
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
BiosTouch Class Reference
Glue between the BIOS and the TouchPanel interface. More...
#include <BiosTouch.h>
Inherits TouchPanel.
Public Member Functions | |
TouchError | init () |
Initialize the touch controller. | |
bool | isTouchSupported () |
Tests if a touch controller is available or not. | |
virtual TouchError | read (touch_coordinate_t &coord) |
Read coordinates from the touch panel. | |
virtual TouchError | read (touch_coordinate_t *coord, int num) |
Read up to num coordinates from the touch panel. | |
virtual TouchError | info (bool *resistive, int *maxPoints, bool *calibrated) |
Returns information about the touch panel. | |
virtual TouchError | calibrateStart () |
Start to calibrate the display. | |
virtual TouchError | getNextCalibratePoint (uint16_t *x, uint16_t *y, bool *last=NULL) |
Get the next calibration point. | |
virtual TouchError | waitForCalibratePoint (bool *morePoints, uint32_t timeout) |
Wait for a calibration point to have been pressed and recored. | |
Static Public Member Functions | |
static BiosTouch & | instance () |
Get the only instance of the BiosTouch. |
Detailed Description
Glue between the BIOS and the TouchPanel interface.
Definition at line 31 of file BiosTouch.h.
Member Function Documentation
BiosTouch::TouchError calibrateStart | ( | ) | [virtual] |
Start to calibrate the display.
- Returns:
- Ok on success An error code on failure
Implements TouchPanel.
Definition at line 346 of file BiosTouch.cpp.
BiosTouch::TouchError getNextCalibratePoint | ( | uint16_t * | x, |
uint16_t * | y, | ||
bool * | last = NULL |
||
) | [virtual] |
Get the next calibration point.
Draw an indicator on the screen at the coordinates and ask the user to press/click on the indicator. Please note that waitForCalibratePoint() must be called after this method.
- Parameters:
-
x the x coordinate is written to this argument y the y coordinate is written to this argument last true if this is the last coordinate in the series
- Returns:
- Ok on success An error code on failure
Implements TouchPanel.
Definition at line 357 of file BiosTouch.cpp.
BiosTouch::TouchError info | ( | bool * | resistive, |
int * | maxPoints, | ||
bool * | calibrated | ||
) | [virtual] |
Returns information about the touch panel.
- Parameters:
-
resistive true for Resistive, false for Capacitive maxPoints the maximum number of simultaneous touches calibration true if the controller can be calibrated
- Returns:
- Ok on success An error code on failure
Implements TouchPanel.
Definition at line 324 of file BiosTouch.cpp.
BiosTouch::TouchError init | ( | ) |
Initialize the touch controller.
This method must be called before calibrating or reading data from the controller
- Returns:
- Ok on success An error code on failure
Definition at line 252 of file BiosTouch.cpp.
static BiosTouch& instance | ( | ) | [static] |
Get the only instance of the BiosTouch.
- Returns:
- The display
Definition at line 38 of file BiosTouch.h.
bool isTouchSupported | ( | ) |
Tests if a touch controller is available or not.
Note that this function only returns a valid value after the display has been intitialized.
- Returns:
- true if there is a touch controller
Definition at line 336 of file BiosTouch.cpp.
BiosTouch::TouchError read | ( | touch_coordinate_t * | coord, |
int | num | ||
) | [virtual] |
Read up to num coordinates from the touch panel.
- Parameters:
-
coords a list of at least num coordinates num the number of coordinates to read
- Returns:
- Ok on success An error code on failure
Implements TouchPanel.
Definition at line 313 of file BiosTouch.cpp.
BiosTouch::TouchError read | ( | touch_coordinate_t & | coord ) | [virtual] |
Read coordinates from the touch panel.
In case of multitouch (capacitive touch screen) only the first touch will be returned.
- Parameters:
-
coord pointer to coordinate object. The read coordinates will be written to this object.
- Returns:
- Ok on success An error code on failure
Implements TouchPanel.
Definition at line 302 of file BiosTouch.cpp.
BiosTouch::TouchError waitForCalibratePoint | ( | bool * | morePoints, |
uint32_t | timeout | ||
) | [virtual] |
Wait for a calibration point to have been pressed and recored.
This method must be called just after getNextCalibratePoint().
- Parameters:
-
morePoints true is written to this argument if there are more calibrations points available; otherwise it will be false timeout maximum number of milliseconds to wait for a calibration point. Set this argument to 0 to wait indefinite.
- Returns:
- Ok on success An error code on failure
Implements TouchPanel.
Definition at line 368 of file BiosTouch.cpp.
Generated on Tue Jul 12 2022 14:18:31 by
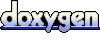