adaptation for book and plug demo
Fork of BLE_API by
CallChainOfFunctionPointersWithContext< ContextType > Class Template Reference
Group one or more functions in an instance of a CallChainOfFunctionPointersWithContext, then call them in sequence using CallChainOfFunctionPointersWithContext::call(). More...
#include <CallChainOfFunctionPointersWithContext.h>
Inherits SafeBool< CallChainOfFunctionPointersWithContext< ContextType > >.
Public Types | |
typedef FunctionPointerWithContext < ContextType > * | pFunctionPointerWithContext_t |
The type of each callback in the callchain. | |
Public Member Functions | |
CallChainOfFunctionPointersWithContext () | |
Create an empty chain. | |
pFunctionPointerWithContext_t | add (void(*function)(ContextType context)) |
Add a function at the front of the chain. | |
template<typename T > | |
pFunctionPointerWithContext_t | add (T *tptr, void(T::*mptr)(ContextType context)) |
Add a function at the front of the chain. | |
pFunctionPointerWithContext_t | add (const FunctionPointerWithContext< ContextType > &func) |
Add a function at the front of the chain. | |
bool | detach (const FunctionPointerWithContext< ContextType > &toDetach) |
Detach a function pointer from a callchain. | |
void | clear (void) |
Clear the call chain (remove all functions in the chain). | |
bool | hasCallbacksAttached (void) const |
Check whether the callchain contains any callbacks. | |
void | call (ContextType context) |
Call all the functions in the chain in sequence. | |
void | call (ContextType context) const |
Same as call() above, but const. | |
void | operator() (ContextType context) const |
Same as call(), but with function call operator. | |
bool | toBool () const |
Bool conversion operation. | |
operator BoolType_t () const | |
Bool operator implementation, derived class has to provide bool toBool() const function. | |
Protected Types | |
typedef void(base::* | BoolType_t )() const |
The bool type is a pointer to method which can be used in boolean context. | |
Protected Member Functions | |
void | invalidTag () const |
Non implemented call, use to disallow conversion between unrelated types. | |
void | trueTag () const |
Member function which indicate true value. |
Detailed Description
template<typename ContextType>
class CallChainOfFunctionPointersWithContext< ContextType >
Group one or more functions in an instance of a CallChainOfFunctionPointersWithContext, then call them in sequence using CallChainOfFunctionPointersWithContext::call().
Used mostly by the interrupt chaining code, but can be used for other purposes.
Example:
CallChainOfFunctionPointersWithContext<void *> chain; void first(void *context) { printf("'first' function.\n"); } void second(void *context) { printf("'second' function.\n"); } class Test { public: void f(void *context) { printf("A::f (class member).\n"); } }; int main() { Test test; chain.add(second); chain.add_front(first); chain.add(&test, &Test::f); chain.call(); }
Definition at line 59 of file CallChainOfFunctionPointersWithContext.h.
Member Typedef Documentation
typedef void(base::* BoolType_t)() const [protected, inherited] |
The bool type is a pointer to method which can be used in boolean context.
Definition at line 35 of file SafeBool.h.
typedef FunctionPointerWithContext<ContextType>* pFunctionPointerWithContext_t |
The type of each callback in the callchain.
Definition at line 64 of file CallChainOfFunctionPointersWithContext.h.
Constructor & Destructor Documentation
Create an empty chain.
Definition at line 70 of file CallChainOfFunctionPointersWithContext.h.
Member Function Documentation
pFunctionPointerWithContext_t add | ( | void(*)(ContextType context) | function ) |
Add a function at the front of the chain.
- Parameters:
-
[in] function A pointer to a void function.
- Returns:
- The function object created for
function
.
Definition at line 86 of file CallChainOfFunctionPointersWithContext.h.
pFunctionPointerWithContext_t add | ( | T * | tptr, |
void(T::*)(ContextType context) | mptr | ||
) |
Add a function at the front of the chain.
- Parameters:
-
[in] tptr Pointer to the object to call the member function on. [in] mptr Pointer to the member function to be called.
- Returns:
- The function object created for
tptr
andmptr
.
Definition at line 101 of file CallChainOfFunctionPointersWithContext.h.
pFunctionPointerWithContext_t add | ( | const FunctionPointerWithContext< ContextType > & | func ) |
Add a function at the front of the chain.
- Parameters:
-
[in] func The FunctionPointerWithContext to add.
- Returns:
- The function object created for
func
.
Definition at line 113 of file CallChainOfFunctionPointersWithContext.h.
void call | ( | ContextType | context ) | const |
Same as call() above, but const.
Definition at line 189 of file CallChainOfFunctionPointersWithContext.h.
void call | ( | ContextType | context ) |
Call all the functions in the chain in sequence.
Definition at line 182 of file CallChainOfFunctionPointersWithContext.h.
void clear | ( | void | ) |
Clear the call chain (remove all functions in the chain).
Definition at line 159 of file CallChainOfFunctionPointersWithContext.h.
bool detach | ( | const FunctionPointerWithContext< ContextType > & | toDetach ) |
Detach a function pointer from a callchain.
- Parameters:
-
[in] toDetach FunctionPointerWithContext to detach from this callchain.
- Returns:
- true if a function pointer has been detached and false otherwise.
- Note:
- It is safe to remove a function pointer while the chain is traversed by call(ContextType).
Definition at line 128 of file CallChainOfFunctionPointersWithContext.h.
bool hasCallbacksAttached | ( | void | ) | const |
Check whether the callchain contains any callbacks.
- Returns:
- true if the callchain is not empty and false otherwise.
Definition at line 175 of file CallChainOfFunctionPointersWithContext.h.
void invalidTag | ( | ) | const [protected, inherited] |
Non implemented call, use to disallow conversion between unrelated types.
operator BoolType_t | ( | ) | const [inherited] |
Bool operator implementation, derived class has to provide bool toBool() const function.
Definition at line 100 of file SafeBool.h.
void operator() | ( | ContextType | context ) | const |
Same as call(), but with function call operator.
void first(bool); void second(bool); CallChainOfFunctionPointerWithContext<bool> foo; foo.attach(first); foo.attach(second); // call the callchain like a function foo(true);
Definition at line 220 of file CallChainOfFunctionPointersWithContext.h.
bool toBool | ( | ) | const |
Bool conversion operation.
- Returns:
- true if the callchain is not empty and false otherwise.
Definition at line 229 of file CallChainOfFunctionPointersWithContext.h.
void trueTag | ( | ) | const [protected, inherited] |
Member function which indicate true value.
Definition at line 45 of file SafeBool.h.
Generated on Wed Jul 13 2022 09:31:10 by
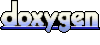