High level Bluetooth Low Energy API and radio abstraction layer
Dependents: BLE_ANCS_SDAPI BLE_temperature BLE_HeartRate BLE_ANCS_SDAPI_IRC ... more
SafeBool.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_API_SAFE_BOOL_H_ 00018 #define BLE_API_SAFE_BOOL_H_ 00019 00020 /* Safe bool idiom, see : http://www.artima.com/cppsource/safebool.html */ 00021 00022 namespace SafeBool_ { 00023 /** 00024 * @brief Base class for all intances of SafeBool. 00025 * This base class reduces instantiation of trueTag function. 00026 */ 00027 class base { 00028 template<typename> 00029 friend class SafeBool; 00030 00031 protected: 00032 /** 00033 * The bool type is a pointer to method which can be used in boolean context. 00034 */ 00035 typedef void (base::*BoolType_t)() const; 00036 00037 /** 00038 * Non implemented call, use to disallow conversion between unrelated types. 00039 */ 00040 void invalidTag() const; 00041 00042 /** 00043 * Member function which indicate true value. 00044 */ 00045 void trueTag() const {} 00046 }; 00047 00048 00049 } 00050 00051 /** 00052 * @brief template class SafeBool use CRTP to made boolean conversion easy and correct. 00053 * Derived class should implement the function bool toBool() const to make this work. Inheritance 00054 * should be public. 00055 * 00056 * @tparam T Type of the derived class 00057 * 00058 * @code 00059 * 00060 * class A : public SafeBool<A> { 00061 * public: 00062 * 00063 * // boolean conversion 00064 * bool toBool() { 00065 * 00066 * } 00067 * }; 00068 * 00069 * class B : public SafeBool<B> { 00070 * public: 00071 * 00072 * // boolean conversion 00073 * bool toBool() const { 00074 * 00075 * } 00076 * }; 00077 * 00078 * A a; 00079 * B b; 00080 * 00081 * // will compile 00082 * if(a) { 00083 * 00084 * } 00085 * 00086 * // compilation error 00087 * if(a == b) { 00088 * 00089 * } 00090 * 00091 * 00092 * @endcode 00093 */ 00094 template <typename T> 00095 class SafeBool : public SafeBool_::base { 00096 public: 00097 /** 00098 * Bool operator implementation, derived class has to provide bool toBool() const function. 00099 */ 00100 operator BoolType_t() const { 00101 return (static_cast<const T*>(this))->toBool() 00102 ? &SafeBool<T>::trueTag : 0; 00103 } 00104 }; 00105 00106 /** 00107 * Avoid conversion to bool between different classes. 00108 */ 00109 template <typename T, typename U> 00110 void operator==(const SafeBool<T>& lhs,const SafeBool<U>& rhs) { 00111 lhs.invalidTag(); 00112 // return false; 00113 } 00114 00115 /** 00116 * Avoid conversion to bool between different classes. 00117 */ 00118 template <typename T,typename U> 00119 void operator!=(const SafeBool<T>& lhs,const SafeBool<U>& rhs) { 00120 lhs.invalidTag(); 00121 // return false; 00122 } 00123 00124 #endif /* BLE_API_SAFE_BOOL_H_ */
Generated on Tue Jul 12 2022 12:49:01 by
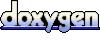