
tmeperature to mobile phone
Dependencies: BLE_API MPU6050 mbed nRF51822
Fork of BLE_HeartRate by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "nRF51822n.h" 00019 #include "MPU6050.h" 00020 00021 nRF51822n nrf; /* BLE radio driver */ 00022 00023 Serial pc(USBTX, USBRX); 00024 MPU6050 mpu(P0_22, P0_20); 00025 00026 /* Battery Level Service */ 00027 uint8_t batt = 88; /* Battery level */ 00028 00029 GattService battService (GattService::UUID_BATTERY_SERVICE); 00030 GattCharacteristic battLevel (GattCharacteristic::UUID_BATTERY_LEVEL_CHAR, 00031 1, 00032 1, 00033 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY | 00034 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00035 00036 GattService tempService (GattService::UUID_HEALTH_THERMOMETER_SERVICE); 00037 GattCharacteristic tempLevel (GattCharacteristic::UUID_TEMPERATURE_MEASUREMENT_CHAR, 00038 2, 00039 3, 00040 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00041 GattCharacteristic tempLocation ( 00042 GattCharacteristic::UUID_BODY_SENSOR_LOCATION_CHAR, 00043 1, 00044 1, 00045 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00046 00047 /* Heart Rate Service */ 00048 /* Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.heart_rate.xml */ 00049 /* HRM Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.heart_rate_measurement.xml */ 00050 /* Location: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.body_sensor_location.xml */ 00051 GattService hrmService (GattService::UUID_HEART_RATE_SERVICE); 00052 GattCharacteristic hrmRate ( 00053 GattCharacteristic::UUID_HEART_RATE_MEASUREMENT_CHAR, 00054 2, 00055 3, 00056 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00057 GattCharacteristic hrmLocation ( 00058 GattCharacteristic::UUID_BODY_SENSOR_LOCATION_CHAR, 00059 1, 00060 1, 00061 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00062 00063 /* Device Information service */ 00064 uint8_t deviceName[] = {'Y', 'o', 'u','Y', 'o', 'u'}; 00065 GattService deviceInformationService ( 00066 GattService::UUID_DEVICE_INFORMATION_SERVICE); 00067 GattCharacteristic deviceManufacturer ( 00068 GattCharacteristic::UUID_MANUFACTURER_NAME_STRING_CHAR, 00069 sizeof(deviceName), 00070 sizeof(deviceName), 00071 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00072 uint8_t version[]={'Y', 'o', 'u','Y', 'o', 'u',' ','1','.','0'}; 00073 GattCharacteristic firmwareVersion ( 00074 GattCharacteristic::UUID_FIRMWARE_REVISION_STRING_CHAR, 00075 sizeof(version), 00076 sizeof(version), 00077 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00078 00079 /* Advertising data and parameters */ 00080 GapAdvertisingData advData; 00081 GapAdvertisingData scanResponse; 00082 GapAdvertisingParams advParams (GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00083 uint16_t uuid16_list[] = {GattService::UUID_BATTERY_SERVICE, 00084 GattService::UUID_DEVICE_INFORMATION_SERVICE, 00085 GattService::UUID_HEART_RATE_SERVICE, 00086 GattService::UUID_HEALTH_THERMOMETER_SERVICE}; 00087 00088 /**************************************************************************/ 00089 /*! 00090 @brief This custom class can be used to override any GapEvents 00091 that you are interested in handling on an application level. 00092 */ 00093 /**************************************************************************/ 00094 class GapEventHandler : public GapEvents 00095 { 00096 virtual void onTimeout(void) 00097 { 00098 pc.printf("Advertising Timeout!\r\n"); 00099 // Restart the advertising process with a much slower interval, 00100 // only start advertising again after a button press, etc. 00101 } 00102 00103 virtual void onConnected(void) 00104 { 00105 pc.printf("Connected!\r\n"); 00106 } 00107 00108 virtual void onDisconnected(void) 00109 { 00110 pc.printf("Disconnected!\r\n"); 00111 pc.printf("Restarting the advertising process\r\n"); 00112 nrf.getGap().startAdvertising(advParams); 00113 } 00114 }; 00115 00116 /**************************************************************************/ 00117 /*! 00118 @brief This custom class can be used to override any GattServerEvents 00119 that you are interested in handling on an application level. 00120 */ 00121 /**************************************************************************/ 00122 class GattServerEventHandler : public GattServerEvents 00123 { 00124 //virtual void onDataSent(uint16_t charHandle) {} 00125 //virtual void onDataWritten(uint16_t charHandle) {} 00126 00127 virtual void onUpdatesEnabled(uint16_t charHandle) 00128 { 00129 if (charHandle == hrmRate.getHandle()) { 00130 pc.printf("Heart rate notify enabled\r\n"); 00131 } 00132 if (charHandle == tempLevel.getHandle()) { 00133 pc.printf("Temperature notify enabled\r\n"); 00134 } 00135 } 00136 00137 virtual void onUpdatesDisabled(uint16_t charHandle) 00138 { 00139 if (charHandle == hrmRate.getHandle()) { 00140 pc.printf("Heart rate notify disabled\r\n"); 00141 } 00142 if (charHandle == tempLevel.getHandle()) { 00143 pc.printf("Temperature notify disabled\r\n"); 00144 } 00145 } 00146 00147 //virtual void onConfirmationReceived(uint16_t charHandle) {} 00148 }; 00149 00150 /**************************************************************************/ 00151 /*! 00152 @brief Program entry point 00153 */ 00154 /**************************************************************************/ 00155 int main(void) 00156 { 00157 float accdata[3]; 00158 uint16_t time=0; 00159 wait(1); 00160 pc.baud(38400); 00161 00162 /* Setup the local GAP/GATT event handlers */ 00163 nrf.getGap().setEventHandler(new GapEventHandler()); 00164 nrf.getGattServer().setEventHandler(new GattServerEventHandler()); 00165 00166 /* Initialise the nRF51822 */ 00167 pc.printf("Initialising the nRF51822...\r\n"); 00168 nrf.init(); 00169 00170 /* Make sure we get a clean start */ 00171 nrf.reset(); 00172 00173 /* Add BLE-Only flag and complete service list to the advertising data */ 00174 advData.addFlags(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00175 advData.addData(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, 00176 (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00177 advData.addAppearance(GapAdvertisingData::HEART_RATE_SENSOR_HEART_RATE_BELT); 00178 nrf.getGap().setAdvertisingData(advData, scanResponse); 00179 00180 /* Add the Battery Level service */ 00181 battService.addCharacteristic(battLevel); 00182 nrf.getGattServer().addService(battService); 00183 00184 /* Add the Device Information service */ 00185 deviceInformationService.addCharacteristic(deviceManufacturer); 00186 deviceInformationService.addCharacteristic(firmwareVersion); 00187 nrf.getGattServer().addService(deviceInformationService); 00188 00189 /* Add the Heart Rate service */ 00190 hrmService.addCharacteristic(hrmRate); 00191 hrmService.addCharacteristic(hrmLocation); 00192 nrf.getGattServer().addService(hrmService); 00193 00194 tempService.addCharacteristic(tempLevel); 00195 tempService.addCharacteristic(tempLocation); 00196 nrf.getGattServer().addService(tempService); 00197 00198 /* Start advertising (make sure you've added all your data first) */ 00199 nrf.getGap().startAdvertising(advParams); 00200 00201 00202 mpu.setFrequency(100000); 00203 wait_ms(10); 00204 if (mpu.testConnection()) 00205 { 00206 pc.printf("MPU connection succeeded!\r\n"); 00207 } 00208 else 00209 { 00210 pc.printf("MPU connection failed!\r\n"); //Todo: If connection fails, retry a couple times. Try resetting MPU (this would need another wire?) 00211 } 00212 wait(2); 00213 mpu.setSleepMode(false); 00214 mpu.setBW(MPU6050_BW_20); 00215 mpu.setGyroRange(MPU6050_GYRO_RANGE_500); 00216 mpu.setAcceleroRange(MPU6050_ACCELERO_RANGE_2G); 00217 00218 00219 /* Wait until we are connected to a central device before updating 00220 * anything */ 00221 pc.printf("Waiting for a connection ...\r\n"); 00222 while (!nrf.getGap().state.connected) { 00223 } 00224 wait(3); 00225 00226 pc.printf("Connected!\r\n"); 00227 00228 /* Now that we're live, update the battery level characteristic, and */ 00229 /* change the device manufacturer characteristic to 'mbed' */ 00230 nrf.getGattServer().updateValue(battLevel.getHandle(), (uint8_t *)&batt, 00231 sizeof(batt)); 00232 nrf.getGattServer().updateValue(deviceManufacturer.getHandle(), 00233 deviceName, 00234 sizeof(deviceName)); 00235 nrf.getGattServer().updateValue(firmwareVersion.getHandle(), 00236 version, 00237 sizeof(version)); 00238 00239 /* Set the heart rate monitor location (one time only) */ 00240 /* See --> https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.body_sensor_location.xml */ 00241 uint8_t location = 0x03; /* Finger */ 00242 uint8_t hrmCounter = 100; 00243 nrf.getGattServer().updateValue(hrmLocation.getHandle(), 00244 (uint8_t *)&location, 00245 sizeof(location)); 00246 nrf.getGattServer().updateValue(tempLocation.getHandle(), 00247 (uint8_t *)&location, 00248 sizeof(location)); 00249 00250 00251 for (;; ) 00252 { 00253 wait(1); 00254 00255 /* Update battery level 00256 batt++; 00257 if (batt > 100) { 00258 batt = 72; 00259 } 00260 nrf.getGattServer().updateValue(battLevel.getHandle(), 00261 (uint8_t *)&batt, 00262 sizeof(batt)); */ 00263 00264 /* Update the HRM measurement */ 00265 /* First byte = 8-bit values, no extra info, Second byte = uint8_t HRM value */ 00266 /* See --> https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.heart_rate_measurement.xml */ 00267 hrmCounter++; 00268 if (hrmCounter == 175) { 00269 hrmCounter = 100; 00270 } 00271 uint8_t bpm[2] = {0x00, hrmCounter}; 00272 nrf.getGattServer().updateValue(hrmRate.getHandle(), bpm, sizeof(bpm)); 00273 00274 float f =mpu.getTemp(); 00275 int t = f; 00276 uint8_t temper[2]; 00277 temper[0]=t>>8; 00278 temper[1]=t; 00279 nrf.getGattServer().updateValue(tempLevel.getHandle(), temper, sizeof(temper)); 00280 00281 pc.printf("HRM:"); 00282 pc.printf("%d",hrmCounter); 00283 pc.printf(" Temperature:"); 00284 pc.printf("%.2f",f); 00285 pc.printf(" "); 00286 mpu.getAccelero(accdata); 00287 time++; 00288 pc.printf("X:%.2f Y:%.2f Z:%.2f Time:%ds\r\n",accdata[0], accdata[1], accdata[2],time); 00289 } 00290 }
Generated on Thu Jul 14 2022 14:47:14 by
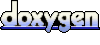