High level Bluetooth Low Energy API and radio abstraction layer
Dependents: BLE_ANCS_SDAPI BLE_temperature BLE_HeartRate BLE_ANCS_SDAPI_IRC ... more
GattService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVICE_H__ 00018 #define __GATT_SERVICE_H__ 00019 00020 #include "UUID.h" 00021 #include "GattCharacteristic.h" 00022 00023 class GattService { 00024 public: 00025 enum { 00026 UUID_ALERT_NOTIFICATION_SERVICE = 0x1811, 00027 UUID_BATTERY_SERVICE = 0x180F, 00028 UUID_BLOOD_PRESSURE_SERVICE = 0x1810, 00029 UUID_CURRENT_TIME_SERVICE = 0x1805, 00030 UUID_CYCLING_SPEED_AND_CADENCE = 0x1816, 00031 UUID_DEVICE_INFORMATION_SERVICE = 0x180A, 00032 UUID_ENVIRONMENTAL_SERVICE = 0x181A, 00033 UUID_GLUCOSE_SERVICE = 0x1808, 00034 UUID_HEALTH_THERMOMETER_SERVICE = 0x1809, 00035 UUID_HEART_RATE_SERVICE = 0x180D, 00036 UUID_HUMAN_INTERFACE_DEVICE_SERVICE = 0x1812, 00037 UUID_IMMEDIATE_ALERT_SERVICE = 0x1802, 00038 UUID_LINK_LOSS_SERVICE = 0x1803, 00039 UUID_NEXT_DST_CHANGE_SERVICE = 0x1807, 00040 UUID_PHONE_ALERT_STATUS_SERVICE = 0x180E, 00041 UUID_REFERENCE_TIME_UPDATE_SERVICE = 0x1806, 00042 UUID_RUNNING_SPEED_AND_CADENCE = 0x1814, 00043 UUID_SCAN_PARAMETERS_SERVICE = 0x1813, 00044 UUID_TX_POWER_SERVICE = 0x1804 00045 }; 00046 00047 public: 00048 /** 00049 * @brief Creates a new GattService using the specified 16-bit 00050 * UUID, value length, and properties. 00051 * 00052 * @note The UUID value must be unique and is normally >1. 00053 * 00054 * @param[in] uuid 00055 * The UUID to use for this service. 00056 * @param[in] characteristics 00057 * A pointer to an array of characteristics to be included within this service. 00058 * @param[in] numCharacteristics 00059 * The number of characteristics. 00060 */ 00061 GattService(const UUID &uuid, GattCharacteristic *characteristics[], unsigned numCharacteristics) : 00062 _primaryServiceID(uuid), 00063 _characteristicCount(numCharacteristics), 00064 _characteristics(characteristics), 00065 _handle(0) { 00066 /* empty */ 00067 } 00068 00069 /** 00070 * Get this service's UUID. 00071 * 00072 * @return A reference to the service's UUID. 00073 */ 00074 const UUID &getUUID(void) const { 00075 return _primaryServiceID; 00076 } 00077 00078 /** 00079 * Get handle of the service declaration attribute in the ATT table. 00080 * 00081 * @return The service's handle. 00082 */ 00083 uint16_t getHandle(void) const { 00084 return _handle; 00085 } 00086 00087 /** 00088 * Get the total number of characteristics within this service. 00089 * 00090 * @return The total number of characteristics within this service. 00091 */ 00092 uint8_t getCharacteristicCount(void) const { 00093 return _characteristicCount; 00094 } 00095 00096 /** 00097 * Set the handle of the service declaration attribute in the ATT table. 00098 * 00099 * @param[in] handle 00100 * The service's handle. 00101 */ 00102 void setHandle(uint16_t handle) { 00103 _handle = handle; 00104 } 00105 00106 /** 00107 * Get this service's characteristic at a specific index. 00108 * 00109 * @param[in] index 00110 * The index of the characteristic. 00111 * 00112 * @return A pointer to the characterisitic at index @p index. 00113 */ 00114 GattCharacteristic *getCharacteristic(uint8_t index) { 00115 if (index >= _characteristicCount) { 00116 return NULL; 00117 } 00118 00119 return _characteristics[index]; 00120 } 00121 00122 private: 00123 /** 00124 * This service's UUID. 00125 */ 00126 UUID _primaryServiceID; 00127 /** 00128 * Total number of characteristics within this service. 00129 */ 00130 uint8_t _characteristicCount; 00131 /** 00132 * An array with pointers to the characteristics added to this service. 00133 */ 00134 GattCharacteristic **_characteristics; 00135 /** 00136 * Handle of the service declaration attribute in the ATT table. 00137 * 00138 * @note This handle is generally assigned by the underlying BLE stack when the 00139 * service is added to the ATT table. 00140 */ 00141 uint16_t _handle; 00142 }; 00143 00144 #endif /* ifndef __GATT_SERVICE_H__ */
Generated on Tue Jul 12 2022 12:49:01 by
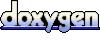