
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
DMBoard.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "DMBoard.h" 00019 00020 #if defined(DM_BOARD_USE_DISPLAY) 00021 #include "BiosDisplay.h" 00022 #endif 00023 #if defined(DM_BOARD_USE_TOUCH) 00024 #include "BiosTouch.h" 00025 #endif 00026 00027 #if defined(DM_BOARD_ENABLE_MEASSURING_PINS) 00028 #include "meas.h" 00029 #endif 00030 00031 #include "dm_rtc.h" 00032 00033 /****************************************************************************** 00034 * Configuration Compatibility Control 00035 *****************************************************************************/ 00036 00037 #if defined(DM_BOARD_USE_USB_DEVICE) && defined(DM_BOARD_USE_USB_HOST) 00038 #error The hardware supports either USB Device or USB Host - not both at the same time 00039 #endif 00040 00041 #if defined(DM_BOARD_USE_USBSERIAL_IN_RTOSLOG) && !defined(DM_BOARD_USE_USB_DEVICE) 00042 #error Cannot use USBSerial in RtosLog without DM_BOARD_USE_USB_DEVICE 00043 #endif 00044 00045 #if defined(DM_BOARD_USE_TOUCH) && !defined(DM_BOARD_USE_DISPLAY) 00046 #error Cannot have touch controller without a display! 00047 #endif 00048 00049 /****************************************************************************** 00050 * Defines and typedefs 00051 *****************************************************************************/ 00052 00053 #if defined(DM_BOARD_DISABLE_STANDARD_PRINTF) 00054 class DevNull : public Stream { 00055 00056 public: 00057 DevNull(const char *name=NULL) : Stream(name) {} 00058 00059 protected: 00060 virtual int _getc() {return 0;} 00061 virtual int _putc(int c) {return c;} 00062 }; 00063 00064 static DevNull null("null"); 00065 #endif 00066 00067 00068 /****************************************************************************** 00069 * Local variables 00070 *****************************************************************************/ 00071 00072 /****************************************************************************** 00073 * Private Functions 00074 *****************************************************************************/ 00075 00076 DMBoard::DMBoard() : 00077 _initialized(false), 00078 #if defined(DM_BOARD_USE_MCI_FS) 00079 _mcifs(P4_16), 00080 _mciFatFs("mci"), 00081 #endif 00082 #if defined(DM_BOARD_USE_QSPI_FS) 00083 _qspifs("qspi"), 00084 #endif 00085 _buzzer(P1_5), 00086 _button(P2_10), 00087 _led1(LED1), 00088 _led2(LED2), 00089 _led3(LED3), 00090 _led4(LED4) 00091 { 00092 } 00093 00094 DMBoard::~DMBoard() 00095 { 00096 } 00097 00098 /****************************************************************************** 00099 * Public Functions 00100 *****************************************************************************/ 00101 00102 DMBoard::BoardError DMBoard::init() 00103 { 00104 BoardError err = Ok; 00105 if (!_initialized) { 00106 do { 00107 00108 /* 00109 * By default mbed OS 5 comes with the MPU enabled and prevents execution 00110 * from RAM and writes to ROM. We need to be able to execute from EAM 00111 * (e.g. the BIOS functionality) and also write to ROM area (we are e.g. 00112 * using the EEPROM peripheral in the LPC4088 which has an address space 00113 * within the ROM area). 00114 */ 00115 mbed_mpu_manager_lock_ram_execution(); 00116 mbed_mpu_manager_lock_rom_write(); 00117 00118 // Turn off the buzzer 00119 _buzzer.period_ms(1); 00120 _buzzer = 0; 00121 00122 // Make sure the button is configured correctly 00123 _button.mode(PullUp); 00124 00125 // Turn off all LEDs 00126 _led1 = 1; 00127 _led2 = 1; 00128 _led3 = 0; 00129 _led4 = 0; 00130 00131 // Make sure that the logger is always initialized even if 00132 // other initialization tasks fail 00133 _logger.init(); 00134 00135 #if defined(DM_BOARD_ENABLE_MEASSURING_PINS) 00136 _INTERNAL_INIT_MEAS(); 00137 #endif 00138 00139 #if defined(DM_BOARD_DISABLE_STANDARD_PRINTF) 00140 // Kill all ouput of calls to printf() so that there is no 00141 // simultaneous calls into the non-thread-safe standard libraries. 00142 // User code should use the RtosLogger anyway. 00143 freopen("/null", "w", stdout); 00144 #endif 00145 00146 #if defined(DM_BOARD_USE_MCI_FS) 00147 _mciFatFs.mount(&_mcifs); 00148 #endif 00149 00150 #if defined(DM_BOARD_USE_QSPI) || defined(DM_BOARD_USE_QSPI_FS) 00151 if (SPIFI::instance().init() != SPIFI::Ok) { 00152 err = SpifiError; 00153 break; 00154 } 00155 #endif 00156 00157 #if defined(DM_BOARD_USE_DISPLAY) 00158 if (display()->init() != Display::DisplayError_Ok) { 00159 err = DisplayError; 00160 break; 00161 } 00162 #endif 00163 00164 #if defined(DM_BOARD_USE_TOUCH) 00165 if (BiosTouch::instance().init() != TouchPanel::TouchError_Ok) { 00166 err = TouchError; 00167 break; 00168 } 00169 #endif 00170 00171 #if defined(DM_BOARD_USE_REGISTRY) 00172 if (Registry::instance().load() != Registry::Ok) { 00173 err = RegistryError; 00174 break; 00175 } 00176 #endif 00177 /* 00178 * With mbed OS 5 the rtc driver in target/hal has been disabled 00179 * Adding an external rtc driver instead. 00180 */ 00181 attach_rtc(&dm_read_rtc, &dm_write_rtc, &dm_init_rtc, &dm_isenabled_rtc); 00182 00183 _initialized = true; 00184 } while(0); 00185 } 00186 return err; 00187 } 00188 00189 void DMBoard::setLED(Leds led, bool on) 00190 { 00191 switch(led) { 00192 case Led1: 00193 _led1 = (on ? 0 : 1); 00194 break; 00195 case Led2: 00196 _led2 = (on ? 0 : 1); 00197 break; 00198 case Led3: 00199 _led3 = (on ? 1 : 0); 00200 break; 00201 case Led4: 00202 _led4 = (on ? 1 : 0); 00203 break; 00204 } 00205 } 00206 00207 void DMBoard::buzzer(int frequency, int duration_ms) 00208 { 00209 if (frequency <= 0) { 00210 _buzzer = 0; 00211 } else { 00212 _buzzer.period_us(1000000/frequency); 00213 _buzzer = 0.5; 00214 } 00215 if (duration_ms > 0) { 00216 ThisThread::sleep_for(duration_ms); 00217 _buzzer = 0; 00218 } 00219 } 00220 00221 bool DMBoard::buttonPressed() 00222 { 00223 return _button.read() == 0; 00224 } 00225 00226 TouchPanel* DMBoard::touchPanel() 00227 { 00228 #if defined(DM_BOARD_USE_TOUCH) 00229 if (BiosTouch::instance().isTouchSupported()) { 00230 return &BiosTouch::instance(); 00231 } 00232 #endif 00233 return NULL; 00234 } 00235 00236 Display* DMBoard::display() 00237 { 00238 #if defined(DM_BOARD_USE_DISPLAY) 00239 return &BiosDisplay::instance(); 00240 #else 00241 return NULL; 00242 #endif 00243 }
Generated on Tue Jul 12 2022 14:18:31 by
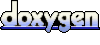