fix nrf51822 i2c & spi conflict
Dependencies: BLE_API eMPL_MPU6050 nRF51822
Fork of Seeed_Tiny_BLE_Flash by
W25Q16BV.h
00001 // W25Q16BV.h 00002 00003 #ifndef W25Q16BV_H 00004 #define W25Q16BV_H 00005 00006 #include "mbed.h" 00007 //#include "BitBangedSPI.h" 00008 00009 #define SPI_FREQ 1000000 00010 #define SPI_MODE 0 00011 #define SPI_NBIT 8 00012 00013 #define POWERUP_INST 0xAB 00014 #define STATUS1_INST 0x05 00015 #define STATUS2_INST 0x35 00016 #define JDEC_INST 0x9F 00017 #define UNIQUE_INST 0x4B 00018 #define WE_INST 0x06 00019 #define WD_INST 0x04 00020 #define R_INST 0x03 00021 #define W_INST 0x02 00022 #define S_ERASE_INST 0x20 /* 4KB sector erase */ 00023 #define B_ERASE_INST 0xD8 /* 64KB block erase */ 00024 #define C_ERASE_INST 0x60 00025 00026 #define DUMMY_ADDR 0x00 00027 00028 #define WAIT_US_TRES1 5 /* Power Up: 3us */ 00029 //#define WAIT_US_TPUW 10000 /* Power Up Write Time: 1-10ms */ 00030 //#define WAIT_US_TBP 50 /* Byte Program Time: 20-50us */ 00031 //#define WAIT_US_TPP 3000 /* Page Program Time: 0.7-3ms */ 00032 //#define WAIT_US_TSE 400000 /* Sector Erase Time: 30-400ms */ 00033 //#define WAIT_US_TBE 1000000 /* 64KB Block Erase Time: 1000ms */ 00034 //#define WAIT_US_TCE 10000000 /* Chip Erase Time: 3-10s */ 00035 00036 //#define ADDR_BMASK2 0x00ff0000 00037 //#define ADDR_BMASK1 0x0000ff00 00038 //#define ADDR_BMASK0 0x000000ff 00039 00040 //#define ADDR_BSHIFT2 16 00041 //#define ADDR_BSHIFT1 8 00042 //#define ADDR_BSHIFT0 0 00043 00044 #define PAGE_SIZE 256 00045 #define SECTOR_SIZE 4096 00046 #define NUM_SECTORS 512 00047 #define NUM_64KB_BLOCKS 32 00048 00049 #define STATUS_1_BUSY 0x01 00050 00051 class W25Q16BV /*: public BitBangedSPI*/ { 00052 public: 00053 W25Q16BV(PinName mosi, PinName miso, PinName sclk, PinName cs); 00054 00055 int readByte(int addr); // takes a 24-bit (3 bytes) address and returns the data (1 byte) at that location 00056 int readByte(int a2, int a1, int a0); // takes the address in 3 separate bytes A[23,16], A[15,8], A[7,0] 00057 void readStream(int addr, char* buf, int count); // takes a 24-bit address, reads count bytes, and stores results in buf 00058 00059 void readJEDEC(uint8_t* manId, uint8_t* memType, uint8_t* cap); 00060 uint8_t readStatus1(); 00061 uint8_t readStatus2(); 00062 00063 void writeByte(int addr, int data); // takes a 24-bit (3 bytes) address and a byte of data to write at that location 00064 void writeByte(int a2, int a1, int a0, int data); // takes the address in 3 separate bytes A[23,16], A[15,8], A[7,0] 00065 void writeStream(int addr, char* buf, int count); // write count bytes of data from buf to memory, starting at addr 00066 00067 void chipErase(); // erase all data on chip 00068 bool blockErase(int startBlock, int num=1); // erase all data in the specified number of 64KB blocks, return false if block number is invalid 00069 bool sectorErase(int startSector, int num=1); // erase all data in the specified number of 4KB sectors, return false if sector number is invalid 00070 00071 private: 00072 00073 void exitDeepPowerDown(); 00074 void waitWhileBusy(); 00075 00076 void writeEnable(); // write enable 00077 void writeDisable(); // write disable 00078 void chipEnable(); // chip enable 00079 void chipDisable(); // chip disable 00080 00081 // BitBangedSPI _spi; 00082 SPI _spi; 00083 DigitalOut _cs; 00084 }; 00085 00086 #endif 00087
Generated on Tue Jul 12 2022 14:43:28 by
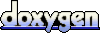