
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
GPDMA Class Reference
Wrapper for the General Purpose DMA controller. More...
#include <GPDMA.h>
Public Member Functions | |
void | init () |
Initializes the GPDMA. | |
void | deinit () |
Shuts down the GPDMA. | |
DMAChannels | acquireChannel (void(*irqfunc)(void), DMAChannels suggested=DMACh3) |
Get a free GPDMA channel for one DMA connection. | |
void | releaseChannel (DMAChannels ch) |
Release a previously acquired channel. | |
LPC_GPDMACH_TypeDef * | getDirectRegisters (DMAChannels ch) |
Returns the raw registers for the GPDMA channel for direct manipulation. | |
bool | transfer (GPDMA_Channel_CFG_T *cfg, uint32_t CtrlWord, uint32_t LinkListItem, uint8_t SrcPeripheral, uint8_t DstPeripheral) |
Does the actual DMA transfer. | |
Static Public Member Functions | |
static GPDMA & | instance () |
Get the only instance of the GPDMA. |
Detailed Description
Wrapper for the General Purpose DMA controller.
The GPDMA class allows multiple users of DMA to claim one or more of the eight available DMA channels and to register handlers for the interrupts. This centralized control is needed as there are eight channels but only one DMA IRQ.
By having to request a channel to use it is possible to avoid having hardcoded DMA channel number in the code
An example of use:
void handler() { _eventSuccess = LPC_GPDMA->IntTCStat & (1<<ch); LPC_GPDMA->IntTCClear = (1<<ch); } void foo() { GPDMA* dma = &GPDMA::instance(); dma->init(); DMAChannels ch = dma->acquireChannel(handler) if (ch == DMACh_Unavailable) { // handle error } // prepare the channel data (see e.g. gpdma_transfer_from_mci() in MCIFileSystem) ... // start the transfer dma->transfer(&cfg, ctrl_word, 0, GPDMA_CONN_SDC, GPDMA_CONN_MEMORY); // wait for transfer to complete (e.g. by handler() setting a flag) ... // return the channel now that it is no longer needed dma->releaseChannel(ch); }
Definition at line 137 of file GPDMA.h.
Member Function Documentation
GPDMA::DMAChannels acquireChannel | ( | void(*)(void) | irqfunc, |
DMAChannels | suggested = DMACh3 |
||
) |
Get a free GPDMA channel for one DMA connection.
The function will start to look for the suggested channel. If it is in use then the next, higher, channel number will be tested. The default is set to use DMACh3 which has medium priority.
To acquire a channel with the highest available priority call this function with DMACh0 as parameter.
- Parameters:
-
func the interrupt callback function suggested the first channel to look for
- Returns:
- DMACh0 to DMACh7 on success DMACh_Unavailable if there are no channels available
void deinit | ( | ) |
LPC_GPDMACH_TypeDef * getDirectRegisters | ( | DMAChannels | ch ) |
void init | ( | ) |
static GPDMA& instance | ( | ) | [static] |
void releaseChannel | ( | DMAChannels | ch ) |
bool transfer | ( | GPDMA_Channel_CFG_T * | cfg, |
uint32_t | CtrlWord, | ||
uint32_t | LinkListItem, | ||
uint8_t | SrcPeripheral, | ||
uint8_t | DstPeripheral | ||
) |
Does the actual DMA transfer.
- Parameters:
-
cfg the DMA configuration CtrlWord data for the DMA channel's CONTROL register LinkListItem address to the linked list or 0 if not used SrcPeripheral source of the transfer (GPDMA_CONN_MEMORY or GPDMA_CONN_SDC) DstPeripheral destination of the transfer (GPDMA_CONN_MEMORY or GPDMA_CONN_SDC)
- Returns:
- True on success, false on failure
Generated on Tue Jul 12 2022 14:18:31 by
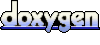