
DeepCover Embedded Security in IoT: Public-key Secured Data Paths
Dependencies: MaximInterface
GenericMemberIterator< Const, Encoding, Allocator > Class Template Reference
(Constant) member iterator for a JSON object value More...
#include <document.h>
Public Types | |
typedef GenericMemberIterator | Iterator |
Iterator type itself. | |
typedef GenericMemberIterator < true, Encoding, Allocator > | ConstIterator |
Constant iterator type. | |
typedef GenericMemberIterator < false, Encoding, Allocator > | NonConstIterator |
Non-constant iterator type. | |
typedef BaseType::pointer | Pointer |
Pointer to (const) GenericMember. | |
typedef BaseType::reference | Reference |
Reference to (const) GenericMember. | |
typedef BaseType::difference_type | DifferenceType |
Signed integer type (e.g. ptrdiff_t ) | |
Public Member Functions | |
GenericMemberIterator () | |
Default constructor (singular value) | |
GenericMemberIterator (const NonConstIterator &it) | |
Iterator conversions to more const. | |
DifferenceType | operator- (ConstIterator that) const |
Distance. | |
stepping | |
Iterator & | operator++ () |
Iterator & | operator-- () |
Iterator | operator++ (int) |
Iterator | operator-- (int) |
increment/decrement | |
Iterator | operator+ (DifferenceType n) const |
Iterator | operator- (DifferenceType n) const |
Iterator & | operator+= (DifferenceType n) |
Iterator & | operator-= (DifferenceType n) |
relations | |
bool | operator== (ConstIterator that) const |
bool | operator!= (ConstIterator that) const |
bool | operator<= (ConstIterator that) const |
bool | operator>= (ConstIterator that) const |
bool | operator< (ConstIterator that) const |
bool | operator> (ConstIterator that) const |
dereference | |
Reference | operator* () const |
Pointer | operator-> () const |
Reference | operator[] (DifferenceType n) const |
Friends | |
class | GenericValue< Encoding, Allocator > |
class | GenericMemberIterator |
Detailed Description
template<bool Const, typename Encoding, typename Allocator>
class GenericMemberIterator< Const, Encoding, Allocator >
(Constant) member iterator for a JSON object value
- Template Parameters:
-
Const Is this a constant iterator? Encoding Encoding of the value. (Even non-string values need to have the same encoding in a document) Allocator Allocator type for allocating memory of object, array and string.
This class implements a Random Access Iterator for GenericMember elements of a GenericValue, see ISO/IEC 14882:2003(E) C++ standard, 24.1 [lib.iterator.requirements].
- Note:
- This iterator implementation is mainly intended to avoid implicit conversions from iterator values to
NULL
, e.g. from GenericValue::FindMember. -
Define
RAPIDJSON_NOMEMBERITERATORCLASS
to fall back to a pointer-based implementation, if your platform doesn't provide the C++ <iterator> header.
Definition at line 101 of file document.h.
Member Typedef Documentation
typedef GenericMemberIterator<true,Encoding,Allocator> ConstIterator |
Constant iterator type.
Definition at line 116 of file document.h.
typedef BaseType::difference_type DifferenceType |
Signed integer type (e.g. ptrdiff_t
)
Definition at line 125 of file document.h.
typedef GenericMemberIterator Iterator |
Iterator type itself.
Definition at line 114 of file document.h.
typedef GenericMemberIterator<false,Encoding,Allocator> NonConstIterator |
Non-constant iterator type.
Definition at line 118 of file document.h.
typedef BaseType::pointer Pointer |
Pointer to (const) GenericMember.
Definition at line 121 of file document.h.
typedef BaseType::reference Reference |
Reference to (const) GenericMember.
Definition at line 123 of file document.h.
Constructor & Destructor Documentation
Default constructor (singular value)
Creates an iterator pointing to no element.
- Note:
- All operations, except for comparisons, are undefined on such values.
Definition at line 131 of file document.h.
GenericMemberIterator | ( | const NonConstIterator & | it ) |
Iterator conversions to more const.
- Parameters:
-
it (Non-const) iterator to copy from
Allows the creation of an iterator from another GenericMemberIterator that is "less const". Especially, creating a non-constant iterator from a constant iterator are disabled:
- const -> non-const (not ok)
- const -> const (ok)
- non-const -> const (ok)
- non-const -> non-const (ok)
- Note:
- If the
Const
template parameter is alreadyfalse
, this constructor effectively defines a regular copy-constructor. Otherwise, the copy constructor is implicitly defined.
Definition at line 149 of file document.h.
Member Function Documentation
DifferenceType operator- | ( | ConstIterator | that ) | const |
Distance.
Definition at line 187 of file document.h.
Generated on Tue Jul 12 2022 12:06:51 by
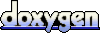