Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Dependents: testUniGraphic_150217 maze_TFT_MMA8451Q TFT_test_frdm-kl25z TFT_test_NUCLEO-F411RE ... more
ILI9327.cpp
00001 /* mbed UniGraphic library - Device specific class 00002 * Copyright (c) 2015 Giuliano Dianda 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 #include "Protocols.h " 00006 #include "ILI9327.h" 00007 00008 ////////////////////////////////////////////////////////////////////////////////// 00009 // display settings /////////////////////////////////////////////////////// 00010 ///////////////////////////////////////////////////////////////////////// 00011 00012 // put in constructor 00013 //#define LCDSIZE_X 240 // display X pixels, TFTs are usually portrait view 00014 //#define LCDSIZE_Y 400 // display Y pixels 00015 00016 00017 00018 ILI9327::ILI9327(proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name , unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00019 : TFT(displayproto, port, CS, reset, DC, WR, RD, LCDSIZE_X, LCDSIZE_Y, name) 00020 { 00021 hw_reset(); 00022 BusEnable(true); 00023 identify(); // will collect tftID, set mipistd flag 00024 init(); 00025 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00026 // scrollbugfix=1; // when scrolling 1 line, the last line disappears, set to 1 to fix it, for ili9481 is set automatically in identify() 00027 set_orientation(0); 00028 FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. Give a try 00029 cls(); 00030 locate(0,0); 00031 } 00032 ILI9327::ILI9327(proto_t displayproto, PinName* buspins, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name , unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00033 : TFT(displayproto, buspins, CS, reset, DC, WR, RD, LCDSIZE_X, LCDSIZE_Y, name) 00034 { 00035 hw_reset(); 00036 BusEnable(true); 00037 identify(); // will collect tftID, set mipistd flag 00038 init(); 00039 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00040 // scrollbugfix=1; // when scrolling 1 line, the last line disappears, set to 1 to fix it, for ili9481 is set automatically in identify() 00041 set_orientation(0); 00042 FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. Give a try 00043 cls(); 00044 locate(0,0); 00045 } 00046 ILI9327::ILI9327(proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, PinName DC, const char *name , unsigned int LCDSIZE_X , unsigned int LCDSIZE_Y ) 00047 : TFT(displayproto, Hz, mosi, miso, sclk, CS, reset, DC, LCDSIZE_X, LCDSIZE_Y, name) 00048 { 00049 hw_reset(); //TFT class forwards to Protocol class 00050 BusEnable(true); //TFT class forwards to Protocol class 00051 identify(); // will collect tftID and set mipistd flag 00052 init(); // per display custom init cmd sequence, implemented here 00053 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00054 // scrollbugfix=1; // when scrolling 1 line, the last line disappears, set to 1 to fix it, for ili9481 is set automatically in identify() 00055 set_orientation(0); //TFT class does for MIPI standard and some ILIxxx 00056 FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. Give a try 00057 cls(); 00058 locate(0,0); 00059 } 00060 // read display ID 00061 void ILI9327::identify() 00062 { 00063 // ILI9327 custom cmd 00064 tftID=rd_reg_data32(0xEF); 00065 mipistd=true; 00066 } 00067 // reset and init the lcd controller 00068 void ILI9327::init() 00069 { 00070 wr_cmd8(0x11); 00071 thread_sleep_for(150); 00072 00073 wr_cmd8(0xF3); //Set EQ 00074 wr_data8(0x08); 00075 wr_data8(0x20); 00076 wr_data8(0x20); 00077 wr_data8(0x08); 00078 00079 wr_cmd8(0xE7); 00080 wr_data8(0x60); //OPON 00081 00082 wr_cmd8(0xD1); // Set VCOM 00083 wr_data8(0x00); 00084 wr_data8(0x5D); 00085 wr_data8(0x15); 00086 00087 wr_cmd8(0xD0); //Set power related setting 00088 wr_data8(0x07); 00089 wr_data8(0x02); //VGH:15V,VGL:-7.16V (BOE LCD: VGH:12~18V,VGL:-6~10V) 00090 wr_data8(0x8B); 00091 wr_data8(0x03); 00092 wr_data8(0xD4); 00093 00094 wr_cmd8(0x3A); // Set_pixel_format 00095 wr_data8(0x55); //0x55:16bit/pixel,65k;0x66:18bit/pixel,262k; 00096 00097 wr_cmd8(0x36); //Set_address_mode 00098 wr_data8(0x38); 00099 00100 wr_cmd8(0x20); //Exit_invert_mode 00101 00102 wr_cmd8(0xC1); //Set Normal/Partial mode display timing 00103 wr_data8(0x10); 00104 wr_data8(0x1A); 00105 wr_data8(0x02); 00106 wr_data8(0x02); 00107 00108 wr_cmd8(0xC0); //Set display related setting 00109 wr_data8(0x10); 00110 wr_data8(0x31); 00111 wr_data8(0x00); 00112 wr_data8(0x00); 00113 wr_data8(0x01); 00114 wr_data8(0x02); 00115 00116 wr_cmd8(0xC4); //Set waveform timing 00117 wr_data8(0x01); 00118 00119 wr_cmd8(0xC5); //Set oscillator 00120 wr_data8(0x04); //72Hz 00121 wr_data8(0x01); 00122 00123 wr_cmd8(0xD2); //Set power for normal mode 00124 wr_data8(0x01); 00125 wr_data8(0x44); 00126 00127 wr_cmd8(0xC8); //Set gamma 00128 wr_data8(0x00); 00129 wr_data8(0x77); 00130 wr_data8(0x77); 00131 wr_data8(0x00); 00132 wr_data8(0x04); 00133 wr_data8(0x00); 00134 wr_data8(0x00); 00135 wr_data8(0x00); 00136 wr_data8(0x77); 00137 wr_data8(0x00); 00138 wr_data8(0x00); 00139 wr_data8(0x08); 00140 wr_data8(0x00); 00141 wr_data8(0x00); 00142 wr_data8(0x00); 00143 00144 wr_cmd8(0xCA); //Set DGC LUT 00145 wr_data8(0x00); 00146 00147 wr_cmd8(0xEA); //3-Gamma Function Control 00148 wr_data8(0x80); // enable 00149 00150 wr_cmd8(0x29); // Set_display_on 00151 thread_sleep_for(150); 00152 00153 }
Generated on Tue Jul 12 2022 13:49:07 by
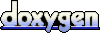