An interrupt-driven interface to 4x4 keypad.
Dependents: FYPFinalProgram FYPFinalizeProgram KEYS Proyect_Patric_electronic_door_MSC_Ok_ESP ... more
FPointer Class Reference
FPointer - Adds callbacks that take and return a 32bit uint32_t data type. More...
#include <FPointer.h>
Public Member Functions | |
FPointer () | |
Constructor. | |
void | attach (uint32_t(*function)(uint32_t)=0) |
attach - Overloaded attachment function. | |
template<class T > | |
void | attach (T *item, uint32_t(T::*method)(uint32_t)) |
attach - Overloaded attachment function. | |
uint32_t | call (uint32_t arg) |
call - Overloaded callback initiator. | |
uint32_t | call (void) |
call - Overloaded callback initiator. | |
Protected Attributes | |
uint32_t(* | c_callback )(uint32_t) |
C callback function pointer. | |
FPointerDummy * | obj_callback |
C++ callback object/method pointer (the object part). | |
uint32_t(FPointerDummy::* | method_callback )(uint32_t) |
C++ callback object/method pointer (the method part). |
Detailed Description
FPointer - Adds callbacks that take and return a 32bit uint32_t data type.
The Mbed library supplies a callback using the FunctionPointer object as defined in FunctionPointer.h However, this callback system does not allow the caller to pass a value to the callback. Likewise, the callback itself cannot return a value.
FPointer operates in the same way but allows the callback function to be passed one arg, a uint32_t value. Additionally, the callback can return a single uint32_t value. The reason for using uint32_t is that the Mbed and the microcontroller (LPC1768) have a natural data size of 32bits and this means we can use the uint32_t as a pointer. See example1.h for more information. This example passes an "int" by passing a pointer to that int as a 32bit value. Using this technique you can pass any value you like. All you have to do is pass a pointer to your value cast to (uint32_t). Your callback can the deference it to get the original value.
example2.h shows how to do the same thing but demostrates how to specify the callback into a class object/method.
Finally, example3.h shows how to pass multiple values. In this example we define a data structure and in the callback we pass a pointer to that data structure thus allowing the callback to again get the values.
Note, when passing pointers to variables to the callback, if the callback function/method changes that variable's value then it will also change the value the caller sees. If C pointers are new to you, you are strongly advised to read up on the subject. It's pointers that often get beginners into trouble when mis-used.
- See also:
- example1.h
- example2.h
- example3.h
- http://mbed.org/handbook/C-Data-Types
- http://mbed.org/projects/libraries/svn/mbed/trunk/FunctionPointer.h
Definition at line 66 of file FPointer.h.
Constructor & Destructor Documentation
FPointer | ( | ) |
Constructor.
Definition at line 83 of file FPointer.h.
Member Function Documentation
void attach | ( | uint32_t(*)(uint32_t) | function = 0 ) |
attach - Overloaded attachment function.
Attach a C type function pointer as the callback.
Note, the callback function prototype must be:-
uint32_t myCallbackFunction(uint32_t);
- Parameters:
-
A C function pointer to call.
Definition at line 99 of file FPointer.h.
void attach | ( | T * | item, |
uint32_t(T::*)(uint32_t) | method | ||
) |
attach - Overloaded attachment function.
Attach a C++ type object/method pointer as the callback.
Note, the callback method prototype must be:-
public:
uint32_t myCallbackFunction(uint32_t);
- Parameters:
-
A C++ object pointer. A C++ method within the object to call.
Definition at line 114 of file FPointer.h.
uint32_t call | ( | uint32_t | arg ) |
call - Overloaded callback initiator.
call the callback function.
- Parameters:
-
uint32_t The value to pass to the callback.
- Returns:
- uint32_t The value the callback returns.
Definition at line 126 of file FPointer.h.
uint32_t call | ( | void | ) |
call - Overloaded callback initiator.
Call the callback function without passing an argument. The callback itself is passed NULL. Note, the callback prototype should still be uint32_t callback(uint32_t).
- Returns:
- uint32_t The value the callback returns.
Definition at line 146 of file FPointer.h.
Field Documentation
uint32_t(* c_callback)(uint32_t) [protected] |
C callback function pointer.
Definition at line 71 of file FPointer.h.
uint32_t(FPointerDummy::* method_callback)(uint32_t) [protected] |
C++ callback object/method pointer (the method part).
Definition at line 77 of file FPointer.h.
FPointerDummy* obj_callback [protected] |
C++ callback object/method pointer (the object part).
Definition at line 74 of file FPointer.h.
Generated on Tue Jul 12 2022 19:57:39 by
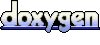