Project Submission (late)
Dependencies: mbed
Player.cpp
00001 #include "Player.h" 00002 #include "mbed.h" 00003 00004 const Vector2Di UP = {0,1}; 00005 const Vector2Di DOWN = {0,-1}; 00006 00007 Player::Player(Maze* mazePtr) { 00008 maze = mazePtr; 00009 pos = UP; // {0,1} is a temporary value to initialise the variable 00010 direction = DOWN; // due to matrices index increasing as you go down 00011 // and their acces being [row][column], the standard (x,y) co-ordinate system is 00012 // effectively reversed so even though the direction is down, from our perspective 00013 // (if we were looking down on the maze) it would be up. 00014 } 00015 00016 /* Function used to check the value of a cell in the maze from any given cell 00017 (not necessarily the player's current position) 00018 this function is used a lot in the Drawer (along with other information from the player) 00019 so it is important the function is not bound to the player's current location 00020 */ 00021 bool Player::checkLocation(Vector2Di origin, double angle, int checkVal) { 00022 Vector2Di peekDirection = direction; 00023 peekDirection.rotateVector(angle); 00024 origin.addVector(peekDirection); 00025 if (maze->mazeMatrix[origin.y][origin.x] == checkVal) { 00026 // eg if index == 1 that indicates a solid cell 00027 // or if index == 2 that is the maze exit 00028 return true; 00029 } else { 00030 return false; 00031 } 00032 } 00033 00034 void Player::walk() { 00035 if (checkLocation(pos,0,0)) { 00036 printf("v = (%i, %i)\nwalking\n", pos.x, pos.y); 00037 pos.addVector(direction); 00038 printf("v = (%i, %i)\n", pos.x, pos.y); 00039 } 00040 }; 00041 00042 void Player::turnLeft() { direction.rotateVector(-PI/2); }; 00043 00044 void Player::turnRight() { direction.rotateVector(PI/2); }; 00045 00046 void Player::stepBack() { 00047 if (checkLocation(pos,PI,0)) { 00048 pos.addVector(-direction); 00049 } 00050 };
Generated on Thu Jul 14 2022 20:06:29 by
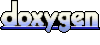