Project Submission (late)
Dependencies: mbed
Player Class Reference
#include <Player.h>
Public Member Functions | |
Player (Maze *maze) | |
Create a Player object. | |
bool | checkLocation (Vector2Di origin, double angle, int checkVal) |
Check's the value of the cell in a specified direction from the current cell. | |
void | walk () |
Move the player's position forward one in their current direction. | |
void | turnLeft () |
Rotates the player's current direction by 90 degrees counter-clockwise. | |
void | turnRight () |
Rotates the player's current direction by 90 degrees clockwise. | |
void | stepBack () |
Moves the player one cell backwards. |
Detailed Description
Player Class.
This class exists to store information about the player's location in the maze. more specifically, it stores their coordinates and their direction, both as vectors. It also facilitates the player's control of the camera via the gamepad.
Version 1.0
- Date:
- May 2019
Definition at line 16 of file Player.h.
Constructor & Destructor Documentation
Create a Player object.
- Parameters:
-
maze - a maze object so the Player class has access to the maze matrix
This constructor does not set the player's position (not to the desired position anyway).
The player's proper position is assigned after the Player object's initial creation.
Definition at line 7 of file Player.cpp.
Member Function Documentation
bool checkLocation | ( | Vector2Di | origin, |
double | angle, | ||
int | checkVal | ||
) |
Check's the value of the cell in a specified direction from the current cell.
- Parameters:
-
origin - vector of the co-ordinates to check from. Not necessarily the Player's current position. angle - double representing the angle to rotate by before checking in front, will always be a multiple of PI/2. checkVal - integer, checkLocation returns true if the checked cell has this value.
Definition at line 21 of file Player.cpp.
void stepBack | ( | ) |
Moves the player one cell backwards.
Will not work if the cell behind the player is not open.
Definition at line 46 of file Player.cpp.
void turnLeft | ( | ) |
Rotates the player's current direction by 90 degrees counter-clockwise.
Definition at line 42 of file Player.cpp.
void turnRight | ( | ) |
Rotates the player's current direction by 90 degrees clockwise.
Definition at line 44 of file Player.cpp.
void walk | ( | ) |
Move the player's position forward one in their current direction.
Performs validity check first. Won't increment position if cell infront is solid (i.e. 1)
Definition at line 34 of file Player.cpp.
Generated on Thu Jul 14 2022 20:06:29 by
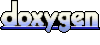