
Animation demo with MIP8F_SPI_Ver60
Dependencies: mbed MIP8F_SPI_Ver60 MIP8f_FRDM_LineBuffer_sample MIP8f_FRDM_TransferMode_sample
Adafruit_LEDBackpack.cpp
00001 /*************************************************** 00002 This is a library for our I2C LED Backpacks 00003 00004 Designed specifically to work with the Adafruit LED Matrix backpacks 00005 ----> http://www.adafruit.com/products/ 00006 ----> http://www.adafruit.com/products/ 00007 00008 These displays use I2C to communicate, 2 pins are required to 00009 interface. There are multiple selectable I2C addresses. For backpacks 00010 with 2 Address Select pins: 0x70, 0x71, 0x72 or 0x73. For backpacks 00011 with 3 Address Select pins: 0x70 thru 0x77 00012 00013 Adafruit invests time and resources providing this open source code, 00014 please support Adafruit and open-source hardware by purchasing 00015 products from Adafruit! 00016 00017 Written by Limor Fried/Ladyada for Adafruit Industries. 00018 BSD license, all text above must be included in any redistribution 00019 ****************************************************/ 00020 00021 /* 00022 * Modified by Luiz Hespanha (http://www.d3.do) 8/16/2013 for use in LPC1768 00023 */ 00024 00025 #include "mbed.h" 00026 #include "Adafruit_LEDBackpack.h" 00027 #include "Adafruit_GFX.h" 00028 00029 void Adafruit_LEDBackpack::setBrightness(uint8_t b) { 00030 if (b > 15) b = 15; 00031 uint8_t c = 0xE0 | b; 00032 char foo[1]; 00033 foo[0] = c; 00034 _i2c->write(i2c_addr, foo, 1); 00035 } 00036 00037 void Adafruit_LEDBackpack::blinkRate(uint8_t b) { 00038 if (b > 3) b = 0; // turn off if not sure 00039 uint8_t c = HT16K33_BLINK_CMD | HT16K33_BLINK_DISPLAYON | (b << 1); 00040 char foo[1]; 00041 foo[0] = c; 00042 _i2c->write(i2c_addr, foo, 1); 00043 } 00044 00045 Adafruit_LEDBackpack::Adafruit_LEDBackpack(I2C *i2c): _i2c(i2c) { 00046 } 00047 00048 void Adafruit_LEDBackpack::begin(uint8_t _addr = 0x70) { 00049 i2c_addr = _addr << 1; 00050 00051 char foo[1]; 00052 foo[0] = 0x21; 00053 00054 _i2c->write(i2c_addr, foo, 1); // turn on oscillator 00055 00056 blinkRate(HT16K33_BLINK_OFF); 00057 00058 setBrightness(15); // max brightness 00059 } 00060 00061 void Adafruit_LEDBackpack::writeDisplay(void) { 00062 char foo[17]; 00063 foo[0] = 0x00; 00064 int j = 0; 00065 for (uint8_t i=1; i<=16; i+=2) { 00066 int x = displaybuffer[j] & 0xFF; 00067 foo[i] = x; 00068 int x2 = displaybuffer[j] >> 8; 00069 foo[i+1] = x2; 00070 j++; 00071 } 00072 _i2c->write(i2c_addr, foo, 17); 00073 } 00074 00075 void Adafruit_LEDBackpack::clear(void) { 00076 for (uint8_t i=0; i<8; i++) { 00077 displaybuffer[i] = 0; 00078 } 00079 } 00080 00081 Adafruit_8x8matrix::Adafruit_8x8matrix(I2C *i2c) : Adafruit_LEDBackpack(i2c), Adafruit_GFX(8, 8) { 00082 } 00083 00084 void Adafruit_8x8matrix::drawPixel(int16_t x, int16_t y, uint16_t color) { 00085 if ((y < 0) || (y >= 8)) return; 00086 if ((x < 0) || (x >= 8)) return; 00087 00088 // check rotation, move pixel around if necessary 00089 switch (getRotation()) { 00090 case 1: 00091 swap(x, y); 00092 x = 8 - x - 1; 00093 break; 00094 case 2: 00095 x = 8 - x - 1; 00096 y = 8 - y - 1; 00097 break; 00098 case 3: 00099 swap(x, y); 00100 y = 8 - y - 1; 00101 break; 00102 } 00103 00104 // wrap around the x 00105 x += 7; 00106 x %= 8; 00107 00108 00109 if (color) { 00110 displaybuffer[y] |= 1 << x; 00111 } else { 00112 displaybuffer[y] &= ~(1 << x); 00113 } 00114 }
Generated on Wed Jul 13 2022 14:26:38 by
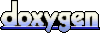