High level Bluetooth Low Energy API and radio abstraction layer
Dependents: BLE_ANCS_SDAPI BLE_temperature BLE_HeartRate BLE_ANCS_SDAPI_IRC ... more
BLEProtocol.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_PROTOCOL_H__ 00018 #define __BLE_PROTOCOL_H__ 00019 00020 #include <stddef.h> 00021 #include <stdint.h> 00022 #include <algorithm> 00023 00024 /** 00025 * A common namespace for types and constants used everywhere in BLE API. 00026 */ 00027 namespace BLEProtocol { 00028 /**< 00029 * A simple container for the enumeration of address-types for Protocol addresses. 00030 * 00031 * Adding a struct to encapsulate the contained enumeration prevents 00032 * polluting the BLEProtocol namespace with the enumerated values. It also 00033 * allows type-aliases for the enumeration while retaining the enumerated 00034 * values. i.e. doing: 00035 * typedef AddressType AliasedType; 00036 * 00037 * would allow the use of AliasedType::PUBLIC in code. 00038 */ 00039 struct AddressType { 00040 /**< Address-types for Protocol addresses. */ 00041 enum Type { 00042 PUBLIC = 0, 00043 RANDOM_STATIC, 00044 RANDOM_PRIVATE_RESOLVABLE, 00045 RANDOM_PRIVATE_NON_RESOLVABLE 00046 }; 00047 }; 00048 typedef AddressType::Type AddressType_t; /**< Alias for AddressType::Type */ 00049 00050 static const size_t ADDR_LEN = 6; /**< Length (in octets) of the BLE MAC address. */ 00051 typedef uint8_t AddressBytes_t[ADDR_LEN]; /**< 48-bit address, in LSB format. */ 00052 00053 /** 00054 * BLE address. It contains an address-type (AddressType_t) and bytes (AddressBytes_t). 00055 */ 00056 struct Address_t { 00057 AddressType_t type; /**< The type of the BLE address. */ 00058 AddressBytes_t address; /**< The BLE address. */ 00059 00060 /** 00061 * Construct an Address_t object with the supplied type and address. 00062 * 00063 * @param[in] typeIn 00064 * The BLE address type. 00065 * @param[in] addressIn 00066 * The BLE address. 00067 */ 00068 Address_t(AddressType_t typeIn, const AddressBytes_t& addressIn) : type(typeIn) { 00069 std::copy(addressIn, addressIn + ADDR_LEN, address); 00070 } 00071 00072 /** 00073 * Empty constructor. 00074 */ 00075 Address_t() : type(), address() { 00076 } 00077 }; 00078 }; 00079 00080 #endif /* __BLE_PROTOCOL_H__ */
Generated on Tue Jul 12 2022 12:49:01 by
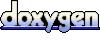