adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
GattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVER_H__ 00018 #define __GATT_SERVER_H__ 00019 00020 #include "Gap.h" 00021 #include "GattService.h" 00022 #include "GattAttribute.h" 00023 #include "GattServerEvents.h" 00024 #include "GattCallbackParamTypes.h" 00025 #include "CallChainOfFunctionPointersWithContext.h" 00026 00027 class GattServer { 00028 public: 00029 /** 00030 * Type for the registered callbacks added to the data sent callchain. 00031 * Refer to GattServer::onDataSent(). 00032 */ 00033 typedef FunctionPointerWithContext<unsigned> DataSentCallback_t; 00034 /** 00035 * Type for the data sent event callchain. Refer to GattServer::onDataSent(). 00036 */ 00037 typedef CallChainOfFunctionPointersWithContext<unsigned> DataSentCallbackChain_t; 00038 00039 /** 00040 * Type for the registered callbacks added to the data written callchain. 00041 * Refer to GattServer::onDataWritten(). 00042 */ 00043 typedef FunctionPointerWithContext<const GattWriteCallbackParams*> DataWrittenCallback_t; 00044 /** 00045 * Type for the data written event callchain. Refer to GattServer::onDataWritten(). 00046 */ 00047 typedef CallChainOfFunctionPointersWithContext<const GattWriteCallbackParams*> DataWrittenCallbackChain_t; 00048 00049 /** 00050 * Type for the registered callbacks added to the data read callchain. 00051 * Refer to GattServer::onDataRead(). 00052 */ 00053 typedef FunctionPointerWithContext<const GattReadCallbackParams*> DataReadCallback_t; 00054 /** 00055 * Type for the data read event callchain. Refer to GattServer::onDataRead(). 00056 */ 00057 typedef CallChainOfFunctionPointersWithContext<const GattReadCallbackParams *> DataReadCallbackChain_t; 00058 00059 /** 00060 * Type for the registered callbacks added to the shutdown callchain. 00061 * Refer to GattServer::onShutdown(). 00062 */ 00063 typedef FunctionPointerWithContext<const GattServer *> GattServerShutdownCallback_t; 00064 /** 00065 * Type for the shutdown event callchain. Refer to GattServer::onShutdown(). 00066 */ 00067 typedef CallChainOfFunctionPointersWithContext<const GattServer *> GattServerShutdownCallbackChain_t; 00068 00069 /** 00070 * Type for the registered callback for various events. Refer to 00071 * GattServer::onUpdatesEnabled(), GattServer::onUpdateDisabled() and 00072 * GattServer::onConfirmationReceived(). 00073 */ 00074 typedef FunctionPointerWithContext<GattAttribute::Handle_t> EventCallback_t; 00075 00076 protected: 00077 /** 00078 * Construct a GattServer instance. 00079 */ 00080 GattServer() : 00081 serviceCount(0), 00082 characteristicCount(0), 00083 dataSentCallChain(), 00084 dataWrittenCallChain(), 00085 dataReadCallChain(), 00086 updatesEnabledCallback(NULL), 00087 updatesDisabledCallback(NULL), 00088 confirmationReceivedCallback(NULL) { 00089 /* empty */ 00090 } 00091 00092 /* 00093 * The following functions are meant to be overridden in the platform-specific sub-class. 00094 */ 00095 public: 00096 00097 /** 00098 * Add a service declaration to the local server ATT table. Also add the 00099 * characteristics contained within. 00100 * 00101 * @param[in] service 00102 * The service to be added. 00103 * 00104 * @return BLE_ERROR_NONE if the service was successfully added. 00105 */ 00106 virtual ble_error_t addService(GattService &service) { 00107 /* Avoid compiler warnings about unused variables. */ 00108 (void)service; 00109 00110 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00111 } 00112 00113 /** 00114 * Read the value of a characteristic from the local GATT server. 00115 * 00116 * @param[in] attributeHandle 00117 * Attribute handle for the value attribute of the characteristic. 00118 * @param[out] buffer 00119 * A buffer to hold the value being read. 00120 * @param[in,out] lengthP 00121 * Length of the buffer being supplied. If the attribute 00122 * value is longer than the size of the supplied buffer, 00123 * this variable will hold upon return the total attribute value length 00124 * (excluding offset). The application may use this 00125 * information to allocate a suitable buffer size. 00126 * 00127 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 00128 */ 00129 virtual ble_error_t read(GattAttribute::Handle_t attributeHandle, uint8_t buffer[], uint16_t *lengthP) { 00130 /* Avoid compiler warnings about unused variables. */ 00131 (void)attributeHandle; 00132 (void)buffer; 00133 (void)lengthP; 00134 00135 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00136 } 00137 00138 /** 00139 * Read the value of a characteristic from the local GATT server. 00140 * 00141 * @param[in] connectionHandle 00142 * Connection handle. 00143 * @param[in] attributeHandle 00144 * Attribute handle for the value attribute of the characteristic. 00145 * @param[out] buffer 00146 * A buffer to hold the value being read. 00147 * @param[in,out] lengthP 00148 * Length of the buffer being supplied. If the attribute 00149 * value is longer than the size of the supplied buffer, 00150 * this variable will hold upon return the total attribute value length 00151 * (excluding offset). The application may use this 00152 * information to allocate a suitable buffer size. 00153 * 00154 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 00155 * 00156 * @note This API is a version of the above, with an additional connection handle 00157 * parameter to allow fetches for connection-specific multivalued 00158 * attributes (such as the CCCDs). 00159 */ 00160 virtual ble_error_t read(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, uint8_t *buffer, uint16_t *lengthP) { 00161 /* Avoid compiler warnings about unused variables. */ 00162 (void)connectionHandle; 00163 (void)attributeHandle; 00164 (void)buffer; 00165 (void)lengthP; 00166 00167 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00168 } 00169 00170 /** 00171 * Update the value of a characteristic on the local GATT server. 00172 * 00173 * @param[in] attributeHandle 00174 * Handle for the value attribute of the characteristic. 00175 * @param[in] value 00176 * A pointer to a buffer holding the new value. 00177 * @param[in] size 00178 * Size of the new value (in bytes). 00179 * @param[in] localOnly 00180 * Should this update be kept on the local 00181 * GATT server regardless of the state of the 00182 * notify/indicate flag in the CCCD for this 00183 * Characteristic? If set to true, no notification 00184 * or indication is generated. 00185 * 00186 * @return BLE_ERROR_NONE if we have successfully set the value of the attribute. 00187 */ 00188 virtual ble_error_t write(GattAttribute::Handle_t attributeHandle, const uint8_t *value, uint16_t size, bool localOnly = false) { 00189 /* Avoid compiler warnings about unused variables. */ 00190 (void)attributeHandle; 00191 (void)value; 00192 (void)size; 00193 (void)localOnly; 00194 00195 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00196 } 00197 00198 /** 00199 * Update the value of a characteristic on the local GATT server. A version 00200 * of the same as the above, with a connection handle parameter to allow updates 00201 * for connection-specific multivalued attributes (such as the CCCDs). 00202 * 00203 * @param[in] connectionHandle 00204 * Connection handle. 00205 * @param[in] attributeHandle 00206 * Handle for the value attribute of the characteristic. 00207 * @param[in] value 00208 * A pointer to a buffer holding the new value. 00209 * @param[in] size 00210 * Size of the new value (in bytes). 00211 * @param[in] localOnly 00212 * Should this update be kept on the local 00213 * GattServer regardless of the state of the 00214 * notify/indicate flag in the CCCD for this 00215 * Characteristic? If set to true, no notification 00216 * or indication is generated. 00217 * 00218 * @return BLE_ERROR_NONE if we have successfully set the value of the attribute. 00219 */ 00220 virtual ble_error_t write(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, const uint8_t *value, uint16_t size, bool localOnly = false) { 00221 /* Avoid compiler warnings about unused variables. */ 00222 (void)connectionHandle; 00223 (void)attributeHandle; 00224 (void)value; 00225 (void)size; 00226 (void)localOnly; 00227 00228 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00229 } 00230 00231 /** 00232 * Determine the updates-enabled status (notification or indication) for the current connection from a characteristic's CCCD. 00233 * 00234 * @param[in] characteristic 00235 * The characteristic. 00236 * @param[out] enabledP 00237 * Upon return, *enabledP is true if updates are enabled, else false. 00238 * 00239 * @return BLE_ERROR_NONE if the connection and handle are found. False otherwise. 00240 */ 00241 virtual ble_error_t areUpdatesEnabled(const GattCharacteristic &characteristic, bool *enabledP) { 00242 /* Avoid compiler warnings about unused variables. */ 00243 (void)characteristic; 00244 (void)enabledP; 00245 00246 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00247 } 00248 00249 /** 00250 * Determine the connection-specific updates-enabled status (notification or indication) from a characteristic's CCCD. 00251 * 00252 * @param[in] connectionHandle 00253 * The connection handle. 00254 * @param[in] characteristic 00255 * The characteristic. 00256 * @param[out] enabledP 00257 * Upon return, *enabledP is true if updates are enabled, else false. 00258 * 00259 * @return BLE_ERROR_NONE if the connection and handle are found. False otherwise. 00260 */ 00261 virtual ble_error_t areUpdatesEnabled(Gap::Handle_t connectionHandle, const GattCharacteristic &characteristic, bool *enabledP) { 00262 /* Avoid compiler warnings about unused variables. */ 00263 (void)connectionHandle; 00264 (void)characteristic; 00265 (void)enabledP; 00266 00267 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00268 } 00269 00270 /** 00271 * A virtual function to allow underlying stacks to indicate if they support 00272 * onDataRead(). It should be overridden to return true as applicable. 00273 * 00274 * @return true if onDataRead is supported, false otherwise. 00275 */ 00276 virtual bool isOnDataReadAvailable() const { 00277 return false; /* Requesting action from porters: override this API if this capability is supported. */ 00278 } 00279 00280 /* 00281 * APIs with non-virtual implementations. 00282 */ 00283 public: 00284 /** 00285 * Add a callback for the GATT event DATA_SENT (which is triggered when 00286 * updates are sent out by GATT in the form of notifications). 00287 * 00288 * @param[in] callback 00289 * Event handler being registered. 00290 * 00291 * @note It is possible to chain together multiple onDataSent callbacks 00292 * (potentially from different modules of an application) to receive updates 00293 * to characteristics. 00294 * 00295 * @note It is also possible to set up a callback into a member function of 00296 * some object. 00297 */ 00298 void onDataSent(const DataSentCallback_t& callback) { 00299 dataSentCallChain.add(callback); 00300 } 00301 00302 /** 00303 * Same as GattServer::onDataSent(), but allows the possibility to add an object 00304 * reference and member function as handler for DATA_SENT event 00305 * callbacks. 00306 * 00307 * @param[in] objPtr 00308 * Pointer to the object of a class defining the member callback 00309 * function (@p memberPtr). 00310 * @param[in] memberPtr 00311 * The member callback (within the context of an object) to be 00312 * invoked. 00313 */ 00314 template <typename T> 00315 void onDataSent(T *objPtr, void (T::*memberPtr)(unsigned count)) { 00316 dataSentCallChain.add(objPtr, memberPtr); 00317 } 00318 00319 /** 00320 * @brief Provide access to the callchain of DATA_SENT event callbacks. 00321 * 00322 * @return A reference to the DATA_SENT event callback chain. 00323 */ 00324 DataSentCallbackChain_t& onDataSent() { 00325 return dataSentCallChain; 00326 } 00327 00328 /** 00329 * Set up a callback for when an attribute has its value updated by or at the 00330 * connected peer. For a peripheral, this callback is triggered when the local 00331 * GATT server has an attribute updated by a write command from the peer. 00332 * For a central, this callback is triggered when a response is received for 00333 * a write request. 00334 * 00335 * @param[in] callback 00336 * Event handler being registered. 00337 * 00338 * @note It is possible to chain together multiple onDataWritten callbacks 00339 * (potentially from different modules of an application) to receive updates 00340 * to characteristics. Many services, such as DFU and UART, add their own 00341 * onDataWritten callbacks behind the scenes to trap interesting events. 00342 * 00343 * @note It is also possible to set up a callback into a member function of 00344 * some object. 00345 * 00346 * @note It is possible to unregister a callback using onDataWritten().detach(callback) 00347 */ 00348 void onDataWritten(const DataWrittenCallback_t& callback) { 00349 dataWrittenCallChain.add(callback); 00350 } 00351 00352 /** 00353 * Same as GattServer::onDataWritten(), but allows the possibility to add an object 00354 * reference and member function as handler for data written event 00355 * callbacks. 00356 * 00357 * @param[in] objPtr 00358 * Pointer to the object of a class defining the member callback 00359 * function (@p memberPtr). 00360 * @param[in] memberPtr 00361 * The member callback (within the context of an object) to be 00362 * invoked. 00363 */ 00364 template <typename T> 00365 void onDataWritten(T *objPtr, void (T::*memberPtr)(const GattWriteCallbackParams *context)) { 00366 dataWrittenCallChain.add(objPtr, memberPtr); 00367 } 00368 00369 /** 00370 * @brief Provide access to the callchain of data written event callbacks. 00371 * 00372 * @return A reference to the data written event callbacks chain. 00373 * 00374 * @note It is possible to register callbacks using onDataWritten().add(callback). 00375 * 00376 * @note It is possible to unregister callbacks using onDataWritten().detach(callback). 00377 */ 00378 DataWrittenCallbackChain_t& onDataWritten() { 00379 return dataWrittenCallChain; 00380 } 00381 00382 /** 00383 * Setup a callback to be invoked on the peripheral when an attribute is 00384 * being read by a remote client. 00385 * 00386 * @param[in] callback 00387 * Event handler being registered. 00388 * 00389 * @return BLE_ERROR_NOT_IMPLEMENTED if this functionality isn't available; 00390 * else BLE_ERROR_NONE. 00391 * 00392 * @note This functionality may not be available on all underlying stacks. 00393 * You could use GattCharacteristic::setReadAuthorizationCallback() as an 00394 * alternative. Refer to isOnDataReadAvailable(). 00395 * 00396 * @note It is possible to chain together multiple onDataRead callbacks 00397 * (potentially from different modules of an application) to receive updates 00398 * to characteristics. Services may add their own onDataRead callbacks 00399 * behind the scenes to trap interesting events. 00400 * 00401 * @note It is also possible to set up a callback into a member function of 00402 * some object. 00403 * 00404 * @note It is possible to unregister a callback using onDataRead().detach(callback). 00405 */ 00406 ble_error_t onDataRead(const DataReadCallback_t& callback) { 00407 if (!isOnDataReadAvailable()) { 00408 return BLE_ERROR_NOT_IMPLEMENTED; 00409 } 00410 00411 dataReadCallChain.add(callback); 00412 return BLE_ERROR_NONE; 00413 } 00414 00415 /** 00416 * Same as GattServer::onDataRead(), but allows the possibility to add an object 00417 * reference and member function as handler for data read event 00418 * callbacks. 00419 * 00420 * @param[in] objPtr 00421 * Pointer to the object of a class defining the member callback 00422 * function (@p memberPtr). 00423 * @param[in] memberPtr 00424 * The member callback (within the context of an object) to be 00425 * invoked. 00426 */ 00427 template <typename T> 00428 ble_error_t onDataRead(T *objPtr, void (T::*memberPtr)(const GattReadCallbackParams *context)) { 00429 if (!isOnDataReadAvailable()) { 00430 return BLE_ERROR_NOT_IMPLEMENTED; 00431 } 00432 00433 dataReadCallChain.add(objPtr, memberPtr); 00434 return BLE_ERROR_NONE; 00435 } 00436 00437 /** 00438 * @brief Provide access to the callchain of data read event callbacks. 00439 * 00440 * @return A reference to the data read event callbacks chain. 00441 * 00442 * @note It is possible to register callbacks using onDataRead().add(callback). 00443 * 00444 * @note It is possible to unregister callbacks using onDataRead().detach(callback). 00445 */ 00446 DataReadCallbackChain_t& onDataRead() { 00447 return dataReadCallChain; 00448 } 00449 00450 /** 00451 * Setup a callback to be invoked to notify the user application that the 00452 * GattServer instance is about to shutdown (possibly as a result of a call 00453 * to BLE::shutdown()). 00454 * 00455 * @param[in] callback 00456 * Event handler being registered. 00457 * 00458 * @note It is possible to chain together multiple onShutdown callbacks 00459 * (potentially from different modules of an application) to be notified 00460 * before the GattServer is shutdown. 00461 * 00462 * @note It is also possible to set up a callback into a member function of 00463 * some object. 00464 * 00465 * @note It is possible to unregister a callback using onShutdown().detach(callback) 00466 */ 00467 void onShutdown(const GattServerShutdownCallback_t& callback) { 00468 shutdownCallChain.add(callback); 00469 } 00470 00471 /** 00472 * Same as GattServer::onShutdown(), but allows the possibility to add an object 00473 * reference and member function as handler for shutdown event 00474 * callbacks. 00475 * 00476 * @param[in] objPtr 00477 * Pointer to the object of a class defining the member callback 00478 * function (@p memberPtr). 00479 * @param[in] memberPtr 00480 * The member callback (within the context of an object) to be 00481 * invoked. 00482 */ 00483 template <typename T> 00484 void onShutdown(T *objPtr, void (T::*memberPtr)(const GattServer *)) { 00485 shutdownCallChain.add(objPtr, memberPtr); 00486 } 00487 00488 /** 00489 * @brief Provide access to the callchain of shutdown event callbacks. 00490 * 00491 * @return A reference to the shutdown event callbacks chain. 00492 * 00493 * @note It is possible to register callbacks using onShutdown().add(callback). 00494 * 00495 * @note It is possible to unregister callbacks using onShutdown().detach(callback). 00496 */ 00497 GattServerShutdownCallbackChain_t& onShutdown() { 00498 return shutdownCallChain; 00499 } 00500 00501 /** 00502 * Set up a callback for when notifications or indications are enabled for a 00503 * characteristic on the local GATT server. 00504 * 00505 * @param[in] callback 00506 * Event handler being registered. 00507 */ 00508 void onUpdatesEnabled(EventCallback_t callback) { 00509 updatesEnabledCallback = callback; 00510 } 00511 00512 /** 00513 * Set up a callback for when notifications or indications are disabled for a 00514 * characteristic on the local GATT server. 00515 * 00516 * @param[in] callback 00517 * Event handler being registered. 00518 */ 00519 void onUpdatesDisabled(EventCallback_t callback) { 00520 updatesDisabledCallback = callback; 00521 } 00522 00523 /** 00524 * Set up a callback for when the GATT server receives a response for an 00525 * indication event sent previously. 00526 * 00527 * @param[in] callback 00528 * Event handler being registered. 00529 */ 00530 void onConfirmationReceived(EventCallback_t callback) { 00531 confirmationReceivedCallback = callback; 00532 } 00533 00534 /* Entry points for the underlying stack to report events back to the user. */ 00535 protected: 00536 /** 00537 * Helper function that notifies all registered handlers of an occurrence 00538 * of a data written event. This function is meant to be called from the 00539 * BLE stack specific implementation when a data written event occurs. 00540 * 00541 * @param[in] params 00542 * The data written parameters passed to the registered 00543 * handlers. 00544 */ 00545 void handleDataWrittenEvent(const GattWriteCallbackParams *params) { 00546 dataWrittenCallChain.call(params); 00547 } 00548 00549 /** 00550 * Helper function that notifies all registered handlers of an occurrence 00551 * of a data read event. This function is meant to be called from the 00552 * BLE stack specific implementation when a data read event occurs. 00553 * 00554 * @param[in] params 00555 * The data read parameters passed to the registered 00556 * handlers. 00557 */ 00558 void handleDataReadEvent(const GattReadCallbackParams *params) { 00559 dataReadCallChain.call(params); 00560 } 00561 00562 /** 00563 * Helper function that notifies the registered handler of an occurrence 00564 * of updates enabled, updates disabled and confirmation received events. 00565 * This function is meant to be called from the BLE stack specific 00566 * implementation when any of these events occurs. 00567 * 00568 * @param[in] type 00569 * The type of event that occurred. 00570 * @param[in] attributeHandle 00571 * The handle of the attribute that was modified. 00572 */ 00573 void handleEvent(GattServerEvents::gattEvent_e type, GattAttribute::Handle_t attributeHandle) { 00574 switch (type) { 00575 case GattServerEvents::GATT_EVENT_UPDATES_ENABLED: 00576 if (updatesEnabledCallback) { 00577 updatesEnabledCallback(attributeHandle); 00578 } 00579 break; 00580 case GattServerEvents::GATT_EVENT_UPDATES_DISABLED: 00581 if (updatesDisabledCallback) { 00582 updatesDisabledCallback(attributeHandle); 00583 } 00584 break; 00585 case GattServerEvents::GATT_EVENT_CONFIRMATION_RECEIVED: 00586 if (confirmationReceivedCallback) { 00587 confirmationReceivedCallback(attributeHandle); 00588 } 00589 break; 00590 default: 00591 break; 00592 } 00593 } 00594 00595 /** 00596 * Helper function that notifies all registered handlers of an occurrence 00597 * of a data sent event. This function is meant to be called from the 00598 * BLE stack specific implementation when a data sent event occurs. 00599 * 00600 * @param[in] count 00601 * Number of packets sent. 00602 */ 00603 void handleDataSentEvent(unsigned count) { 00604 dataSentCallChain.call(count); 00605 } 00606 00607 public: 00608 /** 00609 * Notify all registered onShutdown callbacks that the GattServer is 00610 * about to be shutdown and clear all GattServer state of the 00611 * associated object. 00612 * 00613 * This function is meant to be overridden in the platform-specific 00614 * sub-class. Nevertheless, the sub-class is only expected to reset its 00615 * state and not the data held in GattServer members. This shall be achieved 00616 * by a call to GattServer::reset() from the sub-class' reset() 00617 * implementation. 00618 * 00619 * @return BLE_ERROR_NONE on success. 00620 */ 00621 virtual ble_error_t reset(void) { 00622 /* Notify that the instance is about to shutdown */ 00623 shutdownCallChain.call(this); 00624 shutdownCallChain.clear(); 00625 00626 serviceCount = 0; 00627 characteristicCount = 0; 00628 00629 dataSentCallChain.clear(); 00630 dataWrittenCallChain.clear(); 00631 dataReadCallChain.clear(); 00632 updatesEnabledCallback = NULL; 00633 updatesDisabledCallback = NULL; 00634 confirmationReceivedCallback = NULL; 00635 00636 return BLE_ERROR_NONE; 00637 } 00638 00639 protected: 00640 /** 00641 * The total number of services added to the ATT table. 00642 */ 00643 uint8_t serviceCount; 00644 /** 00645 * The total number of characteristics added to the ATT table. 00646 */ 00647 uint8_t characteristicCount; 00648 00649 private: 00650 /** 00651 * Callchain containing all registered callback handlers for data sent 00652 * events. 00653 */ 00654 DataSentCallbackChain_t dataSentCallChain; 00655 /** 00656 * Callchain containing all registered callback handlers for data written 00657 * events. 00658 */ 00659 DataWrittenCallbackChain_t dataWrittenCallChain; 00660 /** 00661 * Callchain containing all registered callback handlers for data read 00662 * events. 00663 */ 00664 DataReadCallbackChain_t dataReadCallChain; 00665 /** 00666 * Callchain containing all registered callback handlers for shutdown 00667 * events. 00668 */ 00669 GattServerShutdownCallbackChain_t shutdownCallChain; 00670 /** 00671 * The registered callback handler for updates enabled events. 00672 */ 00673 EventCallback_t updatesEnabledCallback; 00674 /** 00675 * The registered callback handler for updates disabled events. 00676 */ 00677 EventCallback_t updatesDisabledCallback; 00678 /** 00679 * The registered callback handler for confirmation received events. 00680 */ 00681 EventCallback_t confirmationReceivedCallback; 00682 00683 private: 00684 /* Disallow copy and assignment. */ 00685 GattServer(const GattServer &); 00686 GattServer& operator=(const GattServer &); 00687 }; 00688 00689 #endif /* ifndef __GATT_SERVER_H__ */
Generated on Wed Jul 13 2022 09:31:10 by
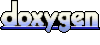