Hi Raharja,
Just to clarify, the problem is not that the mbed is slow; it will certainly beat a AVR 16MHz hands down; the problem is in the program logic. The program has two interrupts, and it is simply that when your interrupt that runs for a long time is active (Ticker), it stops your timing critical interrupt from firing (InterruptIn), as they are at the same priority level.
A solution that we'll fold in to the libraries is to run the timers at a lower priority level, and also expose the priorities so they are configurable by the user, but in the mean time here is an example to show how you can solve the problem yourself. Basically, just set the timer interrupts to be at a lower priority, and all should work fine! The timers all use TIMER3, so we can call the NVIC_SetPriority function to change it's priority.
Here is an example program showing the behaviour, both before and after changing the priority.
// Example running the mbed Tickers at a lower priority
#include "mbed.h"
volatile int counter = 0;
void timing_critical() {
counter++;
}
void long_event() {
wait_ms(50);
}
PwmOut out(p25);
InterruptIn in(p26);
Ticker tick;
int main() {
out.period_ms(10);
out.pulsewidth_ms(5);
in.rise(&timing_critical);
printf("1) InterruptIn only...\n");
for(int i=0; i<5; i++) {
counter = 0;
wait(1);
printf("counts/sec = %d\n", counter);
}
tick.attach(&long_event, 0.1);
printf("2) InterruptIn plus long running occasional ticker event...\n");
for(int i=0; i<5; i++) {
counter = 0;
wait(1);
printf("count/sec = %d\n", counter);
}
printf("3) InterruptIn plus long running occasional ticker event at lower priority...\n");
NVIC_SetPriority(TIMER3_IRQn, 255); // set mbed tickers to lower priority than other things
for(int i=0; i<5; i++) {
counter = 0;
wait(1);
printf("counter = %d\n", counter);
}
}
You can see the results here:
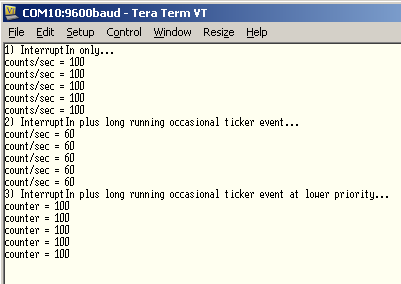
Importantly, the problem you are seeing has very little to do with the speed of the processor, and speed is not the source of your issue. We'll fold this in to the libraries as a fix so you get this result by default for the common case.
Hope this helps clear up the problem you were seeing, and shows it is not about speed, but simply what we are telling the microcontroller to do!
Simon
Dear All,
I am using mbed NXP LPC1768, why it looks so slow?
I use it to read low-cost IMU @100Hz and 115200bps, via a serial port (p28, p27), using 'attach' interrupt function. A PPM RC receiver connected to p11 set as 'InteruptIn' and I use timer to capture the 5 0.7-1.5mS signals with 16mS of period. 4 I2C devices driven by an i2C bus (p9, p10) by 100Hz update rate. I send some data trough RF Modem via a serial (p13, p14) @36Hz and 115200bps.
When I try the tasks one-by-one, it is okay.
But when I integrate the whole system: the IMU data can not be acquired perfectly, the PPM signals can not be captured correctly and so many error data unit in data sending. When I increase over 36Hz in 115200bps, it is more terrible.
In some project file I meet error "Expected a member name (E133)" when I call the timer start() function. But it not happen when make another new project file and I just copy paste the whole code from the old project file.
Please explain to me why? I love the way to use this board but these unexpected problems make me stuck to continue my prototyping project.
Thank you,
/rhj