High level Bluetooth Low Energy API and radio abstraction layer
Dependents: BLE_ANCS_SDAPI BLE_temperature BLE_HeartRate BLE_ANCS_SDAPI_IRC ... more
UUID.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __UUID_H__ 00018 #define __UUID_H__ 00019 00020 #include <stdint.h> 00021 #include <string.h> 00022 #include <algorithm> 00023 00024 #include "blecommon.h" 00025 00026 /** 00027 * A trivial converter for single hexadecimal character to an unsigned integer. 00028 * 00029 * @param c 00030 * Hexadecimal character. 00031 * 00032 * @return The corresponding value as unsigned integer. 00033 */ 00034 static uint8_t char2int(char c) { 00035 if ((c >= '0') && (c <= '9')) { 00036 return c - '0'; 00037 } else if ((c >= 'a') && (c <= 'f')) { 00038 return c - 'a' + 10; 00039 } else if ((c >= 'A') && (c <= 'F')) { 00040 return c - 'A' + 10; 00041 } else { 00042 return 0; 00043 } 00044 } 00045 00046 /** 00047 * An instance of this class represents a Universally Unique Identifier (UUID) 00048 * in the BLE API. 00049 */ 00050 class UUID { 00051 public: 00052 /** 00053 * Enumeration of the possible types of UUIDs in BLE with regards to length. 00054 */ 00055 enum UUID_Type_t { 00056 UUID_TYPE_SHORT = 0, /**< Short 16-bit UUID. */ 00057 UUID_TYPE_LONG = 1 /**< Full 128-bit UUID. */ 00058 }; 00059 00060 /** 00061 * Enumeration to specify byte ordering of the long version of the UUID. 00062 */ 00063 typedef enum { 00064 MSB, /**< Most-significant byte first (at the smallest address) */ 00065 LSB /**< least-significant byte first (at the smallest address) */ 00066 } ByteOrder_t; 00067 00068 /** 00069 * Type for a 16-bit UUID. 00070 */ 00071 typedef uint16_t ShortUUIDBytes_t; 00072 00073 /** 00074 * Length of a long UUID in bytes. 00075 */ 00076 static const unsigned LENGTH_OF_LONG_UUID = 16; 00077 /** 00078 * Type for a 128-bit UUID. 00079 */ 00080 typedef uint8_t LongUUIDBytes_t[LENGTH_OF_LONG_UUID]; 00081 00082 /** 00083 * Maximum length of a string representation of a UUID not including the 00084 * null termination ('\0'): two characters per 00085 * byte plus four '-' characters. 00086 */ 00087 static const unsigned MAX_UUID_STRING_LENGTH = LENGTH_OF_LONG_UUID * 2 + 4; 00088 00089 public: 00090 00091 /** 00092 * Creates a new 128-bit UUID. 00093 * 00094 * @note The UUID is a unique 128-bit (16 byte) ID used to identify 00095 * different service or characteristics on the BLE device. 00096 * 00097 * @param stringUUID 00098 * The 128-bit (16-byte) UUID as a human readable const-string. 00099 * Format: XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX 00100 * Upper and lower case supported. Hyphens are optional, but only 00101 * upto four of them. The UUID is stored internally as a 16 byte 00102 * array, LSB (little endian), which is opposite from the string. 00103 */ 00104 UUID(const char* stringUUID) : type(UUID_TYPE_LONG), baseUUID(), shortUUID(0) { 00105 bool nibble = false; 00106 uint8_t byte = 0; 00107 size_t baseIndex = 0; 00108 uint8_t tempUUID[LENGTH_OF_LONG_UUID]; 00109 00110 /* 00111 * Iterate through string, abort if NULL is encountered prematurely. 00112 * Ignore upto four hyphens. 00113 */ 00114 for (size_t index = 0; (index < MAX_UUID_STRING_LENGTH) && (baseIndex < LENGTH_OF_LONG_UUID); index++) { 00115 if (stringUUID[index] == '\0') { 00116 /* Error abort */ 00117 break; 00118 } else if (stringUUID[index] == '-') { 00119 /* Ignore hyphen */ 00120 continue; 00121 } else if (nibble) { 00122 /* Got second nibble */ 00123 byte |= char2int(stringUUID[index]); 00124 nibble = false; 00125 00126 /* Store copy */ 00127 tempUUID[baseIndex++] = byte; 00128 } else { 00129 /* Got first nibble */ 00130 byte = char2int(stringUUID[index]) << 4; 00131 nibble = true; 00132 } 00133 } 00134 00135 /* Populate internal variables if string was successfully parsed */ 00136 if (baseIndex == LENGTH_OF_LONG_UUID) { 00137 setupLong(tempUUID, UUID::MSB); 00138 } else { 00139 const uint8_t sig[] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x10, 0x00, 00140 0x80, 0x00, 0x00, 0x80, 0x5F, 0x9B, 0x34, 0xFB }; 00141 setupLong(sig, UUID::MSB); 00142 } 00143 } 00144 00145 /** 00146 * Creates a new 128-bit UUID. 00147 * 00148 * @param[in] longUUID 00149 * The 128-bit (16-byte) UUID value. 00150 * @param[in] order 00151 * The bit order of the UUID, MSB by default. 00152 * 00153 * @note The UUID is a unique 128-bit (16 byte) ID used to identify 00154 * different service or characteristics on the BLE device. 00155 */ 00156 UUID(const LongUUIDBytes_t longUUID, ByteOrder_t order = UUID::MSB) : type(UUID_TYPE_LONG), baseUUID(), shortUUID(0) { 00157 setupLong(longUUID, order); 00158 } 00159 00160 /** 00161 * Creates a new 16-bit UUID. 00162 * 00163 * For efficiency, and because 16 bytes would take a large chunk of the 00164 * 27-byte data payload length of the Link Layer, the BLE specification adds 00165 * two additional UUID formats: 16-bit and 32-bit UUIDs. These shortened 00166 * formats can be used only with UUIDs that are defined in the Bluetooth 00167 * specification (listed by the Bluetooth SIG as standard 00168 * Bluetooth UUIDs). 00169 * 00170 * To reconstruct the full 128-bit UUID from the shortened version, insert 00171 * the 16-bit short value (indicated by xxxx, including leading zeros) into 00172 * the Bluetooth Base UUID: 00173 * 00174 * 0000xxxx-0000-1000-8000-00805F9B34FB 00175 * 00176 * @param[in] _shortUUID 00177 * The short UUID value. 00178 * 00179 * @note Shortening is not available for UUIDs that are not derived from the 00180 * Bluetooth Base UUID. Such non-standard UUIDs are commonly called 00181 * vendor-specific UUIDs. In these cases, you’ll need to use the full 00182 * 128-bit UUID value at all times. 00183 * 00184 * @note The UUID is a unique 16-bit (2 byte) ID used to identify 00185 * different service or characteristics on the BLE device. 00186 * 00187 * @note We do not yet support 32-bit shortened UUIDs. 00188 */ 00189 UUID(ShortUUIDBytes_t _shortUUID) : type(UUID_TYPE_SHORT), baseUUID(), shortUUID(_shortUUID) { 00190 /* Empty */ 00191 } 00192 00193 /** 00194 * Copy constructor. 00195 * 00196 * @param[in] source 00197 * The UUID to copy. 00198 */ 00199 UUID(const UUID &source) { 00200 type = source.type; 00201 shortUUID = source.shortUUID; 00202 memcpy(baseUUID, source.baseUUID, LENGTH_OF_LONG_UUID); 00203 } 00204 00205 /** 00206 * The empty constructor. 00207 * 00208 * @note The type of the resulting UUID instance is UUID_TYPE_SHORT and the 00209 * value BLE_UUID_UNKNOWN. 00210 */ 00211 UUID(void) : type(UUID_TYPE_SHORT), shortUUID(BLE_UUID_UNKNOWN) { 00212 /* empty */ 00213 } 00214 00215 /** 00216 * Fill in a 128-bit UUID; this is useful when the UUID is not known at the 00217 * time of the object construction. 00218 * 00219 * @param[in] longUUID 00220 * The UUID value to copy. 00221 * @param[in] order 00222 * The byte ordering of the UUID at @p longUUID. 00223 */ 00224 void setupLong(const LongUUIDBytes_t longUUID, ByteOrder_t order = UUID::MSB) { 00225 type = UUID_TYPE_LONG; 00226 if (order == UUID::MSB) { 00227 /* 00228 * Switch endian. Input is big-endian, internal representation 00229 * is little endian. 00230 */ 00231 std::reverse_copy(longUUID, longUUID + LENGTH_OF_LONG_UUID, baseUUID); 00232 } else { 00233 std::copy(longUUID, longUUID + LENGTH_OF_LONG_UUID, baseUUID); 00234 } 00235 shortUUID = (uint16_t)((baseUUID[13] << 8) | (baseUUID[12])); 00236 } 00237 00238 public: 00239 /** 00240 * Check whether this UUID is short or long. 00241 * 00242 * @return UUID_TYPE_SHORT if the UUID is short, UUID_TYPE_LONG otherwise. 00243 */ 00244 UUID_Type_t shortOrLong(void) const { 00245 return type; 00246 } 00247 00248 /** 00249 * Get a pointer to the UUID value based on the current UUID type. 00250 * 00251 * @return A pointer to the short UUID if the type is set to 00252 * UUID_TYPE_SHORT. Otherwise, a pointer to the long UUID if the 00253 * type is set to UUID_TYPE_LONG. 00254 */ 00255 const uint8_t *getBaseUUID(void) const { 00256 if (type == UUID_TYPE_SHORT) { 00257 return (const uint8_t*)&shortUUID; 00258 } else { 00259 return baseUUID; 00260 } 00261 } 00262 00263 /** 00264 * Get the short UUID. 00265 * 00266 * @return The short UUID. 00267 */ 00268 ShortUUIDBytes_t getShortUUID(void) const { 00269 return shortUUID; 00270 } 00271 00272 /** 00273 * Get the length (in bytes) of the UUID based on its type. 00274 * 00275 * @retval sizeof(ShortUUIDBytes_t) if the UUID type is UUID_TYPE_SHORT. 00276 * @retval LENGTH_OF_LONG_UUID if the UUID type is UUID_TYPE_LONG. 00277 */ 00278 uint8_t getLen(void) const { 00279 return ((type == UUID_TYPE_SHORT) ? sizeof(ShortUUIDBytes_t) : LENGTH_OF_LONG_UUID); 00280 } 00281 00282 /** 00283 * Overload == operator to enable UUID comparisons. 00284 * 00285 * @param[in] other 00286 * The other UUID in the comparison. 00287 * 00288 * @return true if this == @p other, false otherwise. 00289 */ 00290 bool operator== (const UUID &other) const { 00291 if ((this->type == UUID_TYPE_SHORT) && (other.type == UUID_TYPE_SHORT) && 00292 (this->shortUUID == other.shortUUID)) { 00293 return true; 00294 } 00295 00296 if ((this->type == UUID_TYPE_LONG) && (other.type == UUID_TYPE_LONG) && 00297 (memcmp(this->baseUUID, other.baseUUID, LENGTH_OF_LONG_UUID) == 0)) { 00298 return true; 00299 } 00300 00301 return false; 00302 } 00303 00304 /** 00305 * Overload != operator to enable UUID comparisons. 00306 * 00307 * @param[in] other 00308 * The other UUID in the comparison. 00309 * 00310 * @return true if this != @p other, false otherwise. 00311 */ 00312 bool operator!= (const UUID &other) const { 00313 return !(*this == other); 00314 } 00315 00316 private: 00317 /** 00318 * The UUID type. Refer to UUID_Type_t. 00319 */ 00320 UUID_Type_t type; 00321 /** 00322 * The long UUID value. 00323 */ 00324 LongUUIDBytes_t baseUUID; 00325 /** 00326 * The short UUID value. 00327 */ 00328 ShortUUIDBytes_t shortUUID; 00329 }; 00330 00331 #endif // ifndef __UUID_H__
Generated on Tue Jul 12 2022 12:49:02 by
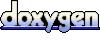