
Self test boot program for testing icarus sensors
Dependencies: BLE_API mbed nRF51822
Fork of BLE_UARTConsole by
ADXL362Sensor.cpp
00001 #include "ADXL362Sensor.h" 00002 00003 00004 00005 ADXL362Sensor::ADXL362Sensor(SPI& spi_,DigitalOut& cs_,void (*debug_)(const char* format, ...)) : BaseSensor(debug_), spi(spi_), cs(cs_) { 00006 cs = UP; 00007 writeRegister(ADXL362_SOFT_RESET, 0x52); 00008 wait_ms(1); 00009 writeRegister(ADXL362_POWER_CTL,0x02); 00010 } 00011 00012 00013 char* ADXL362Sensor::getSimpleName() { 00014 return "ADXL362"; 00015 } 00016 00017 00018 uint32_t ADXL362Sensor::verifyIntegrity(uint32_t* errorResult) { 00019 LOG("Start verfication of ADXL362 Sensor\r\n"); 00020 uint32_t errors = 0; 00021 //Device id is 0xAD 00022 //Device mems id is 0x1D 00023 //Part id is 0xF2 00024 uint32_t sensorId = readRegister32(ADXL362_DEVID_AD); 00025 00026 if (sensorId >> 8 !=0xAD1DF2){ 00027 errorResult[errors++] = ERROR_WRONG_DEVICE_ID; 00028 LOG("Wrong sensorId: %X\r\n",sensorId); 00029 } 00030 00031 //check status registry 00032 uint8_t status = readRegister(ADXL362_STATUS); 00033 00034 //indicate that SEU error was detetcted 00035 if (status & (1 << 7)){ 00036 errorResult[errors++] = ERROR_WRONG_DEVICE_STATE; 00037 LOG("SEU error detected: %X\r\n",status); 00038 } 00039 //check that chip is in awaken state 00040 if (!(status & (1 << 6))){ 00041 errorResult[errors++] = ERROR_DEVICE_SLEEPING; 00042 LOG("Chip not awaken: %X\r\n",status); 00043 } 00044 00045 //perform self test 00046 errors+=selfTest(&errorResult[errors]); 00047 00048 return errors; 00049 } 00050 00051 void ADXL362Sensor::getSensorDetails(sensor_t* sensorDetails) { 00052 00053 } 00054 00055 uint32_t ADXL362Sensor::selfTest(uint32_t* errorResult){ 00056 uint32_t errors = 0; 00057 // 0. base data 00058 int16_t x12,y12,z12; 00059 int16_t test_x12,test_y12,test_z12; 00060 // 1. Read acceleration data for the x-, y-, and z-axes. 00061 refreshAcceleration12(&x12, &y12, &z12); 00062 // 2. Assert self test by setting the ST bit in the SELF_TEST register, Address 0x2E. 00063 writeRegister(ADXL362_SELF_TEST,0x01); 00064 // 3. Wait 1/ODR for the output to settle to its new value. 00065 wait(4.0/ADXL362_ODR); 00066 // 4. Read acceleration data for the x-, y-, and z-axes. 00067 refreshAcceleration12(&test_x12, &test_y12, &test_z12); 00068 // 5. Compare to the values from Step 1, and convert the difference from LSB to mg by multiplying by the sensitivity. If the observed difference falls within the self test output change specification listed in Table 1, then the device passes self test and is deemed operational. 00069 float reactionX = (test_x12-x12) * 0.250f; 00070 float reactionY = (test_y12-y12) * 0.250f; 00071 float reactionZ = (test_z12-z12) * 0.250f; 00072 00073 00074 if (reactionX<230.0F || reactionX>870.0F || 00075 reactionY<-870.0F || reactionY>-230.0F || 00076 reactionZ<270.0F || reactionZ>800.0F){ 00077 errorResult[errors++] = ERROR_ACCE_SELF_TEST_FAILED; 00078 LOG("Reaction: %+5.3f %+5.3f %+5.3f\r\n",reactionX,reactionY,reactionZ); 00079 } 00080 00081 // 6. Deassert self test by clearing the ST bit in the SELF_TEST register, Address 0x2E. 00082 writeRegister(ADXL362_SELF_TEST,0x00); 00083 wait(4.0/ADXL362_ODR); 00084 return errors; 00085 } 00086 00087 void ADXL362Sensor::refreshAcceleration12(int16_t* x, int16_t* y, int16_t* z) 00088 { 00089 int16_t xyzVal[6] = {0, 0, 0, 0, 0, 0}; 00090 00091 cs = DOWN; 00092 spi.write(ADXL362_READ_REGISTER); 00093 spi.write(ADXL362_DATA_12); 00094 00095 for (int i = 0; i < 6; i++) { 00096 xyzVal[i] = spi.write(0x00); 00097 } 00098 00099 *x = (xyzVal[1] << 8) + xyzVal[0]; 00100 *y = (xyzVal[3] << 8) + xyzVal[2]; 00101 *z = (xyzVal[5] << 8) + xyzVal[4]; 00102 00103 cs = UP; 00104 } 00105 00106 uint32_t ADXL362Sensor::readRegister32( uint8_t reg){ 00107 uint32_t val[4] = {0,0,0,0}; 00108 cs = DOWN; 00109 spi.write(ADXL362_READ_REGISTER); 00110 spi.write(reg); 00111 for (int i=0;i<4;i++){ 00112 val[i] = spi.write(0x00); 00113 } 00114 cs = UP; 00115 00116 return (val[0] << 24) + (val[1] << 16) + (val[2] << 8) + val[3]; 00117 } 00118 00119 00120 uint8_t ADXL362Sensor::readRegister( uint8_t reg){ 00121 cs = DOWN; 00122 spi.write(ADXL362_READ_REGISTER); 00123 spi.write(reg); 00124 uint8_t val = spi.write(0x00); 00125 cs = UP; 00126 return (val); 00127 } 00128 00129 void ADXL362Sensor::writeRegister( uint8_t reg, uint8_t cmd ){ 00130 cs = DOWN; 00131 spi.write(ADXL362_WRITE_REGISTER); 00132 spi.write(reg); 00133 spi.write(cmd); 00134 cs = UP; 00135 }
Generated on Wed Jul 13 2022 08:05:01 by
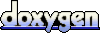