
Stand-alone AVR(328P) writer
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // AVR(328P) stand-alone writer. 00002 // Written for Make: Tokyo meeting 06 00003 // by @ytsuboi 00004 00005 #include "mbed.h" 00006 // TextLCD could be found at http://mbed.org/users/simon/libraries/TextLCD/livod0 00007 #include "TextLCD.h" 00008 00009 LocalFileSystem local("local"); 00010 TextLCD lcd(p24, p26, p27, p28, p29, p30); 00011 SPI avrspi(p11, p12, p13); // mosi, miso, sck 00012 DigitalOut avrrst(p14); 00013 DigitalIn enable(p10); 00014 00015 int main(void) { 00016 char str[100]; 00017 00018 lcd.cls(); 00019 // Start screen 00020 lcd.locate(0, 0); 00021 lcd.printf("mbed Stand-Alone"); 00022 lcd.locate(5, 1); 00023 lcd.printf("AVR writer"); 00024 wait(3); 00025 00026 lcd.locate(0, 0); 00027 lcd.printf("put your AVR and"); 00028 lcd.locate(0, 1); 00029 lcd.printf("push to continue"); 00030 00031 while(1) { 00032 if(!enable) { 00033 break; 00034 } 00035 wait(0.25); 00036 } 00037 00038 avrrst = 1; 00039 wait_ms(27); 00040 avrrst = 0; 00041 wait_ms(27); 00042 00043 // Setup the spi for 8 bit data, high steady state clock, 00044 // second edge capture, with a 100KHz clock rate 00045 00046 avrspi.format(8,0); 00047 avrspi.frequency(100000); 00048 00049 wait_ms(25); 00050 00051 // enter program mode 00052 avrspi.write(0xAC); 00053 avrspi.write(0x53); 00054 int response = avrspi.write(0x00); 00055 avrspi.write(0x00); 00056 00057 lcd.cls(); 00058 lcd.locate(0, 0); 00059 lcd.printf("Enter Prog mode:"); 00060 lcd.locate(9, 1); 00061 if (response == 0x53) { 00062 lcd.printf("Success"); 00063 } else { 00064 lcd.printf("Failed"); 00065 return -1; 00066 } 00067 00068 wait(2); 00069 00070 // Check Device Sig. 00071 //Vendor Code 00072 avrspi.write(0x30); 00073 avrspi.write(0x00); 00074 avrspi.write(0x00); 00075 int sig0 = avrspi.write(0x00); 00076 //Product Family Code and Flash Size 00077 avrspi.write(0x30); 00078 avrspi.write(0x00); 00079 avrspi.write(0x01); 00080 int sig1 = avrspi.write(0x00); 00081 //Part Number 00082 avrspi.write(0x30); 00083 avrspi.write(0x00); 00084 avrspi.write(0x02); 00085 int sig2 = avrspi.write(0x00); 00086 00087 lcd.cls(); 00088 lcd.locate(0, 0); 00089 sprintf(str, "Device: %02X %02X %02X", sig0, sig1, sig2); 00090 lcd.printf(str); 00091 // lcd.printf("Device:"); 00092 lcd.locate(0, 1); 00093 if (sig0==0x1E && sig1==0x95 && sig2==0x0F) { 00094 lcd.printf("ATmega 328P"); 00095 } else if (sig0==0x1E) { 00096 lcd.printf("Unsupport Atmel"); 00097 return -1; 00098 } else if (sig0==0x00 || sig1==0x01 || sig2==0x02) { 00099 lcd.printf("LOCKED!!"); 00100 return -1; 00101 } else if (sig0==0xFF || sig1==0xFF || sig2||0xFF) { 00102 lcd.printf("MISSING!!"); 00103 return -1; 00104 } else { 00105 lcd.printf("UNKNOWN!!"); 00106 return -1; 00107 } 00108 00109 wait(2); 00110 00111 // Erase Flash 00112 lcd.cls(); 00113 lcd.locate(0, 0); 00114 lcd.printf("Erasing Flash..."); 00115 avrspi.write(0xAC); 00116 avrspi.write(0x80); 00117 avrspi.write(0x00); 00118 avrspi.write(0x00); 00119 wait_ms(9); 00120 //poll 00121 do { 00122 avrspi.write(0xF0); 00123 avrspi.write(0x00); 00124 avrspi.write(0x00); 00125 response = avrspi.write(0x00); 00126 } while ((response & 0x01) != 0); 00127 //end poll 00128 lcd.locate(10, 1); 00129 lcd.printf("DONE"); 00130 00131 wait(2); 00132 00133 // Open binary file 00134 lcd.cls(); 00135 lcd.locate(0, 0); 00136 lcd.printf("Opening file..."); 00137 FILE *fp = fopen("/local/AVRCODE.bin", "rb"); 00138 00139 if (fp == NULL) { 00140 lcd.locate(0, 1); 00141 lcd.printf("Failed to open!!"); 00142 return -1; 00143 } else { 00144 lcd.locate(0, 1); 00145 lcd.printf("Opened!!"); 00146 wait(2); 00147 00148 lcd.cls(); 00149 lcd.locate(0, 0); 00150 lcd.printf("Writing binaries"); 00151 // reset AVR 00152 // avrrst = 1; 00153 // wait_ms(27); 00154 // avrrst = 0; 00155 // wait_ms(27); 00156 00157 int pageOffset = 0; 00158 int pageNum = 0; 00159 int n = 0; 00160 int HighLow = 0; 00161 00162 // do programing 00163 while ((n = getc(fp)) != EOF) { 00164 //write loaded page to flash 00165 //Page size of 328P is 64word/page 00166 if (pageOffset == 64) { 00167 avrspi.write(0x4C); 00168 avrspi.write((pageNum >> 2) & 0x3F); 00169 avrspi.write((pageNum & 0x03) << 6); 00170 avrspi.write(0x00); 00171 wait_ms(5); 00172 //poll 00173 do { 00174 avrspi.write(0xF0); 00175 avrspi.write(0x00); 00176 avrspi.write(0x00); 00177 response = avrspi.write(0x00); 00178 } while ((response & 0x01) != 0); 00179 //end poll 00180 pageNum++; 00181 // Total page of 328P is 256page 00182 if (pageNum > 256) { 00183 break; 00184 } 00185 00186 pageOffset = 0; 00187 } 00188 00189 // load low byte 00190 if (HighLow == 0) { 00191 avrspi.write(0x40); 00192 avrspi.write(0x00); 00193 avrspi.write(pageOffset & 0x3F); 00194 avrspi.write(n); 00195 00196 //poll 00197 do { 00198 avrspi.write(0xF0); 00199 avrspi.write(0x00); 00200 avrspi.write(0x00); 00201 response = avrspi.write(0x00); 00202 } while ((response & 0x01) != 0); 00203 //end poll 00204 00205 HighLow = 1; 00206 } 00207 // load high byte 00208 else { 00209 avrspi.write(0x48); 00210 avrspi.write(0x00); 00211 avrspi.write(pageOffset & 0x3F); 00212 avrspi.write(n); 00213 00214 //poll 00215 do { 00216 avrspi.write(0xF0); 00217 avrspi.write(0x00); 00218 avrspi.write(0x00); 00219 response = avrspi.write(0x00); 00220 } while ((response & 0x01) != 0); 00221 //end poll 00222 00223 HighLow = 0; 00224 pageOffset++; 00225 } 00226 } 00227 00228 //close binary file 00229 fclose(fp); 00230 00231 lcd.locate(0, 1); 00232 lcd.printf("DONE!!"); 00233 wait(2); 00234 } 00235 00236 //write low fuse bit 00237 lcd.cls(); 00238 lcd.locate(0, 0); 00239 lcd.printf("Writing Low Fuse"); 00240 avrspi.write(0xAC); 00241 avrspi.write(0xA0); 00242 avrspi.write(0x00); 00243 avrspi.write(0xE2); 00244 wait_ms(5); 00245 //poll 00246 do { 00247 avrspi.write(0xF0); 00248 avrspi.write(0x00); 00249 avrspi.write(0x00); 00250 response = avrspi.write(0x00); 00251 } while ((response & 0x01) != 0); 00252 //end poll 00253 lcd.locate(10, 1); 00254 lcd.printf("DONE"); 00255 wait(1); 00256 00257 //write high fuse bit 00258 lcd.cls(); 00259 lcd.locate(0, 0); 00260 lcd.printf("Writing Hi Fuse"); 00261 avrspi.write(0xAC); 00262 avrspi.write(0xA8); 00263 avrspi.write(0x00); 00264 avrspi.write(0xDA); 00265 wait_ms(5); 00266 //poll 00267 do { 00268 avrspi.write(0xF0); 00269 avrspi.write(0x00); 00270 avrspi.write(0x00); 00271 response = avrspi.write(0x00); 00272 } while ((response & 0x01) != 0); 00273 //end poll 00274 lcd.locate(10, 1); 00275 lcd.printf("DONE"); 00276 wait(1); 00277 00278 //write ext fuse bit 00279 lcd.cls(); 00280 lcd.locate(0, 0); 00281 lcd.printf("Writing Ext Fuse"); 00282 avrspi.write(0xAC); 00283 avrspi.write(0xA4); 00284 avrspi.write(0x00); 00285 avrspi.write(0x05); 00286 wait_ms(5); 00287 //poll 00288 do { 00289 avrspi.write(0xF0); 00290 avrspi.write(0x00); 00291 avrspi.write(0x00); 00292 response = avrspi.write(0x00); 00293 } while ((response & 0x01) != 0); 00294 //end poll 00295 lcd.locate(10, 1); 00296 lcd.printf("DONE"); 00297 wait(1); 00298 00299 // exit program mode 00300 avrrst = 1; 00301 // end 00302 return 0; 00303 } 00304
Generated on Wed Jul 13 2022 06:04:59 by
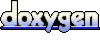