Use this interface to connect to and interact with the WNC M14A2A LTE Cellular Data Module which is provided by Wistron NeWeb Corporation (WNC) when using ARMmbed v5. The interface provides a Networking interface that can be used with the AT&T Cellular IoT Starter Kit that is sold by Avnet (http://cloudconnectkits.org/product/att-cellular-iot-starter-kit).
Dependencies: WncControllerK64F
Dependents: easy-connect-wnc easy-connect easy-connect111
WNC14A2AInterface Class Reference
WNC14A2AInterface class Implementation of the NetworkInterface for WNC14A2A. More...
#include <WNC14A2AInterface.h>
Public Member Functions | |
WNC14A2AInterface (WNCDebug *_dbgUart=NULL) | |
WNC14A2AInterface Constructors... | |
virtual nsapi_error_t | set_credentials (const char *apn=0, const char *username=0, const char *password=0) |
Set the cellular network APN and credentials. | |
virtual nsapi_error_t | connect (const char *apn, const char *username=0, const char *password=0) |
Start the interface. | |
virtual nsapi_error_t | connect () |
Start the interface. | |
virtual nsapi_error_t | disconnect () |
Stop the interface. | |
virtual const char * | get_ip_address () |
Get the internally stored IP address. | |
const char * | get_my_ip_address () |
Get the network assigned IP address. | |
virtual const char * | get_mac_address () |
Get the internally stored MAC address. | |
void | sms_attach (void(*callback)(IOTSMS *)) |
Attach a function to be called when a text is recevieds. | |
void | sms_listen (uint16_t=1000) |
start listening for incomming SMS messages | |
Protected Member Functions | |
virtual nsapi_error_t | gethostbyname (const char *name, SocketAddress *address, nsapi_version_t version) |
Get Host IP by name. | |
virtual NetworkStack * | get_stack () |
Provide access to the NetworkStack object. | |
virtual int | socket_open (void **handle, nsapi_protocol_t proto) |
Open a socket. | |
virtual int | socket_close (void *handle) |
Close the socket. | |
virtual int | socket_bind (void *handle, const SocketAddress &address) |
Bind a server socket to a specific port.FROM NetworkStack. | |
virtual int | socket_listen (void *handle, int backlog) |
Start listening for incoming connections.FROM NetworkStack. | |
virtual int | socket_connect (void *handle, const SocketAddress &address) |
Connects this TCP socket to the server.FROM NetworkStack. | |
virtual int | socket_accept (nsapi_socket_t server, nsapi_socket_t *handle, SocketAddress *address=0) |
Accept a new connection.FROM NetworkStack. | |
virtual int | socket_send (void *handle, const void *data, unsigned size) |
Send data to the remote host.FROM NetworkStack. | |
virtual int | socket_recv (void *handle, void *data, unsigned size) |
Receive data from the remote host.FROM NetworkStack. | |
virtual int | socket_sendto (void *handle, const SocketAddress &address, const void *data, unsigned size) |
Send a packet to a remote endpoint.FROM NetworkStack. | |
virtual int | socket_recvfrom (void *handle, SocketAddress *address, void *buffer, unsigned size) |
Receive a packet from a remote endpoint.FROM NetworkStack. | |
virtual void | socket_attach (void *handle, void(*callback)(void *), void *data) |
Register a callback on state change of the socket.FROM NetworkStack. | |
uint16_t | wnc14a2a_chk_error (void) |
check for errors that may have occured |
Detailed Description
WNC14A2AInterface class Implementation of the NetworkInterface for WNC14A2A.
Definition at line 101 of file WNC14A2AInterface.h.
Constructor & Destructor Documentation
WNC14A2AInterface | ( | WNCDebug * | _dbgUart = NULL ) |
WNC14A2AInterface Constructors...
- Parameters:
-
can include an APN string and/or a debug uart
Definition at line 59 of file WNC14A2AInterface.cpp.
Member Function Documentation
nsapi_error_t connect | ( | const char * | apn, |
const char * | username = 0 , |
||
const char * | password = 0 |
||
) | [virtual] |
Start the interface.
- Parameters:
-
apn Optional name of the network to connect to username Optional username for your APN password Optional password for your APN
- Returns:
- 0 on success, negative error code on failure
Definition at line 129 of file WNC14A2AInterface.cpp.
nsapi_error_t connect | ( | ) | [virtual] |
Start the interface.
Attempts to connect to a cellular network based on supplied credentials
- Returns:
- 0 on success, negative error code on failure
Definition at line 111 of file WNC14A2AInterface.cpp.
nsapi_error_t disconnect | ( | ) | [virtual] |
Stop the interface.
- Returns:
- 0 on success, negative error code on failure
Definition at line 518 of file WNC14A2AInterface.cpp.
const char * get_ip_address | ( | ) | [virtual] |
Get the internally stored IP address.
From NetworkStack Class
- Returns:
- IP address of the interface or null if not yet connected
Definition at line 170 of file WNC14A2AInterface.cpp.
const char * get_mac_address | ( | ) | [virtual] |
Get the internally stored MAC address.
From CellularInterface Class
- Returns:
- MAC address of the interface
Definition at line 491 of file WNC14A2AInterface.cpp.
const char* get_my_ip_address | ( | ) |
Get the network assigned IP address.
- Returns:
- IP address of the interface or null if not yet connected
NetworkStack * get_stack | ( | ) | [protected, virtual] |
Provide access to the NetworkStack object.
- Returns:
- The underlying NetworkStack object
Definition at line 509 of file WNC14A2AInterface.cpp.
nsapi_error_t gethostbyname | ( | const char * | name, |
SocketAddress * | address, | ||
nsapi_version_t | version | ||
) | [protected, virtual] |
nsapi_error_t set_credentials | ( | const char * | apn = 0 , |
const char * | username = 0 , |
||
const char * | password = 0 |
||
) | [virtual] |
Set the cellular network APN and credentials.
- Parameters:
-
apn Optional name of the network to connect to user Optional username for the APN pass Optional password fot the APN
- Returns:
- 0 on success, negative error code on failure
Definition at line 536 of file WNC14A2AInterface.cpp.
void sms_attach | ( | void(*)(IOTSMS *) | callback ) |
Attach a function to be called when a text is recevieds.
- Parameters:
-
callback function pointer to a callback that will accept the message contents when a text is received.
Definition at line 715 of file WNC14A2AInterface.cpp.
void sms_listen | ( | uint16_t | pp = 1000 ) |
start listening for incomming SMS messages
- Parameters:
-
time in msec to check
Definition at line 743 of file WNC14A2AInterface.cpp.
int socket_accept | ( | nsapi_socket_t | server, |
nsapi_socket_t * | handle, | ||
SocketAddress * | address = 0 |
||
) | [protected, virtual] |
Accept a new connection.FROM NetworkStack.
- Parameters:
-
handle Handle in which to store new socket server Socket handle to server to accept from
- Returns:
- 0 on success, negative on failure
- Note:
- This call is not-blocking, if this call would block, must immediately return NSAPI_ERROR_WOULD_WAIT
Definition at line 869 of file WNC14A2AInterface.cpp.
void socket_attach | ( | void * | handle, |
void(*)(void *) | callback, | ||
void * | data | ||
) | [protected, virtual] |
Register a callback on state change of the socket.FROM NetworkStack.
- Parameters:
-
handle Socket handle callback Function to call on state change data Argument to pass to callback
- Note:
- Callback may be called in an interrupt context.
Definition at line 558 of file WNC14A2AInterface.cpp.
int socket_bind | ( | void * | handle, |
const SocketAddress & | address | ||
) | [protected, virtual] |
Bind a server socket to a specific port.FROM NetworkStack.
- Parameters:
-
handle Socket handle address Local address to listen for incoming connections on
- Returns:
- 0 on success, negative on failure.
Definition at line 876 of file WNC14A2AInterface.cpp.
int socket_close | ( | void * | handle ) | [protected, virtual] |
Close the socket.
FROM NetworkStack
- Parameters:
-
handle Socket handle
- Returns:
- 0 on success, negative on failure
- Note:
- On failure, any memory associated with the socket must still be cleaned up
Definition at line 456 of file WNC14A2AInterface.cpp.
int socket_connect | ( | void * | handle, |
const SocketAddress & | address | ||
) | [protected, virtual] |
Connects this TCP socket to the server.FROM NetworkStack.
- Parameters:
-
handle Socket handle address SocketAddress to connect to
- Returns:
- 0 on success, negative on failure
Definition at line 270 of file WNC14A2AInterface.cpp.
int socket_listen | ( | void * | handle, |
int | backlog | ||
) | [protected, virtual] |
Start listening for incoming connections.FROM NetworkStack.
- Parameters:
-
handle Socket handle backlog Number of pending connections that can be queued up at any one time [Default: 1]
- Returns:
- 0 on success, negative on failure
Definition at line 884 of file WNC14A2AInterface.cpp.
int socket_open | ( | void ** | handle, |
nsapi_protocol_t | proto | ||
) | [protected, virtual] |
Open a socket.
FROM NetworkStack
- Parameters:
-
handle Handle in which to store new socket proto Type of socket to open, NSAPI_TCP or NSAPI_UDP
- Returns:
- 0 on success, negative on failure
Definition at line 228 of file WNC14A2AInterface.cpp.
int socket_recv | ( | void * | handle, |
void * | data, | ||
unsigned | size | ||
) | [protected, virtual] |
Receive data from the remote host.FROM NetworkStack.
- Parameters:
-
handle Socket handle data The buffer in which to store the data received from the host size The maximum length of the buffer
- Returns:
- Number of received bytes on success, negative on failure
- Note:
- This call is not-blocking, if this call would block, must immediately return NSAPI_ERROR_WOULD_WAIT
Definition at line 413 of file WNC14A2AInterface.cpp.
int socket_recvfrom | ( | void * | handle, |
SocketAddress * | address, | ||
void * | buffer, | ||
unsigned | size | ||
) | [protected, virtual] |
Receive a packet from a remote endpoint.FROM NetworkStack.
- Parameters:
-
handle Socket handle address Destination for the remote SocketAddress or null buffer The buffer for storing the incoming packet data If a packet is too long to fit in the supplied buffer, excess bytes are discarded size The length of the buffer
- Returns:
- the number of received bytes on success, negative on failure
- Note:
- This call is not-blocking, if this call would block, must immediately return NSAPI_ERROR_WOULD_WAIT
Definition at line 660 of file WNC14A2AInterface.cpp.
int socket_send | ( | void * | handle, |
const void * | data, | ||
unsigned | size | ||
) | [protected, virtual] |
Send data to the remote host.FROM NetworkStack.
- Parameters:
-
handle Socket handle data The buffer to send to the host size The length of the buffer to send
- Returns:
- Number of written bytes on success, negative on failure
- Note:
- This call is not-blocking, if this call would block, must immediately return NSAPI_ERROR_WOULD_WAIT
Definition at line 375 of file WNC14A2AInterface.cpp.
int socket_sendto | ( | void * | handle, |
const SocketAddress & | address, | ||
const void * | data, | ||
unsigned | size | ||
) | [protected, virtual] |
Send a packet to a remote endpoint.FROM NetworkStack.
- Parameters:
-
handle Socket handle address The remote SocketAddress data The packet to be sent size The length of the packet to be sent
- Returns:
- the number of written bytes on success, negative on failure
- Note:
- This call is not-blocking, if this call would block, must immediately return NSAPI_ERROR_WOULD_WAIT
Definition at line 643 of file WNC14A2AInterface.cpp.
uint16_t wnc14a2a_chk_error | ( | void | ) | [protected] |
check for errors that may have occured
- Parameters:
-
none.
- Note:
- this function can be called after any WNC14A2A class operation to determine error specifics if desired.
Definition at line 300 of file WNC14A2AInterface.h.
Generated on Wed Jul 13 2022 08:07:17 by
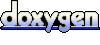