT |
Initialize this value as constant string, without calling destructor. | |
void | SetStringRaw (StringRefType s, Allocator &allocator) |
Initialize this value as copy string with initial data, without calling destructor. | |
void | RawAssign (GenericValue &rhs) RAPIDJSON_NOEXCEPT |
Assignment without calling destructor. | |
Assignment operators | |
GenericValue & | operator= (GenericValue &rhs) RAPIDJSON_NOEXCEPT |
Assignment with move semantics. | |
GenericValue & | operator= (GenericValue &&rhs) RAPIDJSON_NOEXCEPT |
Move assignment in C++11. | |
GenericValue & | operator= (StringRefType str) RAPIDJSON_NOEXCEPT |
Assignment of constant string reference (no copy) | |
Friends | |
class | GenericDocument |
Constructors and destructor. | |
GenericValue () RAPIDJSON_NOEXCEPT | |
Default constructor creates a null value. | |
GenericValue (GenericValue &&rhs) RAPIDJSON_NOEXCEPT | |
Move constructor in C++11. | |
GenericValue (Type type) RAPIDJSON_NOEXCEPT | |
Constructor with JSON value type. | |
template<typename SourceAllocator > | |
GenericValue (const GenericValue< Encoding, SourceAllocator > &rhs, Allocator &allocator) | |
Explicit copy constructor (with allocator) | |
template<typename T > | |
GenericValue (T b, RAPIDJSON_ENABLEIF((internal::IsSame< bool, T >))) RAPIDJSON_NOEXCEPTexplicit GenericValue(bool b) RAPIDJSON_NOEXCEPT | |
Constructor for boolean value. | |
GenericValue (int i) RAPIDJSON_NOEXCEPT | |
Constructor for int value. | |
GenericValue (unsigned u) RAPIDJSON_NOEXCEPT | |
Constructor for unsigned value. | |
GenericValue (int64_t i64) RAPIDJSON_NOEXCEPT | |
Constructor for int64_t value. | |
GenericValue (uint64_t u64) RAPIDJSON_NOEXCEPT | |
Constructor for uint64_t value. | |
GenericValue (double d) RAPIDJSON_NOEXCEPT | |
Constructor for double value. | |
GenericValue (const Ch *s, SizeType length) RAPIDJSON_NOEXCEPT | |
Constructor for constant string (i.e. do not make a copy of string) | |
GenericValue (StringRefType s) RAPIDJSON_NOEXCEPT | |
Constructor for constant string (i.e. do not make a copy of string) | |
GenericValue (const Ch *s, SizeType length, Allocator &allocator) | |
Constructor for copy-string (i.e. do make a copy of string) | |
GenericValue (const Ch *s, Allocator &allocator) | |
Constructor for copy-string (i.e. do make a copy of string) | |
GenericValue (const std::basic_string< Ch > &s, Allocator &allocator) | |
Constructor for copy-string from a string object (i.e. do make a copy of string) | |
GenericValue (Array a) RAPIDJSON_NOEXCEPT | |
Constructor for Array. | |
GenericValue (Object o) RAPIDJSON_NOEXCEPT | |
Constructor for Object. | |
~GenericValue () | |
Destructor. |
Detailed Description
template<typename Encoding, typename Allocator = MemoryPoolAllocator<>>
class GenericValue< Encoding, Allocator >
Represents a JSON value. Use Value for UTF8 encoding and default allocator.
A JSON value can be one of 7 types. This class is a variant type supporting these types.
Use the Value if UTF8 and default allocator
- Template Parameters:
-
Encoding Encoding of the value. (Even non-string values need to have the same encoding in a document) Allocator Allocator type for allocating memory of object, array and string.
Definition at line 540 of file document.h.
Member Typedef Documentation
typedef Allocator AllocatorType |
Allocator type from template parameter.
Reimplemented in GenericDocument< Encoding, Allocator, StackAllocator >.
Definition at line 545 of file document.h.
typedef Encoding::Ch Ch |
Character type derived from Encoding.
Reimplemented in GenericDocument< Encoding, Allocator, StackAllocator >.
Definition at line 546 of file document.h.
typedef GenericMemberIterator<true,Encoding,Allocator>::Iterator ConstMemberIterator |
Constant member iterator for iterating in object.
Definition at line 549 of file document.h.
typedef const GenericValue* ConstValueIterator |
Constant value iterator for iterating in array.
Definition at line 551 of file document.h.
typedef Encoding EncodingType |
Encoding type from template parameter.
Definition at line 544 of file document.h.
typedef GenericMember<Encoding, Allocator> Member |
Name-value pair in an object.
Definition at line 543 of file document.h.
typedef GenericMemberIterator<false,Encoding,Allocator>::Iterator MemberIterator |
Member iterator for iterating in object.
Definition at line 548 of file document.h.
typedef GenericStringRef<Ch> StringRefType |
Reference to a constant string.
Definition at line 547 of file document.h.
typedef GenericValue* ValueIterator |
Value iterator for iterating in array.
Definition at line 550 of file document.h.
typedef GenericValue<Encoding, Allocator> ValueType |
Value type of itself.
Reimplemented in GenericDocument< Encoding, Allocator, StackAllocator >.
Definition at line 552 of file document.h.
Constructor & Destructor Documentation
GenericValue | ( | ) |
Default constructor creates a null value.
Definition at line 562 of file document.h.
GenericValue | ( | GenericValue< Encoding, Allocator > && | rhs ) |
Move constructor in C++11.
Definition at line 566 of file document.h.
GenericValue | ( | Type | type ) | [explicit] |
Constructor with JSON value type.
This creates a Value of specified type with default content.
- Parameters:
-
type Type of the value.
- Note:
- Default content for number is zero.
Definition at line 592 of file document.h.
GenericValue | ( | const GenericValue< Encoding, SourceAllocator > & | rhs, |
Allocator & | allocator | ||
) |
Explicit copy constructor (with allocator)
Creates a copy of a Value by using the given Allocator
- Template Parameters:
-
SourceAllocator allocator of rhs
- Parameters:
-
rhs Value to copy from (read-only) allocator Allocator for allocating copied elements and buffers. Commonly use GenericDocument::GetAllocator().
- See also:
- CopyFrom()
Definition at line 2408 of file document.h.
GenericValue | ( | T | b, |
RAPIDJSON_ENABLEIF((internal::IsSame< bool, T >)) | |||
) | [explicit] |
Constructor for boolean value.
- Parameters:
-
b Boolean value
- Note:
- This constructor is limited to real boolean values and rejects implicitly converted types like arbitrary pointers. Use an explicit cast to
bool
, if you want to construct a boolean JSON value in such cases.
Definition at line 623 of file document.h.
GenericValue | ( | int | i ) | [explicit] |
Constructor for int value.
Definition at line 634 of file document.h.
GenericValue | ( | unsigned | u ) | [explicit] |
Constructor for unsigned value.
Definition at line 640 of file document.h.
GenericValue | ( | int64_t | i64 ) | [explicit] |
Constructor for int64_t value.
Definition at line 646 of file document.h.
GenericValue | ( | uint64_t | u64 ) | [explicit] |
Constructor for uint64_t value.
Definition at line 661 of file document.h.
GenericValue | ( | double | d ) | [explicit] |
Constructor for double value.
Definition at line 673 of file document.h.
GenericValue | ( | const Ch * | s, |
SizeType | length | ||
) |
Constructor for constant string (i.e. do not make a copy of string)
Definition at line 676 of file document.h.
GenericValue | ( | StringRefType | s ) | [explicit] |
Constructor for constant string (i.e. do not make a copy of string)
Definition at line 679 of file document.h.
GenericValue | ( | const Ch * | s, |
SizeType | length, | ||
Allocator & | allocator | ||
) |
Constructor for copy-string (i.e. do make a copy of string)
Definition at line 682 of file document.h.
GenericValue | ( | const Ch * | s, |
Allocator & | allocator | ||
) |
Constructor for copy-string (i.e. do make a copy of string)
Definition at line 685 of file document.h.
GenericValue | ( | const std::basic_string< Ch > & | s, |
Allocator & | allocator | ||
) |
Constructor for copy-string from a string object (i.e. do make a copy of string)
- Note:
- Requires the definition of the preprocessor symbol RAPIDJSON_HAS_STDSTRING.
Definition at line 691 of file document.h.
GenericValue | ( | Array | a ) |
Constructor for Array.
- Parameters:
-
a An array obtained by GetArray()
.
- Note:
Array
is always pass-by-value.- the source array is moved into this value and the sourec array becomes empty.
Definition at line 700 of file document.h.
GenericValue | ( | Object | o ) |
Constructor for Object.
- Parameters:
-
o An object obtained by GetObject()
.
- Note:
Object
is always pass-by-value.- the source object is moved into this value and the sourec object becomes empty.
Definition at line 711 of file document.h.
~GenericValue | ( | ) |
Destructor.
Need to destruct elements of array, members of object, or copy-string.
Definition at line 719 of file document.h.
Member Function Documentation
GenericValue& operator= | ( | GenericValue< Encoding, Allocator > & | rhs ) |
Assignment with move semantics.
- Parameters:
-
rhs Source of the assignment. It will become a null value after assignment.
Definition at line 755 of file document.h.
GenericValue& operator= | ( | GenericValue< Encoding, Allocator > && | rhs ) |
Move assignment in C++11.
Definition at line 764 of file document.h.
GenericValue& operator= | ( | StringRefType | str ) |
Assignment of constant string reference (no copy)
- Parameters:
-
str Constant string reference to be assigned
- Note:
- This overload is needed to avoid clashes with the generic primitive type assignment overload below.
- See also:
- GenericStringRef, operator=(T)
Definition at line 774 of file document.h.
RAPIDJSON_DISABLEIF_RETURN | ( | (internal::IsPointer< T >) | , |
(GenericValue< Encoding, Allocator > &) | |||
) |
Assignment with primitive types.
- Template Parameters:
-
T Either Type, int
,unsigned
,int64_t
,uint64_t
- Parameters:
-
value The value to be assigned.
- Note:
- The source type
T
explicitly disallows all pointer types, especially (const
) Ch*. This helps avoiding implicitly referencing character strings with insufficient lifetime, use SetString(const Ch*, Allocator&) (for copying) or StringRef() (to explicitly mark the pointer as constant) instead. All other pointer types would implicitly convert tobool
, use SetBool() instead. Set boolean value
void RawAssign | ( | GenericValue< Encoding, Allocator > & | rhs ) |
Assignment without calling destructor.
Definition at line 1984 of file document.h.
Initialize this value as object with initial data, without calling destructor.
Definition at line 1947 of file document.h.
void SetStringRaw | ( | StringRefType | s ) |
Initialize this value as constant string, without calling destructor.
Definition at line 1960 of file document.h.
void SetStringRaw | ( | StringRefType | s, |
Allocator & | allocator | ||
) |
Initialize this value as copy string with initial data, without calling destructor.
Definition at line 1967 of file document.h.
Generated on Tue Jul 12 2022 12:06:51 by
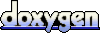